In C++, the `!=` operator is used to determine if two values are not equal, returning a boolean value of `true` or `false` based on the comparison.
#include <iostream>
int main() {
int a = 5;
int b = 10;
if (a != b) {
std::cout << "a and b are not equal." << std::endl;
} else {
std::cout << "a and b are equal." << std::endl;
}
return 0;
}
Understanding Equality in C++
The Concept of Equality
In programming, equality is a fundamental concept that allows us to compare values and determine their relationships. A solid understanding of equality helps in making logical decisions in code, essential for tasks ranging from conditional statements to complex algorithms.
Types of Equality in C++
When it comes to C++, there are two primary types of equality:
Value Equality refers to comparing the values stored in variables. It checks whether two variables hold the same value.
int a = 5;
int b = 5;
if (a == b) {
std::cout << "Equal values!" << std::endl; // Output: Equal values!
}
Reference Equality examines whether two references point to the same memory location. This can be crucial, especially when dealing with pointers or objects.
int a = 5;
int *p1 = &a;
int *p2 = &a;
if (p1 == p2) {
std::cout << "Same reference!" << std::endl; // Output: Same reference!
}
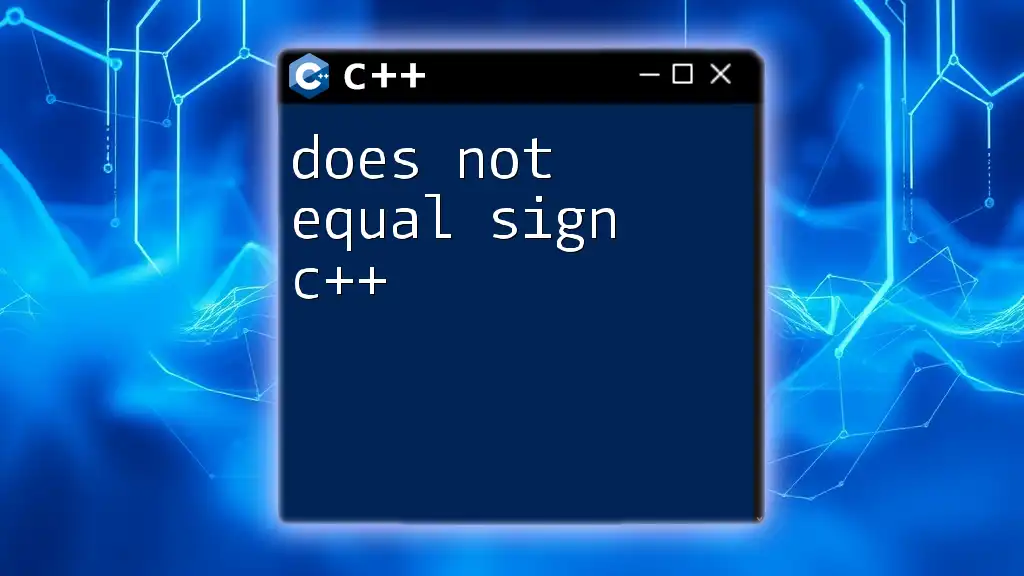
C++ Does Not Equal: `!=` Operator
Purpose of the `!=` Operator
The `!=` operator is crucial in C++ as it allows programmers to evaluate inequality. It checks if two values are not equal to each other, thereby facilitating decision-making in various scenarios, such as loops, conditionals, and data validation.
Using `!=` in C++
Employing the `!=` operator is straightforward and can be illustrated with a simple code example.
int a = 5;
int b = 6;
if (a != b) {
std::cout << "Values are not equal!" << std::endl; // Output: Values are not equal!
}
Practical Examples include comparing different types of variables. Consider, for instance, comparing an integer to a character code or a string, which could lead to interesting results.
