C++ in NetBeans allows developers to efficiently write, compile, and debug C++ applications using an integrated development environment (IDE) that provides helpful features like code completion and project management.
Here's a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful, high-performance programming language that enables developers to create complex applications ranging from system software to game development. It is an extension of the C programming language, incorporating features such as classes and objects, which promotes the principles of object-oriented programming. C++ provides fine-grained control over system resources and memory management, making it a popular choice for applications that require performance optimization.
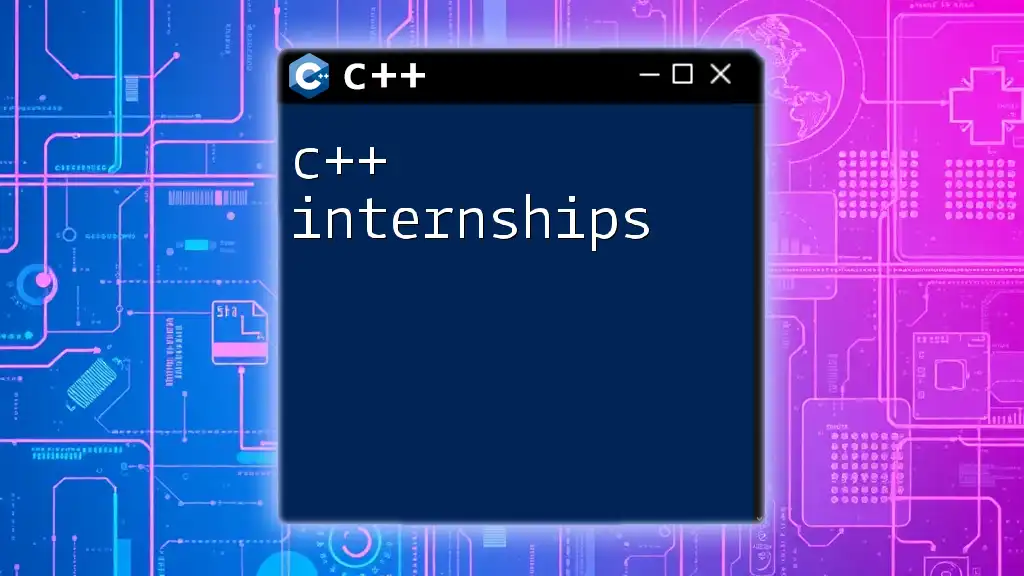
Why Use NetBeans for C++ Development?
NetBeans is a renowned open-source Integrated Development Environment (IDE) that supports multiple programming languages, including C++. Here are some compelling reasons to use NetBeans for your C++ projects:
- User-Friendly Interface: NetBeans has an intuitive interface that is easy to navigate, making it accessible for beginners while still being robust for experienced developers.
- Cross-Platform Compatibility: It runs on various operating systems, such as Windows, macOS, and Linux, allowing developers to work in their preferred environments.
- Integrated Tools and Features: NetBeans offers comprehensive tools that enhance productivity, including project management, debugging, and version control, streamlined all in one place.
