The `atan2` function in C++ computes the angle (in radians) between the positive x-axis and the point given by its coordinates (y, x), effectively handling the quadrant of the angle based on the signs of both components.
#include <iostream>
#include <cmath>
int main() {
double y = 5.0;
double x = 2.0;
double angle = atan2(y, x);
std::cout << "The angle is: " << angle << " radians" << std::endl;
return 0;
}
Understanding Trigonometry Basics
What is Arctangent?
The arctangent function, often denoted as `atan`, is the inverse function of the tangent function. In simpler terms, it finds the angle whose tangent is a given number. The output of the arctangent function is typically defined within the range of \(-\frac{\pi}{2}\) to \(\frac{\pi}{2}\) radians. This limited range can sometimes be problematic when working with coordinates in the Cartesian plane, as it doesn’t adequately account for all quadrants.
Role of `atan2` in Trigonometry
In contrast, the `atan2` function not only computes the arctangent but also considers the signs of both the x and y coordinates. This functionality makes `atan2` particularly valuable in determining the angle in a full 360-degree rotation, spanning the range from \(-\pi\) to \(\pi\) radians (or from 0 to 360 degrees). This is essential in scenarios such as robotics and computer graphics, where directional calculations are critical for movement and positioning.
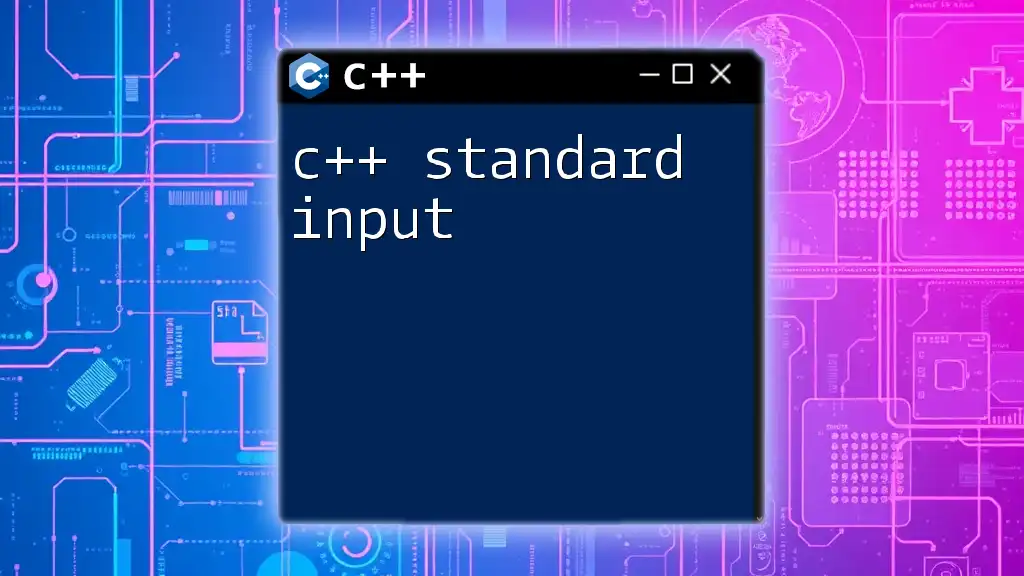
Syntax of `atan2` in C++
The syntax for the `atan2` function is straightforward. It can be described using the following function signature:
double atan2(double y, double x);
- Parameters:
- `y`: The vertical coordinate (opposite side).
- `x`: The horizontal coordinate (adjacent side).
- Return value: A `double` representing the angle in radians.
It is critical to remember the order of parameters in `atan2`, as swapping them will yield different results, potentially leading to incorrect angle computations.
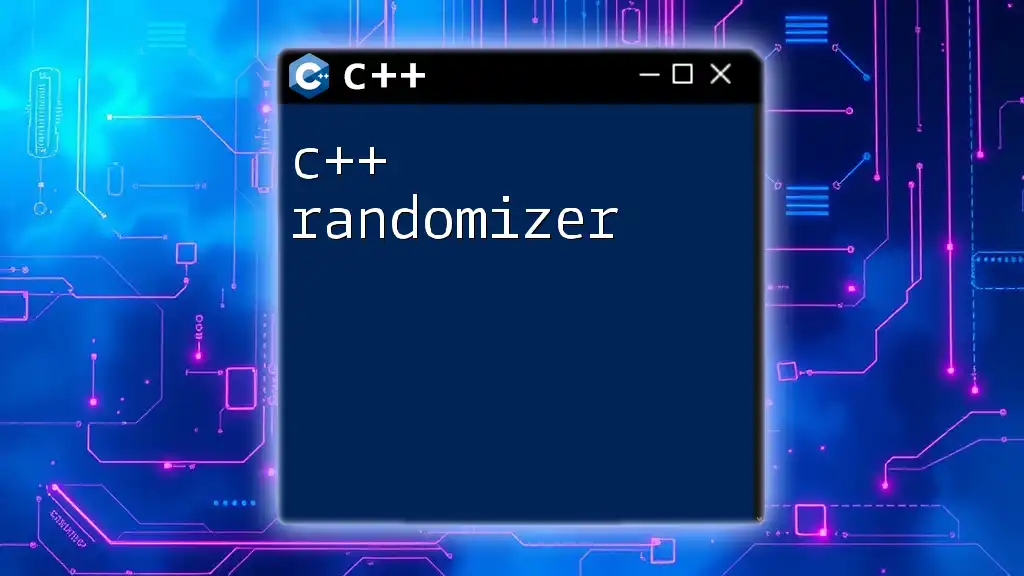
How to Use `atan2` in C++
Basic Example
To illustrate the use of the `atan2` function, consider the following simple example. This code calculates the angle of a point located at (1.0, 1.0).
