In C++, `emplace_back` constructs an element in place within a vector, providing potentially better performance by avoiding unnecessary copies, while `push_back` adds a copy of an object to the end of the vector.
Here’s a code snippet demonstrating the difference:
#include <vector>
#include <string>
#include <iostream>
class MyClass {
public:
MyClass(int a, const std::string& b) : x(a), y(b) {}
int x;
std::string y;
};
int main() {
std::vector<MyClass> myVec;
// Using push_back
MyClass obj1(1, "example");
myVec.push_back(obj1); // copies obj1
// Using emplace_back
myVec.emplace_back(2, "example2"); // constructs directly in-place
// Output to verify
for(const auto& obj : myVec) {
std::cout << obj.x << ": " << obj.y << std::endl;
}
return 0;
}
Understanding C++ Containers
What are C++ Containers?
In C++, containers are essential components that store collections of objects. They provide a wide range of functionalities to manage and manipulate this data effectively. Among the various kinds of containers, sequence containers such as `vector`, `list`, and `deque` are particularly noteworthy due to their dynamic memory management and ease of use.
Importance of Vector in C++
The `std::vector` is one of the most commonly used container types in C++. It allows for dynamic sizing, meaning that it can grow and shrink as needed. This flexibility makes it ideal for situations where the size of the dataset is unknown at compile time, as it can handle multiple data storage needs efficiently. The key features of `std::vector` include:
- Random access: Fast access to elements by index.
- Dynamic resizing: Can grow as needed by allocating new memory.
- Storage of arbitrary types: Can store any data type, including user-defined types.
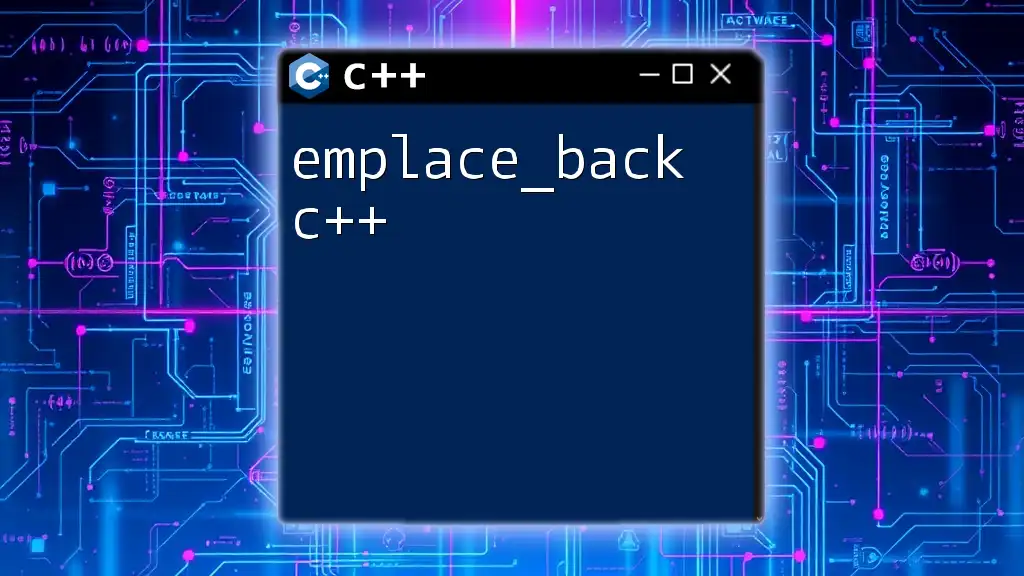
Push_back in C++
What is Push_back?
The `push_back` method is a member function of sequence containers, particularly `std::vector`. It allows you to add elements to the end of the vector, effectively expanding its size by one.
How Push_back Works
When you use `push_back`, the following takes place:
- Copying: If the object you are adding is managed by its own storage, `push_back` will copy that object into the vector's allocated space.
