In C++, `char` is a data type used to store a single character, providing the ability to create character variables that can represent letters, digits, and symbols.
Here’s a simple code snippet demonstrating how to declare and initialize a `char` variable:
char letter = 'A';
What is a Char in C++?
A char is a fundamental data type in C++ that represents a single character. It plays a vital role in programming, particularly when dealing with text and character manipulation. Understanding the `char` data type is essential, as it forms the basis for managing characters in strings and handling textual input/output efficiently.
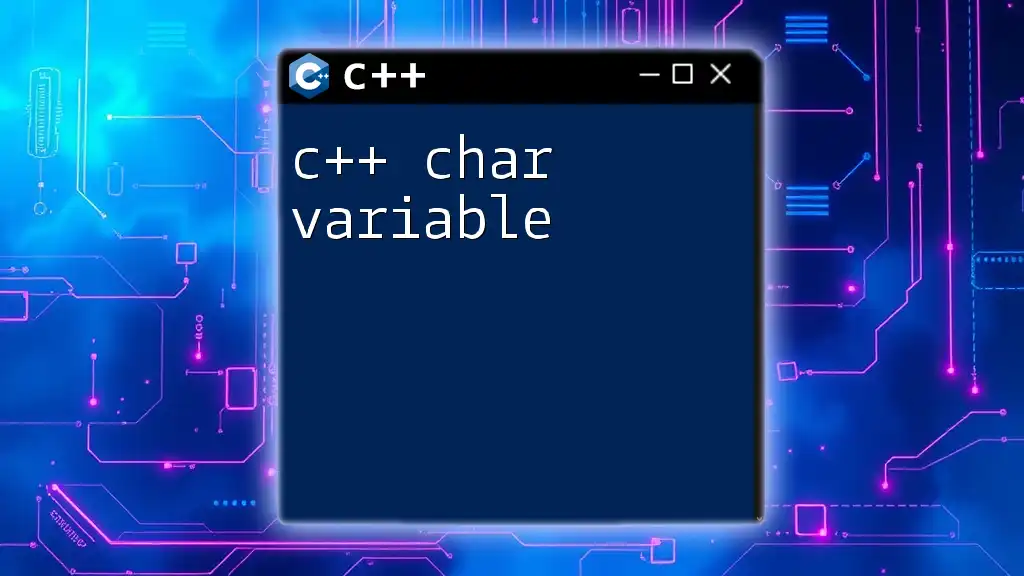
Understanding the C++ Char Data Type
What is a Character in C++?
In programming terms, a character is a basic unit of information that represents a letter, digit, punctuation mark, or any other symbol. In C++, the c++ char data type is used to store and manipulate individual characters.
C++ Char Data Type
The `char` data type has specific characteristics. Primarily, it is 1 byte in size, capable of storing values from -128 to 127 when signed, and from 0 to 255 when unsigned. The range depends on whether you declare it as a signed or unsigned char. This finite range comes from the fact that `char` relies on standards like ASCII or Unicode, which provide specific integer values for each character.
