In C++, the `&` operator is used to obtain the memory address of a variable or to indicate that a function parameter is being passed by reference.
int x = 10;
int* ptr = &x; // ptr now holds the address of x
Understanding the Basics
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. Pointers are fundamental to understanding how data is stored and manipulated in memory.
For example:
int a = 10;
int* p = &a; // Pointer p holds the address of variable a
Here, `p` is a pointer that points to the memory address where `a` is stored. It allows direct access to the value of `a` through the pointer.
What is a Reference?
A reference is an alias for an existing variable. Unlike pointers which can point to different memory addresses, a reference, once initialized, cannot be changed to refer to another variable. This makes references easier to use in certain scenarios.
For example:
int a = 10;
int& ref = a; // ref is a reference to a
In this case, `ref` refers to `a`, and any changes made to `ref` will directly affect `a`.
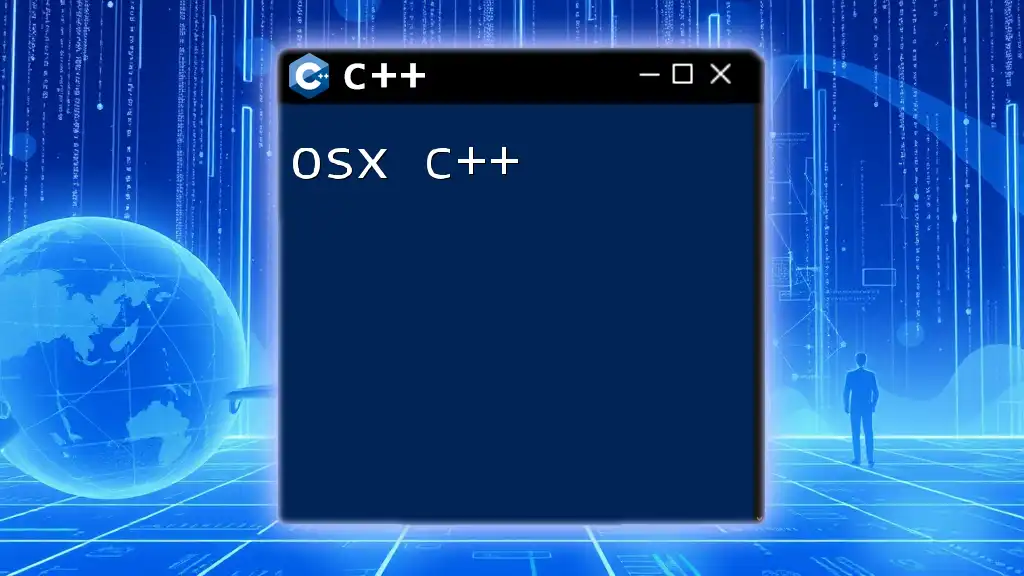
Exploring the "&x" Syntax
The Significance of the Ampersand (&)
In C++, the ampersand (`&`) serves multiple purposes. It indicates the address-of operator when used before a variable, allowing you to capture a variable's memory address. This operator is essential when dealing with pointers and references.
Using "&" in Variable Declaration
The `&` in C++ is not only used with pointers but is also vital in the declaration of references. Understanding the syntax is crucial:
- Pointer Declaration: `int* ptr;` indicates that `ptr` is a pointer to an `int`.
- Reference Declaration: `int& ref;` shows that `ref` is a reference to an `int`.
This syntax distinction emphasizes the difference between pointers and references.
The Process of Address-of Operator
The address-of operator (`&`) allows you to reference the memory address of a variable.
For instance:
int a = 20;
int* p = &a; // p now holds the memory address of a
std::cout << "Address of a: " << p << std::endl; // printing address
In this example, `p` will contain the address of `a`, and using `std::cout`, we can display this address.
