OS X, being a Unix-based operating system, supports C++ development using various tools and libraries, allowing developers to create powerful applications efficiently.
Here is a simple C++ program that prints "Hello, OS X!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, OS X!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful and versatile programming language widely used across various domains, including systems programming, game development, and application software. It is an extension of the C programming language and combines the procedural programming paradigm of C with object-oriented features. The language is known for its performance and efficiency, making it a popular choice for high-performance applications.
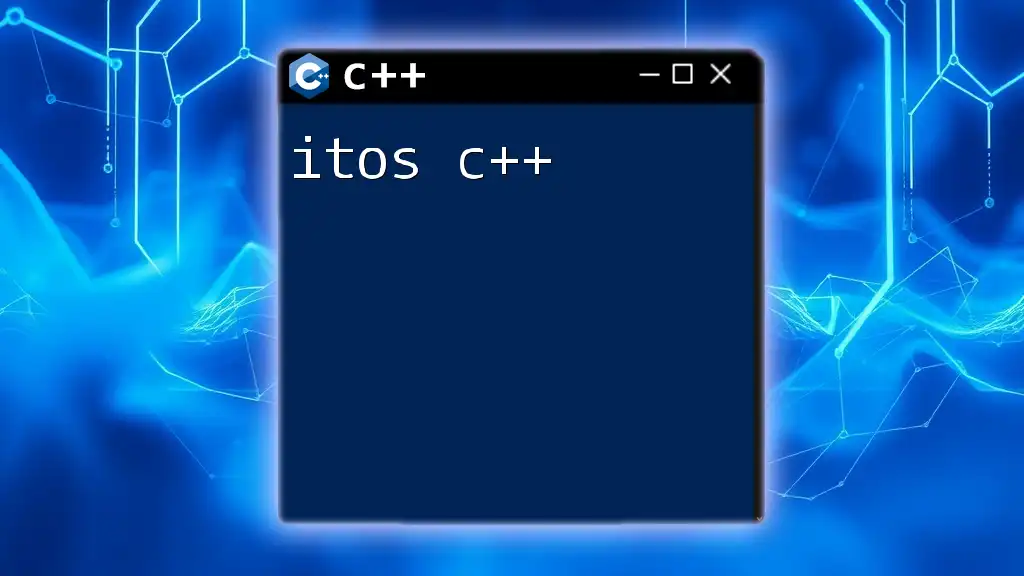
Why Choose macOS for C++ Development?
When it comes to choosing an operating system for C++ development, macOS stands out for several compelling reasons.
- Powerful Integrated Development Environment (IDE): macOS provides developers with Xcode, a robust IDE that includes a code editor, a visual debugger, and integrated build systems, ensuring that developers can work efficiently.
- Seamless Hardware and Software Integration: macOS is optimized for Mac hardware, enabling developers to leverage powerful capabilities for performance-intensive applications.
- Active Developer Community: A vibrant community of developers on macOS fosters collaboration, making it easy to find resources, forums, and tutorials tailored to C++ development.
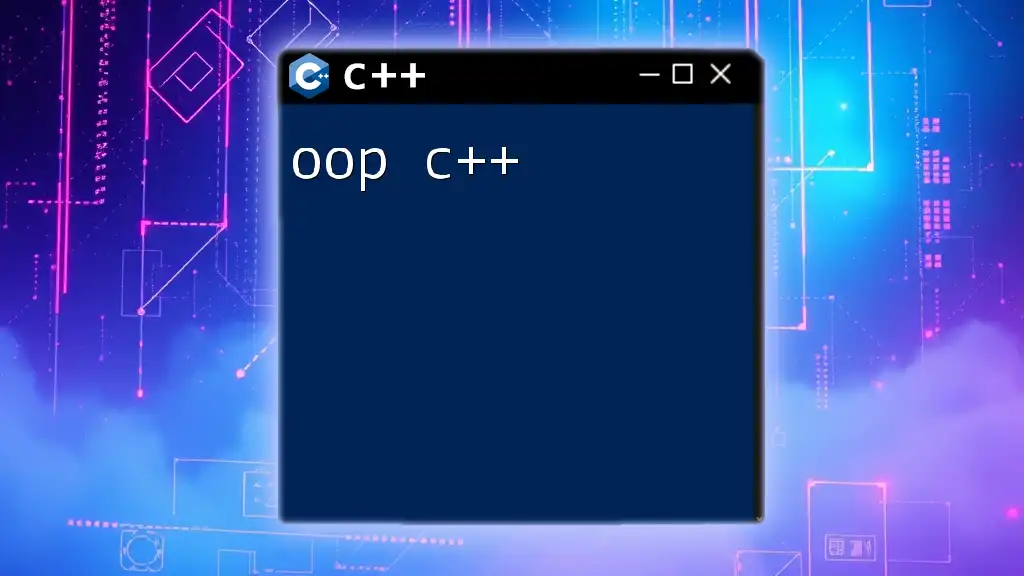
Setting Up Your macOS C++ Development Environment
Installing Xcode
Xcode is the primary IDE for macOS development, featuring support for C++, Swift, and Objective-C. To get started, follow these steps:
- Open the Mac App Store.
