A C++ scope guard ensures that specific cleanup actions are taken when a scope is exited, often used to manage resources without needing to write explicit cleanup code.
Here’s an example of a simple scope guard implementation:
#include <iostream>
#include <functional>
class ScopeGuard {
public:
explicit ScopeGuard(std::function<void()> onExit) : onExit_(onExit) {}
~ScopeGuard() { onExit_(); }
private:
std::function<void()> onExit_;
};
void example() {
ScopeGuard guard([]() {
std::cout << "Cleanup action performed." << std::endl;
});
std::cout << "Executing main logic." << std::endl;
// More code can go here
}
int main() {
example();
return 0;
}
Understanding Scope and Lifetime in C++
What is Scope?
In programming, scope refers to the context in which variables and resources are defined and accessible. In C++, scope determines the lifetime and visibility of variables. There are several kinds of scopes including global, local, and namespace scope, each with its unique rules governing variable accessibility.
The Concept of Scope Guards
A scope guard is a programming construct that helps automate resource management through the use of RAII (Resource Acquisition Is Initialization). The scope guard ensures that certain actions are taken automatically when the scope is exited, whether through normal execution or exceptions. This is especially critical in resource management as it prevents resource leaks, such as failing to release memory or closing file handles.
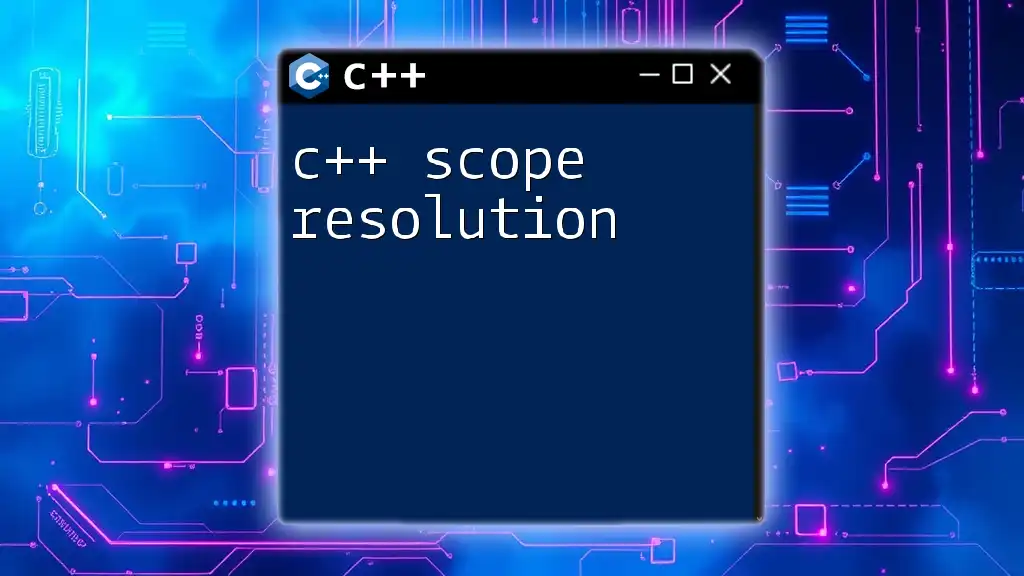
Implementing Scope Guards
Creating a Simple Scope Guard
To create a scope guard, we can define a class that executes a specified function when its instance goes out of scope. Here’s a straightforward implementation:
#include <functional>
class ScopeGuard {
public:
explicit ScopeGuard(std::function<void()> on_exit) : on_exit_(on_exit), dismissed_(false) {}
~ScopeGuard() { if (!dismissed_) on_exit_(); }
void dismiss() { dismissed_ = true; }
private:
std::function<void()> on_exit_;
bool dismissed_;
};
In this class, its destructor calls the on_exit function unless the guard is dismissed. The combination of `std::function` allows for flexible cleanup actions.
