A code runner for C++ allows you to execute C++ programs instantly without the need for complex setups, making it ideal for quick testing and learning.
Here’s a simple code snippet that prints "Hello, World!" in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Code Runner
A C++ Code Runner is a versatile tool that allows programmers to execute their C++ code snippets quickly and efficiently. It simplifies the coding process, enabling users to test and debug their code without the need for a full Integrated Development Environment (IDE). Learning how to utilize a code runner can significantly enhance your coding productivity and learning curve.
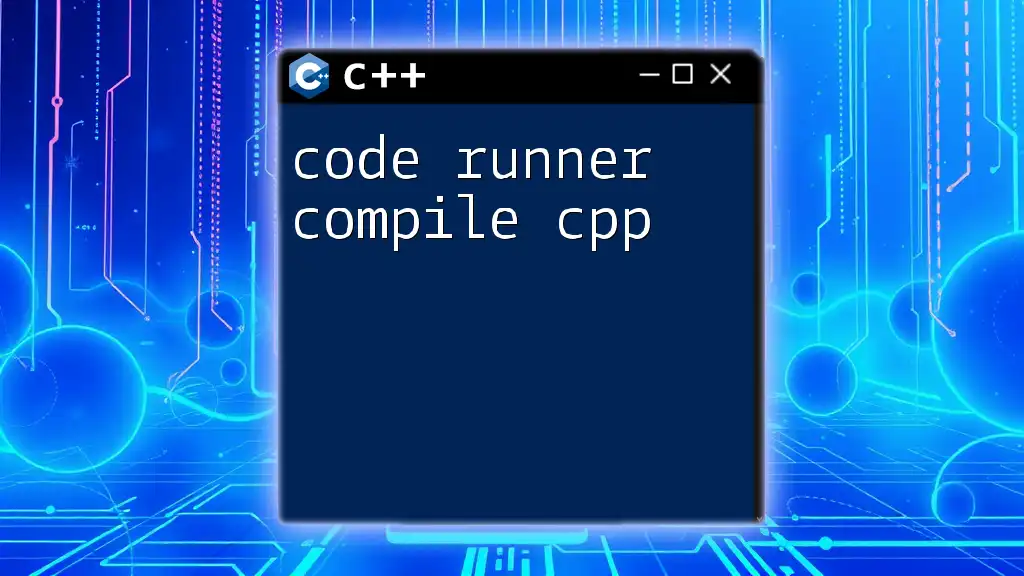
Setting Up Your C++ Code Runner Environment
Choosing a C++ Code Runner
There are various C++ code runners available in the market, each offering unique features and benefits. Some popular options include:
- Online C++ Compilers like repl.it and ideone.com, which require no installation.
- Desktop Applications such as Code::Blocks and Dev-C++ that allow local execution and debugging.
When selecting a code runner, consider the user interface, additional features like debugging support, and community reviews.
Installation Steps
Once you've chosen a code runner, follow these straightforward installation steps:
- For Online Compilers: No installation is needed. Simply visit the website and start coding.
- For Desktop Applications: Download the installer from the official website, run the installer, and follow the on-screen instructions for your specific operating system (Windows, macOS, or Linux).
To optimize your environment, ensure that your system meets the application's hardware and software requirements.
