In C++, there are three primary types of loops—`for`, `while`, and `do-while`—each allowing for repeated execution of a block of code based on specific conditions.
Here’s a brief example of each loop type:
// For Loop Example
for (int i = 0; i < 5; i++) {
cout << "For Loop iteration: " << i << endl;
}
// While Loop Example
int j = 0;
while (j < 5) {
cout << "While Loop iteration: " << j << endl;
j++;
}
// Do-While Loop Example
int k = 0;
do {
cout << "Do-While Loop iteration: " << k << endl;
k++;
} while (k < 5);
Understanding the Core Loop Concepts
What is a Loop?
A loop in programming is a construct that allows for the execution of a block of code multiple times based on a specific condition. Loops enable programmers to run the same code repeatedly without having to write it multiple times, enhancing both code efficiency and readability. This is especially useful in scenarios where the number of iterations is unknown ahead of time.
Common Loop Terminology
It’s essential to understand some key terms associated with loops:
- Iteration: Each execution of the loop's body is called an iteration.
- Control Statements: Direct the flow of the loop, determining when to enter or exit the loop.
- Loop Body: Consists of the code that is executed during each iteration.
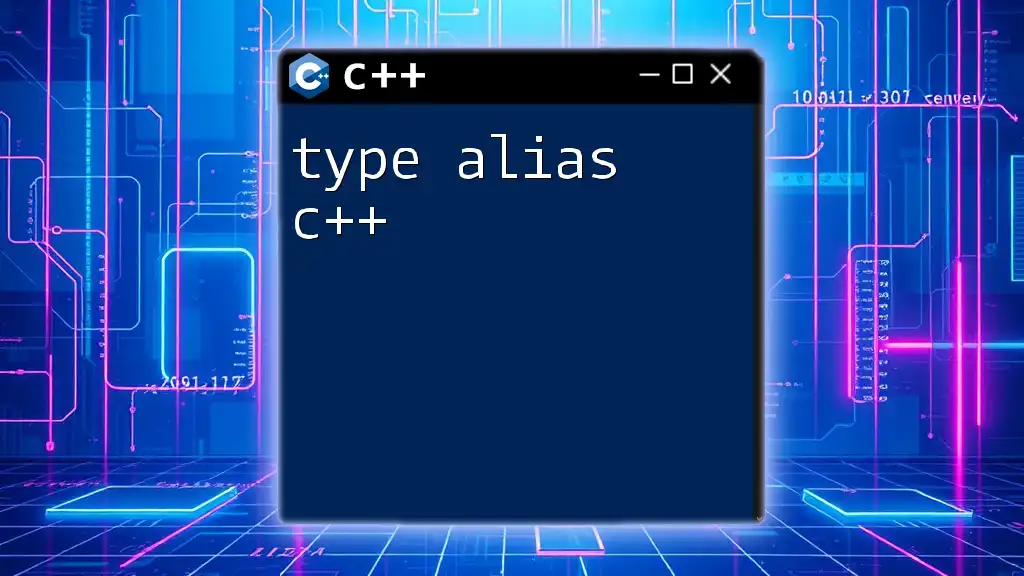
Types of Loops in C++
The `for` Loop
Syntax of a `for` Loop
The `for` loop is typically used when the number of iterations is known beforehand. Its syntax is as follows:
for (initialization; condition; increment/decrement) {
// loop body
}
Example of a `for` Loop
Here's a basic example of a `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration " << i << std::endl;
}
In this example, the loop will iterate five times, printing the iteration number each time.
When to Use a `for` Loop
You should use a `for` loop when:
- The number of iterations is known before entering the loop.
- You need to iterate through arrays or collections with a specific index. This loop is optimal for counting purposes and fixed iterations.
The `while` Loop
Syntax of a `while` Loop
The `while` loop is utilized when the number of iterations is not known in advance. Its syntax is shown below:
while (condition) {
// loop body
}
Example of a `while` Loop
Consider the following example:
int i = 0;
while (i < 5) {
std::cout << "Iteration " << i << std::endl;
i++;
}
This code snippet will also iterate five times, similar to the `for` loop. However, it checks the condition before each iteration.
When to Use a `while` Loop
Utilize a `while` loop when:
- The number of iterations depends on a condition being true.
- You need to wait for a certain event or input from the user. This loop is commonly used in situations where the exit condition is evaluated inside the loop body.
The `do...while` Loop
Syntax of a `do...while` Loop
The `do...while` loop guarantees that the loop body executes at least once, as the condition is checked after the loop body. Its syntax is:
do {
// loop body
} while (condition);
Example of a `do...while` Loop
Here’s an illustrative example:
int i = 0;
do {
std::cout << "Iteration " << i << std::endl;
i++;
} while (i < 5);
This code will work similarly to the previous loops, printing out the iteration number, but it ensures that the loop body executes before the condition is checked.
When to Use a `do...while` Loop
A `do...while` loop is appropriate when:
- You need the loop body to execute at least once regardless of the condition.