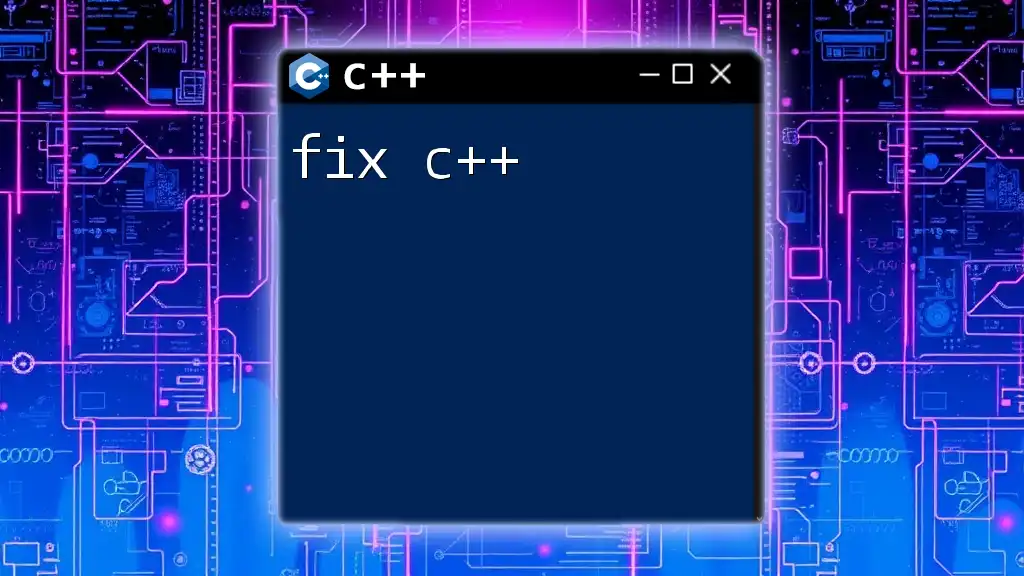
Practical Applications of "&x" in C++
Functions and References
One of the primary uses of references is in function parameters. Passing by reference allows functions to modify the original variable without making a copy. This approach often leads to better performance, especially with large data objects.
For example:
void Increment(int& num) {
num++;
}
In this function, `num` is passed by reference, so any modifications to `num` reflect in the original variable passed to the function.
Returning References from Functions
C++ allows functions to return references as well, which can be beneficial in certain situations. However, it is crucial to ensure that the returned reference refers to valid memory after the function exits.
For example:
int& getElement(int arr[], int index) {
return arr[index]; // Note: Ensure index is valid
}
Here, `getElement` returns a reference to an array element. If the array goes out of scope, using this reference will lead to undefined behavior. Thus, careful consideration is necessary.
Use Cases for Pointers vs. References
While both pointers and references can often achieve the same outcome, they have their unique advantages.
- Pointers:
- Can be reassigned to point to different addresses.
- Can represent a null state (`nullptr`).
- Used in dynamic memory management.
Example of using pointers:
int* p = new int(10); // Dynamic allocation
delete p; // Free memory
- References:
- More intuitive syntax, making the code cleaner.
- Cannot be null or reseat once initialized.
Example of using references:
void process(int& ref) {
ref += 5; // Simpler to use than dereferencing a pointer
}
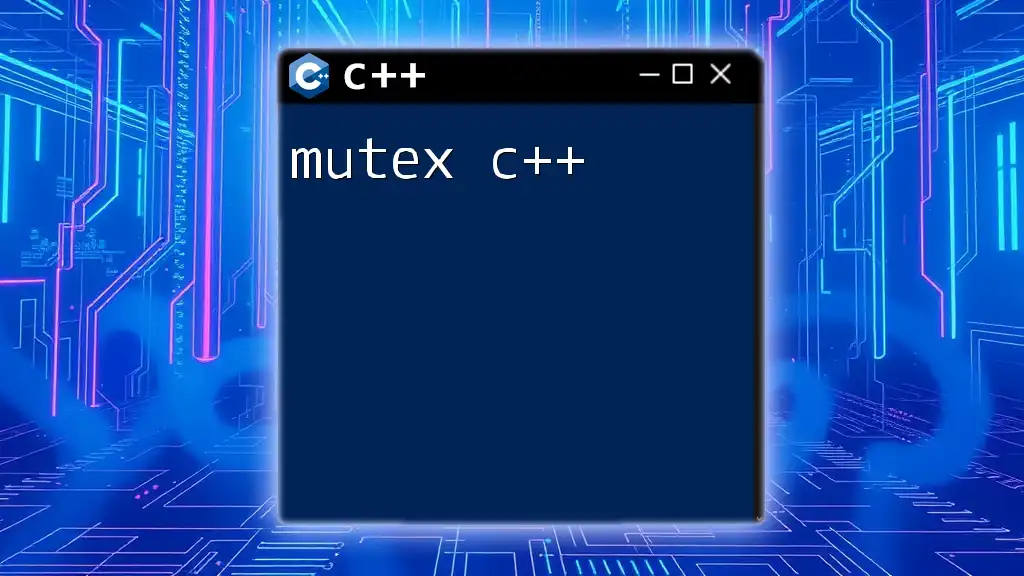
Common Mistakes and Misunderstandings
Misusing the Address-of Operator
One common mistake with the address-of operator is attempting to take the address of an expression or using an uninitialized pointer. It's essential to ensure that pointers are valid before dereferencing them.
For example:
int* q = &(*p); // Ensure p is not NULL before dereferencing!
This could lead to undefined behavior if `p` does not point to a valid address.
Reference Binding to Temporary Objects
A crucial aspect of references in C++ is understanding when they can bind to temporary objects. If a reference is bound to a temporary object, it cannot extend beyond that object's lifetime.