- Move Semantics: In C++11 and beyond, if the object being pushed back is eligible for moving (i.e., it is an rvalue), `push_back` invokes the move constructor, thus transferring ownership of the resources without creating costly copies.
Here’s a basic example demonstrating the use of `push_back`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(10); // Adds 10
numbers.push_back(20); // Adds 20
for (const auto& num : numbers) {
std::cout << num << " "; // Output: 10 20
}
return 0;
}
Performance Considerations
The time complexity of `push_back` is amortized O(1), meaning that while most insertions are performed in constant time, occasionally, the vector will need to resize its allocated memory to accommodate more elements, which can take linear time. Understanding this behavior is crucial for performance optimization, particularly in scenarios involving large data sets.
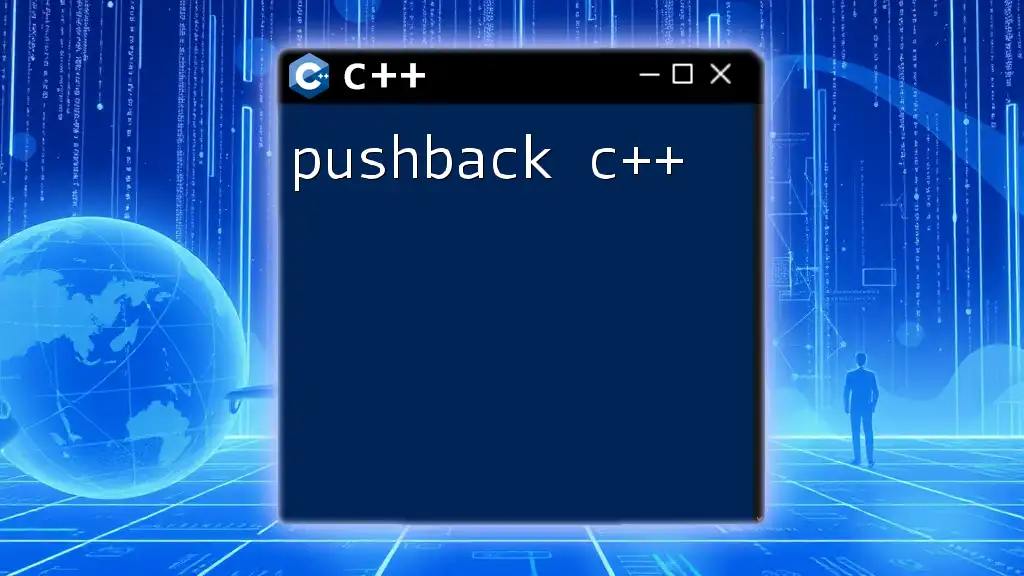
Emplace_back in C++
What is Emplace_back?
The `emplace_back` method is a more modern addition to C++ containers. It allows you to insert new elements constructed in place at the end of the vector. This means the object is created directly in the allocated storage, avoiding unnecessary copies or moves.
How Emplace_back Works
With `emplace_back`, C++ constructs the object in-place. By passing the required arguments, the constructor for the object can be called directly, leading to increased efficiency. Here’s an example that illustrates the in-place construction of objects:
#include <iostream>
#include <vector>
struct Point {
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
int main() {
std::vector<Point> points;
points.emplace_back(1, 2); // Constructs Point(1,2) in-place
points.emplace_back(3, 4);
for (const auto& p : points) {
std::cout << "(" << p.x << ", " << p.y << ") "; // Output: (1, 2) (3, 4)
}
return 0;
}
Performance Considerations
The time complexity of `emplace_back` is also amortized O(1), much like `push_back`. However, it avoids the overhead of extra copy or move operations by constructing the object where it is needed. This is particularly beneficial when working with complex types, as it can lead to noticeable performance improvements.