Example of Using Scope Guard
To illustrate how the C++ scope guard works, consider the following scenario involving a resource that must be released:
class Resource {
public:
void release() { /* Release the resource */ }
};
void doSomething() {
Resource res;
ScopeGuard guard([&res]() { res.release(); });
// Perform operations with res
// If an exception is thrown or the function exits prematurely,
// res will automatically be released.
}
In this case, once `doSomething()` exits—either normally or due to an exception—the `res.release()` method will automatically be called, ensuring proper cleanup without needing explicit management.
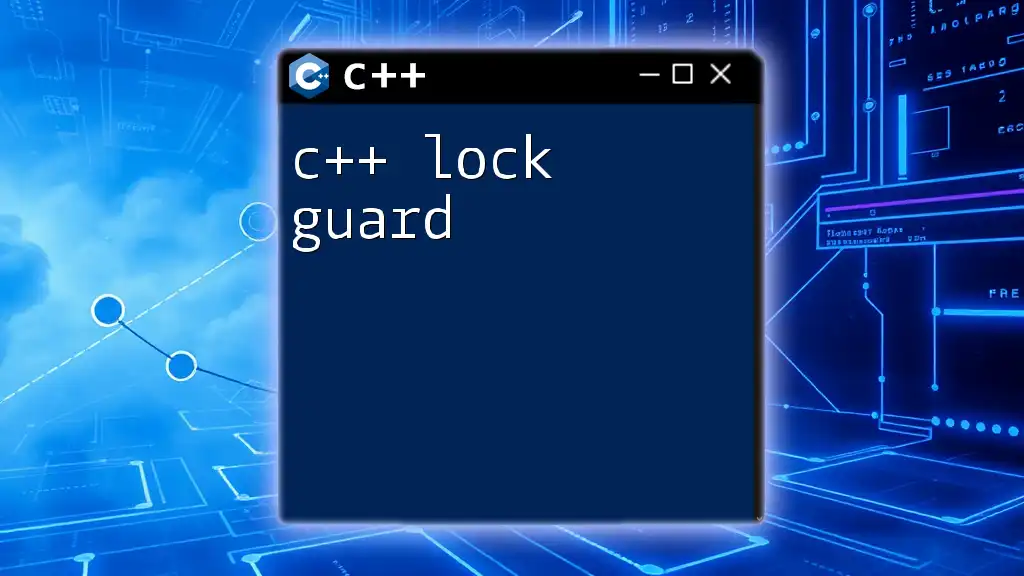
Advanced Usage of Scope Guards
Customizing Scope Guards
The functionality of a scope guard can be extended by adding additional parameters such as an initialization function that can run before the main action:
class CustomScopeGuard {
public:
CustomScopeGuard(std::function<void()> on_exit, std::function<void()> on_start = nullptr)
: on_exit_(on_exit), on_start_(on_start), dismissed_(false) {
if (on_start_) on_start_();
}
~CustomScopeGuard() { if (!dismissed_) on_exit_(); }
void dismiss() { dismissed_ = true; }
private:
std::function<void()> on_exit_;
std::function<void()> on_start_;
bool dismissed_;
};
Here, you can execute specific code when entering the scope, as well as the cleanup code upon exiting. This enhances the versatility of resource management.
Nested Scope Guards
When working with nested scopes, keep in mind that destructors are called in reverse order of construction. Here’s an example:
void nestedScopes() {
ScopeGuard guard1([]() { std::cout << "Guard 1 released"; });
{
ScopeGuard guard2([]() { std::cout << "Guard 2 released"; });
// guard2 will be released first when going out of scope
}
// guard1 will be released next
}
In this scenario, `guard2` is released before `guard1`, demonstrating how inner scopes are managed effectively using C++ scope guards.