For example:
int getValue() {
return 5;
}
int& ref = getValue(); // This compiles but leads to undefined behavior
Here, `ref` refers to a temporary value returned by `getValue`. Using it later would lead to issues, as the temporary no longer exists.
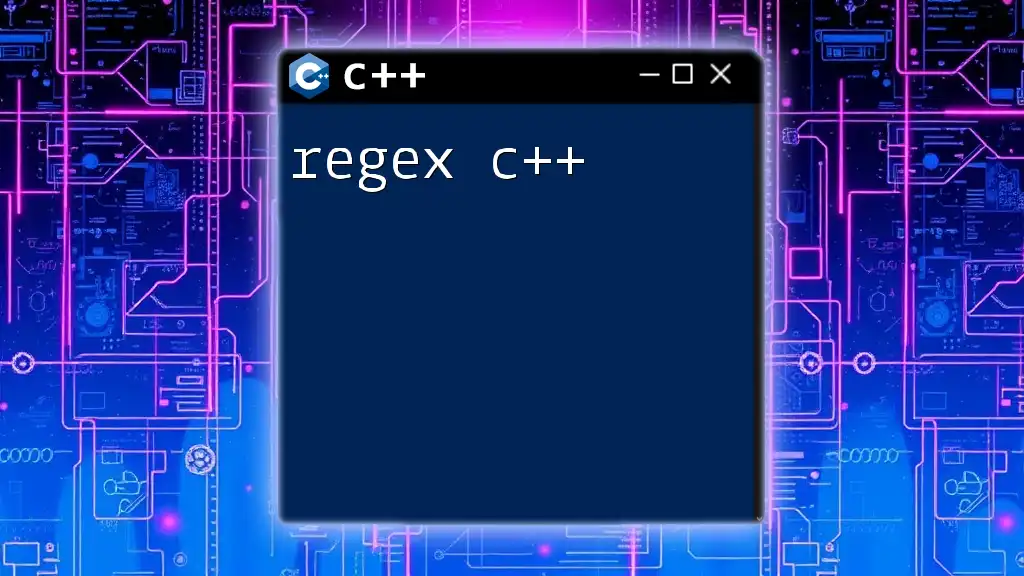
Conclusion
The `&x` syntax in C++ plays an essential role in memory management and variable manipulation. Understanding pointers and references can significantly enhance your programming capabilities, allowing for efficient data handling and function design. Practice is key to mastering these concepts, and leveraging the power of `&` in C++ can lead to cleaner, more efficient code.
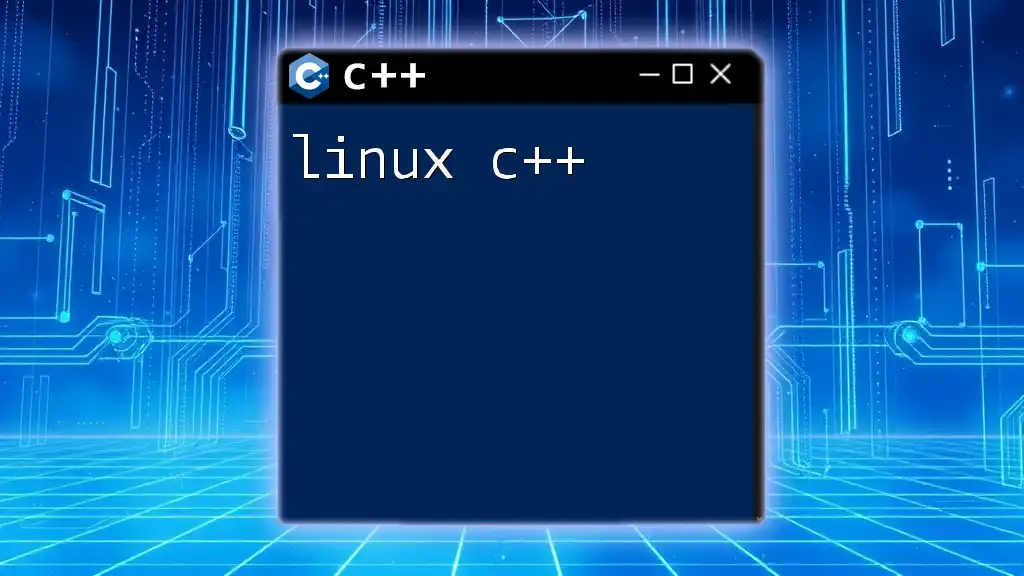
Additional Resources
For further enrichment on the topic of pointers and references in C++, consider exploring C++ documentation, specialized programming books, and joining online communities that discuss C++ programming practices. These resources can provide deeper insights and help reinforce your understanding of pointers and references.