In C++, a `constexpr unordered_map` allows for compile-time evaluation of key-value pairs, enabling efficient constant expression usage within your code.
#include <iostream>
#include <unordered_map>
constexpr std::unordered_map<int, const char*> create_map() {
return { {1, "one"}, {2, "two"}, {3, "three"} };
}
int main() {
constexpr auto my_map = create_map();
std::cout << my_map.at(2) << std::endl; // Output: two
return 0;
}
Understanding `unordered_map`
`unordered_map` is a key-value data structure that provides average constant time complexity for lookups, insertions, and deletions. This container is part of C++’s Standard Library and is often chosen for its efficient handling of non-ordered elements. Unlike `std::map`, which keeps its elements sorted, `unordered_map` organizes elements based on a hash function, making it particularly efficient for scenarios where fast access is paramount.
Key characteristics of `unordered_map` include:
- Hash Table Implementation: Under the hood, `unordered_map` uses a hash table. Keys are processed through a hash function, allowing for comparatively quicker access times.
- No Guarantees on Order: Elements within an `unordered_map` are not stored in any specific order. If order is essential, other containers such as `std::map` should be considered.
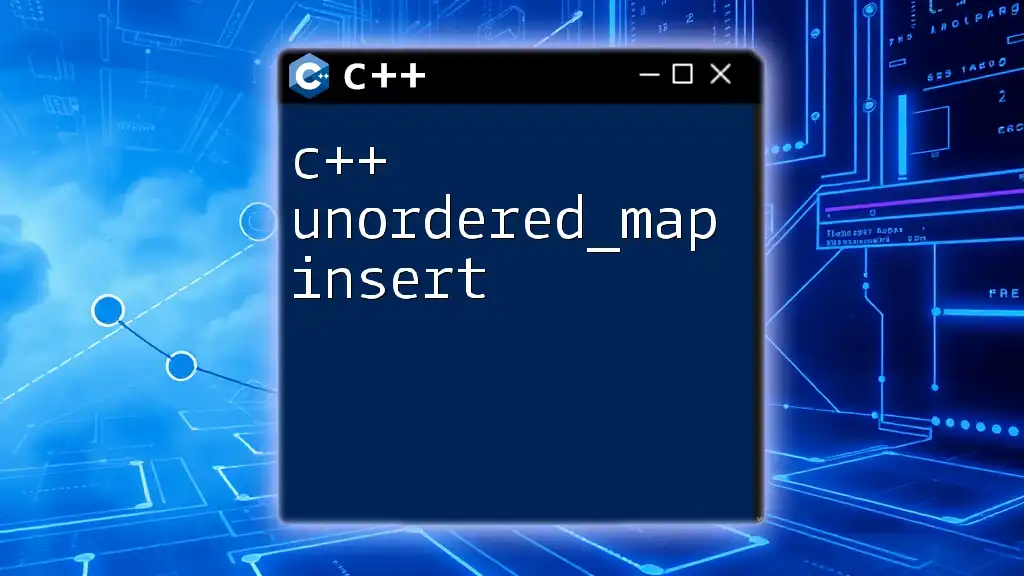
The Need for `constexpr unordered_map`
Although `unordered_map` is efficient for runtime usage, it comes with limitations when it comes to compile-time evaluations. C++20 introduced the concept of `constexpr unordered_map`, allowing `unordered_map` to be initialized and used during compile-time.
The advantages of using `constexpr` in conjunction with `unordered_map` include:
- Immutability: `constexpr` variables are immutable, which makes them ideal for configurations that never change.
- Performance Improvement: Since the data structure is evaluated at compile-time, it allows for faster access during runtime, eliminating the overhead of dynamic allocation.
Real-world use cases for `constexpr unordered_map` can include scenarios like configuration settings or lookup tables that are read-only and known at compile-time.
