In C++, you can convert a `std::string` to a `std::wstring` by using the `std::wstring` constructor along with the `std::mbstowcs` function for proper encoding, as shown in the following code snippet:
#include <string>
#include <locale>
#include <codecvt>
std::string str = "Hello, World!";
std::wstring wstr = std::wstring_convert<std::codecvt_utf8<wchar_t>>().from_bytes(str);
Understanding C++ Strings and Wide Strings
What is a `std::string`?
`std::string` is a part of the C++ Standard Library and represents a sequence of characters. It is a versatile type that provides various utilities for working with text, such as concatenation, searching, and modification. Common use cases for `std::string` include:
- Storing user input
- Processing text files
- Implementing string manipulation functions
What is a `std::wstring`?
`std::wstring` is similar to `std::string` but is designed to work with wide characters. Each character in a `std::wstring` can represent Unicode characters, which makes it essential for applications that need to support internationalization and localization. Key differences and features include:
- Character Size: Wide characters take more memory, as they are typically 2 bytes or more depending on the system.
- Use Cases: `std::wstring` is particularly useful when dealing with languages that contain special characters or when working with Windows APIs that require wide strings.
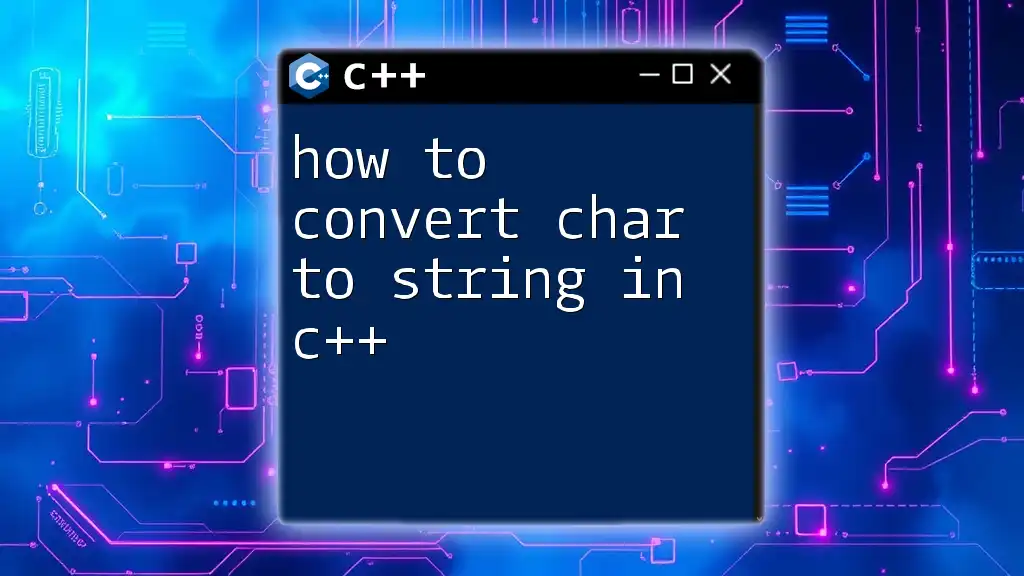
Why Convert `std::string` to `std::wstring`?
Conversion between these types is often necessary for several reasons:
- Unicode Support: Since `std::wstring` can handle a wider range of characters, converting to wstring allows programs to display text in various languages properly.
- Function Compatibility: Many APIs, especially in Windows, require `std::wstring` parameters, necessitating conversion when passing string data to these functions.
- Localization: Applications intended for global markets often need to display text in local languages, making it crucial to know how to convert string to wstring in C++.