#include <iostream>
#include <cmath>
int main() {
double y = 1.0;
double x = 1.0;
double angle = atan2(y, x);
std::cout << "The angle is: " << angle << " radians." << std::endl;
return 0;
}
In this case, the output will indicate an angle of \(\frac{\pi}{4}\) radians, which corresponds to 45 degrees. This result is expected since the point lies in the first quadrant, where both coordinates are positive.
Working with Positive and Negative Values
Positive Quadrant
When both parameters are positive, `atan2` easily computes the angle in the first quadrant. For instance:
double angle1 = atan2(1, 1); // Expected output: π/4 radians
The angle here indicates a 45-degree rotation from the positive x-axis.
Negative Values
The behavior of `atan2` drastically shifts when one or both parameters are negative. For example, using negative coordinates such as in the following code:
double angle2 = atan2(-1, -1); // Expected output: -3π/4 radians
This will yield an angle of \(-\frac{3\pi}{4}\) radians, indicating a rotation of 135 degrees in the clockwise direction, effectively landing the point in the third quadrant.
Special Cases to Note
One of the special cases that often causes confusion is when both coordinates are zero. Inputting `atan2(0, 0)` is undefined mathematically, but in C++, it typically returns a value of 0. This behavior should be handled with care in any application that makes use of `atan2`.
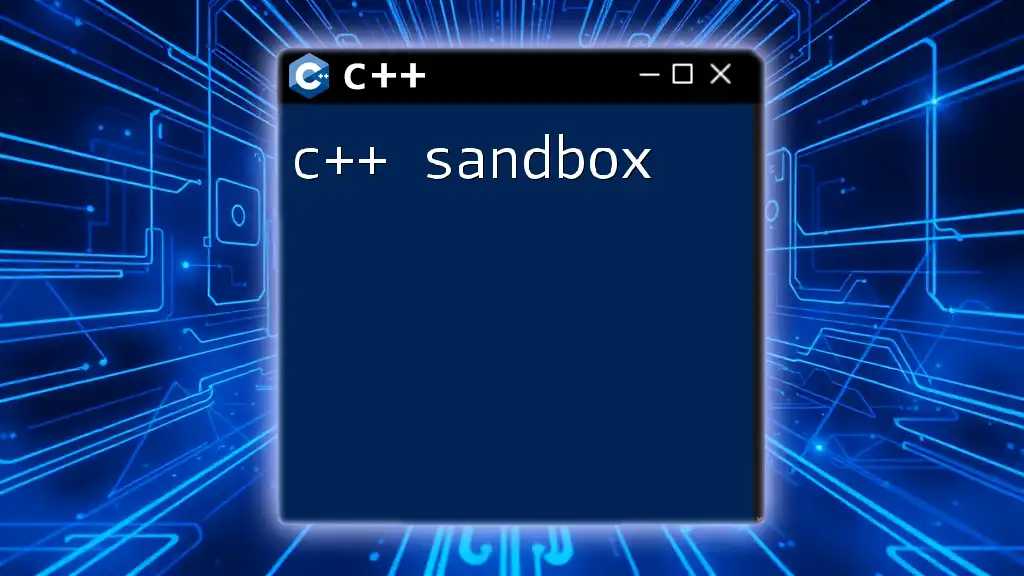
Applications of `atan2` in Real Life
Navigation and Robotics
In fields like navigation and robotics, the `atan2` function is invaluable for calculating angles that guide movements. For instance, if a robot needs to orient itself towards a target object located at coordinates (y, x), using `atan2(y, x)` will yield the angle the robot should turn. This ensures that it can accurately aim in the direction of the target, accounting for all possible quadrants and positions.
