In C++, the `public` access specifier allows class members to be accessible from outside the class, while the `private` access specifier restricts access to those members only within the class itself.
class MyClass {
public:
int publicData; // Accessible from outside the class
private:
int privateData; // Accessible only within MyClass
};
Understanding the Role of Access Specifiers
Access specifiers are an essential feature of C++ that determine how the members (variables and methods) of a class can be accessed from outside the class. They play a crucial role in the principles of object-oriented programming (OOP), particularly in the aspect of encapsulation. Encapsulation is the practice of bundling the data (attributes) and the methods (functions) that operate on the data into a single unit or class. This setup not only helps in keeping the data safe from unauthorized access but also aids in reducing complexity for the endpoint user.
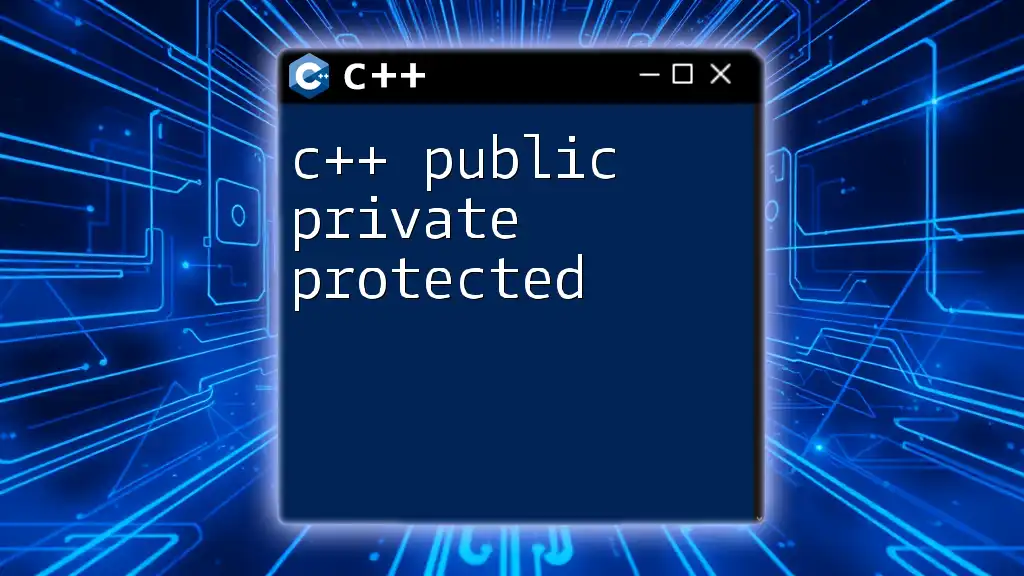
Overview of Public and Private Access Specifiers
In C++, the two primary access specifiers are public and private. Understanding the difference between them is fundamental in mastering class design and data handling. They provide a framework to restrict or permit access to class members, ensuring that sensitive data remains guarded while providing necessary functionality.
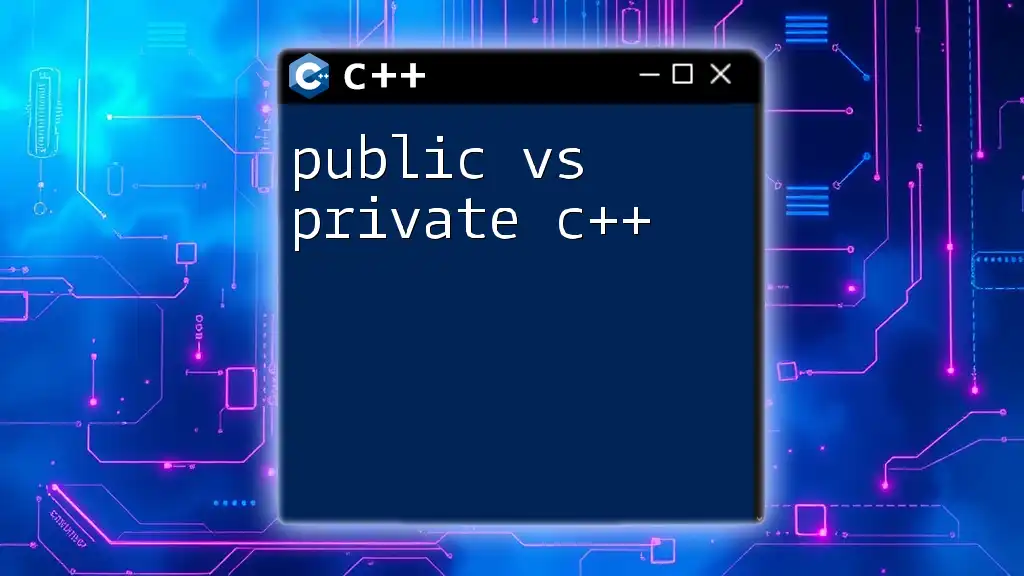
What is a Public Access Specifier?