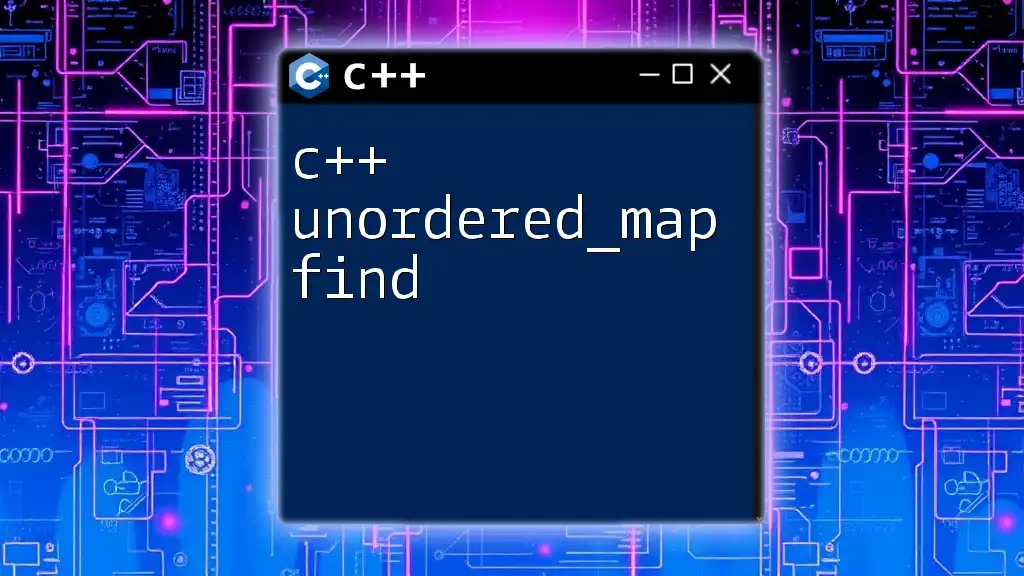
Implementation of `constexpr unordered_map`
Basics of Creating a `constexpr unordered_map`
Creating a `constexpr unordered_map` requires an understanding of how to combine `std::array` for compile-time storage with the properties of `unordered_map`. While `unordered_map` cannot be fully constexpr in prior versions of C++, C++20 expands its capabilities.
Here’s a basic example that highlights how to use `unordered_map`:
#include <array>
#include <string>
#include <unordered_map>
constexpr auto createMap() {
return std::unordered_map<std::string, int>{{"a", 1}, {"b", 2}};
}
Designing the `constexpr unordered_map`
To create a working `constexpr unordered_map`, you need a custom hash function that the compiler can process at compile-time. Below is an example of a custom hash function that can be used:
struct CompileTimeHash {
static constexpr std::size_t hash(const char* str, std::size_t h = 0) {
return (str[h] == 0) ? h : hash(str, h + 1) ^ (h + 1) ^ (str[h]);
}
};
Explanation: This recursive function computes a hash value for a given string and allows for constant-time evaluation during compile time.
Using `constexpr` Storage for Data
To store key-value pairs in a `constexpr` way, you can leverage `std::array` and `std::pair`. Here’s how you can define these:
constexpr std::array<std::pair<const char*, int>, 2> data = {{{"key1", 1}, {"key2", 2}}};
With this setup, you are preparing to create a `unordered_map` from a compile-time array of pairs.
Example of a Complete `constexpr unordered_map` Implementation
Now let’s see a complete working example of a `constexpr unordered_map`. The snippet below demonstrates how to do this while employing `constexpr` for creating a map that can be accessed at compile-time.
#include <iostream>
#include <array>
#include <utility>
constexpr auto make_map() {
constexpr std::array<std::pair<const char*, int>, 3> data = {{{"apple", 1}, {"banana", 2}, {"cherry", 3}}};
return std::unordered_map(data.begin(), data.end());
}
constexpr auto my_map = make_map();
static_assert(my_map.at("apple") == 1);
In this example, `constexpr auto my_map` initializes a map containing fruit names and their corresponding IDs. The `static_assert` statement helps verify that the `unordered_map` functionality is working correctly at compile time.