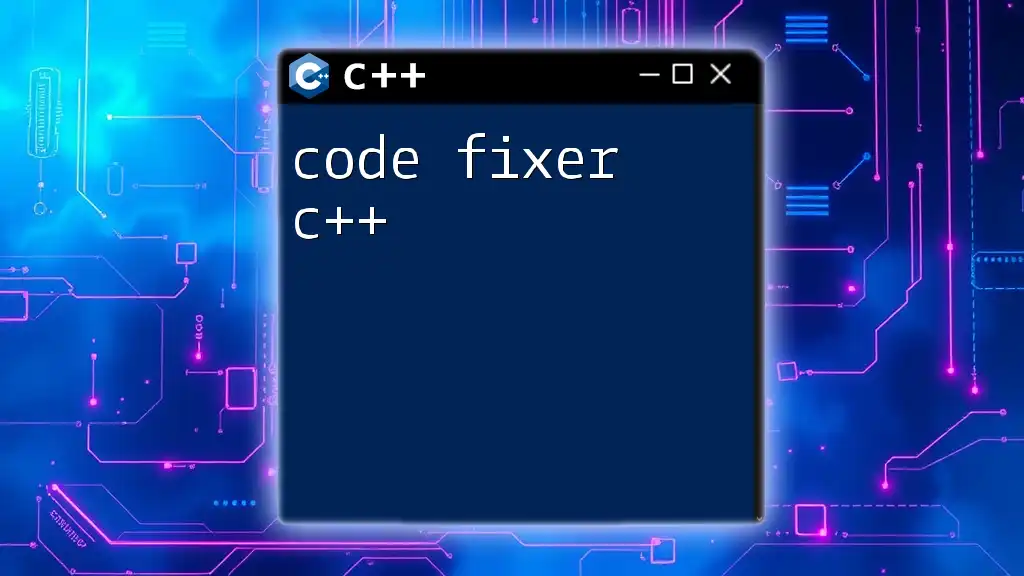
Basic Features of a Code Runner
Running C++ Code
The primary function of a C++ Code Runner is to execute basic C++ code. The process is typically straightforward:
- Write your C++ code in the editor.
- Click on the Run button (or similar) to execute your program.
Consider this simple example of a "Hello World" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This code demonstrates the program's basic functionality, where it includes the `iostream` library and utilizes the `cout` object to print a message to the console.
Compiling C++ Code
Understanding the compilation process is crucial for using any C++ code runner. Compiling transforms your C++ code into machine code that the computer can execute.
Typically, the code runner automatically compiles your code when you hit the Run button. Note the distinction between compilation and execution:
- Compilation: The process of converting C++ source code into an executable file.
- Execution: Running the compiled program to see the output.
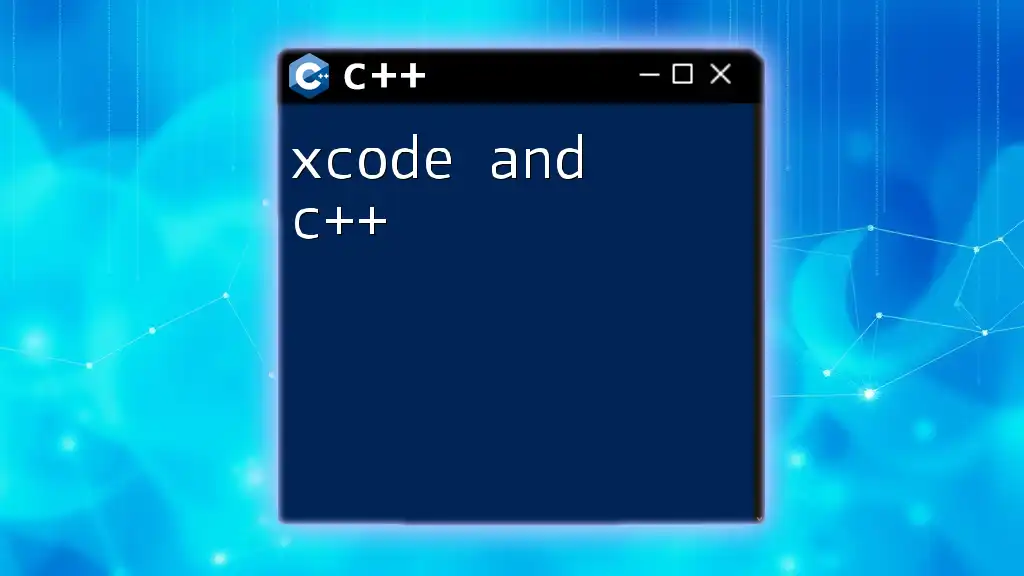
Advanced Features of C++ Code Runners
Debugging Capabilities
Debugging is an essential feature that helps you identify and fix errors in your code. Many code runners provide integrated debugging tools, allowing you to step through your code, inspect variables, and set breakpoints.