Definition and Characteristics of Public
The public access specifier allows the members of a class to be accessible from any part of the program. This means that any class that instantiates an object can directly access public attributes and methods. Its use can significantly enhance interaction with the objects, making them user-friendly and highly functional.
How to Use Public in C++
When defining class members as public, you enable outside access and manipulation, which is beneficial for many programming scenarios. Here’s an example:
class Example {
public:
int publicValue;
void showPublicValue() {
std::cout << publicValue << std::endl;
}
};
In this example, `publicValue` can be accessed directly from an instance of `Example`, and the method `showPublicValue` can be called to output its value. This simplicity in access encourages the use of public members when data is intended to be openly shared.
When to Use Public Access Specifier
Public access is typically used for those attributes and methods that need to be used frequently across different sections of the program. For example, you might declare a public counter variable or methods responsible for user input.
Benefits of Using Public:
- Ease of access: Public members can be accessed without restrictions.
- Enhanced functionality: Allows for creating interfaces that can be readily accessed by users or other components.
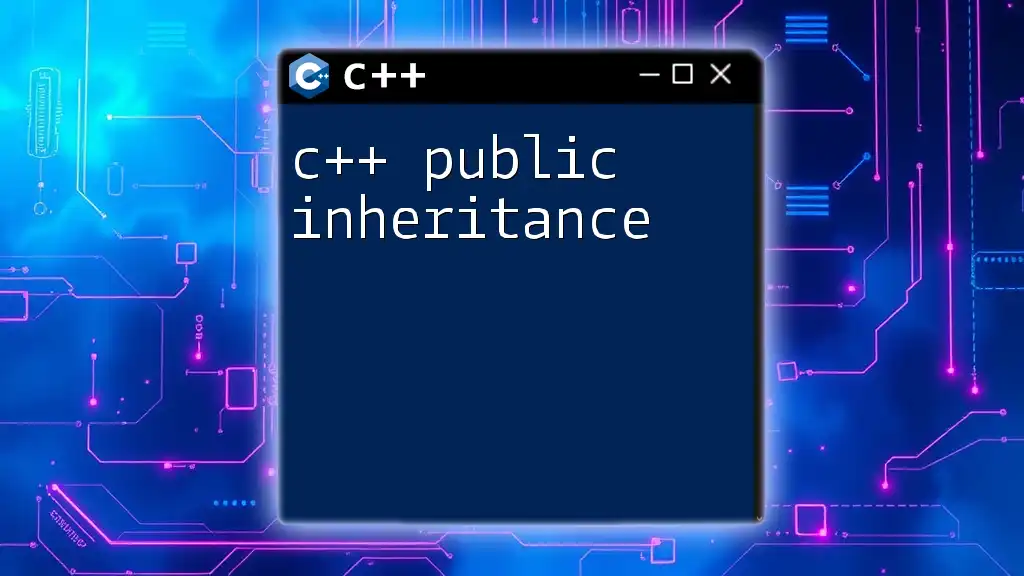
What is a Private Access Specifier?
Definition and Characteristics of Private
In contrast, the private access specifier restricts access to the members of a class from outside its scope. Private members can only be accessed by methods of the owning class or friend classes. This restriction is vital for ensuring that sensitive data is handled securely without external interference.
How to Use Private in C++
Private members are useful for maintaining control over how data is modified or accessed. Here's an example:
class Example {
private:
int privateValue;
public:
void setPrivateValue(int val) {
privateValue = val;
}
int getPrivateValue() {
return privateValue;
}
};
In this example, `privateValue` cannot be accessed directly from outside the class. Instead, it must be manipulated via the provided public methods. This design encapsulates the data, enhancing security and data integrity.
When to Use Private Access Specifier
Private access is crucial when you need to safeguard the internal state of an object. Use it when:
- The data must remain consistent and unaltered by external programs.
- There are specific ways that data must be modified or accessed to maintain validity.
By using private data members, programmers can effectively create robust classes with controlled access.