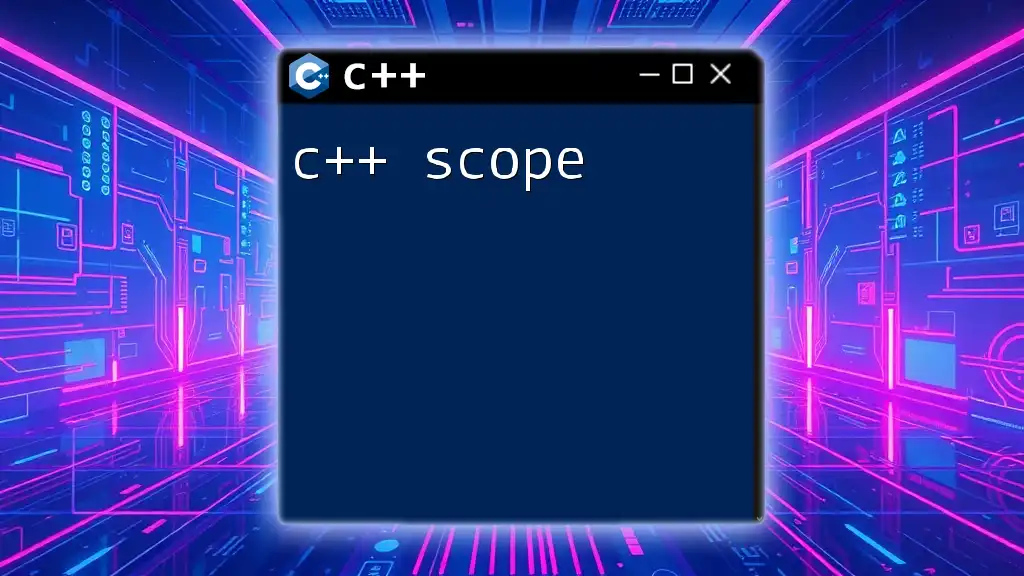
Comparing Scope Guards with Other Resource Management Techniques
Stack-Based Resource Management
When considering resource management, RAII is a key concept that ties closely to scope guards. Both techniques automatically clean up resources when the corresponding object goes out of scope, but scope guards allow for deferred cleanup actions specified at runtime. This flexibility makes them a preferred choice in certain scenarios.
Cleanup Functions vs. Scope Guards
Another alternative for cleanup is to use cleanup functions, such as those registered with `atexit`, but scope guards offer a more immediate and controlled way to manage resources. With scope guards, cleanup happens precisely when the scope is exited, minimizing the chances of forgetting to release resources.
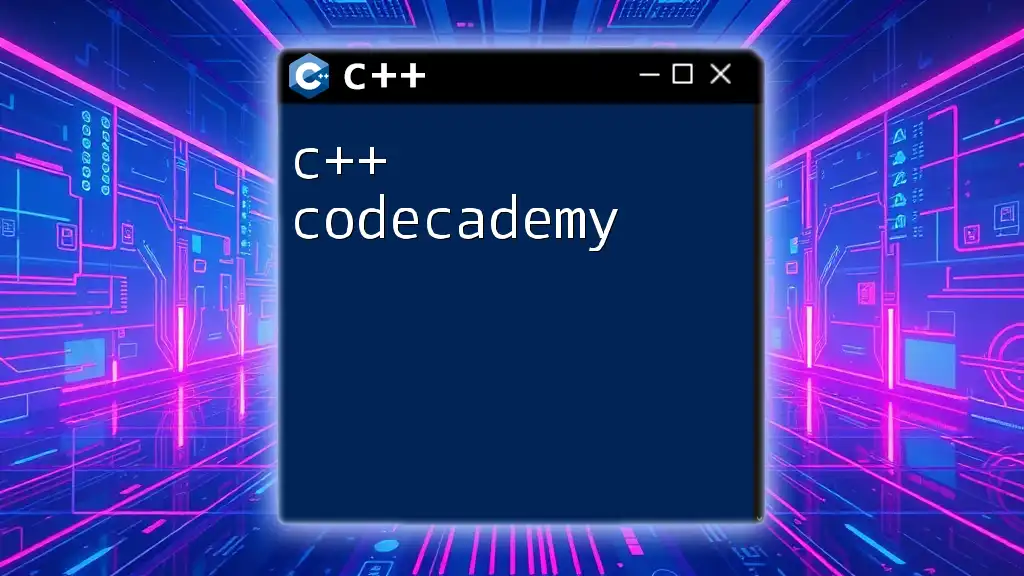
Best Practices for Using Scope Guards
When to Use Scope Guards
Scope guards are ideal in situations where resource management is critical, such as file handling, database connections, or memory management. They are particularly useful where exceptions may lead to premature exit from a scope, potentially causing resource leaks.