Consider the following example, which contains a syntax error:
#include <iostream>
using namespace std;
int main()
cout << "Debugging Example"; // Missing semicolon
return 0;
When you attempt to run this code, a good code runner should point out where the errors exist, aiding you in quick correction.
Code Snippet Management
Managing reusable code snippets can help streamline your coding process. Some code runners allow you to save and organize your code snippets, making them easily accessible for future use.
For example, you might create a snippet for a sorting algorithm to keep it handy:
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
swap(arr[j], arr[j+1]);
}
}
}
}
This snippet can be pasted into your project to quickly implement sorting functionality.
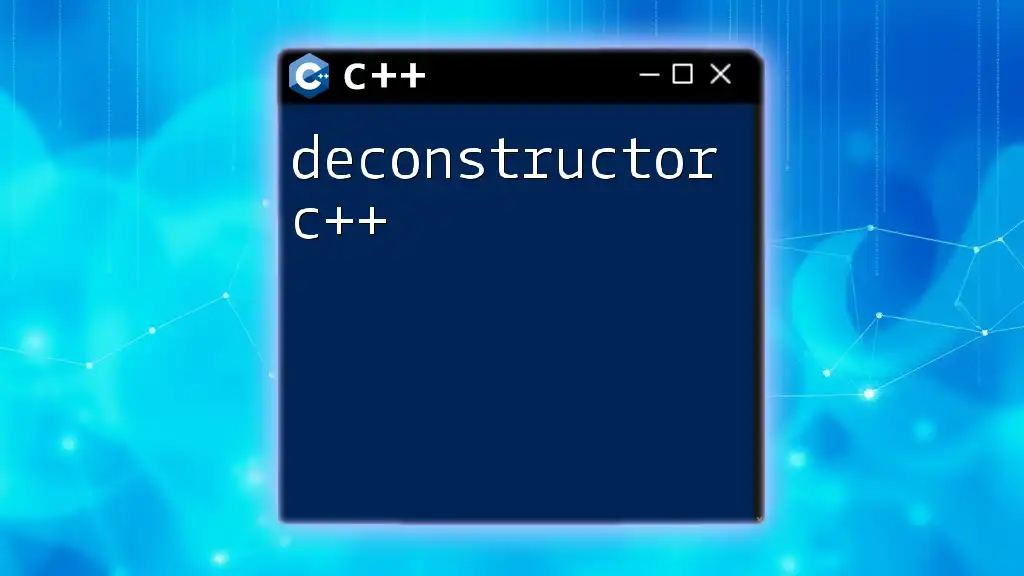
Integrating C++ Code Runners with Other Tools
Version Control Systems
Integrating your C++ code with version control systems like Git is essential for collaboration and code management. Code runners typically operate independently of version control, but you can manually initialize a Git repository.
To create a basic workflow:
- Open the terminal in the project directory.
- Run `git init` to initialize the repository.
- After making changes to your C++ files, use `git add .` to stage your changes.
- Commit your changes with `git commit -m "Your commit message"`.
This method allows you to keep track of changes and collaborate with others seamlessly.
Code Styling and Analysis Tools
Maintaining code quality is vital for readability and maintainability. Utilize code styling and analysis tools alongside your C++ code runner to ensure adherence to coding conventions.
Tools like ClangFormat can automatically format your C++ code, while CPPcheck enables static analysis to catch common pitfalls, ensuring a polished codebase.