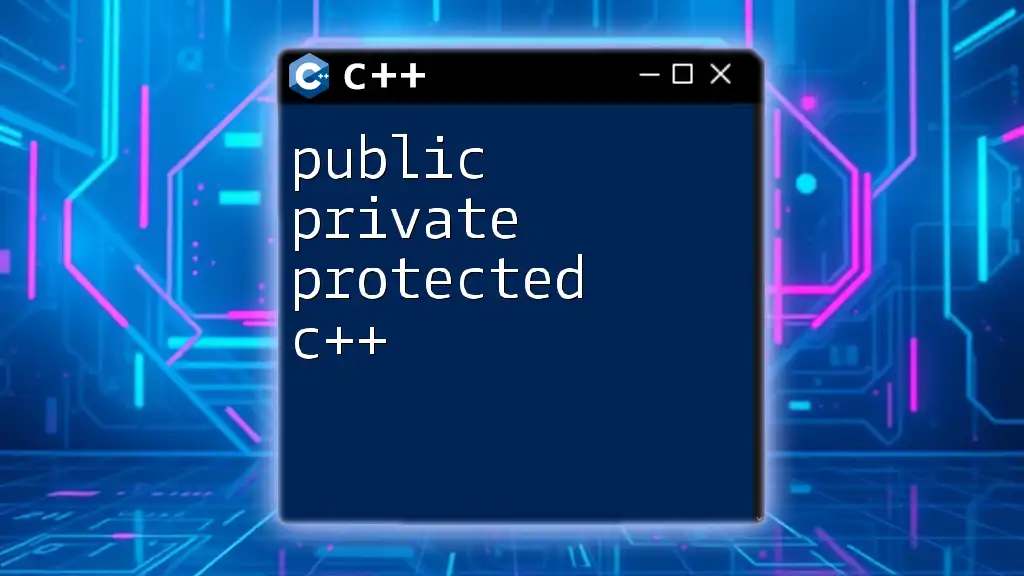
Public vs Private: Key Differences
Access to Members and Methods
The fundamental distinction between public and private access specifiers lies in visibility. Public members are accessible from anywhere in the program, while private members are hidden and can only be accessed internally.
Key Points:
- Public members can be accessed freely.
- Private members can only be accessed by methods within the same class.
Comparative Analysis Table:
Access Level | Accessible by Class | Accessible by Derived Class | Accessible by Outside Class |
---|---|---|---|
Public | Yes | Yes | Yes |
Private | Yes | No | No |
Encapsulation and Data Hiding
Encapsulation relies heavily on the use of public and private access specifiers to achieve data hiding. Public members expose the necessary functionality to the outside world, while private members keep sensitive information secure. This balance is vital for creating maintainable and reliable code.
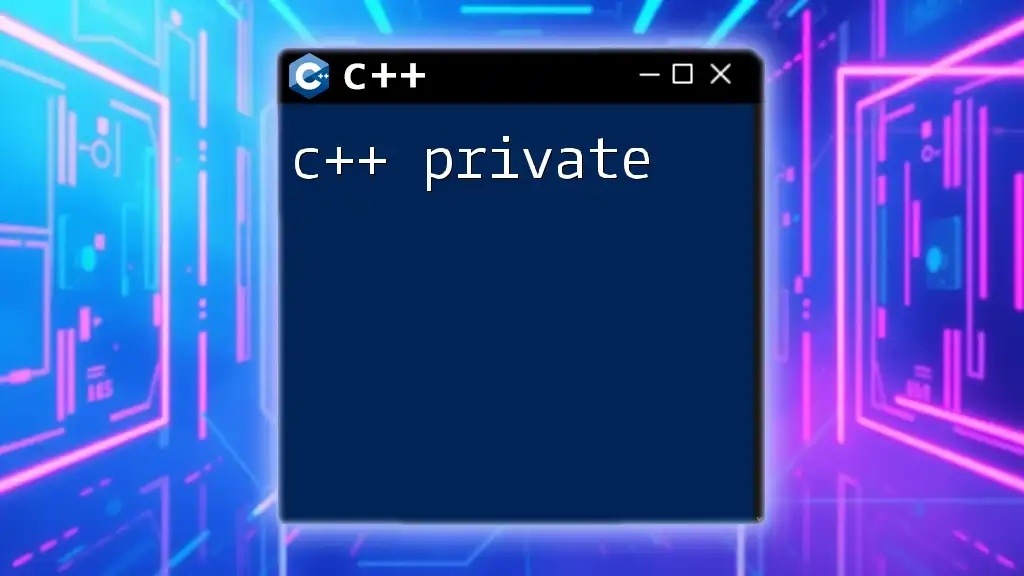
Practical Examples of Public and Private in Action
Example Class Design
Let’s consider a practical example of a BankAccount class, showcasing both public and private members:
class BankAccount {
private:
double balance;
public:
BankAccount(double initialBalance) {
balance = initialBalance;
}
void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
double getBalance() const {
return balance;
}
};
In this design:
- `balance` is a private member, ensuring that it cannot be modified directly from outside the class.
- The `deposit` method is public, enabling controlled interaction with `balance` while ensuring that only valid operations are performed.
Real-world Applications
The ability to effectively apply public and private access specifiers plays a crucial role in the performance of software design. It allows developers to:
- Create more modular code that is easier to maintain and understand.
