The `atan` function in C++ is used to compute the arc tangent (inverse tangent) of a given value, returning the angle in radians whose tangent is that value. Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cmath>
int main() {
double value = 1.0;
double angle = atan(value); // Compute the arc tangent of 1
std::cout << "The angle in radians is: " << angle << std::endl;
return 0;
}
Understanding the Arctan Function
The Mathematical Foundation
Arctangents play a crucial role in trigonometry, representing the inverse of the tangent function. Mathematically, if \(\tan(\theta) = x\), then \(\arctan(x) = \theta\). The output is an angle \( \theta \) expressed in radians that lies between \(-\frac{\pi}{2}\) and \(\frac{\pi}{2}\).
The graphical representation of the arctangent function shows a continuous curve that approaches its horizontal asymptotes at \(y = -\frac{\pi}{2}\) and \(y = \frac{\pi}{2}\). Understanding these properties is vital for effectively using the arctan function in computational scenarios.
The Atan Function in C++
In C++, the atan function is part of the `<cmath>` header, which provides access to mathematical functions. The atan function calculates the arctangent of a number and can be implemented easily.
Syntax of Atan Function
double atan(double x);
Parameters: The function takes a single parameter, `x`, which is the tangent of the angle in radians. The valid range for `x` can be any real number, as the tangent function can take values from \(-\infty\) to \(+\infty\).
Return Value: The output returns the angle in radians for which the tangent value equals `x`. This makes the atan function invaluable for adjusting angles in a program or performing calculations involving rotations and direction.
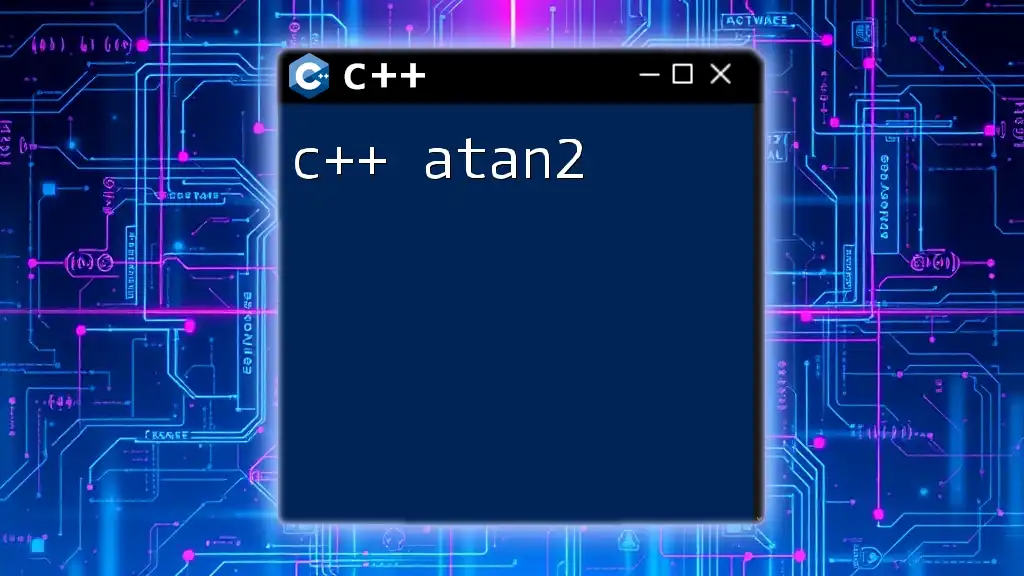
Using the Atan Function in C++
Basic Usage of Atan
To utilize the atan function in your C++ programs, you only need to include the `<cmath>` library. Here's a simple example showcasing how to use it for a basic calculation.
#include <iostream>
#include <cmath>
int main() {
double x = 1.0;
double result = atan(x);
std::cout << "Arctan(" << x << ") = " << result << " radians" << std::endl;
return 0;
}
In this example, we declare a variable `x` with a value of 1.0, and then we calculate the arctan of `x`. The function returns the angle in radians, which corresponds to \( \frac{\pi}{4} \) or 0.7854 radians. The output illustrates how easily we can compute the arctangent.
Converting Atan Output to Degrees
To many, radians can be less intuitive compared to degrees when understanding angles. Converting the output of the `atan` function into degrees will enhance clarity for those accustomed to this measurement.
To convert radians to degrees, we can multiply the result by \( \frac{180}{\pi} \). Below is an example of how to achieve this conversion:
#include <iostream>
#include <cmath>
const double PI = 3.14159265358979323846;
double radToDeg(double radians) {
return radians * (180.0 / PI);
}
int main() {
double x = 1.0;
double resultRad = atan(x);
double resultDeg = radToDeg(resultRad);
std::cout << "Arctan(" << x << ") = " << resultDeg << " degrees" << std::endl;
return 0;
}
The function `radToDeg` converts the radians to degrees, making it clear that the angle we derived from the `atan` function is 45 degrees when `x` is 1.0. Understanding the conversion between these two units is an essential skill in programming.