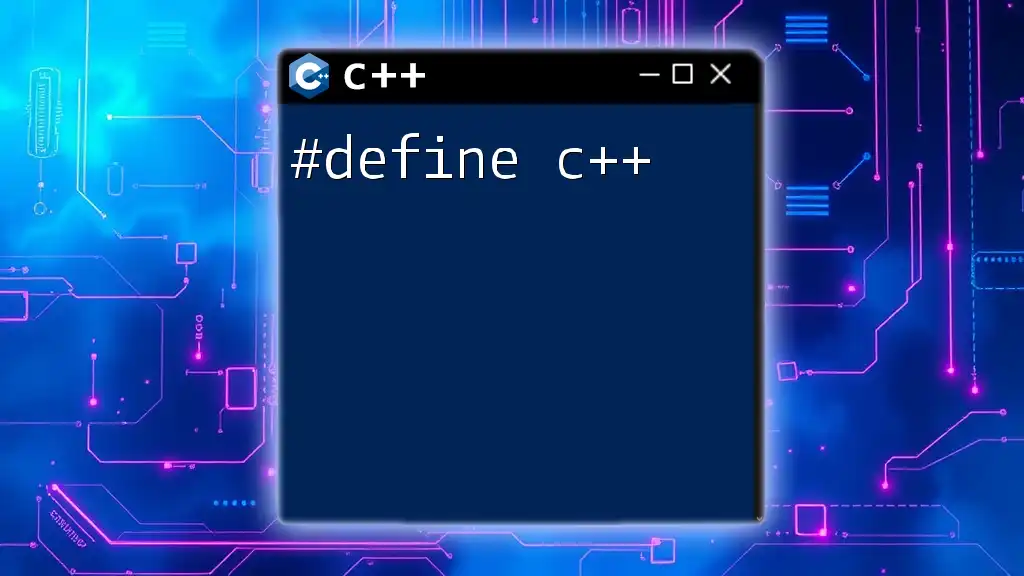
Best Practices for Using C++ Code Runners
Efficient Coding
Writing efficient C++ code not only improves performance but also enhances clarity. Keep your functions focused and concise. For instance, here’s a simple example of refactoring a function:
int add(int a, int b) {
return a + b; // Simple, clear, and efficient
}
Rather than writing unnecessarily complex code, focus on readability and maintainability.
Common Mistakes to Avoid
Beginners often encounter specific pitfalls when using C++ code runners. Here are a few common issues and troubleshooting tips:
- Missing Semicolons: Always ensure each statement ends with a semicolon.
- Mismatched Braces: Ensure every opening brace has a corresponding closing brace.
- Data Type Mismatches: Be cautious about variable types and their compatibility.
Take the time to double-check your code and use the debugging features of your code runner to quickly identify these errors.
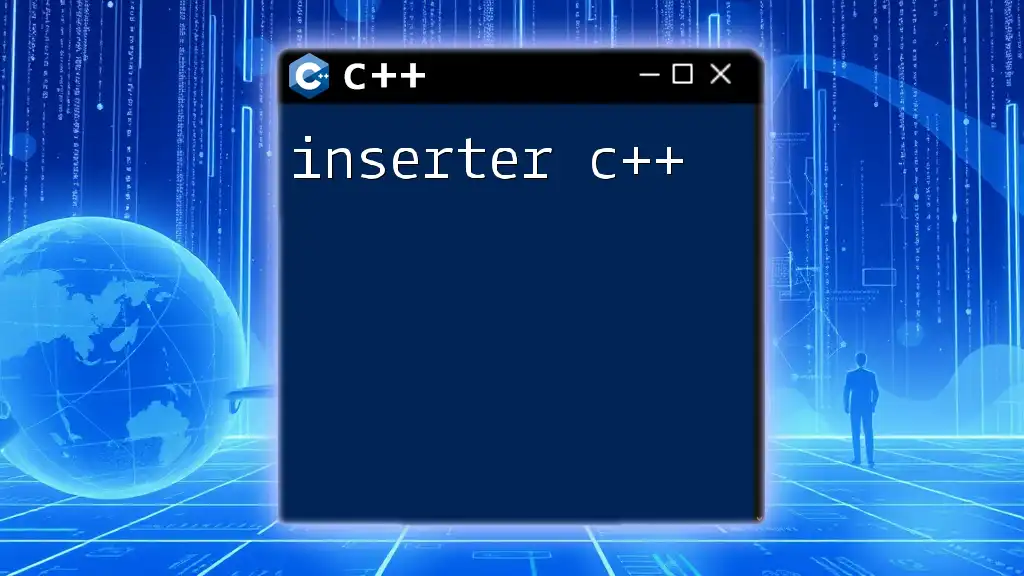
Case Studies and Use Cases
Real-Life Applications of C++ Code Runners
In various industries, C++ is a preferred language for developing efficient software solutions. From game development to system programming, code runners facilitate testing prototypes and small snippets of code.
