A C++ packet sniffer is a tool that captures and analyzes network packets traveling over a network interface for troubleshooting and monitoring purposes.
#include <pcap.h>
#include <iostream>
void packetHandler(u_char *args, const struct pcap_pkthdr *header, const u_char *packet) {
std::cout << "Packet captured with length: " << header->len << std::endl;
}
int main() {
pcap_if_t *alldevs;
pcap_findalldevs(&alldevs, NULL);
pcap_t *handle = pcap_open_live(alldevs->name, BUFSIZ, 1, 1000, NULL, NULL);
pcap_loop(handle, 10, packetHandler, NULL);
pcap_close(handle);
pcap_freealldevs(alldevs);
return 0;
}
Prerequisites
Before diving into creating your C++ packet sniffer, it's crucial to grasp some foundational concepts.
Basic Knowledge of Networking Concepts
Understanding the basics of networking is essential. Familiarize yourself with concepts such as TCP/IP and UDP. TCP (Transmission Control Protocol) provides reliable, ordered, and error-checked delivery of data, while UDP (User Datagram Protocol) offers a faster, simpler service without guaranteed delivery. Recognizing how data packets traverse a network is vital for effective packet sniffing.
Moreover, you should be aware of the protocols in use, as this will help you interpret captured data correctly and identify the network traffic that is most relevant to your analysis.
Familiarity with C++ Programming
Before you start building your sniffer, having a good command of C++ is necessary. Key concepts like pointers, classes, and memory management are recurrent in packet-sniffing applications. If you're unfamiliar with handling external libraries in C++, now is the time to brush up on these skills, as they are pivotal for integration with the pcap library, the cornerstone of packet sniffer development.
Required Software and Libraries
To create a C++ packet sniffer, you’ll need to install specific software and libraries:
- C++ Compiler: Use g++ or a suitable environment like Visual Studio.
- pcap library: This is fundamental for capturing and analyzing packets.
- WinPcap (for Windows users): A packet capture library essential for Windows systems.
Installing these tools is often straightforward, and you can find detailed instructions on the respective websites or repositories.
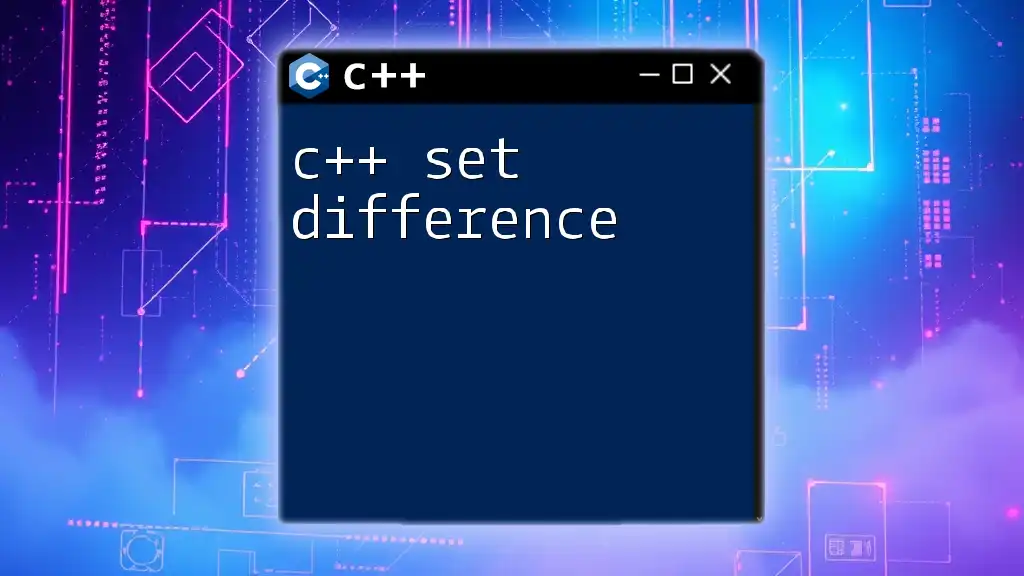
Getting Started with a C++ Packet Sniffer
Setting Up Your Environment
To develop your packet sniffer, start by setting up your programming environment. Depending on your operating system, follow the respective installation guide for your compiler and libraries. Ensure that you have the necessary permissions to execute network operations.