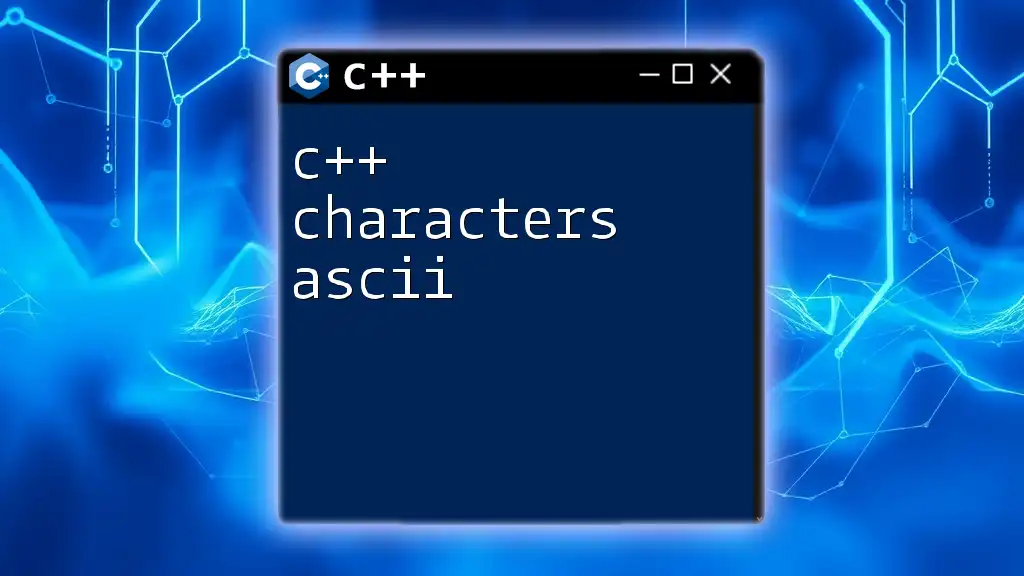
Declaring and Initializing Char Variables
How to Use Char in C++
Declaring and initializing a char variable is straightforward. The syntax for `char` declaration looks like this:
char letter = 'A';
Note that characters in C++ should be enclosed within single quotes (`'`) as opposed to double quotes (`"`), which are used for string literals. For instance:
char digit = '3';
This distinguishes individual characters from strings, which consist of an array of characters.
Character Sets and Encodings
Understanding character sets—most notably ASCII and Unicode—is vital in programming. ASCII is the American Standard Code for Information Interchange, which assigns a unique number to each character. For example, the letter 'A' has an ASCII value of 65:
char character = 'A';
int asciiValue = (int)character; // asciiValue will be 65
With the rise of global software, Unicode has become essential, allowing characters from many different languages and symbols to be represented.
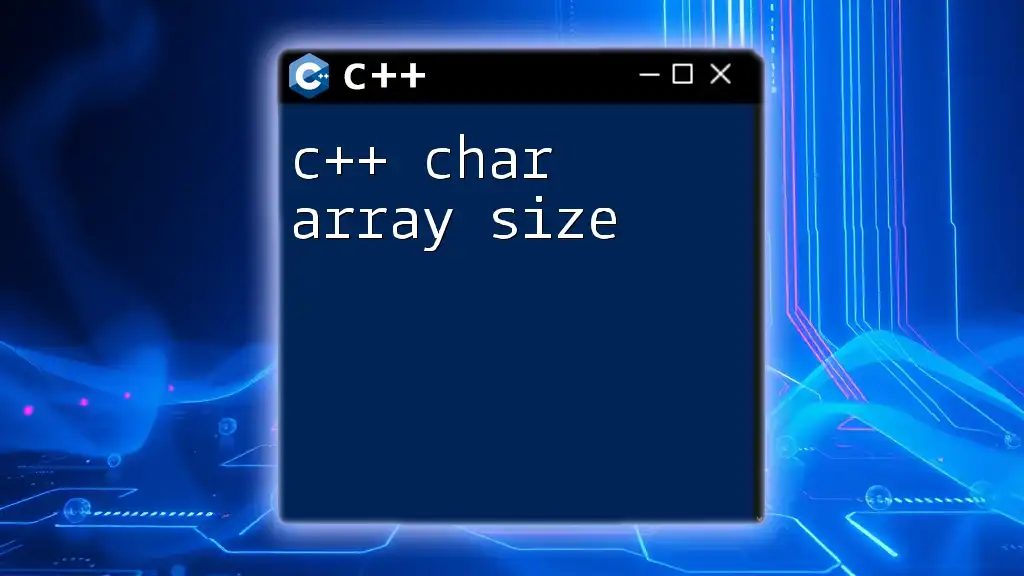
Manipulating Characters in C++
Common Operations with Char
Char manipulation includes various operations such as arithmetic operations. For instance, you can increment or decrement the value of a char, which will affect its ASCII representation:
char c = 'A';
c++; // c is now 'B'
This simple example demonstrates how changing a character's value can lead to different outcomes based on its underlying integer representation.
