In C++, a list of lists can be implemented using nested `std::list` containers, allowing you to create a flexible data structure that accommodates multiple lists within a single list.
Here’s a code snippet demonstrating a list of lists in C++:
#include <iostream>
#include <list>
int main() {
std::list<std::list<int>> listOfLists;
// Adding a list of integers to the list of lists
std::list<int> innerList1 = {1, 2, 3};
std::list<int> innerList2 = {4, 5, 6};
listOfLists.push_back(innerList1);
listOfLists.push_back(innerList2);
// Printing the list of lists
for (const auto& innerList : listOfLists) {
for (int num : innerList) {
std::cout << num << " ";
}
std::cout << std::endl;
}
return 0;
}
Understanding Lists in C++
What is a List?
In C++, a list is an essential data structure that allows for a collection of elements held in a sequential manner. Unlike arrays, lists provide dynamic sizing, allowing elements to be added or removed efficiently. This flexibility is particularly beneficial for applications where the size of the collection can change over time.
Types of Lists in C++
Singly Linked Lists
A singly linked list is a collection of nodes where each node contains data and a pointer to the next node. This structure allows for efficient insertion and deletion since the nodes are not stored in contiguous memory locations.
Advantages:
- Dynamic sizing: Elements can be added or removed without reallocating the entire structure.
- Efficient inserts/deletes: Adding or removing elements takes O(1) time if the position is known.
Disadvantages:
- Linear access: Accessing elements requires traversing from the head, leading to O(n) time complexity.
Doubly Linked Lists
A doubly linked list expands on the singly linked list structure by including pointers to both the next and previous nodes. This feature permits backward traversal of the list, enhancing flexibility.
Use Cases:
- Implementing complex data structures like deques or certain algorithms where double direction traversal is needed.
Why Use Lists of Lists?
A list of lists is essentially a data structure where each element is itself a list. This is particularly useful for representing multidimensional data or hierarchical relationships.
Real-world Applications:
- Representing adjacency lists for graph data structures.
- Managing tables of data where each row may have a variable number of columns.
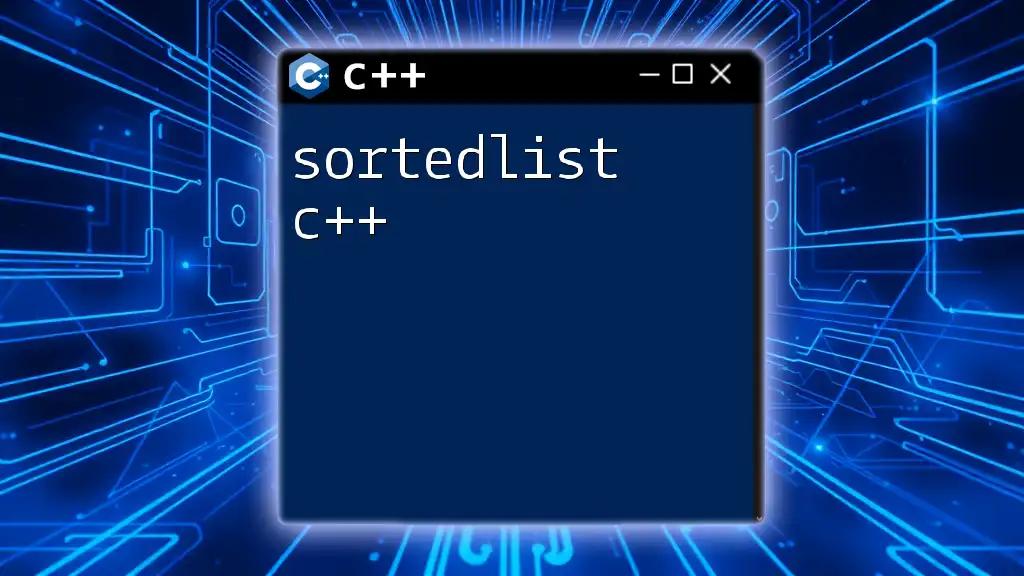
Implementing List of Lists in C++
Using Standard Template Library (STL)
Overview of STL
The Standard Template Library (STL) in C++ provides a rich set of methods for managing collections of objects, making the implementation of a list of lists straightforward and efficient.
Creating a List of Lists with STL
With the `std::list` class in the STL, creating a list of lists becomes seamless.
#include <iostream>
#include <list>
int main() {
std::list<std::list<int>> list_of_lists;
std::list<int> first_list = {1, 2, 3};
std::list<int> second_list = {4, 5, 6};
list_of_lists.push_back(first_list);
list_of_lists.push_back(second_list);
for (const auto& inner_list : list_of_lists) {
for (const auto& value : inner_list) {
std::cout << value << " ";
}
std::cout << std::endl;
}
return 0;
}
The code above demonstrates how to create a list of lists wherein two inner lists are created and added to the main list. The nested loops iterate through each inner list, printing its values, which exemplifies how easily nested structures can be managed using STL.
Custom Implementation of List of Lists
Creating a Node Structure
When opting for a custom implementation, understanding the underlying structure is crucial. A fundamental node structure would consist of:
struct Node {
int data;
Node* next;
Node* down; // For creating list of lists
};
This structure allows each node to point not only to the next node in the list but also to another list, paving the way for a list of lists.
Building a Custom Linked List of Lists
To construct a custom linked list of lists, begin by defining a class to manage the nodes and the collections effectively.
class ListOfLists {
Node* head;
public:
ListOfLists() : head(nullptr) {}
void addList(Node* newListHead) {
// Implementation for adding new lists
}
// Additional methods for manipulation
};
In this class, `head` signifies the starting node of the list. By adding methods to manipulate this structure, such as inserting and deleting lists, one can create a flexible system for managing data.