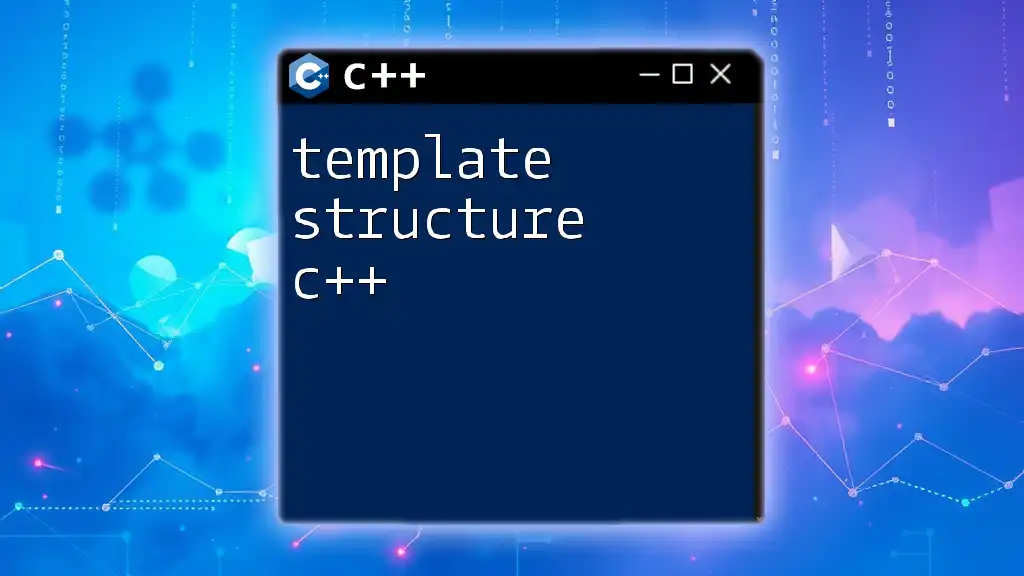
Key Differences Between Emplace_back and Push_back
Construction vs Copy
The core difference between `emplace_back` and `push_back` revolves around how objects are handled:
-
`push_back`: This method will copy or move the object into the vector. If the object is a temporary or an rvalue, it may use move semantics, but generally, it creates a copy of the data.
-
`emplace_back`: This method constructs the object in the memory already allocated for the vector, resulting in no unnecessary copies or moves. It is particularly useful for user-defined types that are expensive to copy.
Use Cases: When to Use Each Method
Choosing between `push_back` and `emplace_back` comes down to your specific situation:
- Use `push_back` when:
- You are adding simple types like integers or strings, where copying is inexpensive.
- The object being added is already fully constructed and stored elsewhere.
- Use `emplace_back` when:
- You are dealing with complex or user-defined types where you want to avoid overhead.
- You need to initialize members right away and prefer to avoid create-and-copy patterns.
Example:
std::vector<std::string> names;
names.push_back("Alice"); // Effectively copying a string
names.emplace_back("Bob"); // Constructing the string in-place
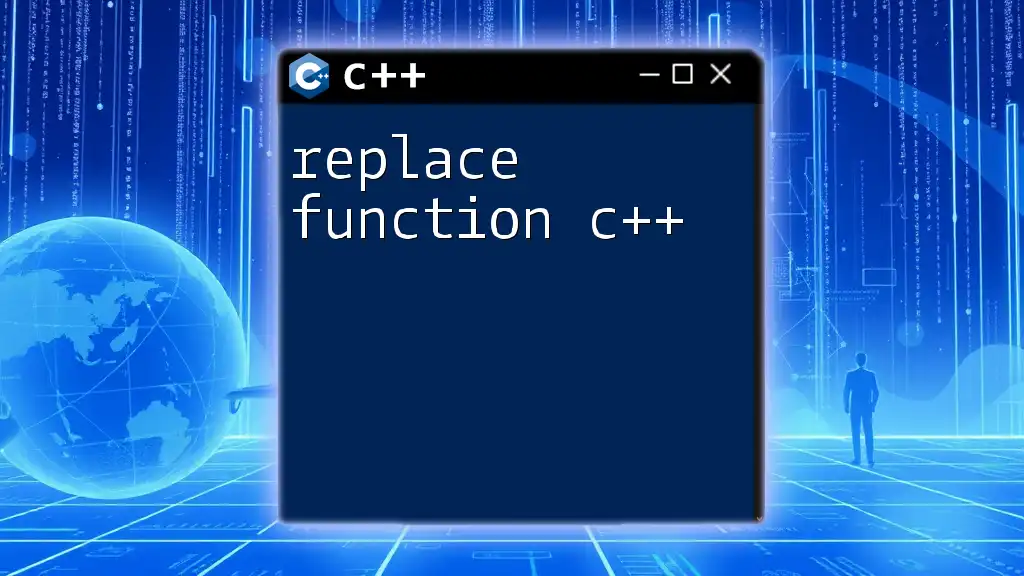
Best Practices for Using Push_back and Emplace_back
General Guidelines
To optimize performance and readability in your code:
- Prefer `emplace_back` for user-defined types and complex objects.
