In C++, a `constexpr` map allows for compile-time evaluation of key-value pairs, ensuring that the map is ready for use in constant expressions when the program is run.
Here's a simple example of a `constexpr` map using C++:
#include <array>
#include <utility>
template <typename K, typename V, std::size_t N>
struct ConstexprMap {
std::array<std::pair<K, V>, N> data;
constexpr V at(const K& key) const {
for (const auto& pair : data) {
if (pair.first == key) {
return pair.second;
}
}
throw std::out_of_range("Key not found");
}
};
constexpr auto myMap = ConstexprMap<int, const char*, 3>{
{ {1, "One"}, {2, "Two"}, {3, "Three"} }
};
// Usage
constexpr const char* value = myMap.at(2); // value is "Two"
Understanding `constexpr` in C++
What is `constexpr`?
`constexpr` is a keyword in C++ that stands for "constant expression." It allows developers to evaluate functions and variables at compile time, rather than at runtime. This capability can significantly enhance the performance of applications, particularly in scenarios where fixed values are repeatedly utilized. By designating a function or variable as `constexpr`, developers can ensure that the compiler will attempt to evaluate that expression at compile time, thus optimizing the resulting executable.
Unlike regular functions that can be executed at runtime, `constexpr` functions are meant for situations where the values are known beforehand. This specificity leads to improved efficiency and fewer runtime errors, creating a safer and more predictable codebase.
Benefits of Using `constexpr`
The primary advantages of using `constexpr` in C++ are:
-
Performance: The most significant benefit is the enhanced performance it brings. Compile-time computations can drastically reduce runtime overhead, especially in performance-critical applications. Functions that are marked as `constexpr` can yield results that the compiler will insert directly into the binary, minimizing function call overhead.
-
Safety: Compile-time evaluation reduces the potential for runtime errors as many errors can be caught at compile time. This results in more reliable code since the logic is verified and validated before execution.
-
Cleaner Code: Using `constexpr` often leads to simpler and more comprehensible code. The clarity added by having constant expressions available at compile time allows developers to focus on developing logic without handling mutable state unnecessarily.
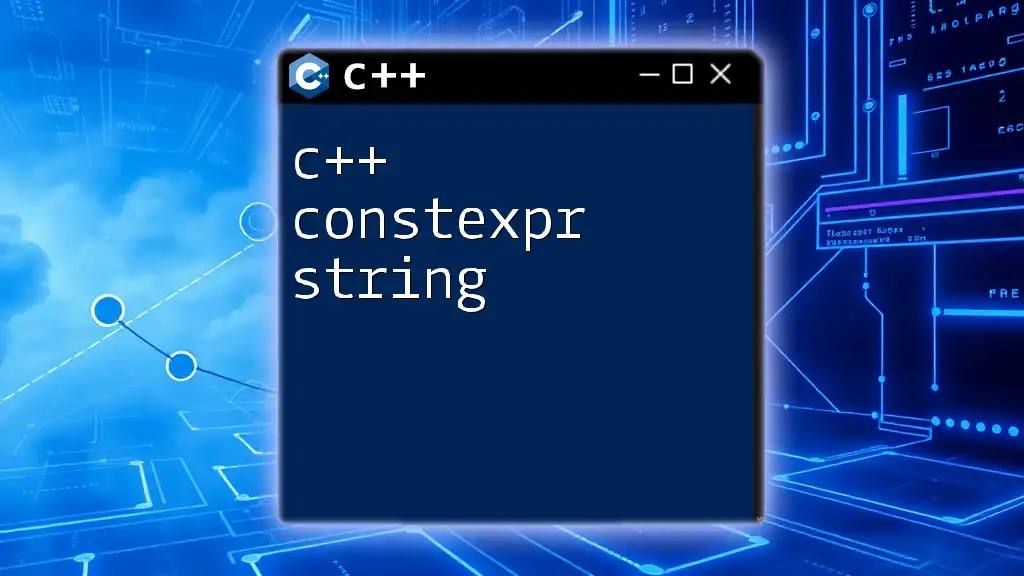
The Concept of Maps in C++
What is a Map?
In the realm of programming, a map is an associative container that holds key-value pairs. The key serves as a unique identifier, allowing the retrieval of the corresponding value efficiently. Maps provide efficient lookups, insertion, and deletion of elements. Common use cases include implementing dictionaries, caches, and any scenario where related data needs to be stored and accessed quickly.