- The logic requires the code to run before checking the loop's exit condition.
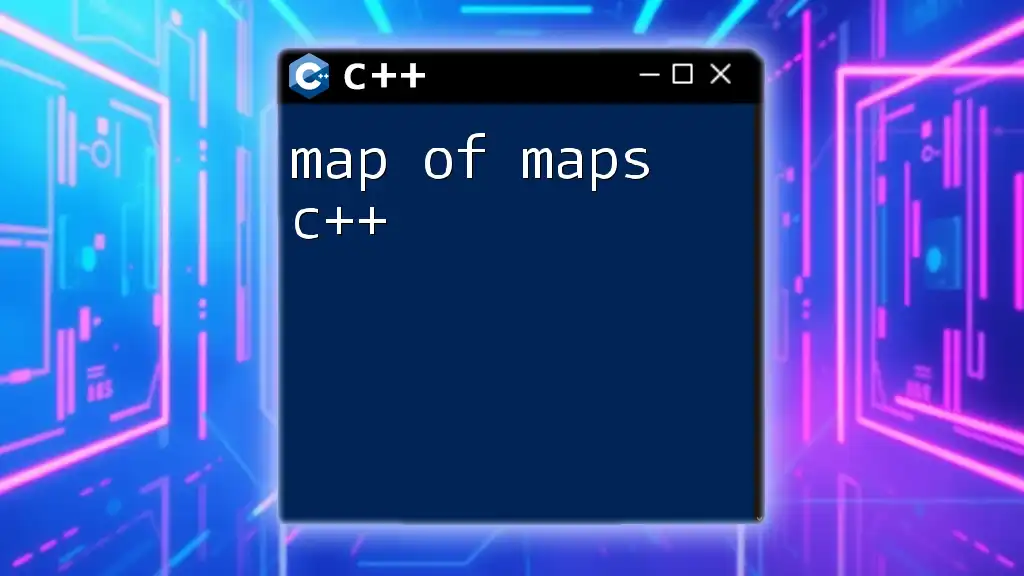
Comparing the Types of Loops
Performance Considerations
The choice of loop can have performance implications. Though in most cases, modern compilers optimize them to perform similarly, it’s still important to consider:
- Efficiency: If you know the number of iterations, use a `for` loop. It can lead to cleaner and potentially faster code since the loop control is typically straightforward.
- Memory Usage: Nested loops may lead to increased memory usage, especially with large datasets or when using complex structures.
Readability and Maintainability
Code readability is crucial for long-term maintenance. For loops are often more concise and easier for other developers to understand at a glance, while while and do...while loops may require more scrutiny to ensure they are functioning correctly.
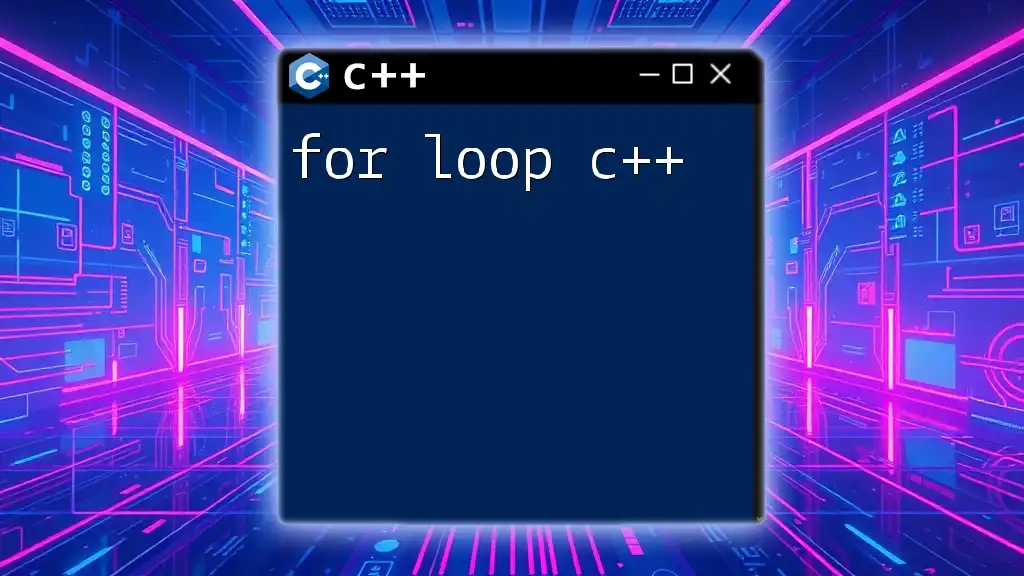
Nested Loops in C++
Understanding Nested Loops
Nested loops are simply loops within loops, allowing for more complex iteration patterns, particularly useful in scenarios like multi-dimensional data processing.
Example of Nested Loops
Consider this example to illustrate nested loops:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
std::cout << "i: " << i << ", j: " << j << std::endl;
}
}
This code will create a grid-like output, iterating through both `i` and `j` values.