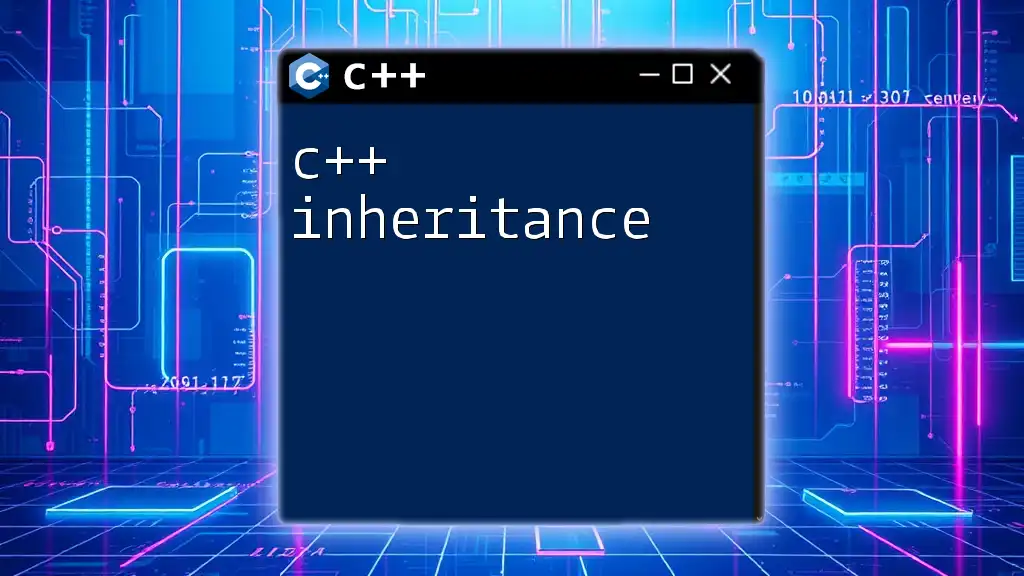
Setting Up NetBeans for C++
Downloading and Installing NetBeans IDE
To get started with C++ on NetBeans, the first step is to download the NetBeans IDE. Here's how to do it:
- Go to the official NetBeans website.
- Click on the download page and select the appropriate version for your operating system.
- Follow the prompts for installation. Ensure to include C/C++ plugins during the setup process.
Configuring NetBeans for C++ Development
After installation, you need to configure NetBeans to compile and run C++ code effectively:
- Set Up the C++ Compiler: Ensure that a compatible C++ compiler, such as GCC or MinGW, is installed on your system.
- Environment Variables: You may need to set the path for your compiler in the system’s environment variables.
This ensures that NetBeans can identify and utilize the C++ compiler during the build process.
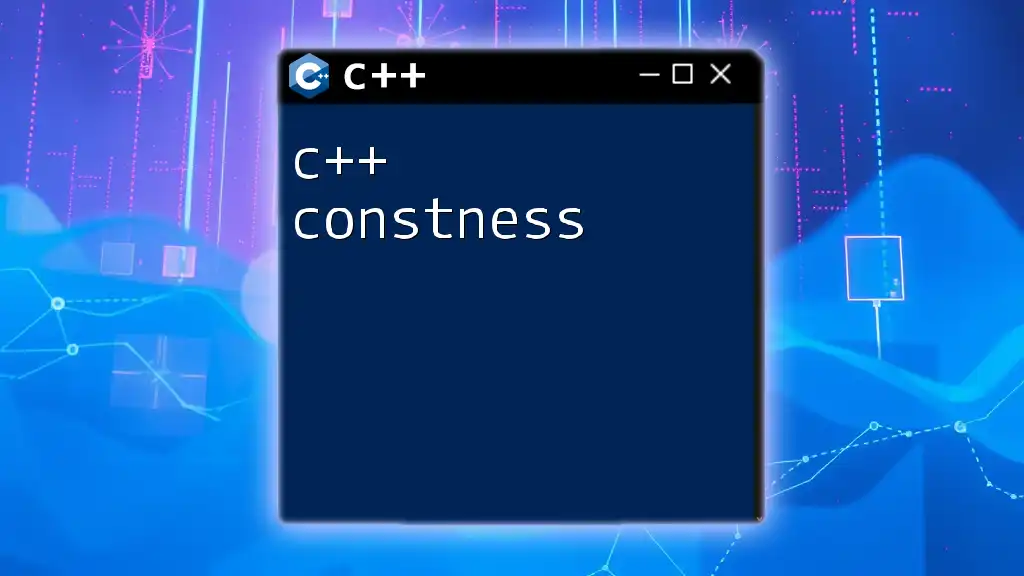
Creating Your First C++ Project in NetBeans
Starting a New Project
Begin your C++ journey by creating a new project in NetBeans:
- Open NetBeans and select File > New Project.
