C++ scope refers to the visibility and lifetime of variables and functions, determined by the block in which they are declared.
#include <iostream>
int globalVar = 10; // Global scope
void exampleFunction() {
int localVar = 20; // Local scope
std::cout << "Local Variable: " << localVar << std::endl;
}
int main() {
exampleFunction();
std::cout << "Global Variable: " << globalVar << std::endl;
// std::cout << localVar; // This would cause an error as localVar is out of scope
return 0;
}
Understanding Scope in C++
What is Scope?
Scope in programming refers to the region or context in which variables, functions, or objects are accessible. It plays a vital role in determining the visibility and lifetime of these entities. Understanding C++ scope is crucial for effective coding practices and avoiding common pitfalls like variable name conflicts.
Types of Scope in C++
Global Scope
Global scope refers to variables or functions that are declared outside of all functions and classes. They are accessible from any part of the program.
Example of Global Variables:
int globalVar = 10; // Global variable
void displayGlobal() {
std::cout << globalVar << std::endl; // Accessing global variable
}
In this example, `globalVar` is defined at a global level. It can be accessed in any function, including `displayGlobal()`. However, improper use of global variables can lead to difficulties in managing larger codebases due to potential name conflicts and unexpected changes.
Local Scope
Local scope restricts access to variables defined within a function. Once the function exits, these variables are destroyed.
Example of Local Variables:
void localScopeExample() {
int localVar = 5; // Local variable
std::cout << localVar << std::endl; // Accessing local variable
}
In this snippet, `localVar` is confined to the `localScopeExample()` function. Attempting to access it outside this context will result in an error, reinforcing the principle of variable encapsulation.
Function Scope
Function scope is strictly related to variables that exist within a specific function. These variables are not visible outside the function.
Example:
void functionScope() {
int count = 0; // Count is scoped to functionScope
}
// count cannot be accessed here.
// std::cout << count; // This would result in a compile error.
Variable `count` is declared in `functionScope` and can only be used within that function. Any attempt to access it from outside will lead to a compile error, demonstrating the strict limitations imposed by function scope.
Block Scope
Block scope refers to variables declared within a pair of braces `{}` in constructs such as loops or conditional statements.
Example:
void blockScopeExample() {
if (true) {
int blockVar = 20; // blockVar is scoped to this block
std::cout << blockVar << std::endl;
}
// blockVar cannot be accessed here.
// std::cout << blockVar; // This would result in a compile error.
}
In this case, `blockVar` is only accessible within the `if` statement block. Once the block ends, `blockVar` ceases to exist, which is a key aspect of block scope.
Class Scope
Class scope refers to variables and functions that are defined within a class. It governs the accessibility of class members.
Example:
class MyClass {
public:
static int staticVar; // Static member accessible via class name
void showVar() {
int classVar = 15; // Scoped to MyClass::showVar
std::cout << staticVar << " " << classVar << std::endl;
}
};
In the `MyClass`, `staticVar` can be accessed through the class itself while `classVar` is limited to the `showVar()` method. This structure allows for organized data management and promotes encapsulation.
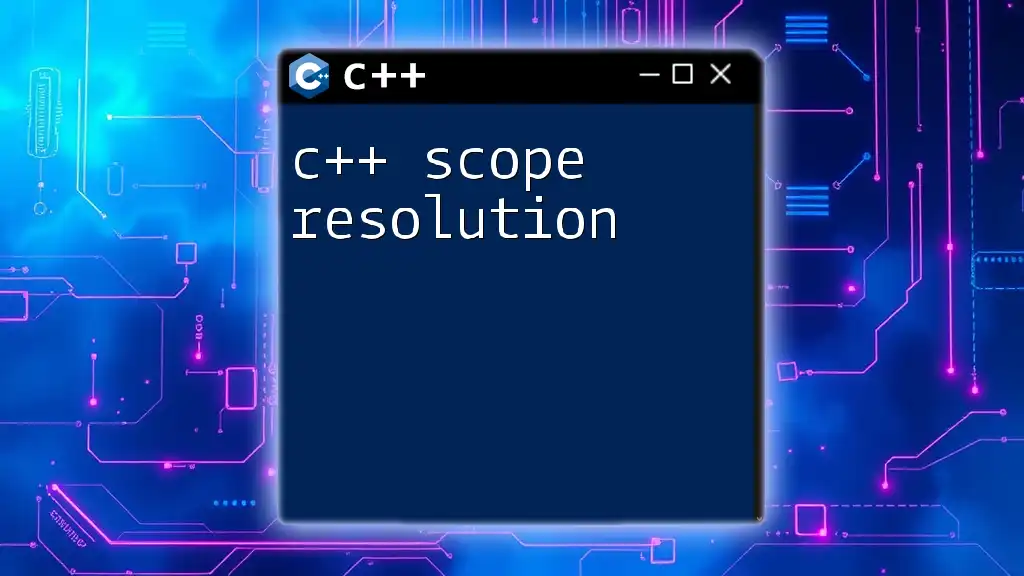
The Importance of Scope
Variable Lifetime and Accessibility
Scope directly affects the lifetime of variables. Variables defined in a narrower scope (such as local or block scope) only exist for as long as that context is active. Conversely, global variables have a lifetime that lasts for the duration of the program.