- Search for Xcode and click Install.
- After installation, it’s essential to install the Command Line Tools, which can be done through the terminal with the following command:
xcode-select --install
Running this command will initiate the installation of essential tools required for C++ compilation.
Configuring Your Development Environment
Besides Xcode, you might consider other IDEs. Some popular ones include:
- CLion, known for its smart code analysis.
- Visual Studio Code, which is lightweight and has customizable extensions.
To set up a new project in Xcode, follow these steps:
- Launch Xcode and select Create a new Xcode project.
- Choose macOS from the platform section and select Command Line Tool.
- Name your project and set the language to C++.
Once your project is created, Xcode’s interface will provide organized access to code files and compiler settings. Creating a "Hello, World!" program is a great first step:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
After writing the code, you can run it by clicking the play button in Xcode, and you should see the output in the console.
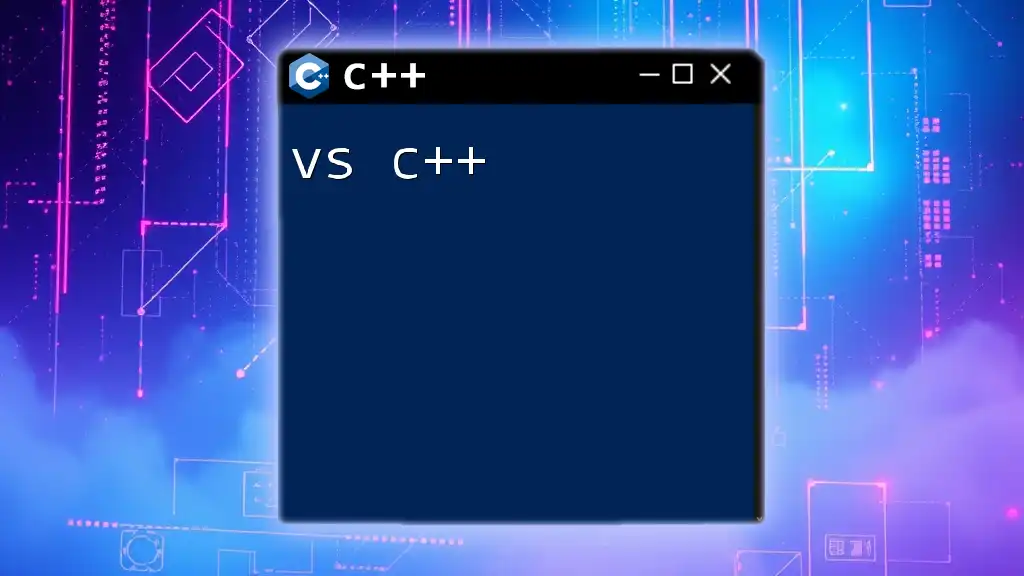
Understanding macOS Specific C++ Libraries
Standard C++ Library
The Standard C++ Library is essential for any C++ developer on macOS. It provides a collection of functions, algorithms, and data structures that facilitate programming tasks. Commonly used headers include:
- `<iostream>` for input and output streams.
- `<vector>`, `<map>`, and `<string>` for dynamic arrays and string manipulations.
macOS Frameworks and Libraries for C++
macOS offers unique frameworks that enhance C++ applications. Here are some notable ones:
- Cocoa: Essential for developing macOS applications with rich user interfaces. C++ can be mixed with Objective-C using Objective-C++ files (.mm).
To illustrate, here is how you might include Cocoa in a simple application:
#import <Cocoa/Cocoa.h>
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSApplication *app = [NSApplication sharedApplication];
NSApplicationMain(argc, argv);
}
return 0;
}
- CoreFoundation: Provides fundamental data types and utility functions.
- OpenGL: A powerful API for rendering 2D and 3D graphics.