- Use `push_back` for simple data types where performance impact is minimal.
- Write code that chooses the right approach based on scenarios to maintain readability and efficiency.
Common Pitfalls
A common mistake is misunderstanding object lifetimes: When you pass temporary objects to `push_back`, the copies can lead to performance drops. Always consider whether the object’s lifespan aligns with your container’s expected usage. For efficiency, prefer `emplace_back` when you can initialize your object in place.
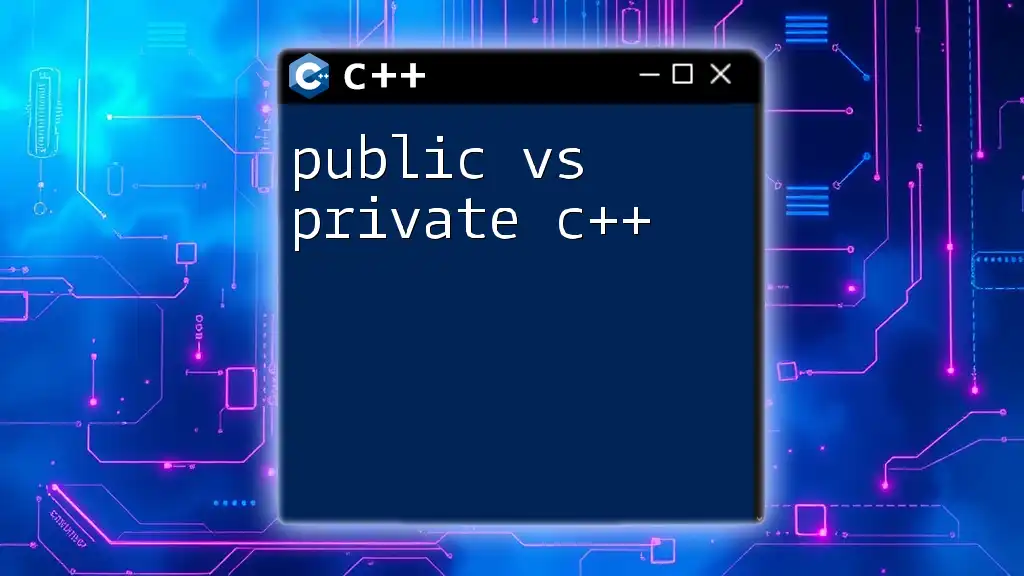
Conclusion
In examining emplace_back vs push_back in C++, we find that each method has its strengths and situational advantages. Understanding when to use each can lead to more efficient code and a deeper grasp of C++ mechanics. Consider your object types, their lifetimes, and your performance needs when deciding which method to implement.
