In C++, logging can be achieved using the `iostream` library to output messages to the console, facilitating debugging and tracking the flow of the application.
#include <iostream>
int main() {
std::cout << "Log: Application has started successfully." << std::endl;
return 0;
}
Understanding the C++ Log Function
Basics of C++ Log Function
The C++ programming language provides a built-in function for calculating logarithms through the `<cmath>` library. The primary function utilized for this purpose is `log()` which computes the natural logarithm (logarithm with base 'e') of a given number. The syntax for the function is as follows:
double log(double x);
The function takes a single argument, `x`, which must be a positive number. If `x` is zero or negative, the function will result in a domain error, typically returning `NaN` (Not a Number).
How to Use the Log Function
Using the `log()` function is straightforward. Below is a simple example demonstrating its usage:
#include <iostream>
#include <cmath>
int main() {
double value = 10.0;
double result = log(value);
std::cout << "The logarithm of " << value << " is: " << result << std::endl;
return 0;
}
In this example, when you compile and run the code, it will output the natural logarithm of 10. It's important to remember that the result given will be in terms of natural logarithm unless otherwise specified.

Natural Logarithm in C++
Definition of Natural Logarithm
The natural logarithm, denoted as ln or `log()` in C++, is the logarithm to the base e, where e is approximately equal to 2.71828. The natural logarithm is important in various scientific and mathematical applications, including exponential growth and decay modeling.
Calculating the Natural Logarithm
You can calculate the natural logarithm using the same function, `log()`. Here's an illustration:
#include <iostream>
#include <cmath>
int main() {
double value = 2.71828; // Approximately e
std::cout << "Natural log of e: " << log(value) << std::endl;
return 0;
}
This code snippet calculates the natural logarithm of the constant e and will return a result very close to 1, as expected.

