In C++, the `public` and `private` access specifiers control the visibility of class members, with `public` making them accessible from outside the class and `private` restricting access to within the class itself.
Here’s a simple code snippet illustrating the difference:
#include <iostream>
using namespace std;
class Example {
public:
int publicVar; // Accessible from outside
private:
int privateVar; // Not accessible from outside
};
int main() {
Example obj;
obj.publicVar = 5; // This works
// obj.privateVar = 10; // This will cause a compilation error
cout << "Public Variable: " << obj.publicVar << endl;
return 0;
}
What are Access Specifiers in C++?
Definition of Access Specifiers
In C++, access specifiers play a crucial role in determining the accessibility of class members from different parts of a program. The three primary access specifiers are public, private, and protected. Each of these access levels provides varying degrees of visibility and accessibility to the class members.
Encapsulation is a core principle of object-oriented programming (OOP) that allows developers to restrict access to certain parts of an object. This is particularly important for maintaining control over data and exposing only what is necessary.
The Role of Encapsulation
Encapsulation ensures that the internal representation of an object is hidden from the outside. This can be achieved by declaring class members as private or protected, allowing only specified functions (often public) to access and modify those members.
Benefits of using encapsulation include:
- Protection from unauthorized access: Critical data remains safe from external manipulation.
- Improved code maintainability: Changes to the internal workings of a class won't affect code that uses it, as long as the interface remains consistent.
Here’s an example demonstrating encapsulation:
#include <iostream>
class Encapsulated {
private:
int secretData;
public:
void setData(int value) {
secretData = value;
}
int getData() {
return secretData;
}
};
int main() {
Encapsulated obj;
obj.setData(42);
std::cout << "Secret Data: " << obj.getData() << std::endl; // Outputs secret data
return 0;
}

Understanding Public Members
Definition of Public Members
Public members within a class are accessible from any part of the program, making them ideal for data that needs to be freely accessed and modified outside the class.
Example of Public Member
Consider the following simple class example that illustrates public members:
#include <iostream>
class Example {
public:
int publicVar;
void display() {
std::cout << "Public Variable: " << publicVar << std::endl;
}
};
int main() {
Example ex;
ex.publicVar = 10; // Accessible from outside the class
ex.display(); // Output: Public Variable: 10
return 0;
}
Use Cases for Public Members
Public members are beneficial in scenarios where you want the user of the class to have the ability to directly manipulate data, such as:
- Configuration settings: Allowing external code to set parameters.
- Data models: Where the structure of the data is meant to be open and simply structured.

Understanding Private Members
Definition of Private Members
Private members are not accessible from outside the class. They are intended for internal use and manipulation only by the class itself and its friends (if any are defined).
Example of Private Member
Here’s an example of a class that uses private members:
#include <iostream>
class Example {
private:
int privateVar;
public:
void setVar(int value) {
privateVar = value; // Accessing private member
}
void display() {
std::cout << "Private Variable: " << privateVar << std::endl;
}
};
int main() {
Example ex;
ex.setVar(10); // Can access private member via public method
ex.display(); // Output: Private Variable: 10
// ex.privateVar = 20; // Error: 'privateVar' is private
return 0;
}
Use Cases for Private Members
Utilizing private members is advantageous when:
- You want to hide internal data structures from outside classes or functions, thereby preventing accidental or unauthorized changes.
- You require controlled access to your data, ensuring that it only changes in predictable ways, through designated functions.
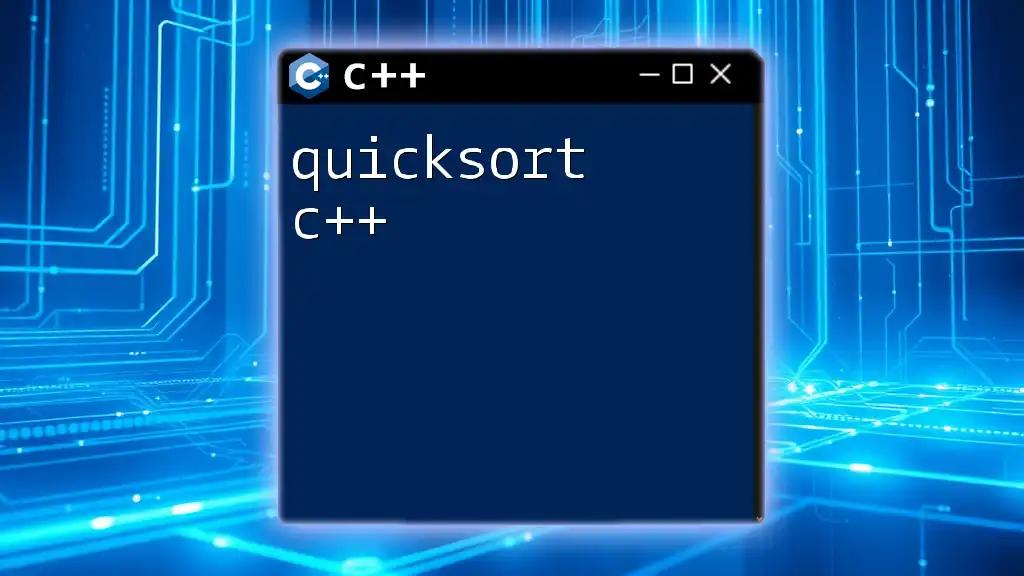
Differences Between Public and Private Members
Accessibility
The most distinct difference between public and private members is their accessibility. Public members can be accessed anywhere in the code, while private members are bound to the class they're declared in. This brings about various implications on class design and functionality.
Analogy
Think of public members like an open door to a store — anyone can walk in and interact with what’s on the shelves. In contrast, private members are akin to back rooms — significant items are locked away, accessible only by authorized personnel (the class itself).
Design Considerations
Choosing between public and private access greatly influences your class design:
-
Public: Using public members can lead to easier accessibility and more straightforward implementation. They can simplify how objects interact with each other but can potentially expose your data to unwanted modifications or misuse.
-
Private: Relying on private members promotes robust applications, as they can help maintain the integrity of your data, allowing only specified functions to manipulate it. This leads to cleaner, more maintainable code, especially in larger systems.

Conclusion
In the realm of public vs private C++, understanding the distinctions and appropriate use cases for these access specifiers is vital for developing robust, maintainable classes. Public members allow flexibility and openness, while private members provide controlled access and data protection. Striking the right balance between these two access levels is essential for effective C++ programming. As you continue to explore the expansive landscape of C++, mastering access modifiers will undeniably enhance your coding skills and efficiency.