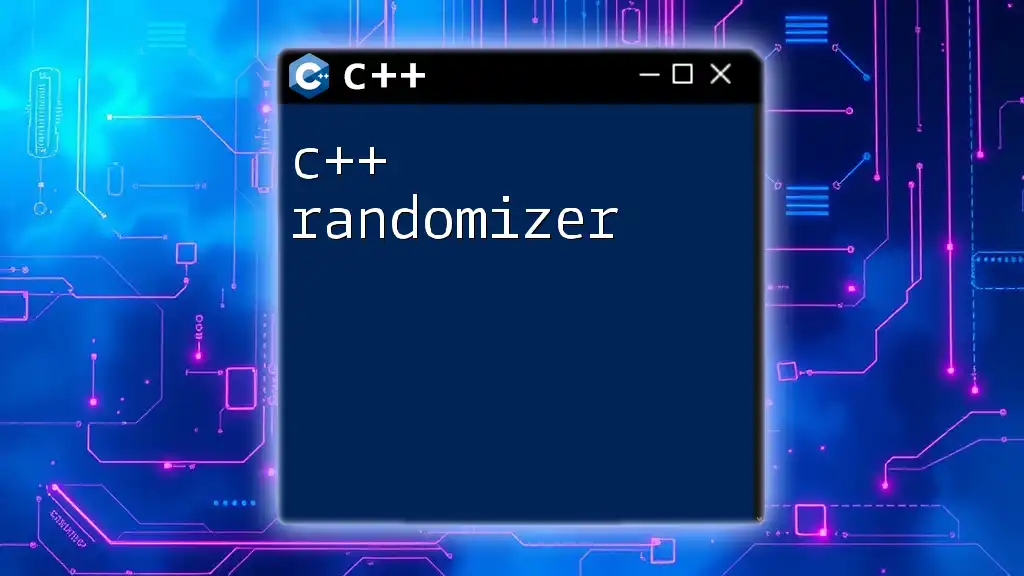
Advanced Applications of the Atan Function
Usage in Financial Calculations
The arctan function isn’t only limited to geometry or physics; it can also be beneficial in finance. Consider risk assessment models where calculating the arctangent of risk variables helps in optimizing portfolios. For example, if the ratio of the expected return of an asset versus its volatility is computed, arctan can convert this ratio into an angle, indicating a measurable risk level.
Implementing Arctan in Geolocation Calculations
When working with geographic data, particularly in mapping applications, arctan provides a mechanism for converting coordinates on a grid to angles. For example, the function can be used to determine the angle required to reach a destination point from a given starting point, allowing for precise navigation even in two-dimensional space.
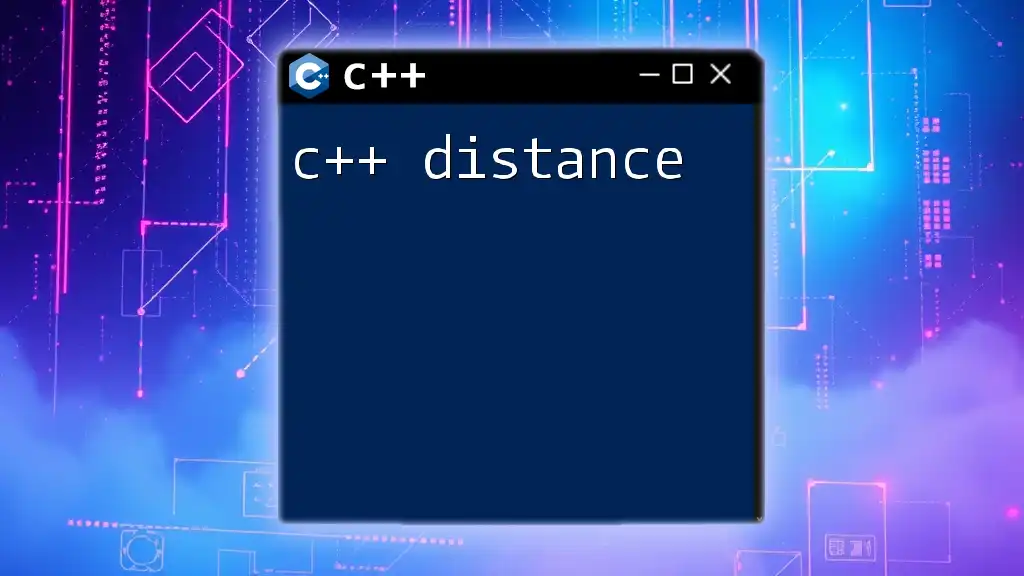
Common Mistakes When Using Arctan in C++
Understanding the Output Range
One common issue programmers encounter is misunderstanding the range of the output from the `atan` function, which only provides values between \(-\frac{\pi}{2}\) and \(\frac{\pi}{2}\) (or -90° to 90°). Failing to acknowledge this can lead to incorrect assumptions, particularly when visualizing results in a multi-quadrant context.