Standard Library vs. `constexpr` Maps
In C++, the Standard Library provides containers such as `std::map` for creating maps with dynamic behavior, allowing keys to be added or removed at runtime. However, when needing constant key-value pairs, integrating `constexpr` maps proves beneficial.
`constexpr` maps, as defined in compile-time expressions, provide a major efficiency advantage in scenarios where the mappings are static and known at compile time. They enable predictable performance and optimized memory usage, atop the conventional `std::map`.
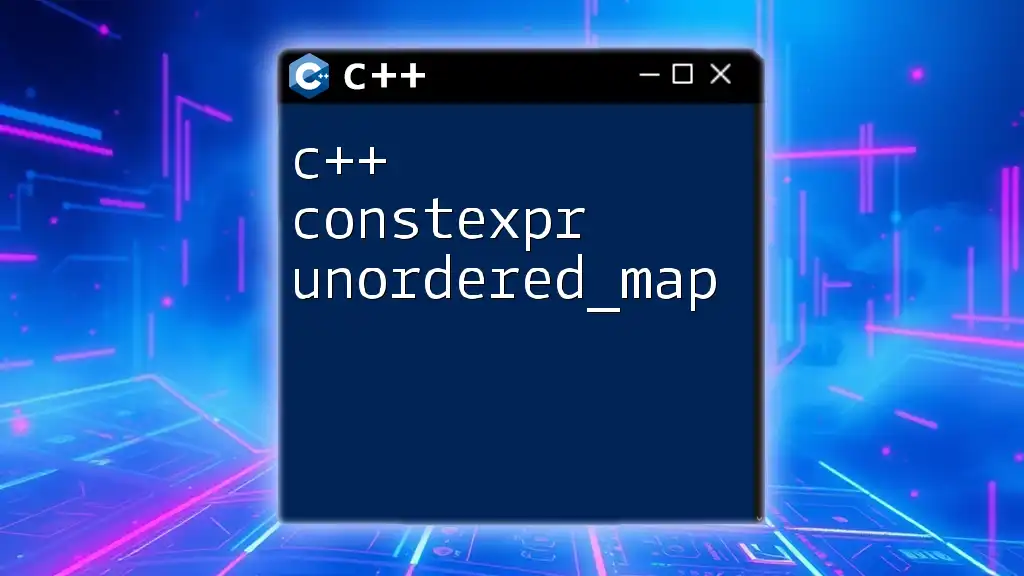
Implementing a `constexpr` Map
Syntax for Creating a `constexpr` Map
The syntax to create `constexpr` variables is straightforward, relying on standard C++ structuring rules. Implementing a `constexpr` map boils down to creating a custom implementation that allows for compile-time evaluation while retaining the map's functionality.
Example: Creating a Simple `constexpr` Map
Here’s an example of how one might define a basic `constexpr` map:
#include <array>
#include <utility>
template <typename K, typename V, std::size_t N>
struct constexpr_map {
std::array<std::pair<K, V>, N> data;
constexpr V get(const K& key) const {
for (const auto& pair : data) {
if (pair.first == key) {
return pair.second;
}
}
throw std::out_of_range("Key not found");
}
};
In this code snippet, the `constexpr_map` struct is defined to host a fixed-size array of key-value pairs. The method `get()` retrieves values associated with specific keys.
Populating the Map
To populate the `constexpr` map effectively, you can initialize it directly with values that are known at compile time:
constexpr constexpr_map<int, const char*, 3> color_map = {{
{1, "Red"},
{2, "Green"},
{3, "Blue"}
}};
Here, `color_map` is initialized with three color mappings, making it straightforward to retrieve values later on.
Accessing Data from a `constexpr` Map
Once the `constexpr` map is set up and populated, you can access its values like so:
constexpr auto color = color_map.get(2); // Will evaluate to "Green"
This line retrieves the value associated with the key `2`, resulting in "Green." Because this retrieval happens at compile time, it remains highly efficient.