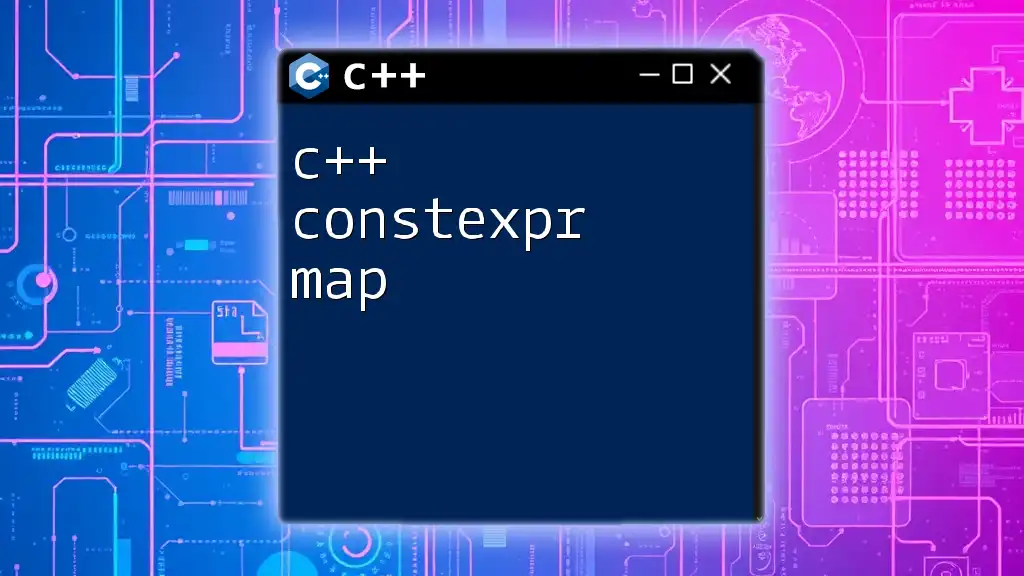
Limitations and Considerations
Despite its advantages, `constexpr unordered_map` comes with certain limitations. For instance:
- Compiler Support: Not all compilers have full support for `constexpr unordered_map`, which may restrict portability across different systems.
- Value Types: The types stored in a `constexpr unordered_map` must also be `constexpr`, which can limit the flexibility of the types you can use.
When employing `constexpr unordered_map`, consider whether its compile-time evaluation contributes noticeably to your program's performance and structure.
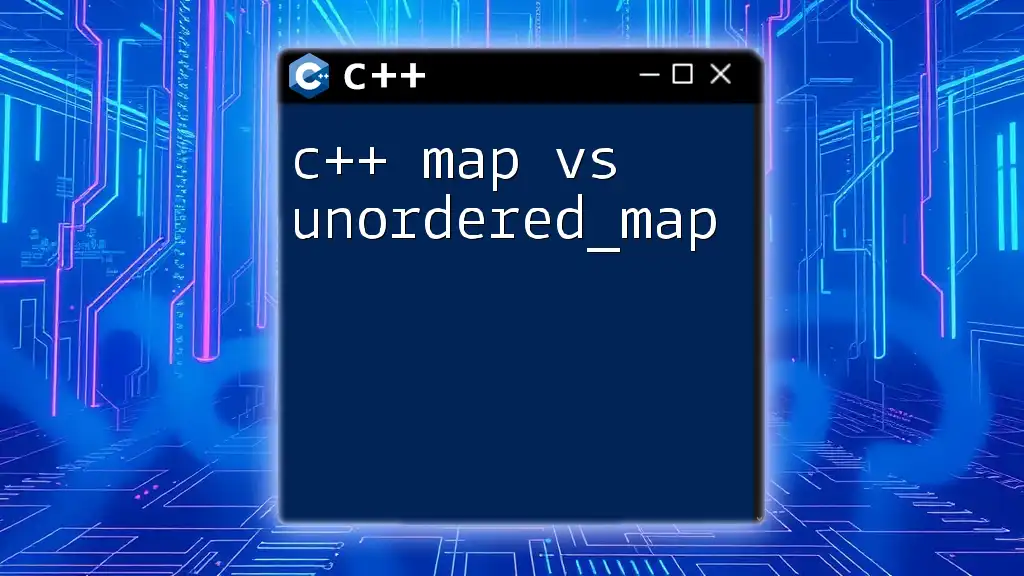
Practical Applications of `constexpr unordered_map`
`constexpr unordered_map` finds its strengths in scenarios where high performance and immutability are essential. Examples include:
- Compile-time Configuration: Store application configurations that don’t change after compilation.