Some notable examples include:
- Game Development: Rapidly testing gameplay mechanics during the development phase.
- Embedded Systems: Prototyping firmware for new hardware devices.
Success Stories
Many users have transitioned from novice to proficient programmers by leveraging C++ code runners. Testimonials highlight how quick execution and immediate feedback lead to a better understanding of programming concepts.
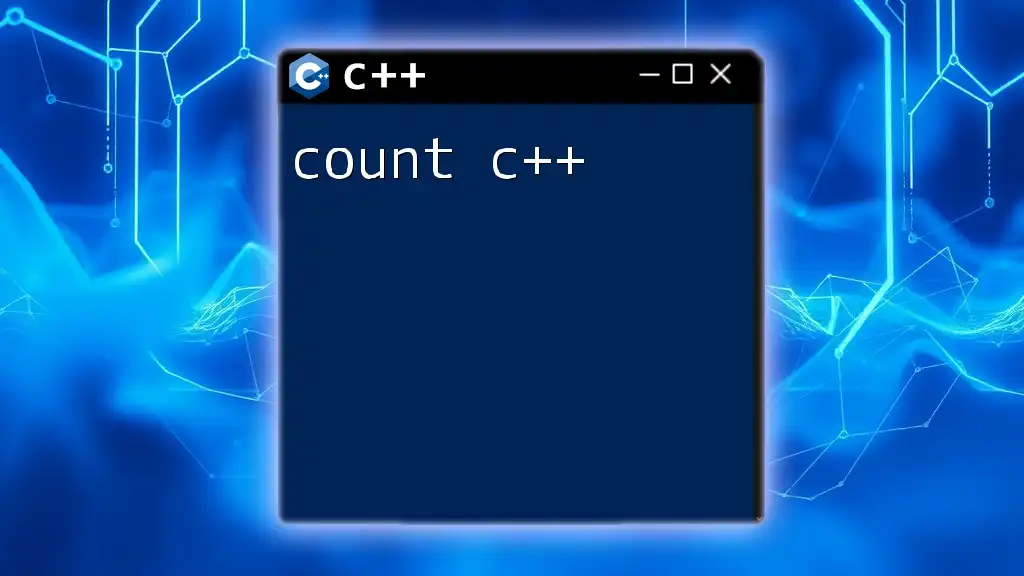
Conclusion
In summary, a C++ code runner serves as an invaluable tool for anyone looking to learn and master C++. By understanding its features, setting up your environment correctly, and adhering to best practices, you can enhance your programming skills significantly.
Encouragement for continuous learning is essential in this ever-evolving field. Utilize the vast array of available resources to deepen your understanding of C++ and coding practices.
