In C++, you can instantiate a class by creating an object of that class, which allocates memory for it and allows you to access its members and methods.
Here's a simple code snippet demonstrating class instantiation:
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass obj; // Instantiate the class
obj.display(); // Call method on the object
return 0;
}
What is a Class in C++?
Definition of a Class
In C++, a class is a blueprint for creating objects. A class encapsulates data for the object and methods to manipulate that data. This encapsulation is a fundamental characteristic of object-oriented programming, allowing developers to model real-world entities and behaviors efficiently. Classes provide reusability and modularity, making code easier to manage and maintain.
Syntax of Class Declaration
To declare a class in C++, you use the following format:
class ClassName {
public:
// Member variables
// Member functions
};
This defines a new data type, `ClassName`, which can contain both attributes (also known as member variables) and behaviors (member functions).
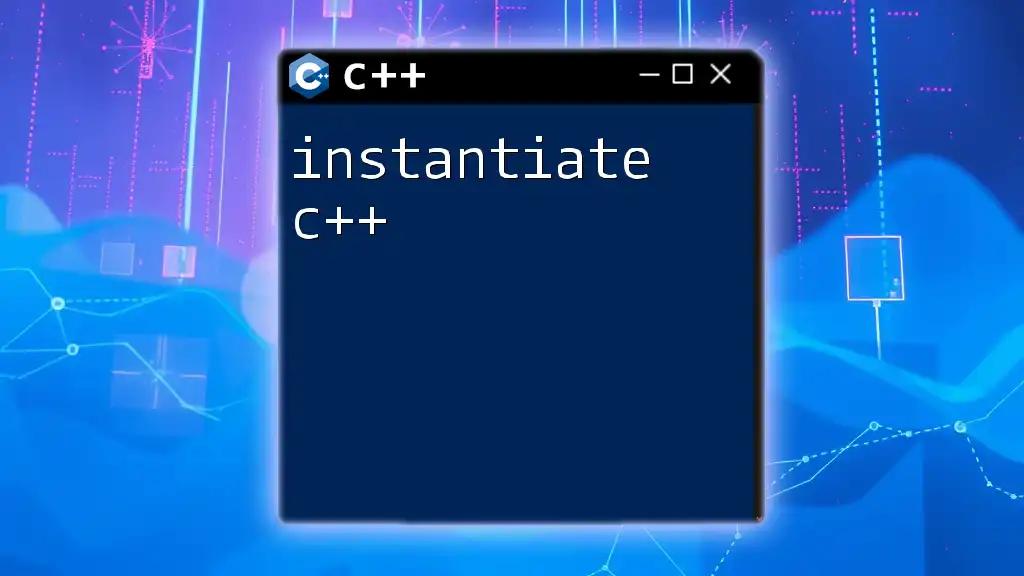
Understanding Class Instantiation in C++
What is Class Instantiation?
Class instantiation refers to the process of creating an object from a class. When you instantiate a class, you're allocating memory for the object and setting it up according to the class's specifications. This process is crucial in C++ because it bridges the gap between defining a class and using it in programs.
Why Instantiation is Important
Instantiation is vital for various reasons:
- Memory Allocation: When you instantiate a class, C++ allocates the needed memory to hold the object.
- Encapsulation: Creating instances of classes enables encapsulating data with functions that operate on that data, fostering better organization.
- Reuse: You can instantiate multiple objects from the same class, enabling code reusability without the need to replicate code for each object.

How to Instantiate a Class in C++
Basic Syntax to Instantiate a Class
To create an object from a class, you use the following syntax:
ClassName objectName;
This line declares a variable `objectName` of type `ClassName`, effectively creating an instance of the class.
Instantiating Using Constructors
Constructors are special member functions called when an object is created, allowing for initial setup.
Default Constructor
A default constructor is one that takes no parameters. It initializes members to default values.
class MyClass {
public:
MyClass() {
// Constructor implementation
std::cout << "Default constructor called!" << std::endl;
}
};
MyClass obj; // Calls the default constructor
Parameterized Constructor
A parameterized constructor allows you to initialize an object with specific values.
class MyClass {
public:
int value;
MyClass(int val) : value(val) {
// Constructor implementation
}
};
MyClass obj(10); // Calls parameterized constructor with value 10
Copy Constructor
A copy constructor creates a new object as a copy of an existing object.
class MyClass {
public:
int value;
MyClass(int val) : value(val) {}
MyClass(const MyClass &obj) : value(obj.value) {
// Copy constructor implementation
}
};
MyClass obj1(20);
MyClass obj2 = obj1; // Calls copy constructor