- Choose C/C++ > C/C++ Application and click Next.
- Provide a project name, location, and choose the appropriate toolchain.
Writing Your First C++ Program
Now, it's time to write your first C++ program! Follow these steps:
- Navigate to the project explorer and find the main.cpp file generated by NetBeans.
- Modify the file with the following sample code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this simple program:
- `#include <iostream>` includes the library for input/output stream operations.
- The `main` function is the entry point of any C++ program.
Building and Running the Program
To see the output of your code, you need to build and run it:
- Click on the Run button (or press F6).
- The output will appear in the output console.
If errors arise, they will be displayed in the output window. Common mistakes include missing semicolons or incorrect syntax.
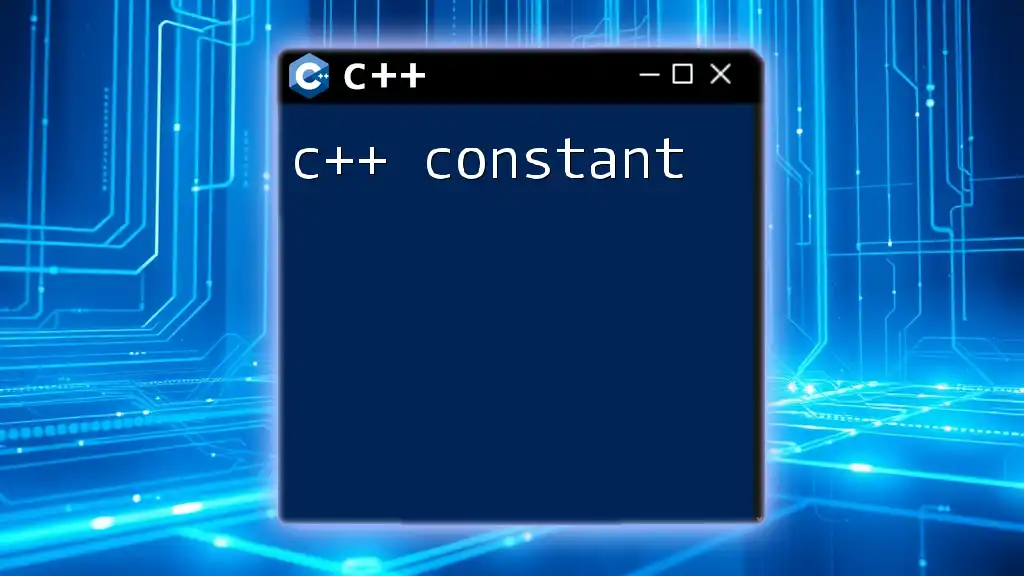
Navigating the NetBeans IDE
Understanding the Interface
The NetBeans IDE provides a seamless environment for C++ development. Familiarize yourself with these key components:
- Editor: The main area where you write your code, featuring syntax highlighting.
- Project Explorer: Here, you can manage your project files and resources.
- Output Console: Displays console output and error messages, allowing for quick debugging.
Utilizing Code Assistance Features
One of the advantages of using an IDE like NetBeans is its extensive coding assistance:
- Auto-completion: As you type, NetBeans suggests completions for identifiers, enhancing coding speed and reducing errors.
- Code hints: Helpful hints appear as you hover over code snippets, aiding in understanding libraries and functions.