Best Practices for Scope Management
Utilizing scope effectively is essential for clean and maintainable code.
Encapsulation: Encapsulating variables within classes or functions helps prevent unauthorized access, which is beneficial when building scalable applications.
Avoiding Name Conflicts: Proper scoping can prevent name conflicts, especially in larger programs. By keeping variable names unique to their scope, you can reduce the chances of errors arising from unintentional variable shadowing.
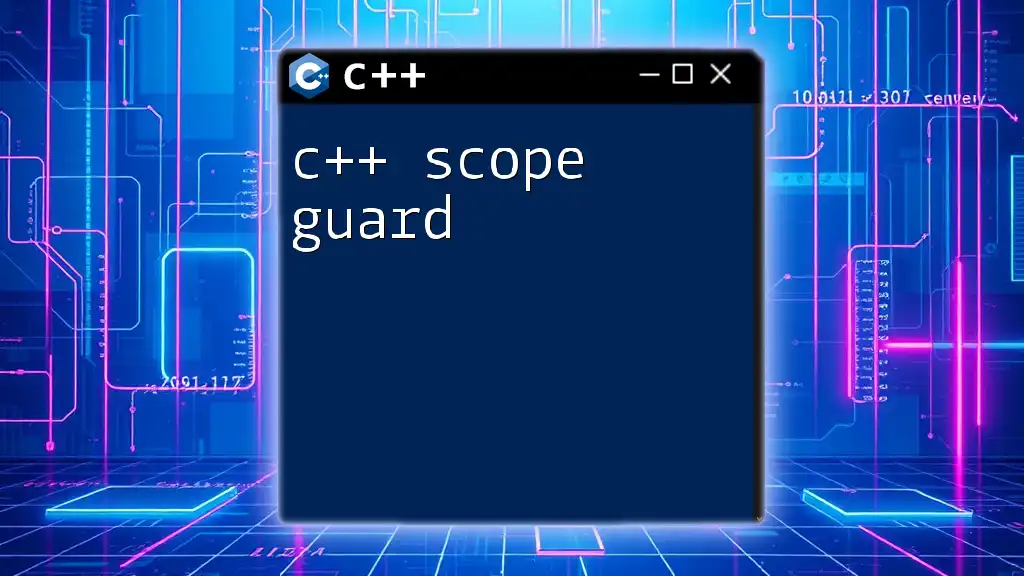
Common Scope-Related Errors
Shadowing Variables
Shadowing occurs when a variable is declared in a local scope with the same name as a variable in an outer scope. This can create confusion and lead to unexpected behaviors.
Example:
int shadowVar = 100;
void shadowingExample() {
int shadowVar = 50; // This shadows the global variable shadowVar
std::cout << shadowVar << std::endl; // Outputs 50
}
In this case, the local `shadowVar` within `shadowingExample` shadows the global `shadowVar`. When printed, it outputs `50` instead of `100`, underscoring the importance of knowing which variable is being referenced.
Access Violations
Access violations can occur if code attempts to access variables that are out of scope, leading to compiler errors. It’s crucial to adhere to scoping rules to prevent such violations, which can create debugging challenges.
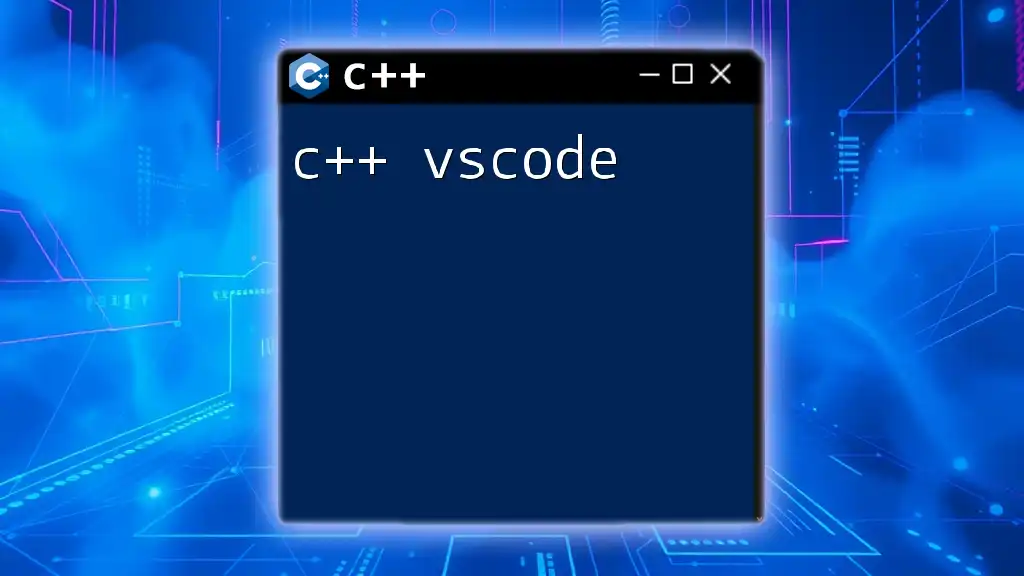
Advanced Scope Concepts
Name Resolution
C++ utilizes a mechanism known as name resolution to determine which variable or function is being called in case of naming conflicts across different scopes. It effectively prioritizes scopes, beginning with the most local and gradually moving outward.