Guidelines for Implementation
To ensure effective use of scope guards, consider adopting the following best practices:
- Use lambda functions: They provide a clean, concise way to define cleanup actions.
- Keep scope guards small: Restrict their usage to simple cleanup tasks, keeping your code maintainable.
- Use dismiss() judiciously: Only call `dismiss()` if you are sure that the cleanup action is not needed, such as when a resource has already been managed elsewhere.
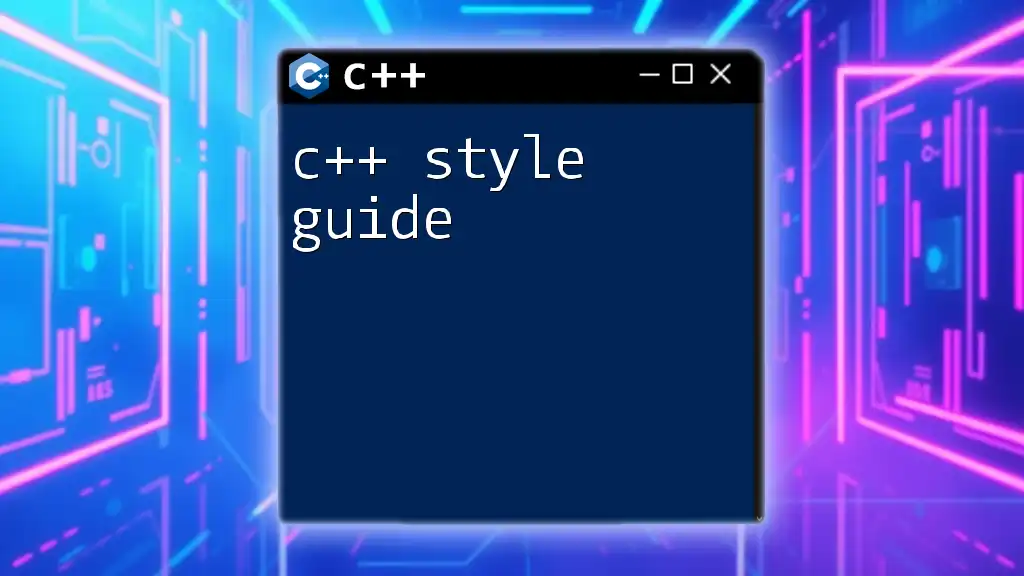
Conclusion
C++ scope guards provide a powerful tool for automatic resource management, greatly enhancing the safety and cleanliness of your code. By ensuring that resources are properly released when a scope exits, they help prevent memory leaks and other resource management issues.
Implementing scope guards in your C++ programming can lead to cleaner and more maintainable code, so be sure to incorporate them into your projects. Start small, experiment, and see how they make your codebase safer and more efficient.