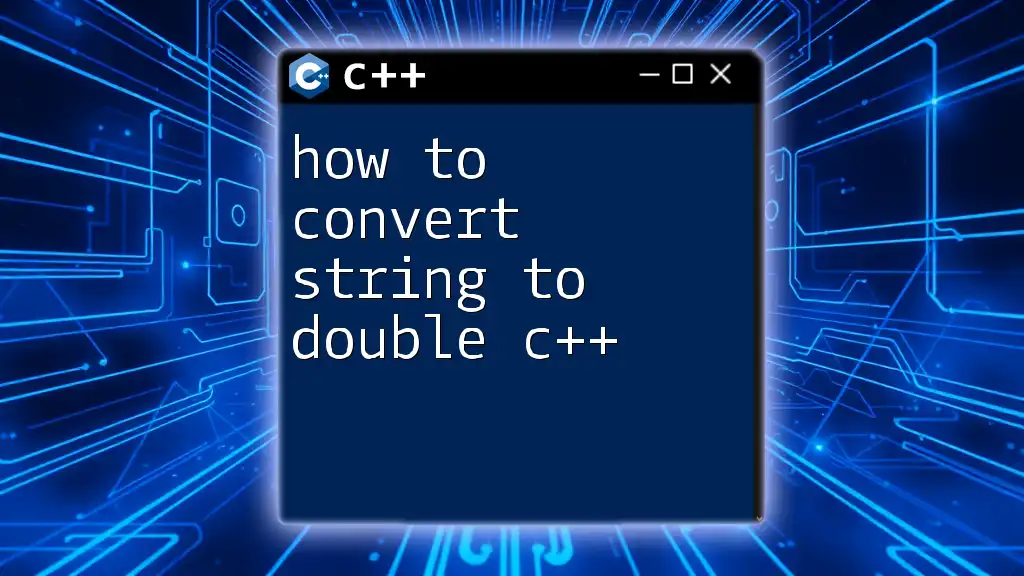
Methods to Convert `std::string` to `std::wstring`
Using `mbstowcs`
The `mbstowcs` function can be an effective way to convert a multibyte character string to a wide character string. This function provides a straightforward approach to handle the conversion.
#include <iostream>
#include <cwchar>
#include <string>
std::wstring convertMbstowcs(const std::string& str) {
size_t size = mbstowcs(nullptr, str.c_str(), 0); // Get required size
std::wstring wstr(size, L'\0'); // Create wstring with required size
mbstowcs(&wstr[0], str.c_str(), str.length() + 1); // Convert
return wstr;
}
Explanation of the code example:
In this example, the `mbstowcs` function first calculates the needed size for the `std::wstring`. It then creates a `std::wstring` of the appropriate size and performs the conversion using the same function again. Remember to handle null characters and ensure your input string is valid before using this method.
Using `std::wstring` Constructor
Another simple approach for converting `std::string` to `std::wstring` is to use the constructor of `std::wstring`. This method leverages the character iterators of the `std::string`.
std::wstring convertConstructor(const std::string& str) {
return std::wstring(str.begin(), str.end());
}
Explanation of the code example:
This method creates a new `std::wstring` directly from the range defined by the `std::string` iterators. It’s a quick and efficient way to convert string data. However, be aware that this method does not handle character encoding properly and may lead to issues if your string contains non-ASCII characters.
Using C++11 `std::codecvt_utf8_utf16`
With the advent of C++11, we can leverage `std::codecvt_utf8_utf16` to streamline the conversion process. This utility allows for proper handling of UTF-8 to UTF-16.
#include <locale>
#include <codecvt>
std::wstring convertCodecvt(const std::string& str) {
std::wstring_convert<std::codecvt_utf8_utf16<wchar_t>> converter;
return converter.from_bytes(str);
}
Explanation of the code example:
This code employs `std::wstring_convert` along with `std::codecvt_utf8_utf16` to handle the conversion comprehensively. It is recommended for modern C++ applications that require accurate character encoding conversion, ensuring characters are not lost during the process.
