To convert a string from uppercase to lowercase in C++, you can use the `transform` function along with `tolower` for each character in the string.
#include <iostream>
#include <algorithm>
int main() {
std::string str = "HELLO WORLD";
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
std::cout << str << std::endl; // Output: hello world
return 0;
}
Understanding Character Encoding
What is ASCII?
ASCII (American Standard Code for Information Interchange) is a character encoding standard that represents text in computers. Each character—whether it’s a letter, number, or symbol—is given a specific numerical value that the system understands. For example, the uppercase letter 'A' corresponds to the ASCII value 65, while its lowercase counterpart 'a' corresponds to the value 97.
Understanding ASCII is crucial when converting from uppercase to lowercase, as the conversion involves a simple arithmetic operation: subtracting 32 from the ASCII value of an uppercase letter will yield the corresponding lowercase letter. This principle forms the foundation of many case conversion techniques.
The Role of Unicode
While ASCII covers basic English characters, Unicode encompasses a much wider range of characters, symbols, and scripts from languages around the world. It ensures that your program can handle text in different languages and character sets. Therefore, when dealing with strings in C++, it's helpful to be aware of how Unicode can affect case conversion and string handling.

Methods for Converting Uppercase to Lowercase
Using the `tolower()` Function
The `tolower()` function is a part of the C++ Standard Library and is declared in the `<cctype>` header. This function is designed to convert a single character from uppercase to lowercase.
Syntax:
int tolower(int ch);
Example:
#include <iostream>
#include <cctype> // for tolower function
int main() {
char upper = 'A';
char lower = tolower(upper);
std::cout << "Uppercase: " << upper << " -> Lowercase: " << lower << std::endl;
return 0;
}
In this example, the `tolower()` function effectively converts 'A' to 'a'. This function only works with single characters, so it needs to be called repeatedly for a whole string, which leads us to the next method.
Using `std::transform` from `<algorithm>`
`std::transform` is a powerful function that allows you to apply a transformation to each element in a range. It can be utilized for converting entire strings from uppercase to lowercase.
Syntax:
template<class InputIterator, class OutputIterator, class UnaryOperation>
OutputIterator transform(InputIterator first1, InputIterator last1, OutputIterator result, UnaryOperation op);
Example:
#include <iostream>
#include <algorithm>
#include <cctype>
#include <string>
int main() {
std::string str = "Hello World";
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
std::cout << str << std::endl; // Output: hello world
return 0;
}
In this example, the `std::transform` function is used to convert each character in the string "Hello World" to its lowercase equivalent. The use of `::tolower` as the unary operation means that `std::transform` applies `tolower` to each character in the string efficiently, allowing for concise and clear code.

Handling Strings and Special Cases
Converting a Whole String
When you want to convert a complete string—and not just a single character—it's essential to loop through each character. This can be done using a simple `for` loop.
Example:
#include <iostream>
#include <string>
int main() {
std::string str = "C++ Programming!";
for (char &c : str) {
c = tolower(c);
}
std::cout << str << std::endl; // Output: c++ programming!
return 0;
}
In the above code, we iterate over each character in the string "C++ Programming!" and convert it to lowercase using the `tolower()` function. This demonstrates the flexibility of manual iteration when specific control is needed.
Dealing with Non-Alpha Characters
It’s important to note how C++ functions handle non-alphabetic characters. Both `tolower()` and `std::transform` ignore numbers, punctuation, and whitespace. This means no unwanted changes happen; for instance, the string "123! Hello" remains "123! hello" after conversion.
Case Sensitivity in C++
C++ is case-sensitive, meaning 'A' and 'a' are treated as distinct characters. This sensitivity is crucial to understand when performing string comparisons or searches. For instance, "HELLO" and "hello" will yield different results when compared directly unless you've converted both to the same case format.

Performance Considerations
Optimizing String Operations
When discussing performance, it’s essential to know which method to employ based on the context of your application. Using `std::transform` is typically more efficient and readable when processing entire strings as compared to using nested loops with `tolower()`. However, if you are working with very large strings, performance profiling may help in deciding the best approach.
It's worth remembering that while the ease of using library functions like `std::transform` is beneficial from a coding perspective, sometimes a custom solution can yield better performance based on the specific use cases.

Conclusion
Throughout this article, we explored multiple ways to convert uppercase to lowercase in C++. From using the straightforward `tolower()` function to applying the powerful `std::transform`, numerous options presented themselves for efficiently handling character case transformations. Understanding how to manipulate strings in C++ enhances your programming skills, allowing for cleaner and more readable code. Encourage your readers to experiment with these techniques as they embark on their C++ programming journey.
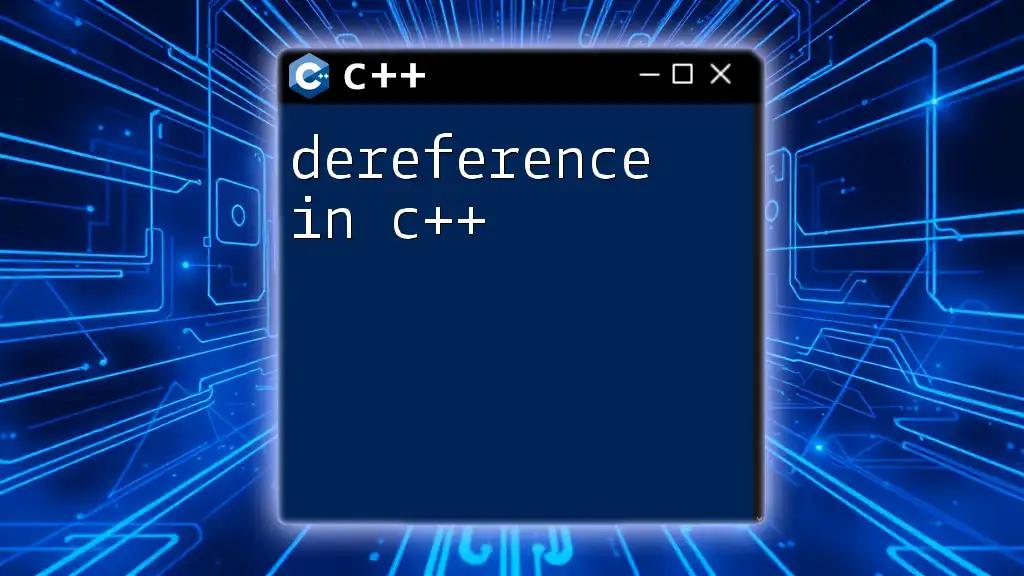
Further Reading and References
For readers wanting to delve deeper into the nuances of C++ string handling, consider checking the C++ documentation, exploring additional string manipulation techniques, or experimenting with more complex examples involving Unicode and advanced algorithms.