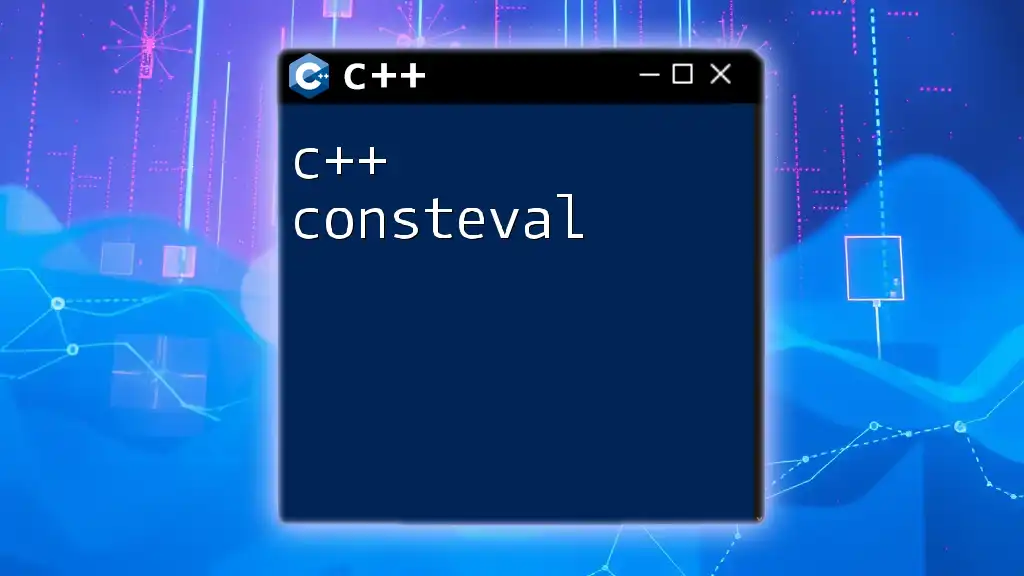
Common Pitfalls with the `!=` Operator
Comparing Different Data Types
One common mistake programmers may encounter is comparing values of different data types. C++ handles type conversion in specific ways, and a misunderstanding here can lead to unexpected results.
int a = 5;
double b = 5.0;
if (a != b) {
std::cout << "C++ considers them equal!" << std::endl; // Output: This line won't execute.
}
In this example, `a` and `b` are compared, but C++ sees them as equal due to its implicit type conversion rules.
Neglecting Operator Overloading
C++ allows for operator overloading, enabling custom behavior for operators like `!=`. Failure to consider how custom types interact with the `!=` operator can result in logical errors.
class Box {
public:
int size;
bool operator!=(const Box &other) const {
return size != other.size;
}
};
Box box1, box2;
if (box1 != box2) {
std::cout << "Boxes are not equal in size!" << std::endl;
}
In this case, the custom `!=` operator allows for coherent behavior when comparing `Box` objects based on their size.
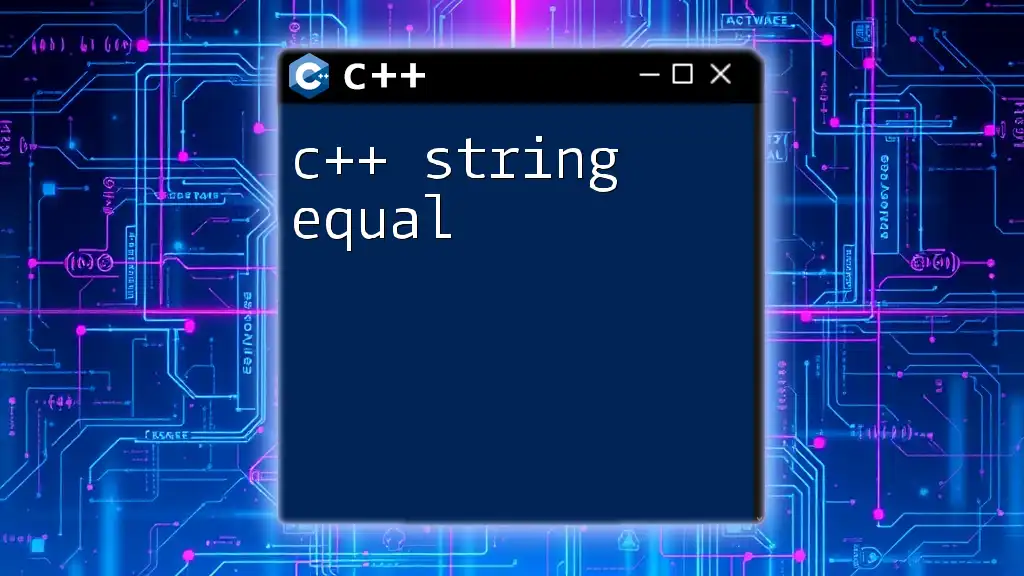
The Role of Boolean Logic in Comparisons
Understanding Boolean Expressions
Boolean expressions in C++ evaluate to either true or false, and they can be combined using logical operators such as `&&` (and), `||` (or), and `!` (not). This allows for complex conditional checks.