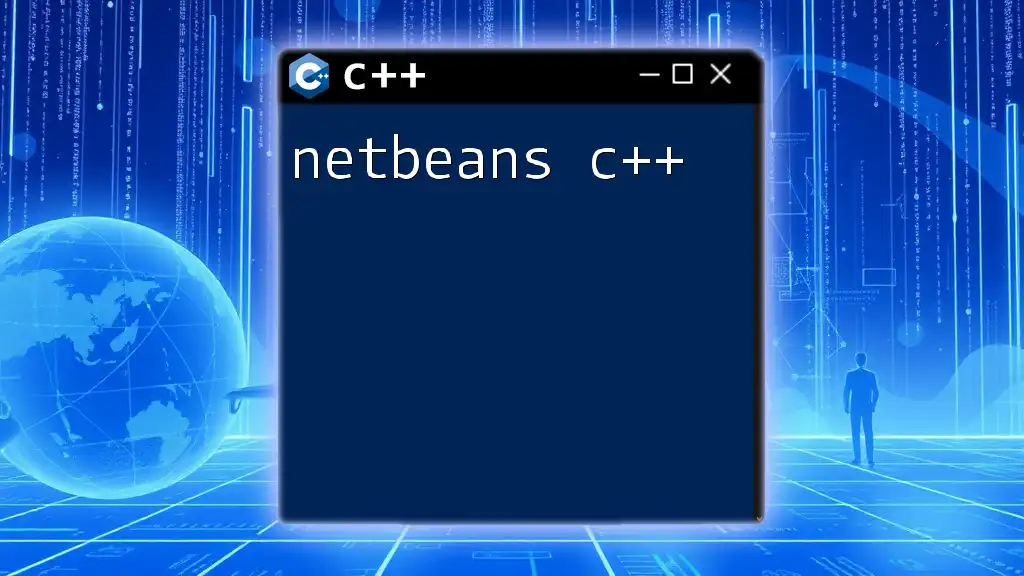
Debugging C++ Programs in NetBeans
Introduction to Debugging
Debugging is a crucial aspect of programming that allows you to identify and correct errors in your code. With NetBeans, you have powerful debugging tools at your disposal.
Setting Breakpoints and Running the Debugger
To start debugging your program:
- Click on the left margin next to the code line where you want to set a breakpoint.
- Right-click your project and select Debug.
Analyzing Variables and Program Flow
Once the debugger is running, you can inspect the program’s behavior:
- Watch variables: You can add variables to the watch list to monitor their values throughout the program's execution.
- Stack traces: Understanding the stack trace helps you see the function calls leading to a particular point in your application, crucial for diagnosing issues.
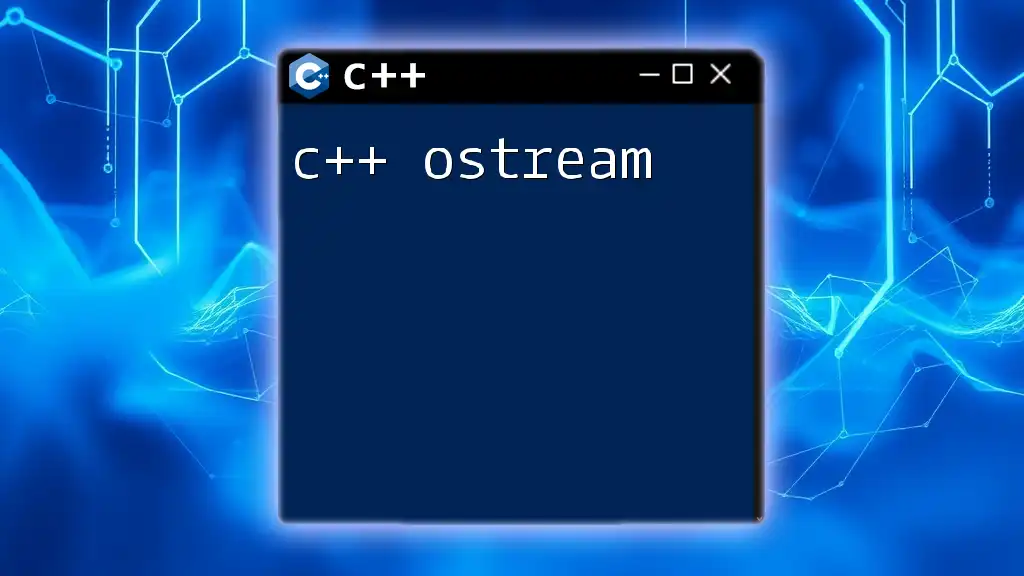
Advanced C++ Features in NetBeans
Using Libraries and Frameworks
For more complex applications, you may want to include external libraries. Here’s how:
- Navigate to the project properties and select the Libraries section.