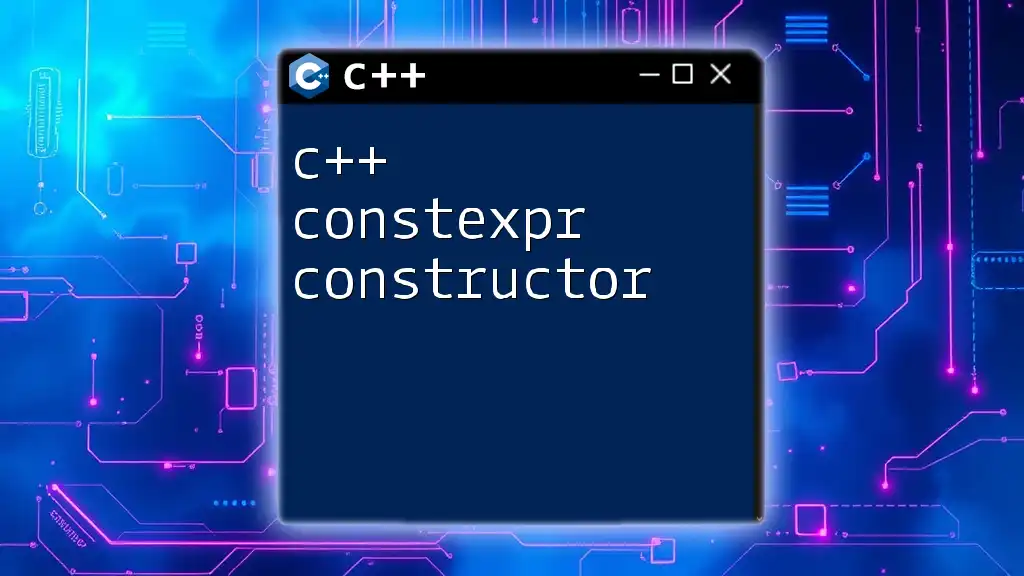
When and Why to Use `constexpr` Maps
Use Cases
Utilizing `constexpr` maps is particularly advantageous in scenarios where the data set is constant and known ahead of time. They can be ideal for:
- Game Development: For storing level configurations or entity states that remain unchanged throughout the game.
- Configuration Storage: When settings or parameters used by an application need to be efficiently accessed without any dynamic overhead.
Limitations of `constexpr` Maps
However, there are constraints to keep in mind. `constexpr` maps cannot be modified after their definition. Trying to change the values or structure dynamically at runtime breaks the concept of compile-time constant expressions. Therefore, if your application calls for frequent modifications or dynamic behaviors, `std::map` or other dynamic data structures would be more appropriate.
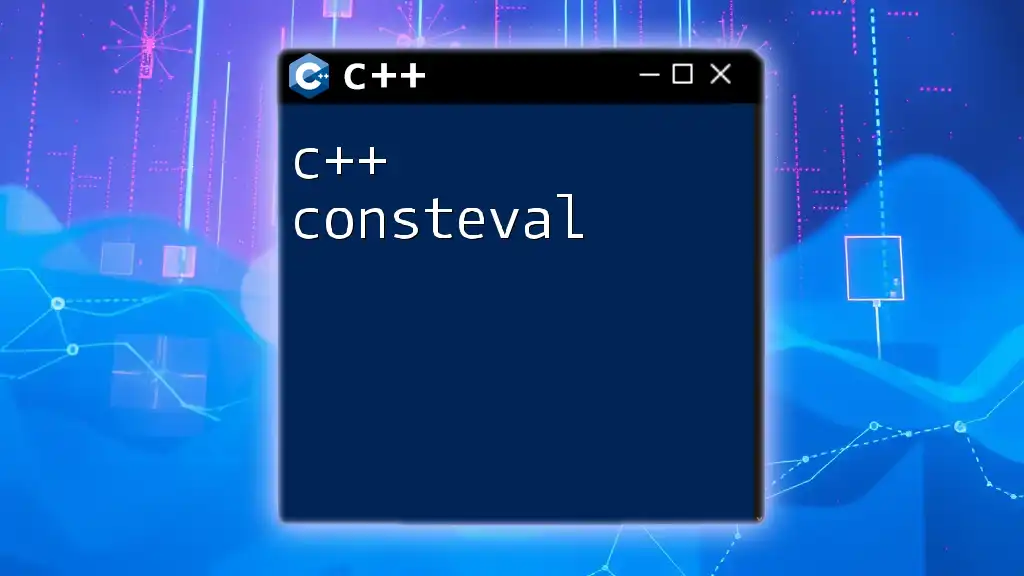
Advanced Features of `constexpr` Maps
Template Specialization
For more complex data structures or when working with various data types, template specialization can provide a flexible solution. Specializing templates allows developers to customize functionality based on the types of keys and values, ensuring scalability.
Recursive Maps
While implementing recursive structures in `constexpr` maps may introduce additional complexity, this feature serves useful in specialized areas. For instance, leveraging recursive definitions can facilitate the creation of trees or nested maps that can still be evaluated at compile time.