Using Char in Strings
In C++, `char` can also be used in conjunction with strings. While a char represents a single character, a string can be thought of as an array of characters. The following example illustrates a char array:
char greeting[] = "Hello";
In this example, `greeting` can handle a sequence of characters while still allowing direct access to each individual `char`.
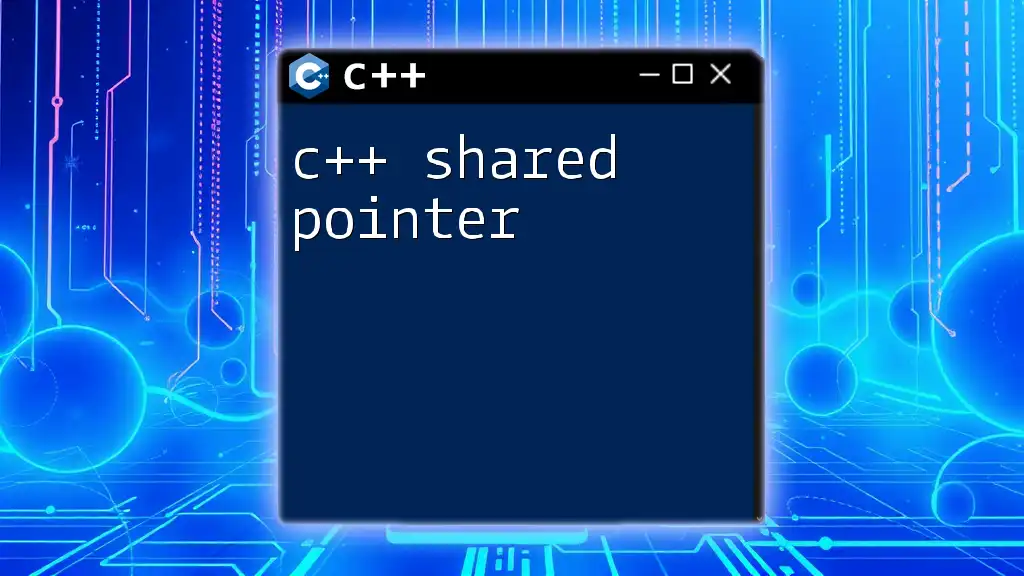
Special Types of Char in C++
Unsigned and Signed Char
Understanding the difference between signed and unsigned char is essential. A signed char can hold both positive and negative values, whereas an unsigned char can only hold positive values. For example:
signed char a = -10; // Valid
unsigned char b = 250; // Valid
This distinction is crucial when working with character encodings, especially when dealing with byte-level manipulations.
Wide Char in C++
The `wchar_t` data type represents wide characters, accommodating a larger range of characters, particularly useful for internationalization. It can store characters from various languages, unlike the standard `char` data type, which is limited primarily to ASCII values.
wchar_t wideChar = L'あ'; // a wide character in Japanese
Using `wchar_t` allows programs to handle input and output from non-Latin scripts seamlessly.