Astronomy and Physics
In astronomy and physics, `atan2` is often employed for coordinate transformations. When observing celestial bodies, it’s crucial to convert from Cartesian coordinates (x, y) to polar coordinates (radius, angle). `atan2` simplifies this process, allowing scientists to describe the position of objects effectively.
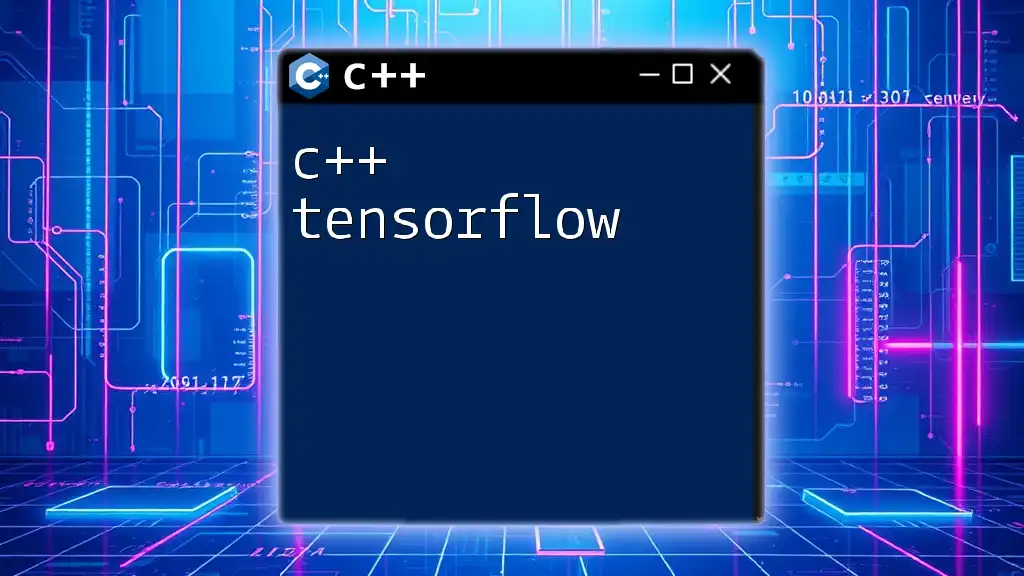
Common Mistakes While Using `atan2`
One prevalent mistake when using `atan2` is misunderstanding the order of parameters. Swapping `x` and `y` can lead to completely erroneous angles. Additionally, failing to account for the full range of output radians may result in incorrect assumptions about the angle's direction.
Suppose you use the following:
double wrongAngle = atan2(x, y);
Here, the angle calculated is not representative of the true direction, potentially leading to navigation errors.
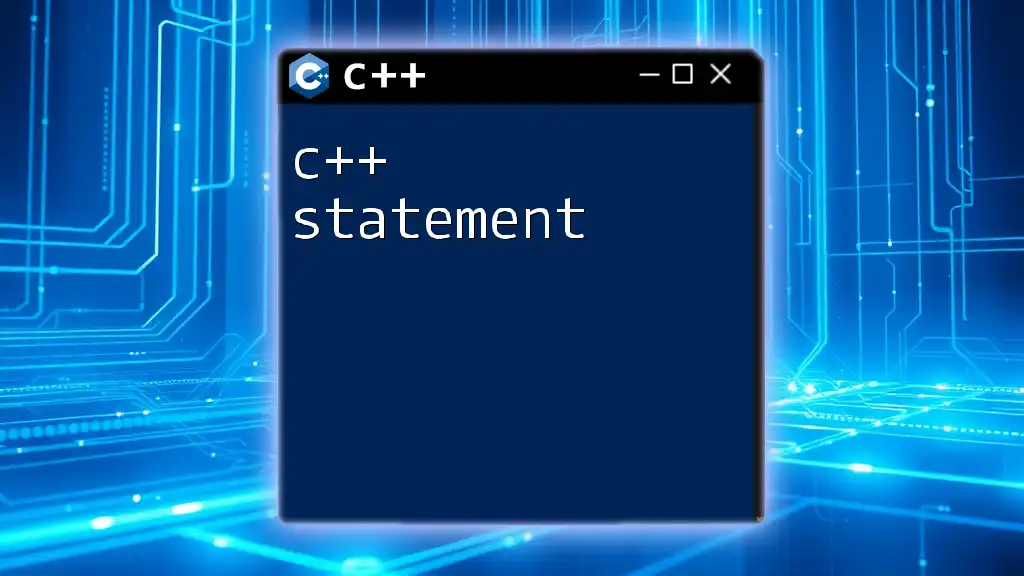
Best Practices for Implementing `atan2`
Code Readability
When implementing `atan2`, maintaining code readability enhances collaborative development. Always include comments explaining the rationale behind calculations. Use meaningful variable names that provide context. For example, using `targetY` and `targetX` instead of generic `y` and `x` will always be more informative.