Understanding the pcap Library
The pcap library is the tool that will allow you to interface with the network layer on your machine. Its abilities include capturing network packets and filtering them based on specific criteria.
You can include the library in your C++ project like so:
#include <pcap.h>
Understanding the functions of this library will be crucial as it provides the backbone for capturing packets efficiently.
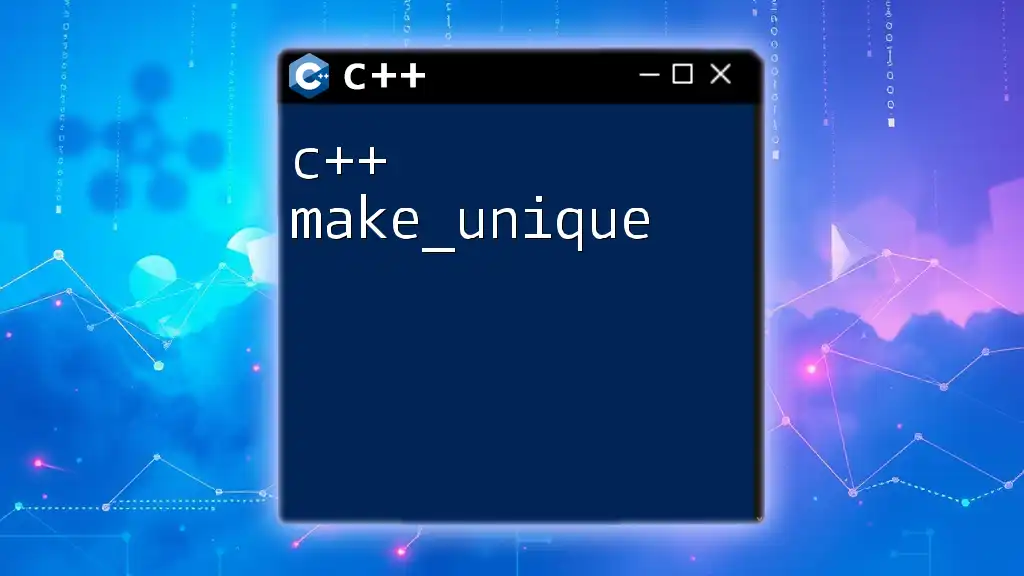
Building Your First Packet Sniffer
Creating a Basic Sniffer Application
Begin constructing your packet sniffer by structuring a basic application to capture network packets. Here’s a simple code snippet that initializes packet capturing:
int main() {
pcap_if_t *alldevsp, *device;
pcap_t *handle;
char errbuf[PCAP_ERRBUF_SIZE];
// Fetch the list of devices
if (pcap_findalldevs(&alldevsp, errbuf) != 0) {
fprintf(stderr, "Error in pcap_findalldevs: %s\n", errbuf);
return 1;
}
// Select a device (for simplicity, selecting the first one)
device = alldevsp;
handle = pcap_open_live(device->name, BUFSIZ, 1, 1000, errbuf);
// You can now capture packets using the handle
}
This code will help you set up a network device for capturing packets.
Capturing Packets with pcap
After setting up the handle, it’s time to initiate the packet capture. Use `pcap_loop` to continuously capture incoming packets, or `pcap_dispatch` for a set number of packets. Here’s how to implement it:
pcap_loop(handle, 10, packet_handler, NULL);
This command tells the program to capture packets in a loop, invoking `packet_handler` for every packet received.
Implementing a Packet Handler Function
The packet handler function processes each packet captured. You'll define a function to extract and display data from the packets. Here’s a basic implementation:
void packet_handler(u_char *args, const struct pcap_pkthdr *header, const u_char *packet) {
printf("Packet captured with length of [%d]\n", header->len);
}
This function prints out the length of each captured packet, providing an insight into the data being transferred across the network.