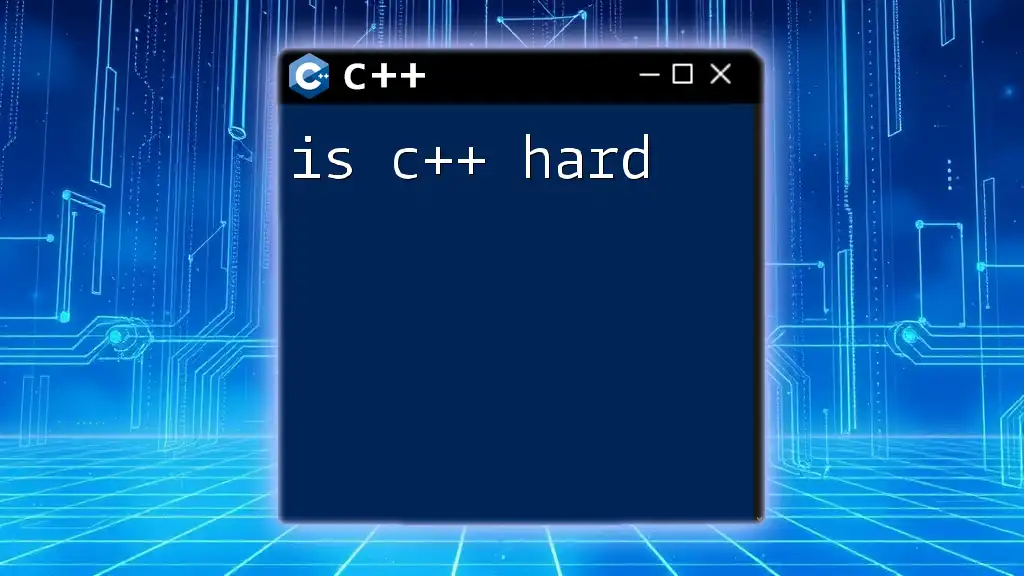
Input and Output of Char
Reading and Writing Characters
Reading and writing chars in C++ can be done using the standard `cin` and `cout` objects. Below is a simple example that demonstrates how to engage user input:
char userInput;
cout << "Enter a character: ";
cin >> userInput;
cout << "You entered: " << userInput << endl;
This example prompts the user to enter a character, capturing the input in the `userInput` variable and then displaying it.
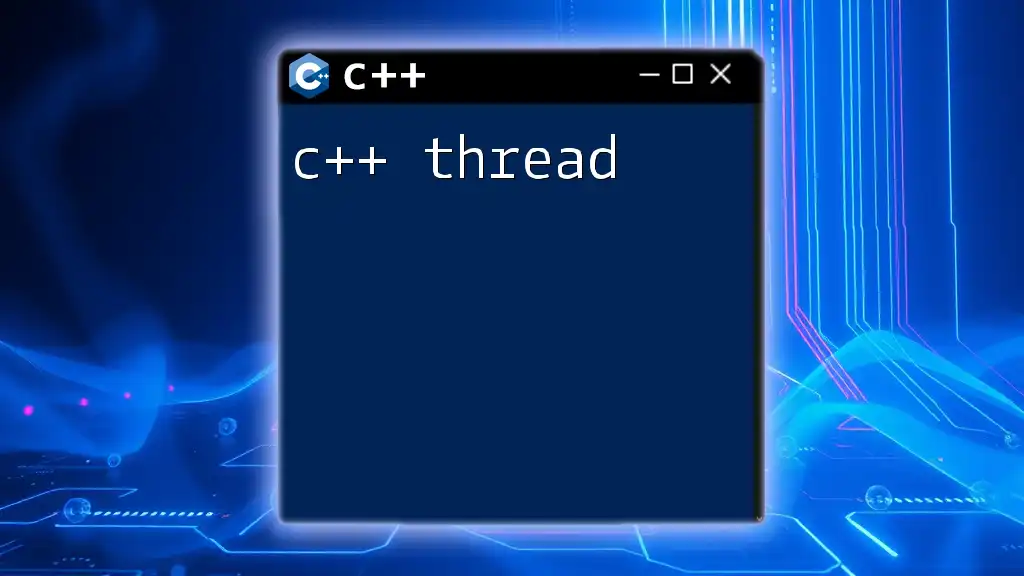
Common Errors and Troubleshooting
Common Mistakes with Char in C++
When working with chars, programmers often confuse char with other types, especially strings. It's essential to remember that characters need to be enclosed in single quotes, while string literals require double quotes. Another common issue is incorrectly using integer or character values interchangeably, which can lead to unexpected results.
Debugging Char Issues
To troubleshoot common issues with char variables, always check your declarations and ensure quotes are correctly used. If you encounter ASCII-related errors, double-check the character values using type casting, as shown earlier.