Performance Considerations
Regarding performance, `atan2` is generally efficient, but understanding the underlying computations can help optimize your code. In performance-critical applications, minimize the frequency of calls to trigonometric functions by caching results when appropriate. When applying `atan2`, test and profile your code to identify areas for optimization.
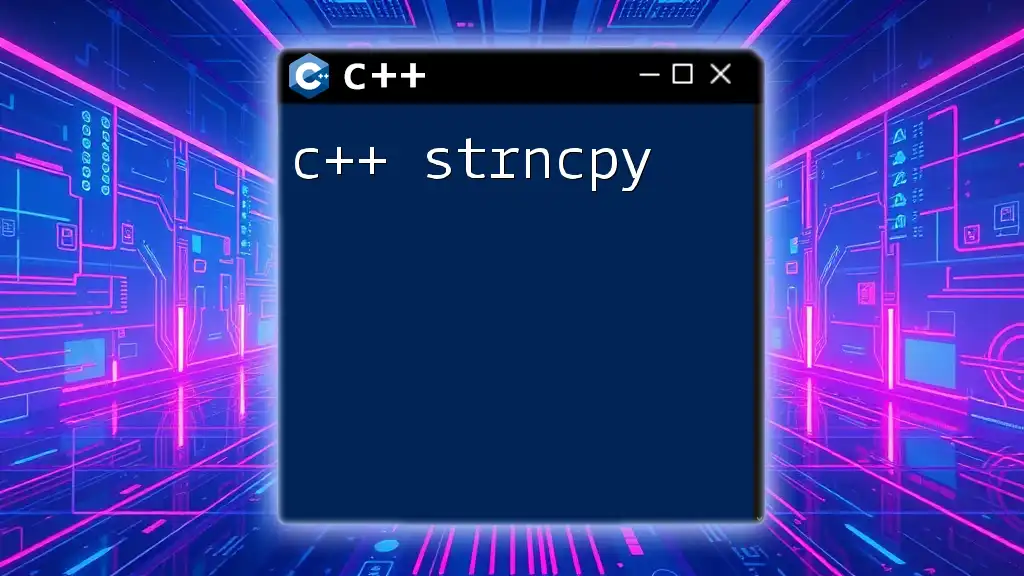
Conclusion
In summary, the `c++ atan2` function is a powerful tool for determining angles and navigating the complexities of trigonometry. By considering the y and x coordinates, `atan2` provides accurate directional angles, crucial for applications in robotics, navigation, and physics. With practice and careful consideration of pitfalls, you can effectively leverage this function in your C++ programs.
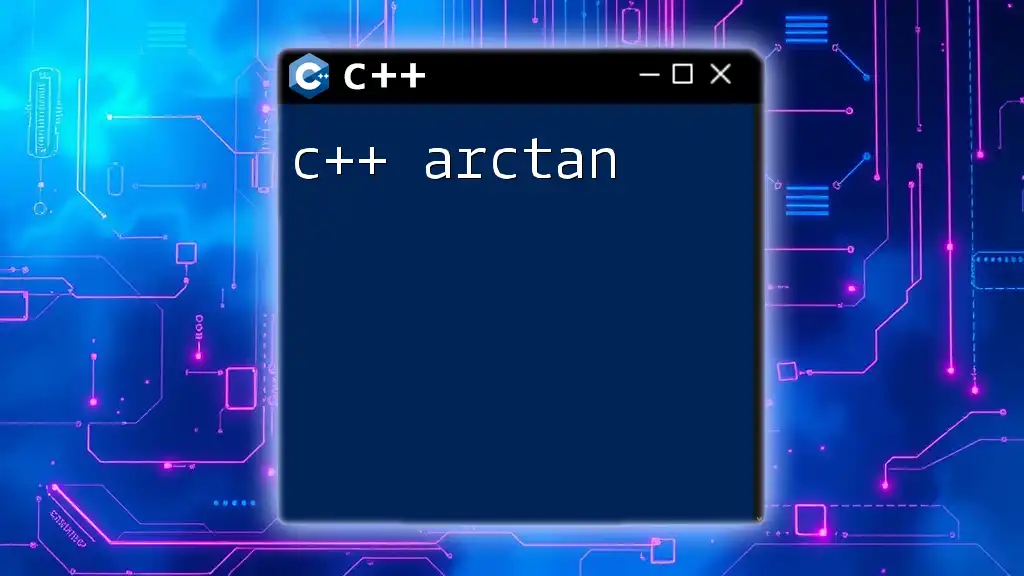
Further Reading and Resources
For those looking to deepen their understanding of trigonometric functions in C++, consider exploring recommended books and online courses. The links to official C++ documentation can also provide additional insights and details on using `atan2` and other mathematical functions effectively in your coding projects.