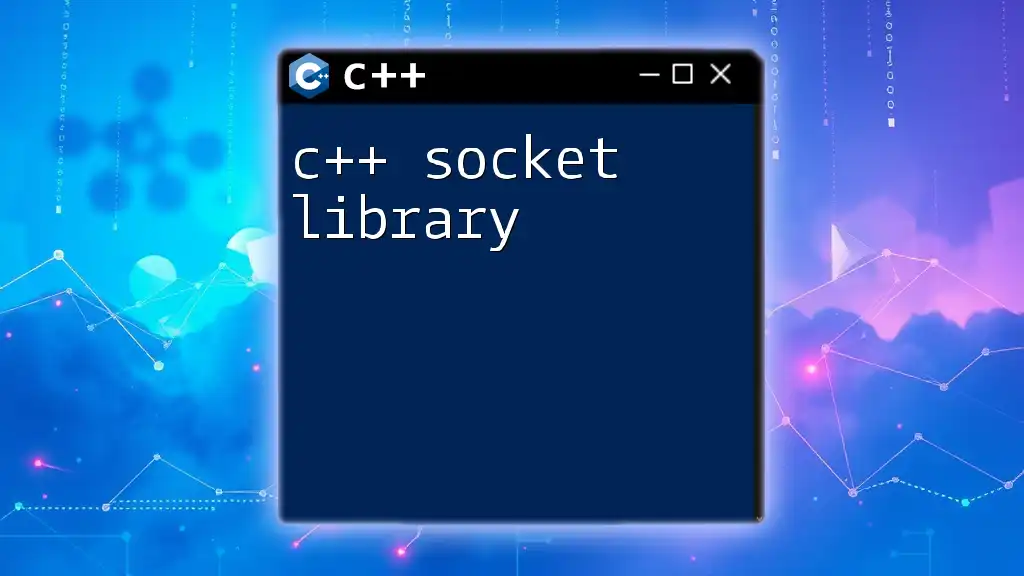
Advanced Packet Sniffing Techniques
Filtering Captured Packets
In real-world scenarios, filtering packets is essential. The Berkeley Packet Filter (BPF) syntax allows you to define specific criteria for what packets to capture. For example:
struct bpf_program filter_program;
char filter_exp[] = "port 80"; // Example filter for HTTP traffic
pcap_compile(handle, &filter_program, filter_exp, 0, PCAP_NETMASK_UNKNOWN);
pcap_setfilter(handle, &filter_program);
This filter ensures that only packets destined for port 80 (HTTP) are captured, thus reducing noise in your output.
Displaying Packet Information
Extracting relevant details from captured packets is a critical function of your sniffer. For TCP/IP packets, you'll often need to display source and destination addresses. Use the following example to retrieve IP address information:
struct iphdr *ip_hdr = (struct iphdr *)(packet + sizeof(struct ether_header));
printf("Source IP: %s\n", inet_ntoa(ip_hdr->saddr));
printf("Destination IP: %s\n", inet_ntoa(ip_hdr->daddr));
This code dissects the packet to produce human-readable IP addresses from the packet raw data.
Saving Captured Packets to File
If you're interested in analyzing network traffic later, consider saving the captured packets to a file. The pcap library allows you to easily write captured packets to a file:
pcap_dumper_t *dumper = pcap_dump_open(handle, "packets.pcap");
Using this, you can save your captured packets in `packets.pcap`, which can later be analyzed using various packet analysis tools.