- Lookup Tables: Construct lookup tables for mathematical computations, static routing tables, or game configuration.
Here’s a snippet demonstrating such a practical use-case:
constexpr auto config = createConfigMap(); // Example use case
Note: Ensure the correctness of the mappings since compile-time values cannot be changed at runtime.
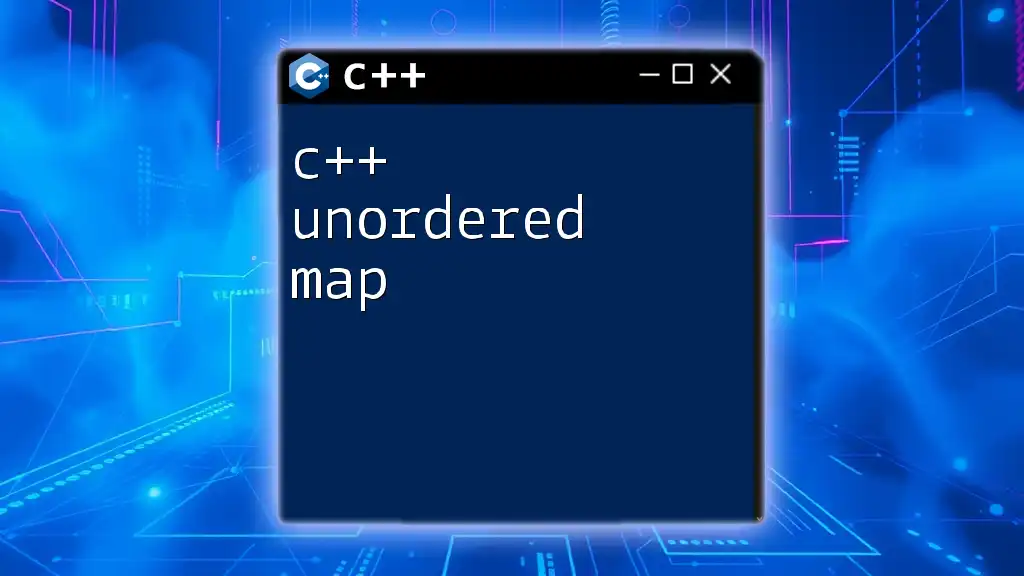
Future of `constexpr` and Unordered Containers
As C++ continues to evolve, the future possibilities for `constexpr` capabilities are promising. Anticipation exists around enhancements in potential C++ standards that may allow for further optimizations and container functionalities that embrace compile-time evaluations. This could reshape how developers approach data structures and impose additional paradigms that improve performance, especially for real-time and performance-critical applications.