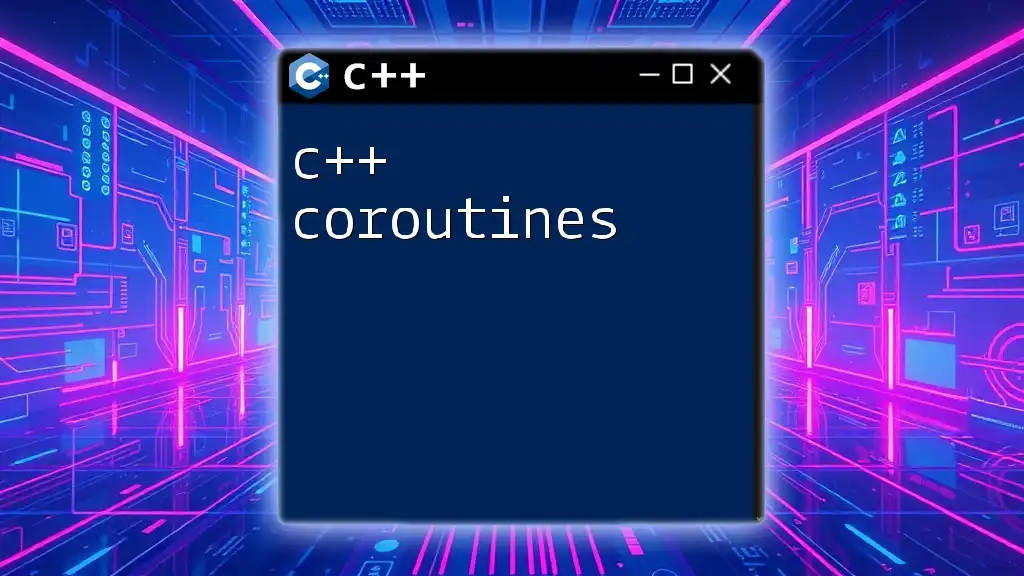
Conclusion
Through this exploration of c++ char, we have delved into its functionality, characteristics, and operations. Having a solid understanding of the `char` data type will significantly enhance your programming skills in C++. Remember to experiment with various char manipulations as you continue your journey in C++. Embrace the versatility of characters, and expand your grasp of string handling and text processing in your coding endeavors.
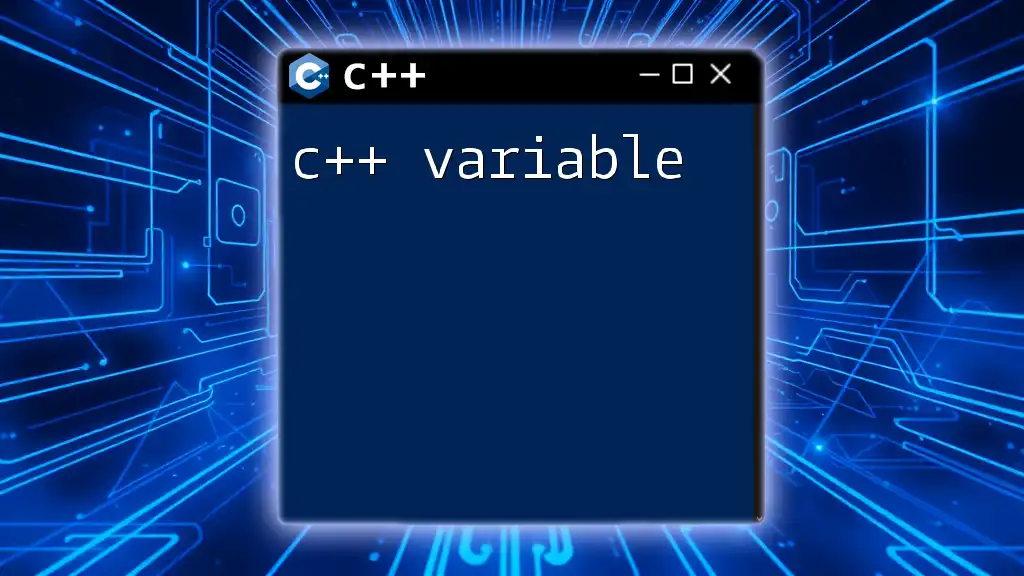
Additional Resources
For those looking to deepen their knowledge further, consider exploring books on C++ programming, online courses, and interactive coding platforms. Practice exercises focusing on character manipulation can also be beneficial, allowing you to solidify your understanding of this foundational concept.