Operations on List of Lists
Adding Elements
Adding elements to a list of lists can be achieved by appending new inner lists to the main list. The insertion operation typically runs in O(1) time because you simply adjust pointers rather than shift elements as would be necessary in an array.
Traversing Lists
Efficient traversal of a list of lists is critical for understanding the contained data. Below is illustrated a method for traversing and printing the values:
void traverse(Node* head) {
while (head) {
std::cout << head->data << " ";
head = head->down; // Traversing the downward link
}
}
This function allows for straightforward iteration, enabling you to display all elements stored within the nested lists.
Deleting Elements
When it comes to deleting elements from a list of lists, careful handling of pointers is vital to avoid memory leaks or segmentation faults. Always ensure that the nodes being removed are safely detached from their neighbors.

Common Use Cases
Multi-dimensional Data Handling
A list of lists is particularly useful in managing multi-dimensional datasets, which can represent matrices or grids where each row may not have uniform columns.
Graph Representation
In graph algorithms, adjacency lists are a perfect candidate for a list of lists structure. Each node in a graph can be represented as a list that contains all nodes adjacent to it, providing a dynamic and efficient representation of the graph.

Performance Considerations
Time Complexity
The average time complexity of common operations—such as insertion and deletion—is O(1). However, accessing elements requires O(n) time for a singly linked list since traversal is required.
Memory Usage
While lists of lists are flexible and allow dynamic sizing, they may incur extra memory overhead due to the need for pointer storage. Efficient memory management practices are advisable to mitigate potential inefficiencies.
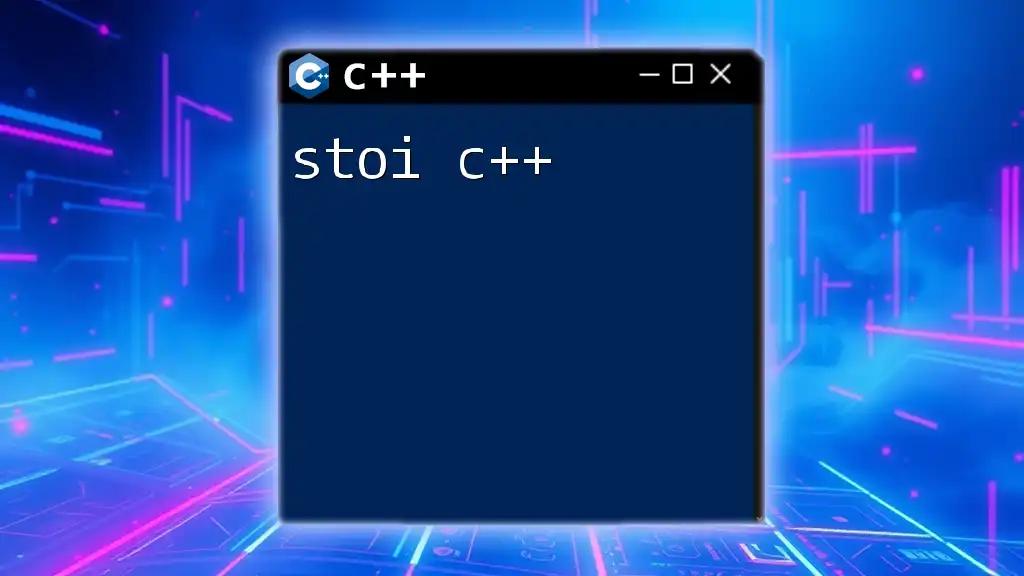
Conclusion
In this article on list of lists c++, we explored the depth of lists and their implementation through both the STL and custom methodologies. Understanding how to leverage such data structures can significantly enhance your programming capabilities and facilitate the effective handling of complex data scenarios. By practicing these concepts, you can develop sophisticated solutions in C++. Be sure to continue exploring resources to further enhance your knowledge and skills in utilizing lists of lists in C++.

FAQs
As you delve into the world of advanced data structures, you may come across common questions regarding lists of lists in C++. Don’t hesitate to research and experiment further to truly master these concepts!