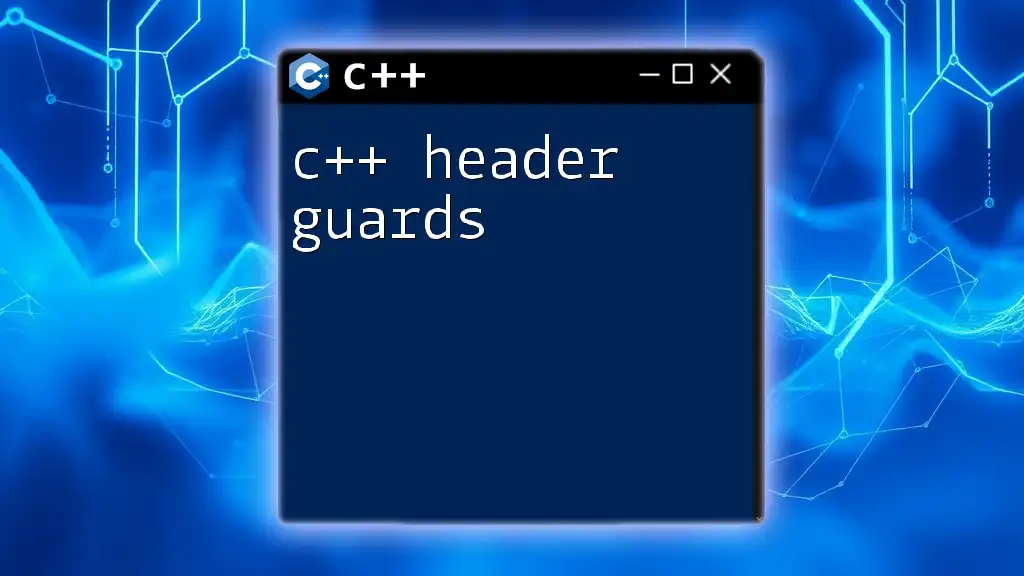
Additional Resources
For further reading on C++ and resource management, consider exploring articles on advanced C++ techniques, books like "Effective Modern C++" by Scott Meyers, or engaging in discussions on C++ forums.
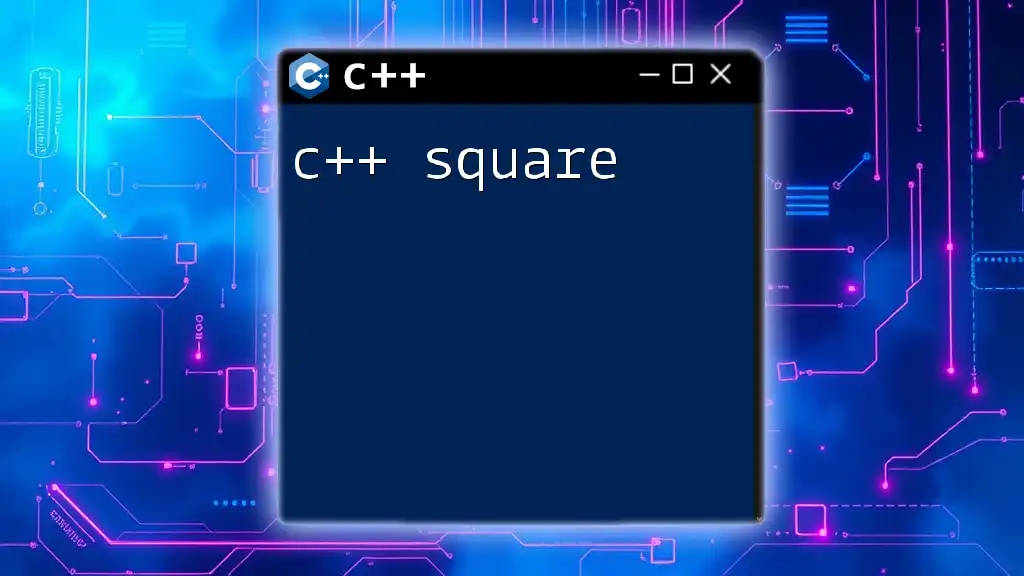
Frequently Asked Questions (FAQ)
What is the primary purpose of a scope guard?
The primary purpose of a scope guard is to ensure that specific actions, usually related to cleanup or resource release, are automatically executed when the scope is exited, whether due to normal flow or exceptions.
Can scope guards be used with exception handling?
Yes, one of the greatest strengths of scope guards is their ability to handle resource management seamlessly, even in the presence of exceptions, ensuring that resources are cleaned up regardless of how the scope exits.
Are there any performance implications of using scope guards?
Using scope guards typically incurs minimal overhead, as they leverage the existing stack unwinding mechanism in C++. The performance impact is negligible compared to the benefits of preventing leaks and maintaining cleaner code.