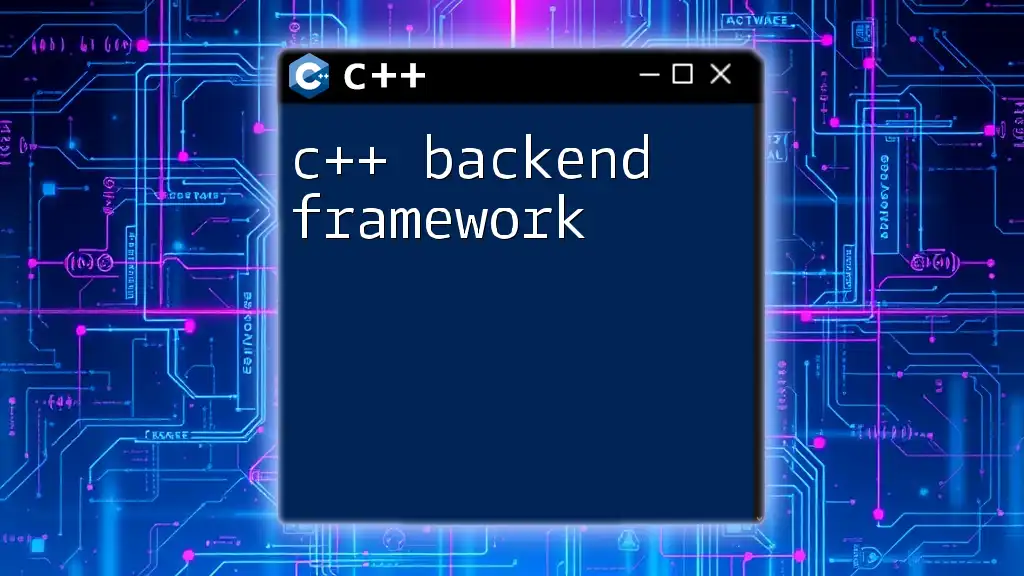
Real-World Applications of C++ Packet Sniffing
Network Monitoring and Troubleshooting
A C++ packet sniffer can be a powerful tool for monitoring network performance and identifying bottlenecks. By analyzing packet flow, network managers can spot irregularities and performance issues promptly.
Security Analyzing
Packet sniffers play an integral role in network security assessments. They can help detect unauthorized access and malicious traffic, providing essential information that can be used to enhance network defenses.
Data Traffic Analysis
Businesses can utilize packet sniffers to analyze data traffic patterns, enabling them to make informed decisions regarding network infrastructure and resource allocation.
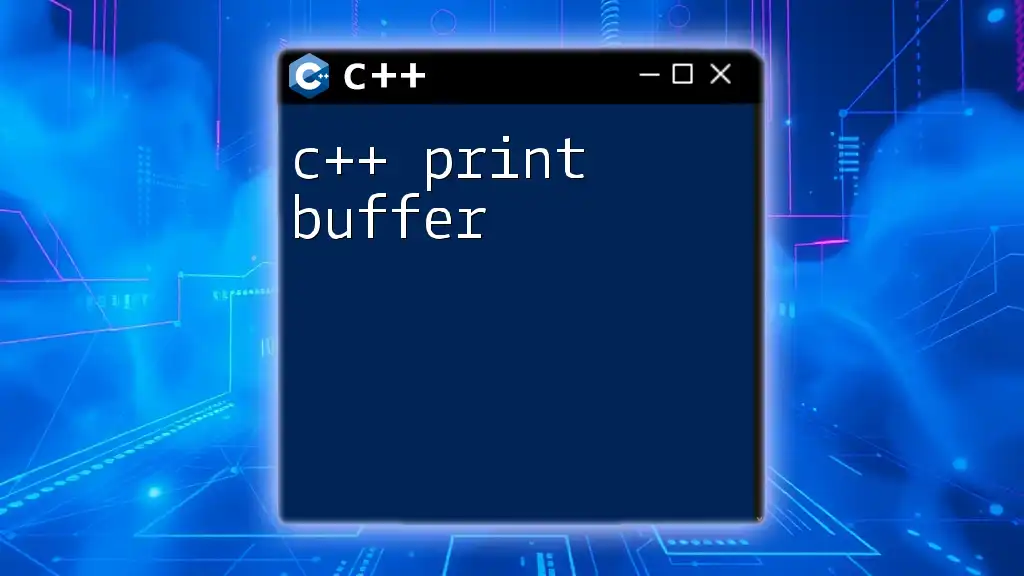
Troubleshooting Common Issues
Compilation Errors
Compilation issues related to missing headers or libraries are common when setting up your environment. Ensure that the pcap library is correctly linked in your project settings, and pay attention to your include paths.
Permission Issues on Packet Capture
Many operating systems restrict direct access to network devices. On Unix-like systems, you may need to run your sniffer with elevated permissions. This typically involves using `sudo` when executing your application. On Windows, ensure that your application is running with administrator privileges.