Logarithm Base Conversion
Understanding Logarithm Bases
In many applications, it may be necessary to convert logarithm bases depending on specific requirements such as mathematical computations or algorithm complexities. The conversion from base `b` to base `k` can be achieved using the formula:
\[ \text{log}_b(a) = \frac{\text{log}_k(a)}{\text{log}_k(b)} \]
Common bases include base 10 and base e.
C++ Implementation for Logarithm Conversion
In C++, you can implement a function to perform base conversion as follows:
#include <iostream>
#include <cmath>
double log_base(double a, double b) {
return log(a) / log(b);
}
int main() {
double x = 100.0; // Value to find log for
double base = 10.0; // Base for conversion
std::cout << "Log of " << x << " to the base " << base << " is: " << log_base(x, base) << std::endl;
return 0;
}
When you run this program, it calculates the logarithm of 100 to the base 10 using the conversion formula.
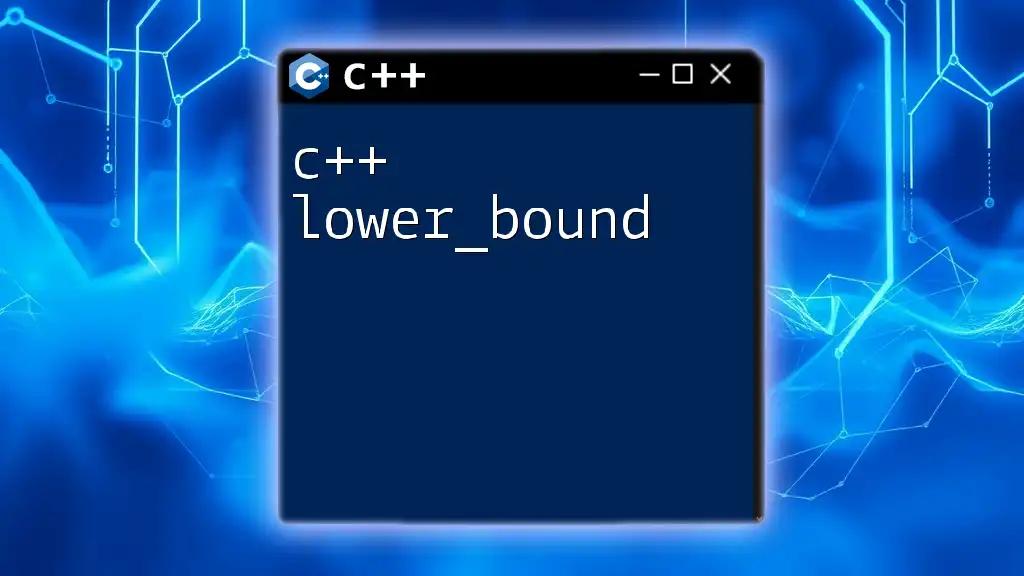
Applications of Logarithms in C++
Use Cases in Algorithms
Logarithms play a crucial role in computational complexity, particularly in algorithms such as binary search which operates in O(log n) time complexity. This efficiency is vital for scaling applications, as logarithmic growth allows for handling increasingly large datasets with minimal performance degradation.
Real-life Applications
Beyond algorithm efficiency, logarithms have numerous practical applications. For instance, in finance, you can use logarithmic functions to compute compound interest over time, where the formula relies heavily on the exponential growth model. Additionally, logarithms are used in various fields like physics, computer science, and information theory.
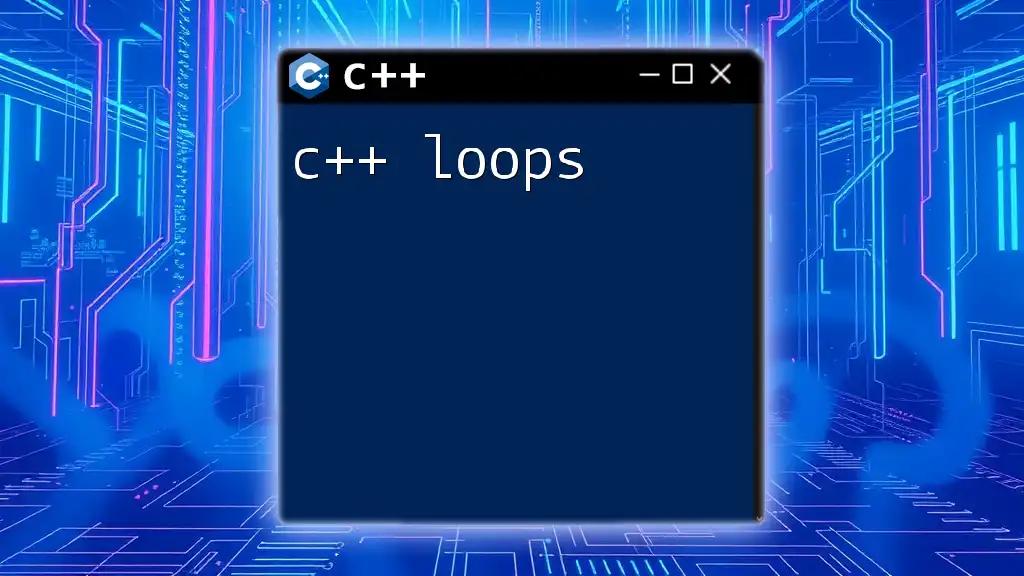
Common Mistakes When Using Logarithms in C++
Avoiding Negative and Zero Inputs
A frequent mistake that developers encounter is using negative values or zero as an input to the `log()` function. Since logarithms are undefined for these values, it's critical to always validate the input before passing it to the function. Incorporating checks can help prevent runtime errors.
Precision Issues with Floating Points
When working with floating-point numbers, precision issues may arise, especially with very small numbers close to zero. It's essential to consider that these calculations can lead to significant rounding errors. Implementing appropriate data types and rounding methods can help mitigate these issues.

Conclusion
In conclusion, the C++ log function offers a powerful tool for a variety of mathematical and computational applications. With a firm understanding of natural logarithms, logarithm conversion, and their applications, you can effectively implement logarithmic functions in your C++ code. Embrace the challenges of using logarithmic calculations and practice regularly to solidify your knowledge!

Additional Resources
For those looking to deepen their understanding, consider exploring further reading on C++ math functions, recommended coding platforms for tackling logarithmic-related challenges, and documentation from C++ standards to enhance your programming skills.