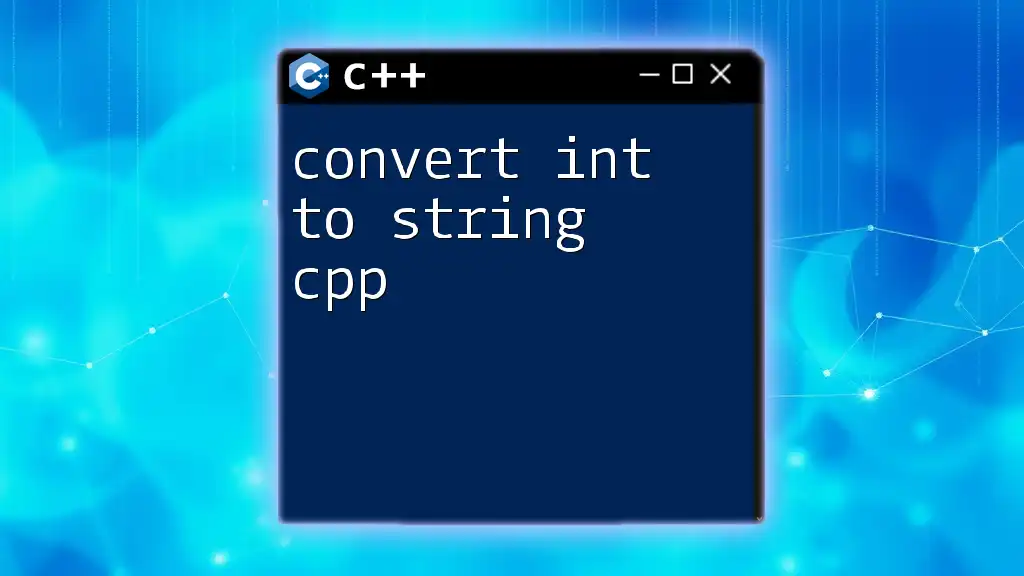
Performance Considerations
When to Use Each Method
Selecting the right method for conversion depends on specific application requirements. Here’s a brief overview:
- Using `mbstowcs`: Best for applications primarily concerned with the C locale and needing simple conversions.
- Using Constructor: Quick and easy for straightforward cases but may not manage character encoding adequately.
- Using `std::codecvt`: Ideal for extensive character handling, especially with multibyte encoded strings.
Efficient Memory Management
When converting large strings or in performance-critical applications, it is vital to:
- Preallocate Memory: When using `mbstowcs`, calculate the required size first to avoid unnecessary reallocations.
- Avoid Memory Leaks: Ensure all allocated resources are properly released after use to maintain optimal performance.
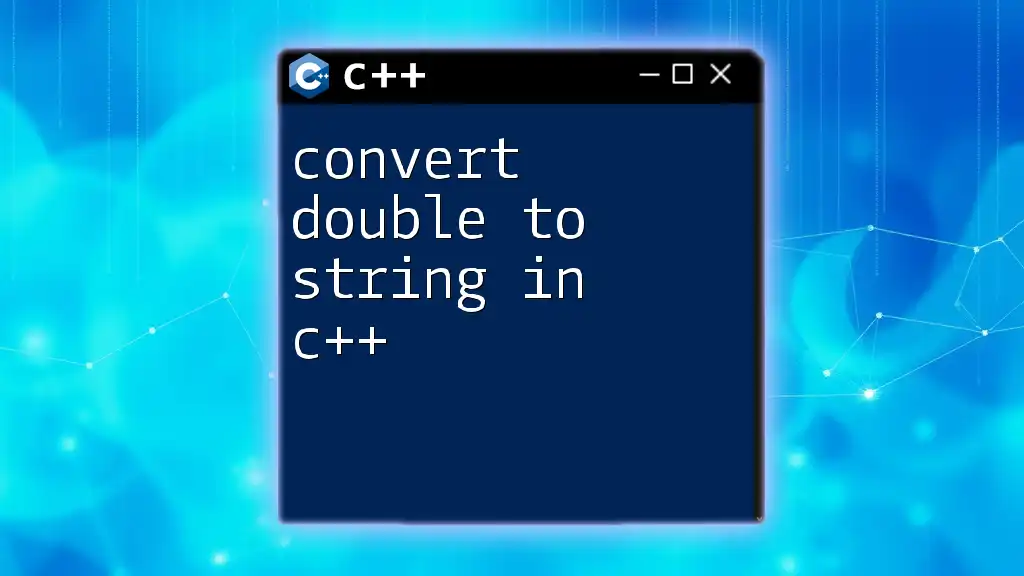
Common Pitfalls to Avoid
Safe Conversion Practices
To achieve successful conversions, consider the following:
- Handling Null Characters: Be especially cautious with strings containing null characters. Both `mbstowcs` and the constructor methods may not act as expected if the source string contains these characters.
- Managing Invalid Characters: Use exception handling or string validation to prevent runtime errors when encountering unexpected characters during conversion.