Using CMake on macOS
CMake is a cross-platform build system that simplifies the process of compiling C++ applications. Here’s how to install it on macOS:
- You can install CMake using Homebrew with the following command:
brew install cmake
- After installation, create a simple CMake project with the following structure:
/MyProject
├── CMakeLists.txt
└── main.cpp
Your `CMakeLists.txt` could look like this:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
add_executable(MyProject main.cpp)
This configuration tells CMake to create an executable from `main.cpp`, using C++11 as the standard. To build the project, navigate to the project directory in the terminal and run:
mkdir build
cd build
cmake ..
make
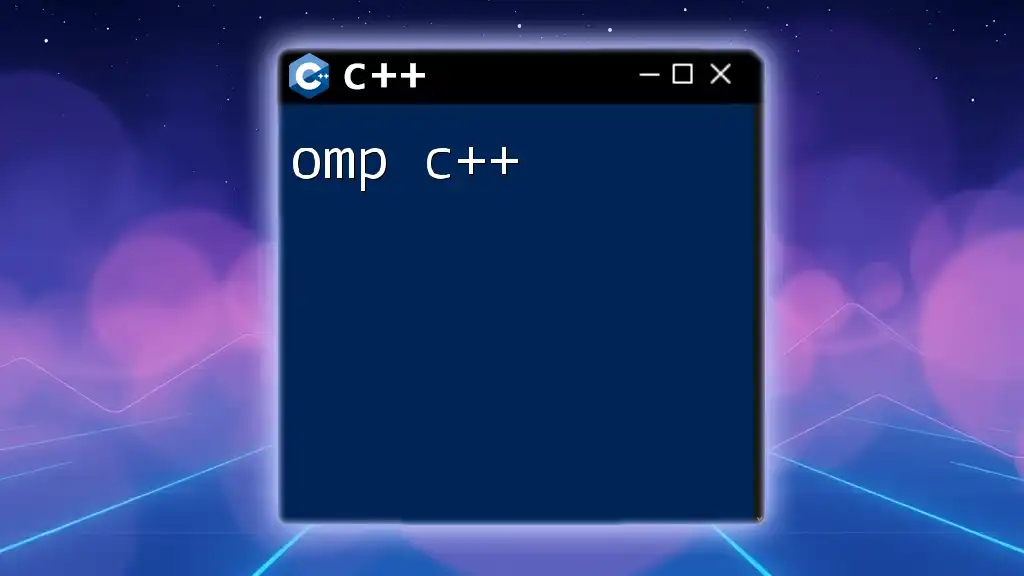
Compiling and Running C++ Code on macOS
Using Terminal for Compilation
The terminal is a powerful tool for compiling C++ code. To compile a simple C++ file, you can use the GNU C++ compiler, g++. Here’s how:
- Open the terminal.
- Navigate to the directory containing your C++ file.
- Use the following command to compile your code:
g++ -o my_program main.cpp
This command will create an executable named `my_program`. Run it using:
./my_program
Debugging C++ Code on macOS
Debugging is vital for any programming project. Xcode provides excellent tools for this purpose. To debug your application in Xcode:
- Set breakpoints by clicking in the gutter next to the line numbers.
- Run your project in Debug mode by clicking the play button (▶).
- When the execution hits a breakpoint, you can inspect variables and control program flow.
Here is a quick example of debugging:
If you have a code snippet like this:
#include <iostream>
int main() {
int a = 5;
int b = 0;
std::cout << "Result: " << a / b << std::endl; // Intentional division by zero
return 0;
}
You can set a breakpoint on the line with the `cout` statement. When you execute the program, it will pause there, allowing you to analyze variable values and step through the execution.
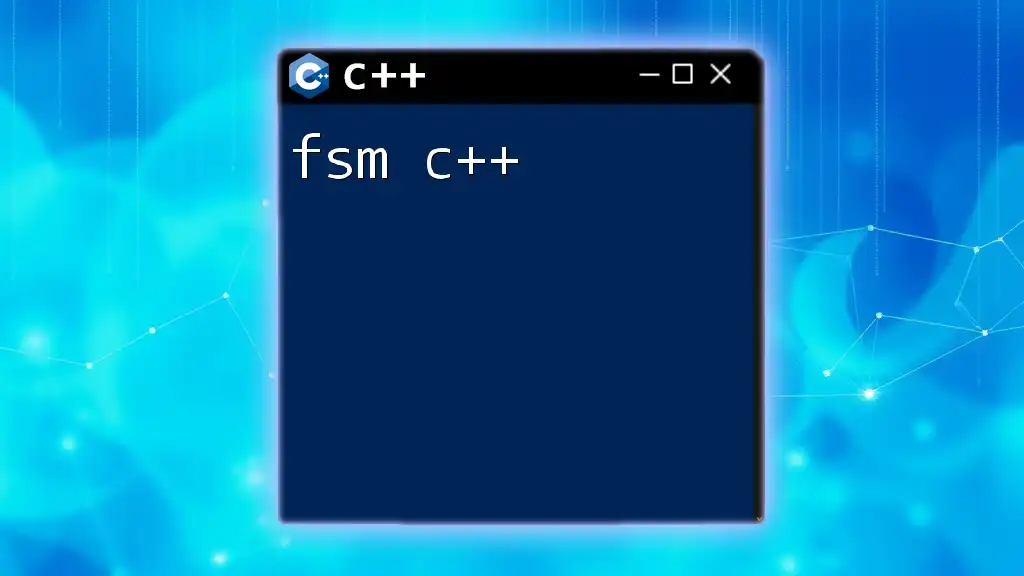
Advanced Topics in C++ on macOS
GUI Development with C++
Creating graphical user interfaces (GUIs) with C++ on macOS often involves using frameworks like Qt. Qt provides a rich set of modules and tools to develop cross-platform GUI applications.