Best Practices for Nested Loops
While handy, nested loops can lead to performance issues if not managed properly:
- Limit the complexity to maintain performance and clarity.
- Avoid unnecessary nesting where possible; seek alternatives such as combining logic or using function calls.
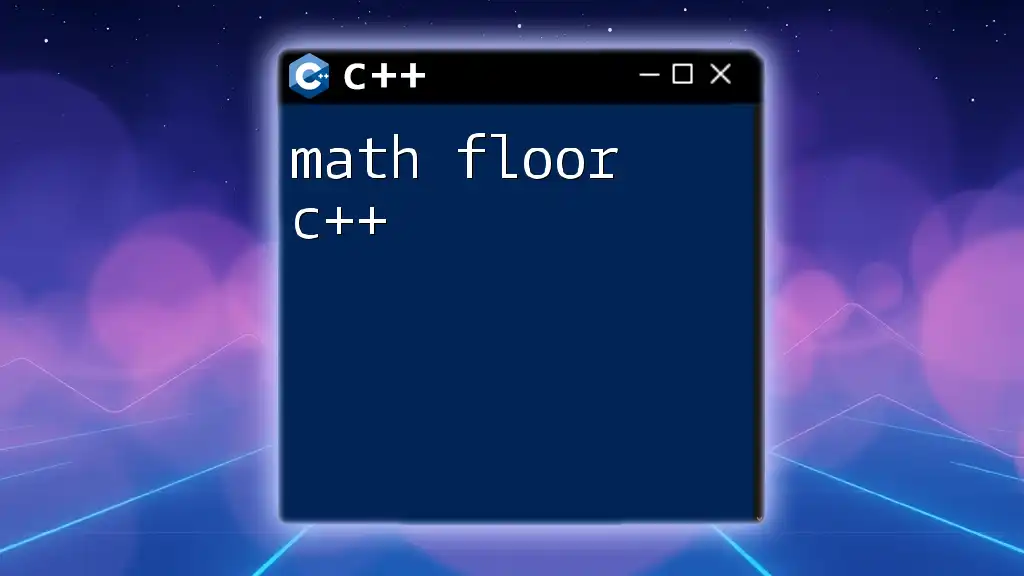
Conclusion
Understanding the types of loops in C++ is crucial for writing efficient and effective code. Each loop type serves specific scenarios best, from fixed iterations with the `for` loop to conditional execution with `while` and guaranteed execution through `do...while`. Emphasizing practice through examples ensures that you become proficient in implementing loops in your programming endeavors.
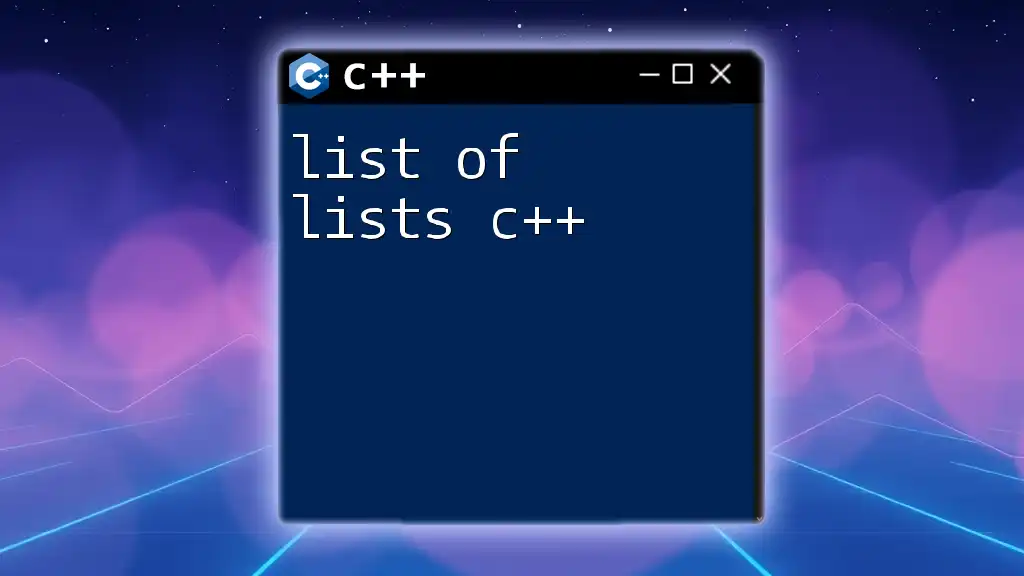
Additional Resources
Recommended Reading
Explore more about C++ loops in books, online courses, and detailed documentation available from trusted programming resources.
Practice Exercises
To reinforce your understanding, try writing loops to solve various problems, such as calculating factorials, handling user input repeatedly, or creating multiplication tables.
Community Engagement
Please share your questions or experiences with using loops in C++. Engage with a community of learners and experts to enhance your C++ programming skills!