if ((a != b) && (b != 0)) {
std::cout << "A is not equal to B and B is not zero!" << std::endl; // Output depends on values of a and b.
}
This example demonstrates using multiple conditions to establish a logic that enhances the effectiveness of comparisons.
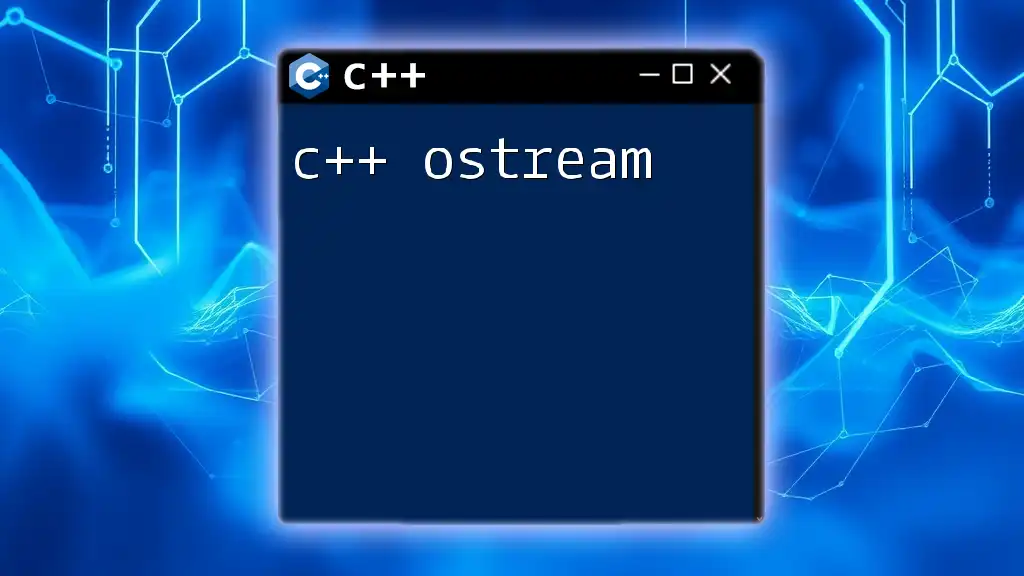
Importance of Data Type Consideration
Standard Data Types and Their Behaviors
C++ has a variety of standard data types (int, float, double, char, etc.), and it's essential to understand how each type behaves in comparisons. For instance, integers usually perform better in performance compared to floating-point types due to precision issues.
Implicit vs. Explicit Conversion
Type conversion is an essential consideration in C++. Implicit conversion occurs when the compiler automatically converts one type to another, which can result in confusion.
int a = 5;
double b = 5.0;
if (a != b) {
std::cout << "They are not considered equal!" << std::endl; // This won't execute.
}
This sort of implicit conversion can lead programmers to believe that variables are unequal when they are, in fact, treated as equal by C++.