- Choose Add Library and include the necessary files.
For example, using the Standard Template Library (STL) can significantly enhance your coding efficiency with data structures and algorithms.
Integrating with Version Control
Using version control systems is vital for any development project. NetBeans provides Git integration, allowing you to manage your code effectively:
- Create a local repository directly from the project explorer.
- Commit changes, create branches, and push to remote repositories seamlessly from the IDE.
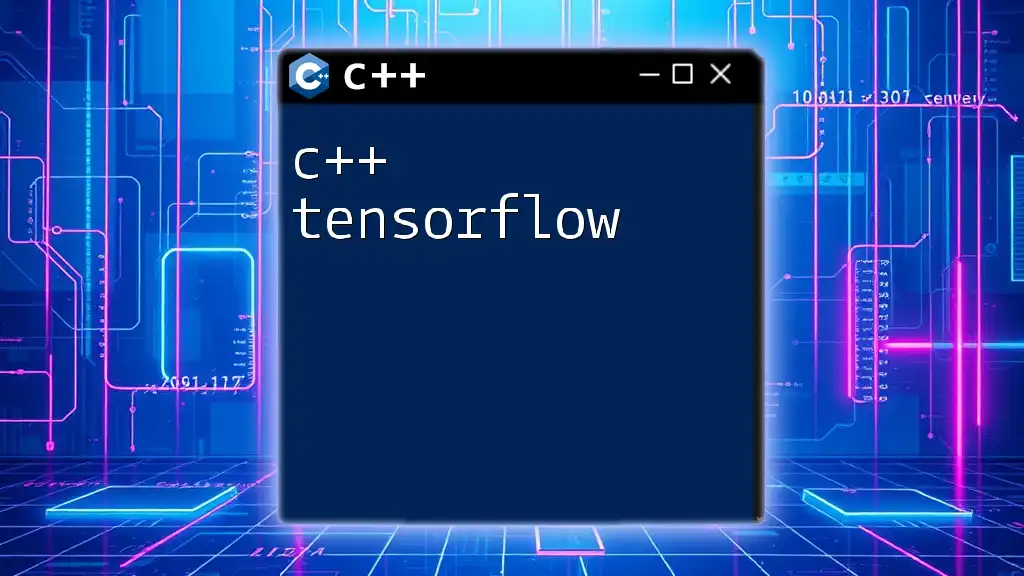
Best Practices for C++ Development in NetBeans
Writing Clean and Maintainable Code
Writing maintainable code is paramount:
- Use clear and descriptive variable and function names to enhance readability.
- Incorporate comments that explain the logic behind complex sections of your code. This is invaluable for future changes or for other team members reviewing your work.
Performance Optimization Techniques
Optimizing C++ code is crucial for ensuring high performance:
- Explore loops and conditional statements to minimize unnecessary computations.
- Utilize profiling tools available in NetBeans to identify performance bottlenecks and optimize accordingly.