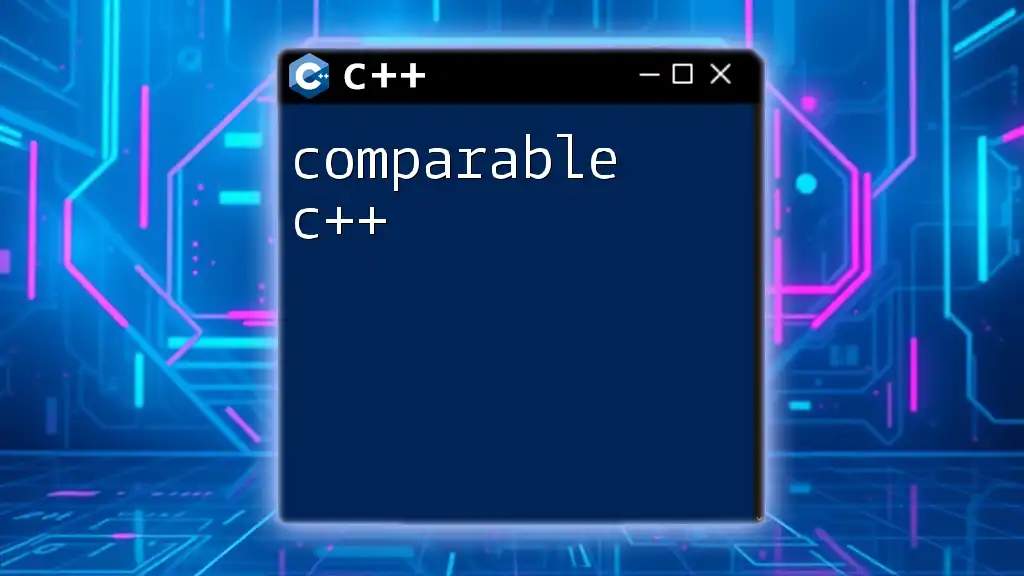
FAQs
Common Questions About C++ Code Runner
- What is the best C++ code runner for beginners? Online platforms like repl.it and ideone.com are user-friendly for beginners.
- Can I run C++ code for free? Yes, numerous online code runners allow free usage with basic features.
- How do I troubleshoot performance issues with my code runner? Ensure you have updated software and check for memory-intensive code practices.
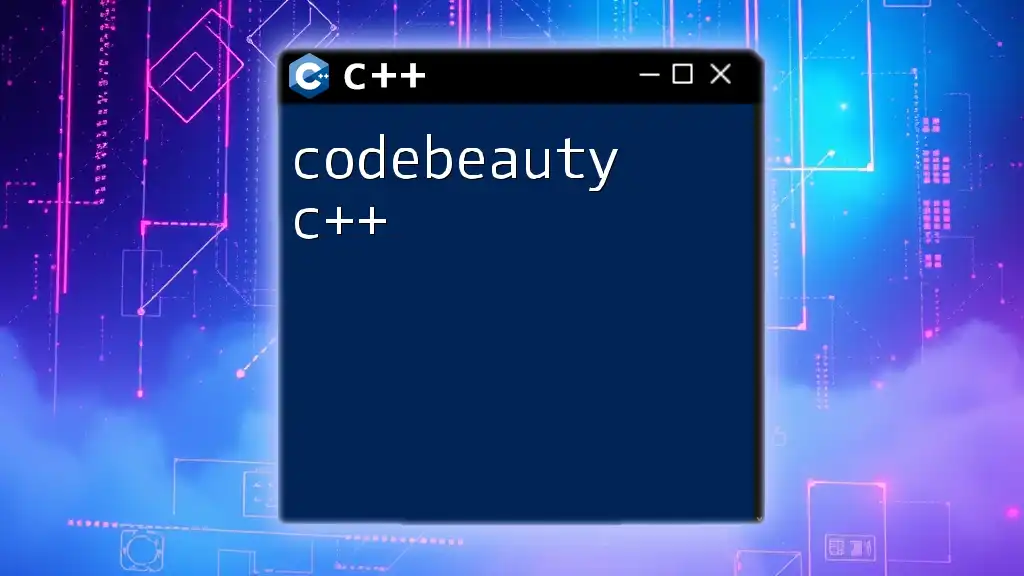
Additional Resources
Links to Tutorials and Documentation
Curated resources can prove helpful for mastering the intricacies of C++. Refer to official documentation, participate in community forums, and explore tutorial sites to keep your skills up to date.
Communities and Forums
Engage with fellow learners and experts in programming communities. Platforms like Stack Overflow and GitHub offer a wealth of information and collaborative opportunities to improve your C++ coding experience.