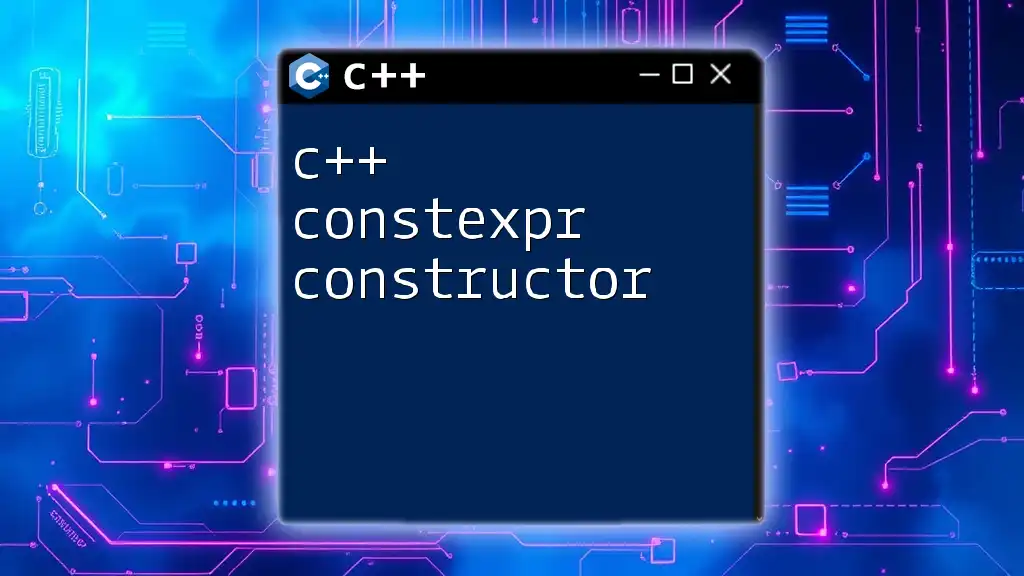
Conclusion
The introduction of `constexpr unordered_map` expands the C++ landscape by offering a powerful tool for developers focused on compile-time computations. By understanding its implementation, advantages, and limitations, programmers can create highly efficient and optimized applications. Embrace the power of `constexpr` in your C++ projects to discover new efficiencies and methodologies in programming. Experiment today and explore the remarkable possibilities C++ has to offer!
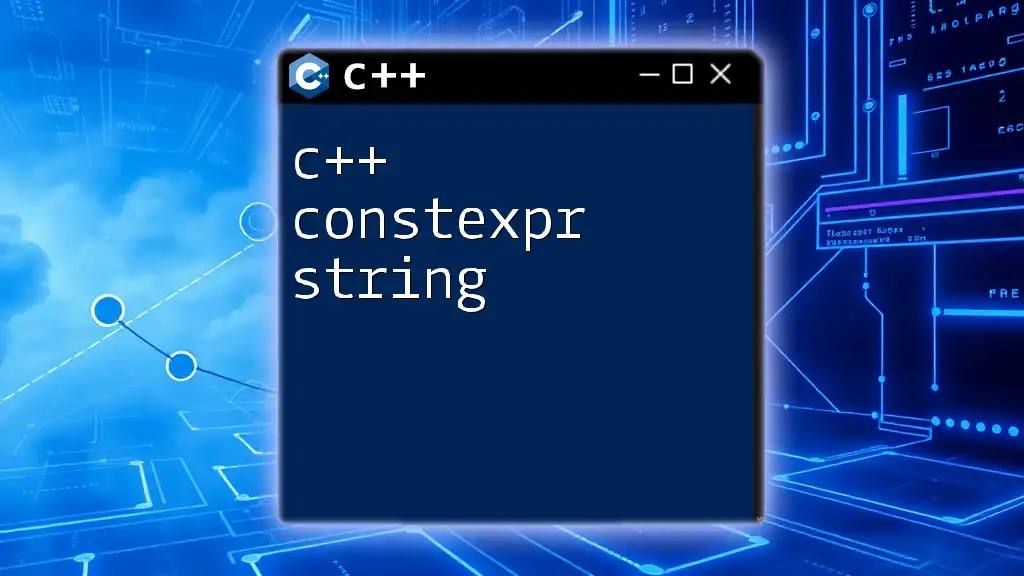
Frequently Asked Questions (FAQs)
Is `constexpr unordered_map` supported in all C++ compilers?
Support for `constexpr unordered_map` varies by compiler and their respective versions. Ensure that you are using a compliant C++20 compiler for the best experience.
What are the alternatives If `constexpr unordered_map` is not available?
If `constexpr unordered_map` isn’t available, consider using `std::array` or `std::tuple` as alternatives to store compile-time mapped data, though lookup efficiency may be affected.
How does `constexpr unordered_map` affect program performance?
By allowing for compile-time initialization, `constexpr unordered_map` can significantly reduce runtime overhead, providing almost instant access and lowering dynamic memory footprint.
Can I use `constexpr unordered_map` with non-POD types?
`constexpr unordered_map` can work with non-POD (Plain Old Data) types, provided they are also constexpr compliant. Ensure to validate these types within the context of compile-time evaluation when designing your map.