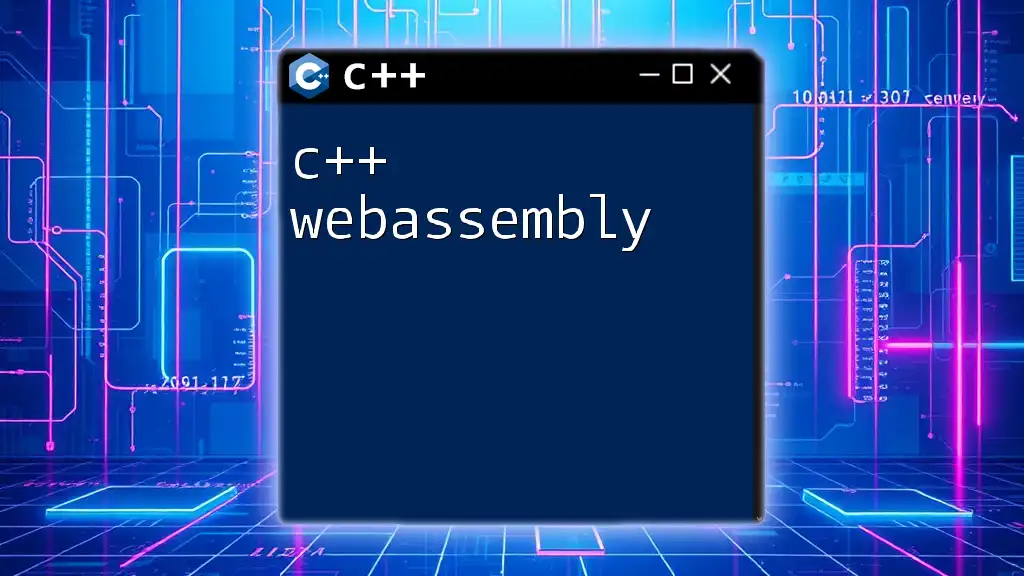
Conclusion
In this guide, we've covered the essentials of leveraging C++ on NetBeans. From setting up your environment, creating your first project, and mastering debugging techniques to more advanced features, you now have a solid foundation to further your C++ development skills.
Whether you're just starting or looking to deepen your existing knowledge, consistent practice and exploration of NetBeans will aid in mastering C++. The journey into C++ and NetBeans will be rewarding, opening doors to endless project possibilities. Explore the resources available, engage with the community, and keep coding!
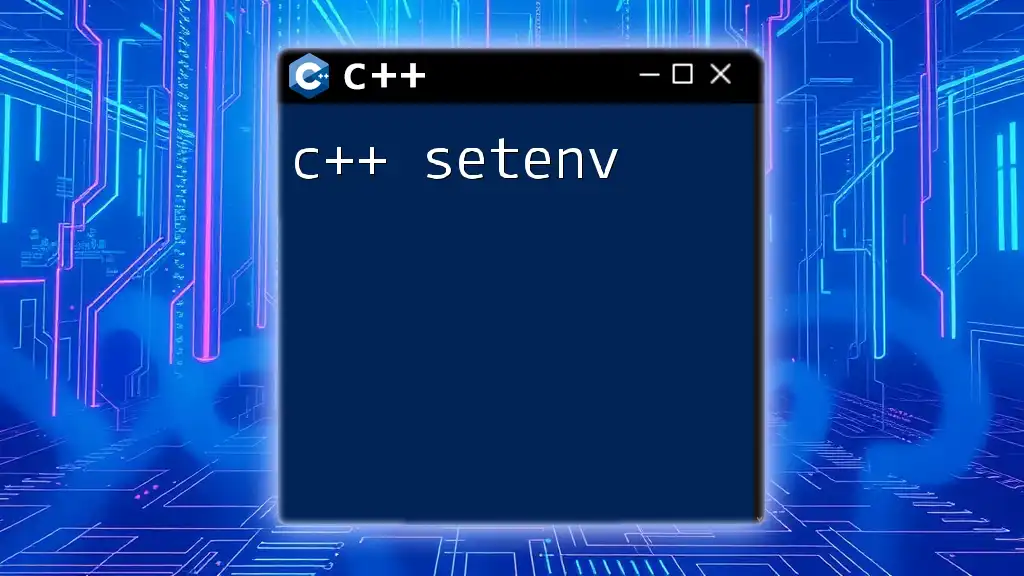
Additional Resources
To expand your learning, utilize the NetBeans and C++ documentation available online, and engage with community forums for support. Connecting with other C++ enthusiasts can provide invaluable insights and foster collaborative growth in your programming journey.