Static and Dynamic Scopes
Unlike static scoping, where the scope is determined at compile-time, dynamic scoping allows visibility of variables based on the call stack at runtime. While C++ primarily uses static scoping, understanding the difference is important when working with languages that utilize dynamic scoping.
Using Namespaces for Scope Management
Namespaces provide a way to encapsulate identifiers and prevent naming collisions, especially in larger projects. By defining different namespaces, you can significantly improve organization and clarity in your code.
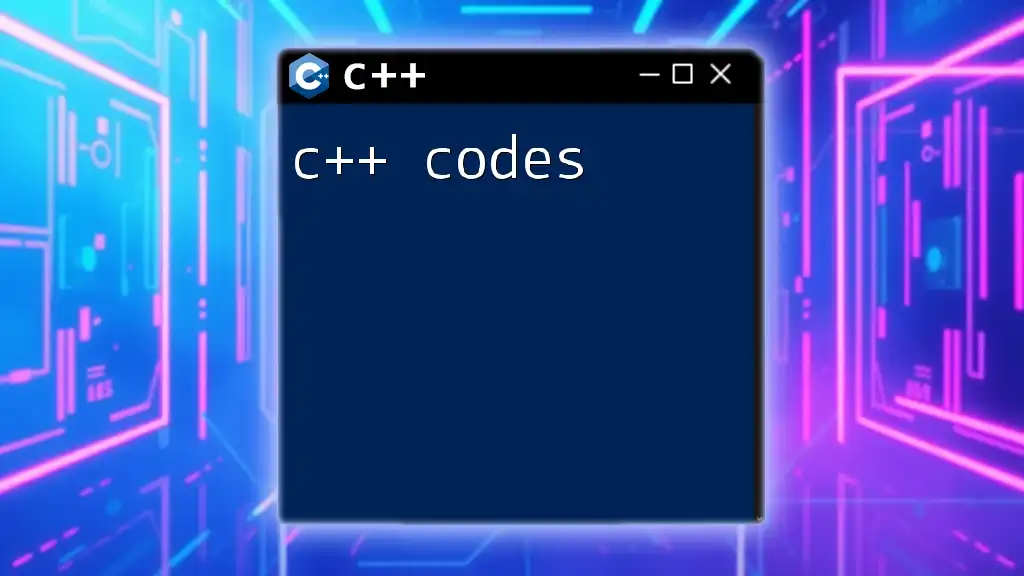
Conclusion
Understanding C++ scope is essential for effective coding and software development. Knowing the different types of scope, how to manage variable visibility, and the implications of scope-related errors equips programmers to write cleaner, more maintainable code. By practicing proper scope management, you can enhance your programming proficiency and build robust applications.
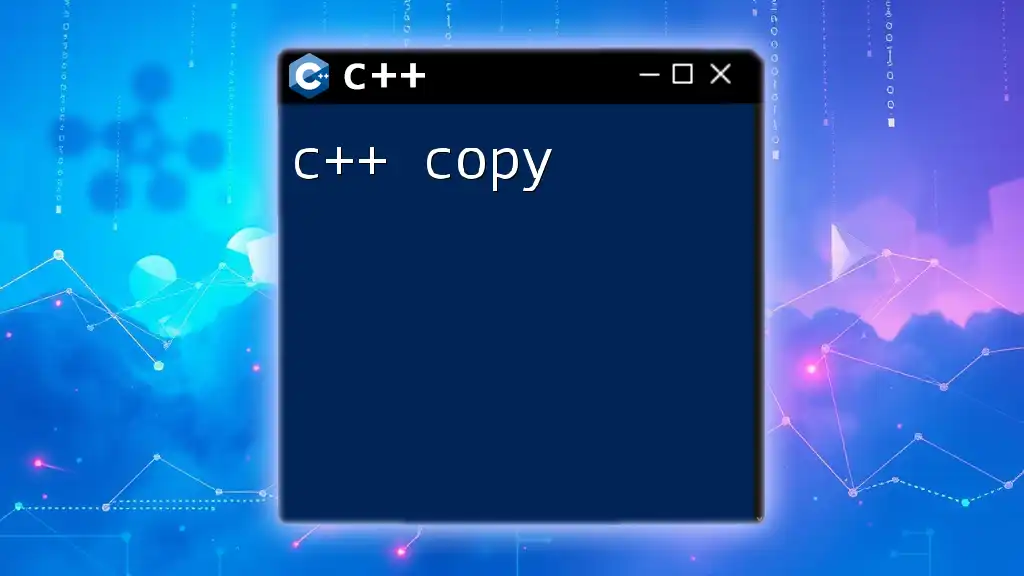
Additional Resources
For further reading on C++ scope, consider exploring the official C++ documentation, tutorials, or expert blogs highlighting best practices in C++. Engaging with these resources will deepen your understanding and aid in your learning journey.