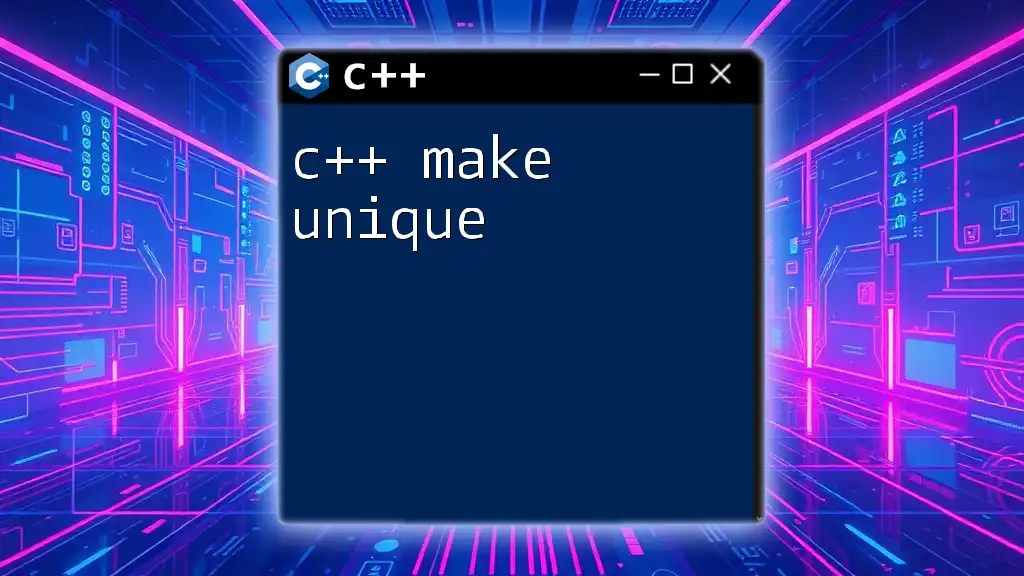
Conclusion
In summary, creating a C++ packet sniffer allows for powerful network analysis, monitoring, and security assessments. By understanding the basics of networking, getting comfortable with C++, and utilizing the pcap library, you can build effective tools to capture and analyze network traffic. Don't hesitate to experiment with additional features, and further your knowledge in C++ programming and networking concepts for a deeper understanding of how data moves across the web.
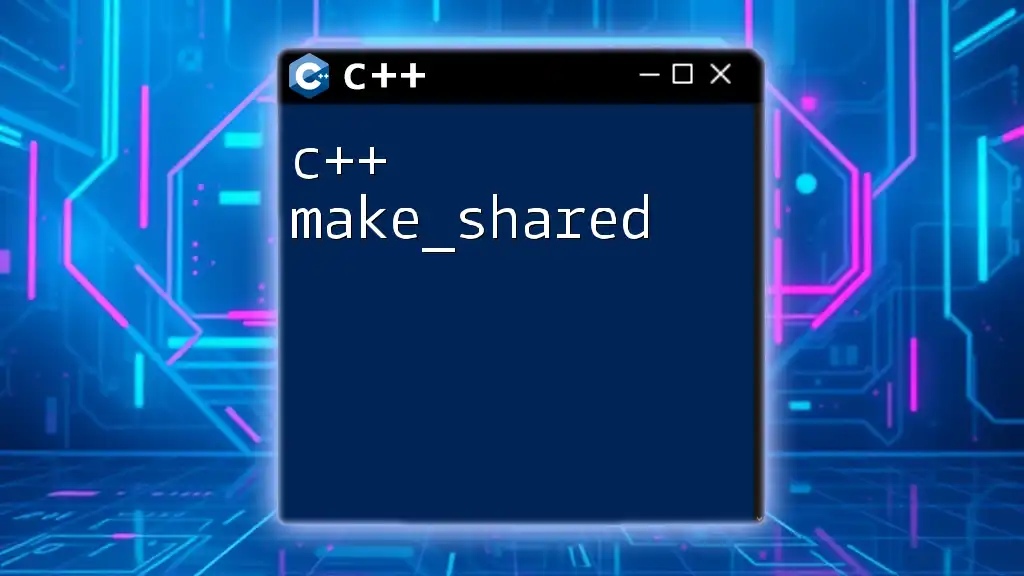
Call to Action
If you're interested in further tutorials or personalized guidance on your programming journey, feel free to reach out! Join our community and enhance your skills in C++ and packet sniffing today.