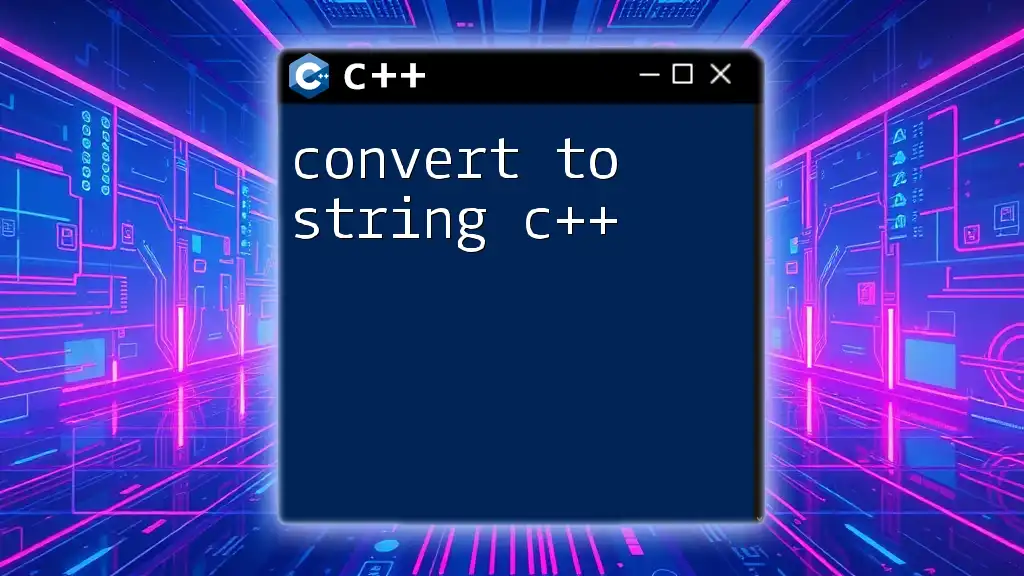
Conclusion
Understanding how to convert string to wstring in C++ is crucial for C++ developers, especially those working on internationalized applications. By knowing the various methods available—from using `mbstowcs` to employing C++11’s `std::codecvt`—developers can handle string data effectively, ensuring proper display and manipulations of text in different languages.
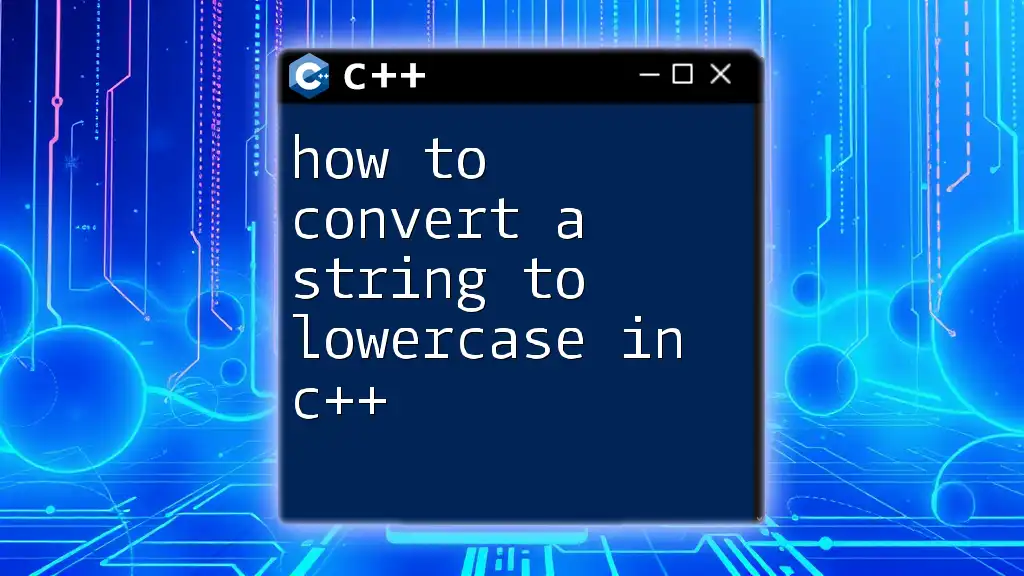
Frequently Asked Questions (FAQs)
Can I convert `std::wstring` back to `std::string`?
Yes, you can use functions such as `wcstombs` to perform the reverse conversion from `std::wstring` to `std::string`.
What should I do if I'm working with different encodings?
Identify the encoding of your input string and utilize corresponding conversion libraries or functions specifically designed for that encoding.
Are there any libraries that facilitate this process?
Yes, libraries like ICU (International Components for Unicode) facilitate robust handling of Unicode strings, providing assorted character conversion functions. These tools can simplify conversions and improve compatibility with various character sets.