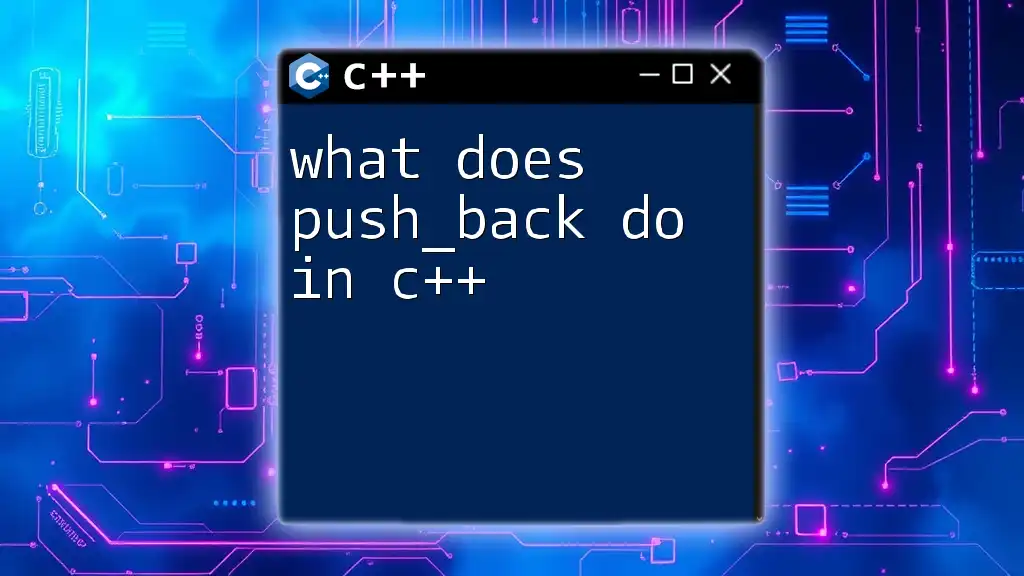
Further Learning Resources
For more details on standard templated containers, refer to C++ documentation or tutorial websites. Engaging with community forums can also provide practical insights and shared experiences from other developers.
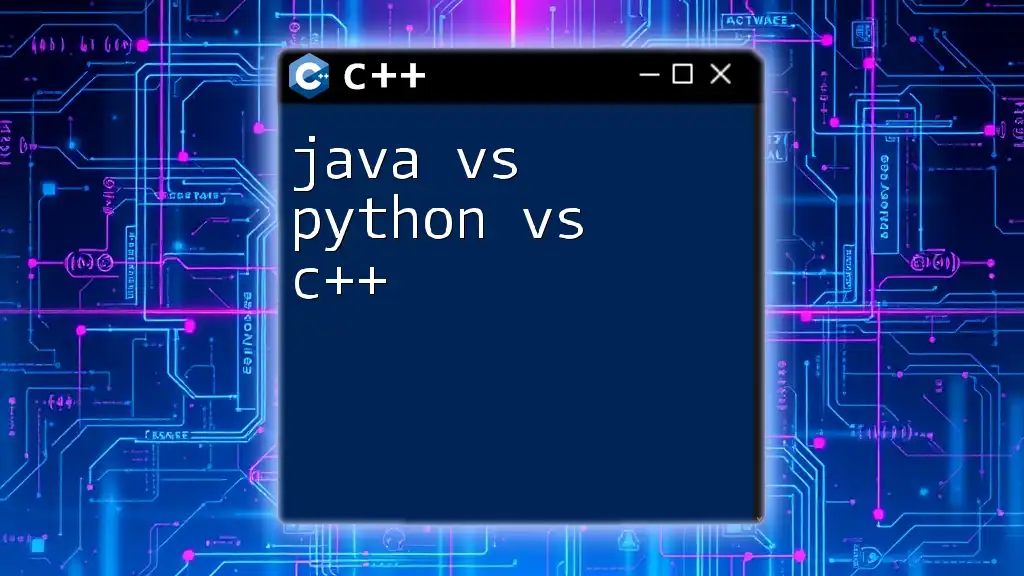
Call to Action
We’d love to hear your thoughts! Share your experiences using `emplace_back` and `push_back` in your C++ projects. Have questions? Don't hesitate to drop a comment below!