- Protect critical data from being altered unintentionally, preventing bugs and maintaining application stability.
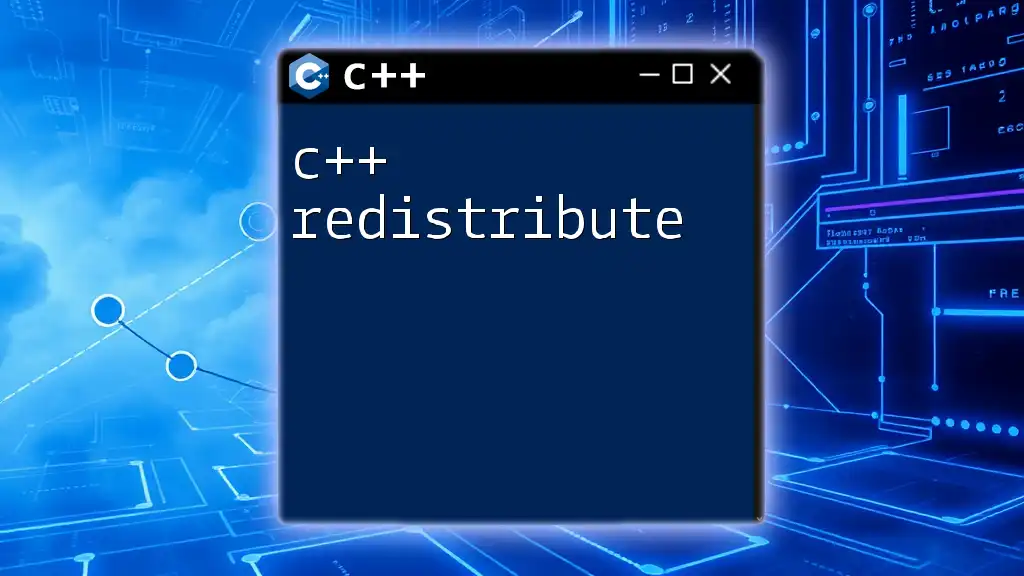
Best Practices for Using Access Specifiers
Guidelines for Effective Use
- Use private for sensitive data to avoid unwanted changes.
- Limit public access to only those methods that need to be exposed.
Combining Public and Private for Optimal Design
Striking the right balance between public and private access is key to effective class design. Always aim for a clear API that exposes only what's necessary, utilizing private methods and attributes to manage internal state while leaving other ends open for interaction.
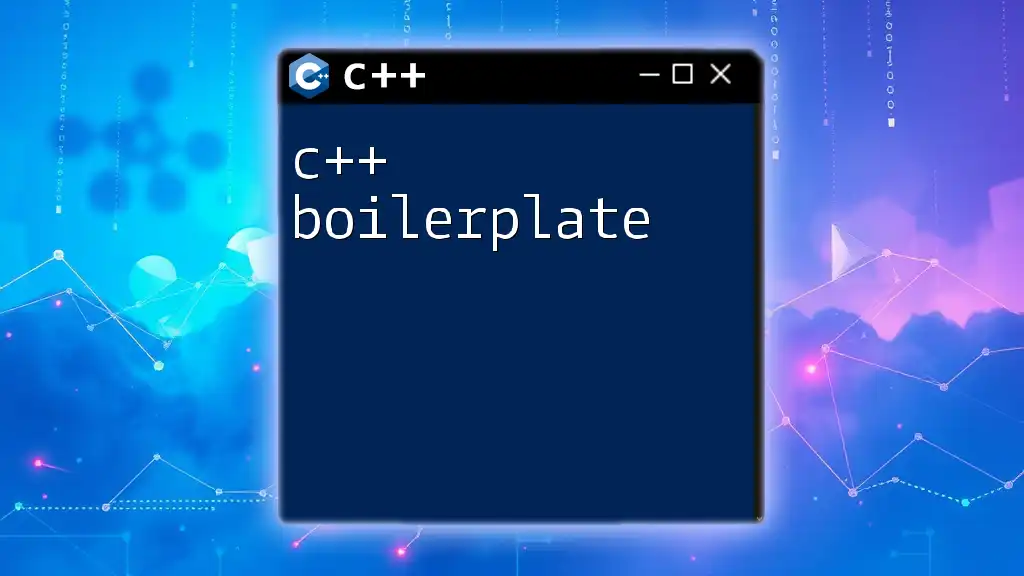
Conclusion
Understanding the differences and applications of C++ public vs private access specifiers is fundamental in crafting effective C++ programs. By applying these concepts judiciously, you can create robust, maintainable, and secure software that leverages the strengths of object-oriented design principles. Remember to experiment with these access specifiers in your projects, and don’t hesitate to share your experiences or questions about using public and private in the comments below.