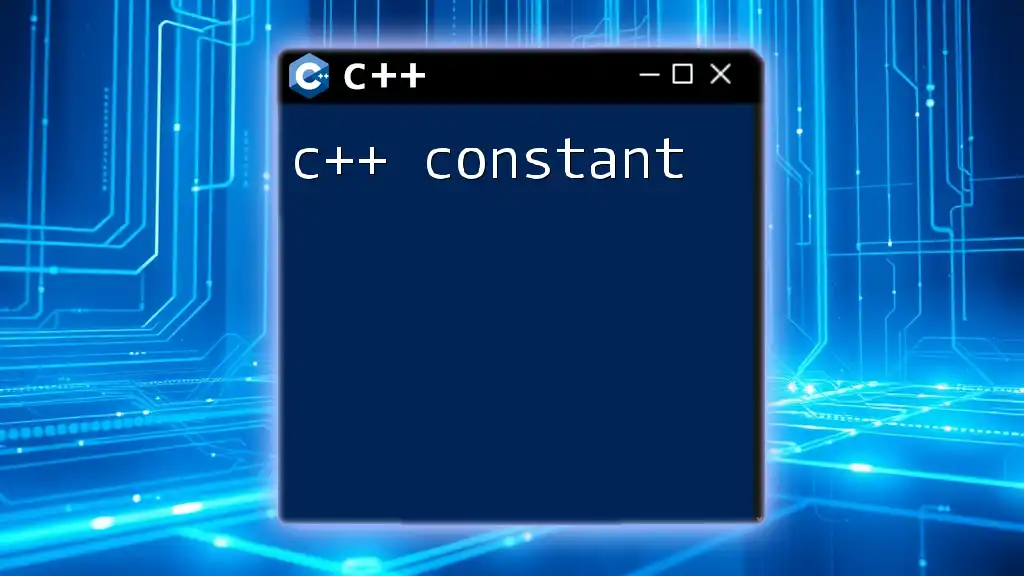
Conclusion
In summary, C++ `constexpr` maps represent a powerful tool in high-performance coding. The ability to create compile-time data structures provides significant advantages in terms of performance, safety, and code clarity. By understanding the workings of `constexpr`, developers can refine their applications to achieve optimal efficiency and reliability. Explore the possibilities with `constexpr` maps in your own projects, and witness the difference it can make in your C++ coding practices.
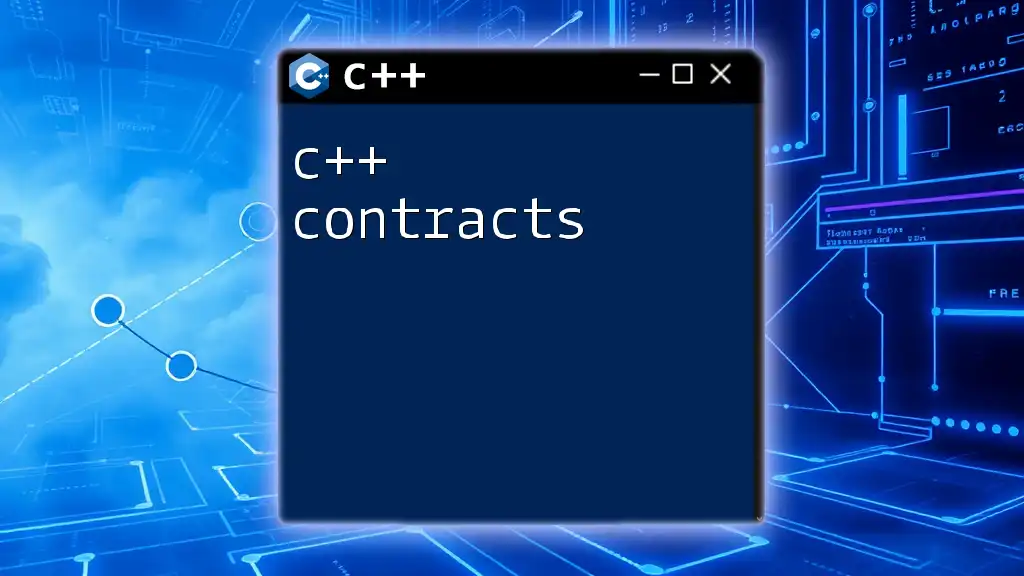
Additional Resources
For further reading and exploration, consider looking into advanced C++ topics related to `constexpr`, exploring more on C++ maps, and enhancing your understanding of modern C++ practices.