Misinterpreting the Argument
Misinterpretation of the input for the atan function is another pitfall. People may mistakenly input improperly scaled values or unclear representations of input data. It’s crucial to ensure that the argument passed into the `atan` function appropriately represents the tangent of an angle for accurate results.
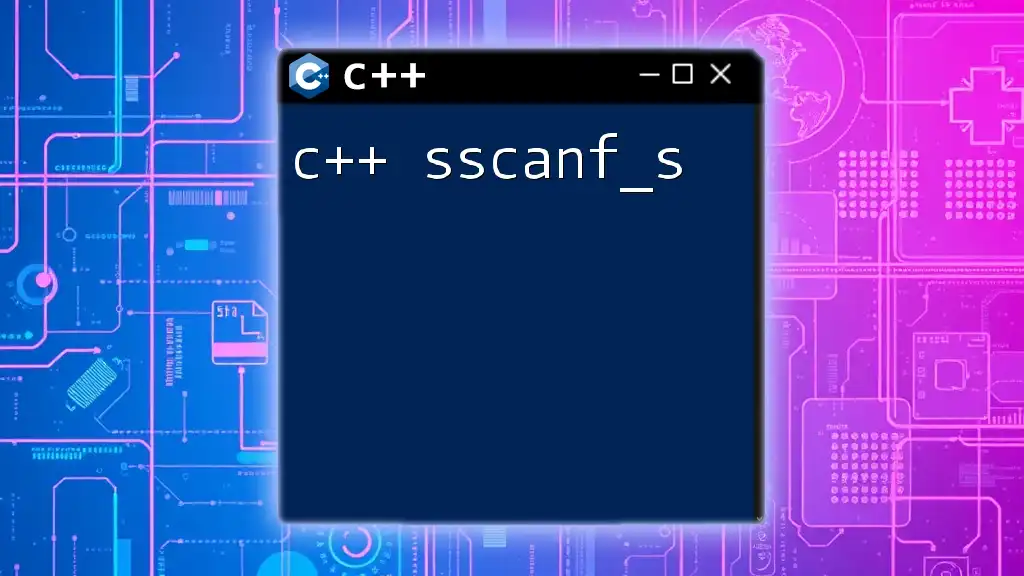
Conclusion
In summary, understanding the `c++ arctan` function allows developers to leverage mathematical principles to solve complex problems efficiently. From financial calculations to geolocation tasks, the applications of arctan are vast and varied.
By practicing code examples and solidifying your grasp on radians and degrees, you'll be equipped to tackle a wide range of programming challenges.
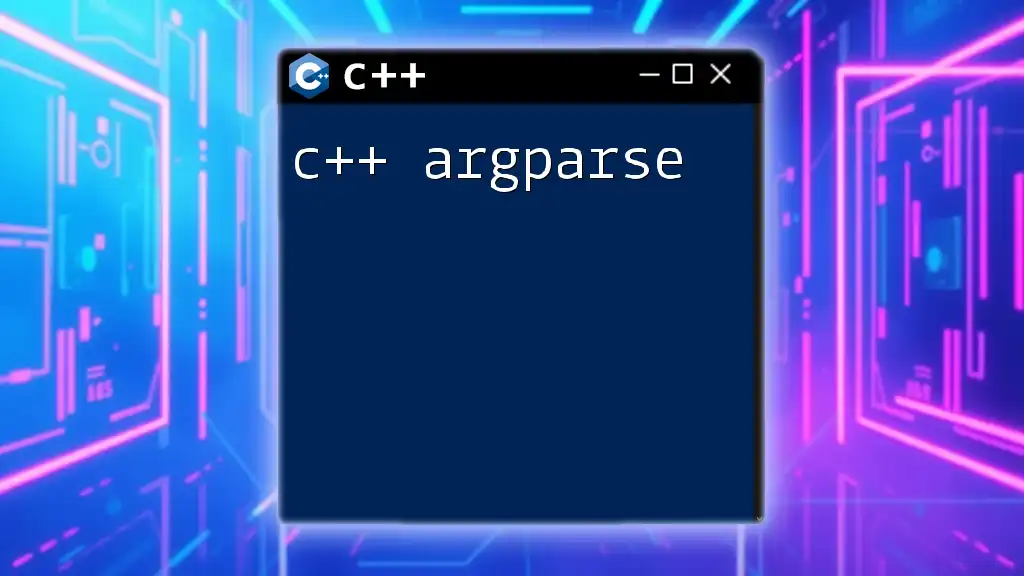
Additional Resources
For further detail, refer to the C++ documentation and reliable mathematical resources to deepen your understanding of functions and their applications. This foundational knowledge will empower you to execute more sophisticated algorithms and computations.
Call to Action
Explore our offerings to master C++ commands effectively and enhance your programming skill set. Get ready to embark on a journey of quick and concise learning!