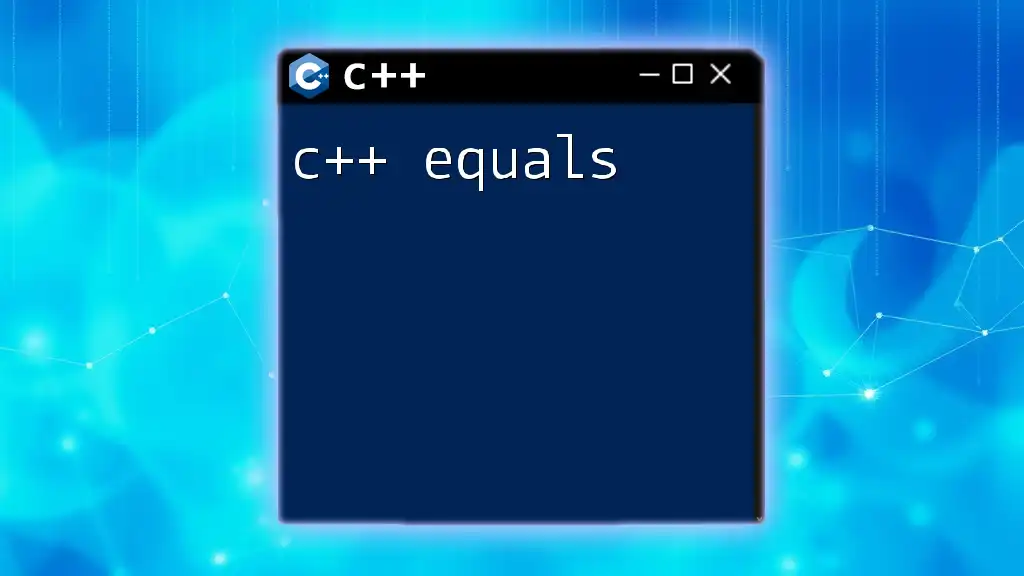
Best Practices for Using `!=` in C++
Clarity and Readability
Clarity and readability are critical in writing code that not only functions correctly but is also easy to maintain. When using comparison operators, strive for straightforward expressions that prioritize readability.
Avoiding Common Mistakes
Avoiding typical pitfalls in comparisons, such as overlooking data types or relying on implicit conversions, is vital. Practice thorough testing of conditions and use helper functions to ensure clarity in complex expressions.
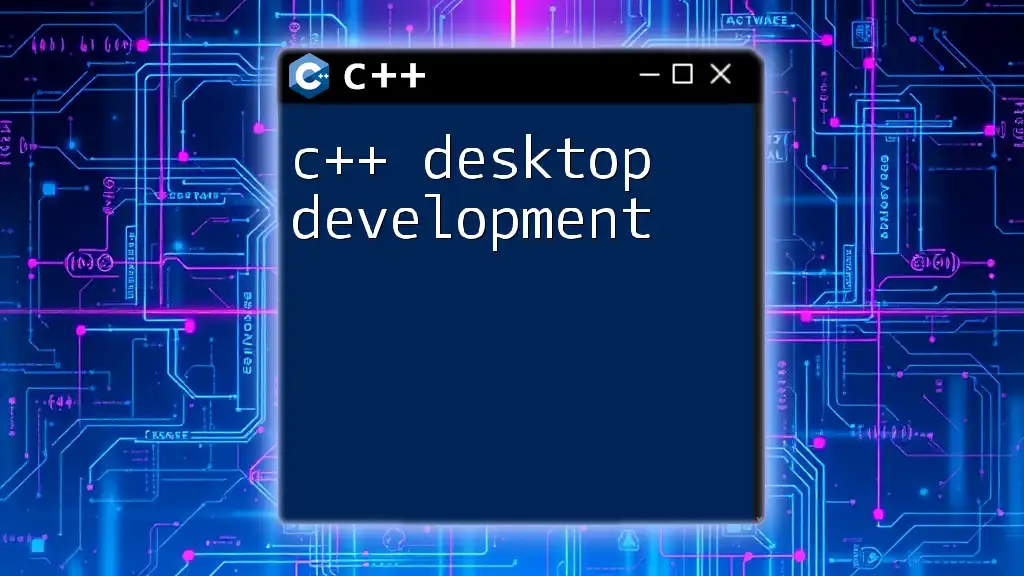
Conclusion
Understanding the nuances of C++ does not equal—particularly how the `!=` operator works—is critical for any developer. By being aware of value versus reference comparisons, potential pitfalls with data types, and using clear boolean logic, programmers can write more robust and maintainable code. As you continue your journey in C++, remember to practice these principles, and don't hesitate to seek out further resources to deepen your understanding of this powerful language.