To get started with Qt:
- Download and install the Qt framework from their official website.
- Create a new project through the Qt Creator, selecting a Qt Widgets Application template.
C++ and macOS System Programming
For those interested in lower-level programming, macOS provides numerous system APIs. System programming allows you to interact closely with the operating system, leveraging capabilities such as file I/O and process management.
For example, you can perform basic file operations with the following C++ snippet:
#include <fstream>
#include <iostream>
int main() {
std::ofstream myfile("example.txt");
if (myfile.is_open()) {
myfile << "Hello, OSX C++!";
myfile.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
In this code, an `example.txt` file is created if it doesn’t exist, and the text “Hello, OSX C++!” is written to it.
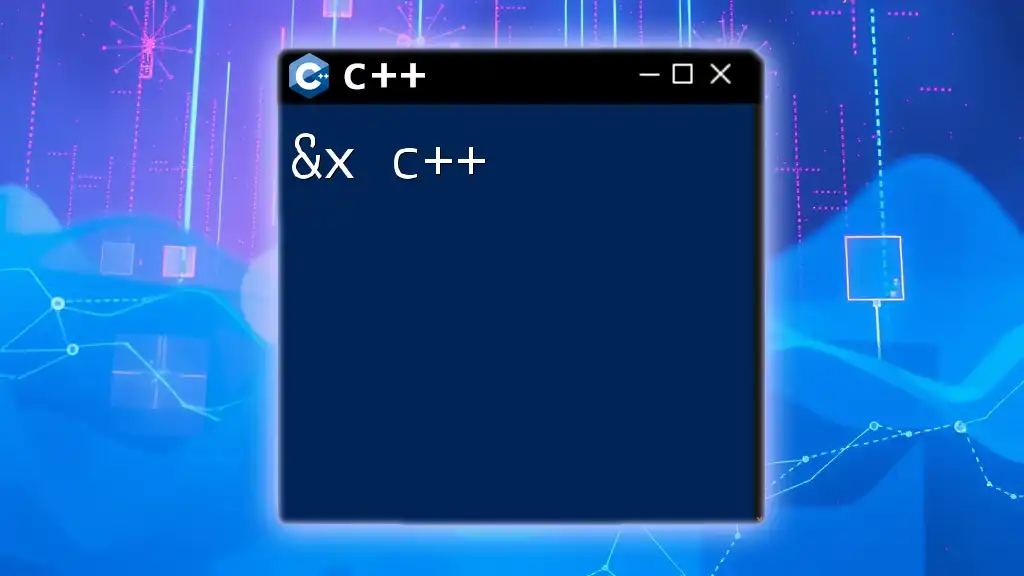
Best Practices and Tips for C++ Development on macOS
Code Organization and Structure
Maintaining an organized project structure is crucial for effective development. A suggested directory layout might include:
- src/: for source files
- include/: for header files
- lib/: for third-party libraries
- tests/: for unit tests
This structure promotes clarity and enables easy navigation through your codebase.
Performance and Optimization
Optimizing C++ code is essential for performance-critical applications. Some general techniques include:
- Memory management: Efficient use of pointers and dynamic memory can minimize memory leaks.
- Algorithm optimization: Utilizing the Standard Template Library (STL) can lead to faster, more efficient code.
To profile your application, Xcode includes Instruments, a powerful tool that can analyze resource usage, memory allocations, and performance bottlenecks.
Community Resources and Support
Being part of the right community can significantly enhance your learning experience. Consider exploring:
- Stack Overflow: A great platform for asking questions and finding answers.
- GitHub: Explore open-source C++ projects and tutorials.