Different Ways to Instantiate Classes in C++
Stack vs Heap Allocation
In C++, there are two primary ways to allocate memory and instantiate classes—on the stack and the heap.
- Stack Allocation: Objects created on the stack have automatic storage duration. When the function they belong to ends, they are destroyed automatically.
MyClass obj; // Stack allocation
- Heap Allocation: Objects created on the heap using the `new` keyword persist until explicitly deleted, providing more control over their lifespan.
MyClass* obj = new MyClass(); // Heap allocation
Destroying the Instance
When you instantiate a class on the heap, proper memory management is essential. You should free the allocated memory to avoid memory leaks:
delete obj; // Frees the memory allocated for obj
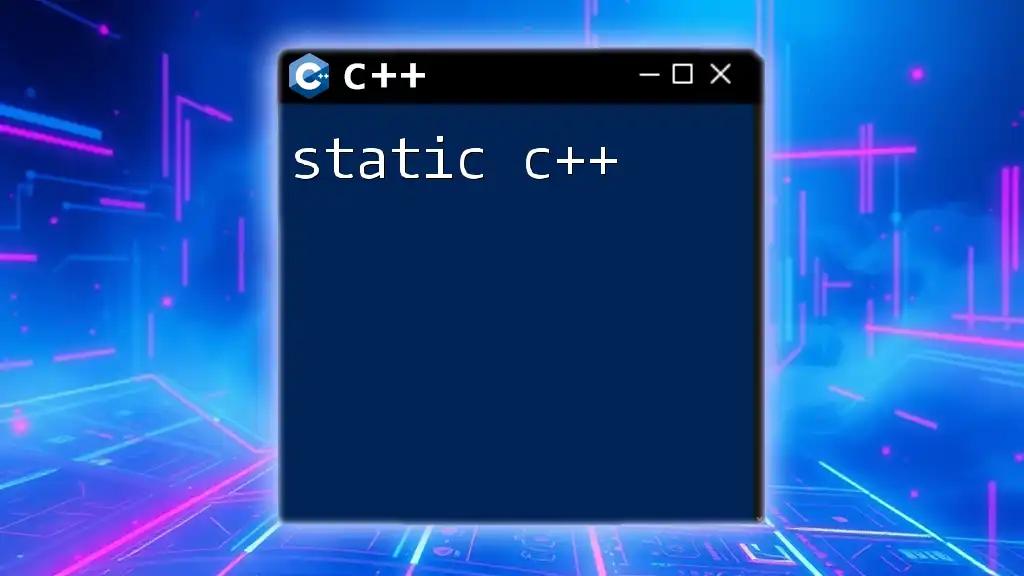
Advanced Topics in Class Instantiation
Instantiation of Abstract Classes and Interfaces
Abstract classes cannot be instantiated directly; instead, they serve as base classes for derived classes. A derived class must implement all pure virtual functions from the abstract class.
class AbstractClass {
public:
virtual void myMethod() = 0; // Pure virtual function
};
class DerivedClass : public AbstractClass {
public:
void myMethod() override {
// Implementation of the abstract method
}
};
AbstractClass* obj = new DerivedClass(); // Instantiate via derived class
Instantiation in Multithreaded Applications
In multithreading, care must be taken when instantiating classes. Thread safety is crucial, particularly when multiple threads might try to access or modify the same instance simultaneously. Use mutexes or other locking mechanisms to ensure safe instantiation and modification of class instances.

Best Practices for Class Instantiation in C++
Consistency and Naming Conventions
Maintain naming conventions for classes and objects to improve readability. Consistent naming helps identify the purpose and functionality of your code quickly.
Memory Management and Cleanup
Always ensure that dynamic instances are properly cleaned up after use. This practice prevents memory leaks and is fundamental in managing resources effectively.

Conclusion
Understanding how to effectively instantiate a class in C++ is essential for anyone looking to master object-oriented programming in C++. By grasping the nuances of constructors, memory management, and the differences between stack and heap allocation, you can create efficient, robust applications. Practice these concepts, and your skills in C++ will deepen significantly over time.
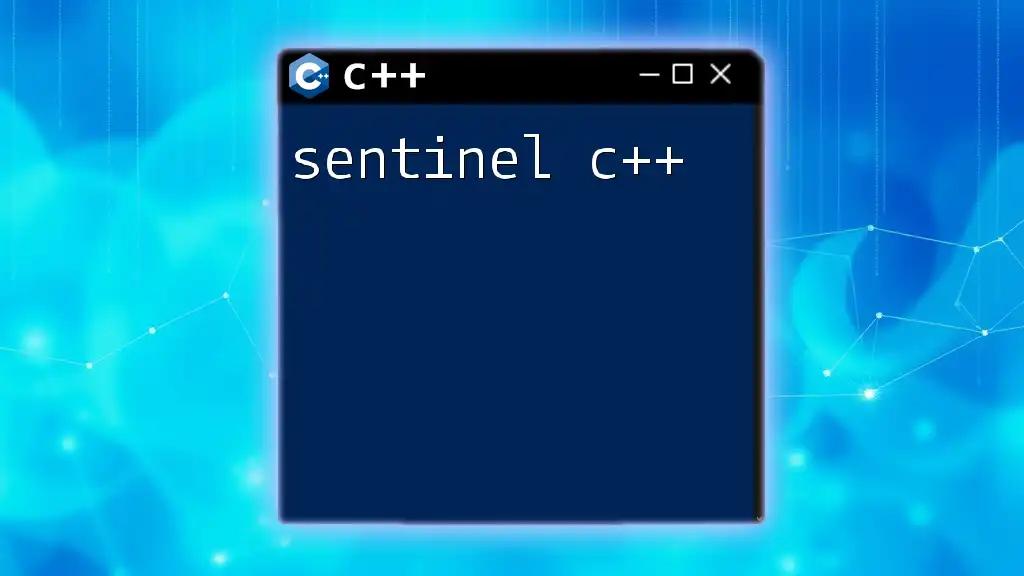
Additional Resources
For those keen to enhance their learning, consider exploring further readings, online tutorials, or video lectures focused on C++ class instantiation and best practices in object-oriented programming.