- MacOS developer forums: Localized help related to C++ development on macOS.
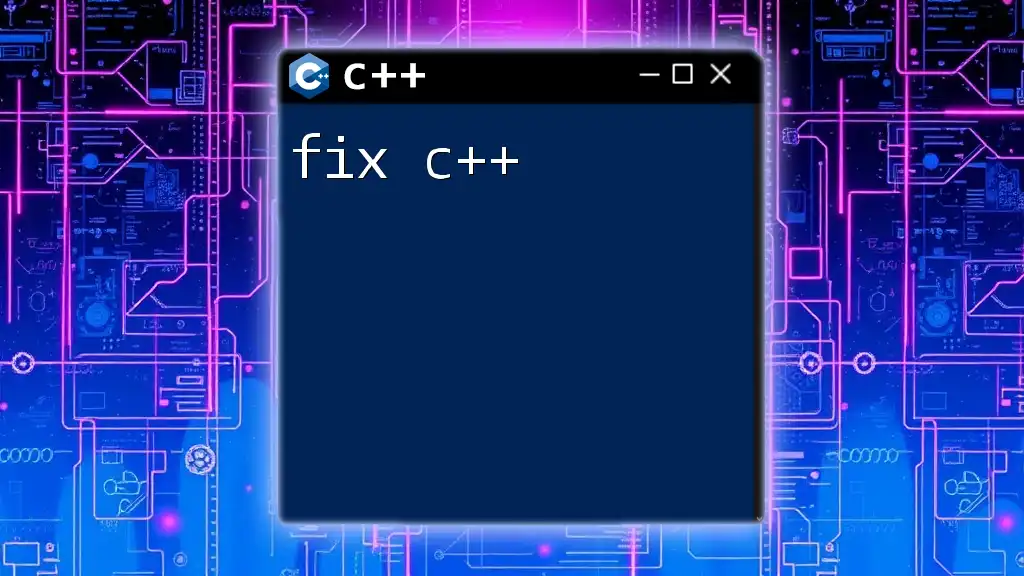
Conclusion
The choice of macOS for C++ development opens doors to a host of powerful tools and resources. By leveraging Xcode and understanding the unique frameworks available, you can create efficient, high-quality applications. As you embark on your C++ journey, remember that the community and resources are here to support you along the way.
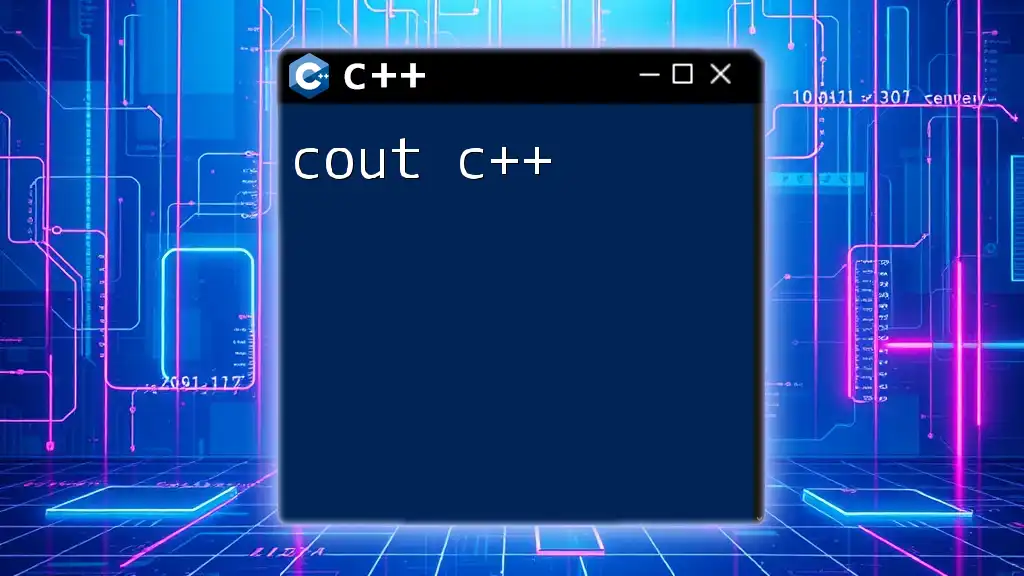
Additional Resources
For further learning and exploration, consider checking out:
- Online courses that specialize in C++ programming.
- Documentation on the C++ Standard Library and macOS APIs.
- GitHub repositories featuring example C++ projects tailored for macOS development.
With this comprehensive guide, you’re now well-equipped to start developing C++ applications on macOS, paving your way in the world of programming.