"Fixing C++ typically involves debugging issues in code, optimizing performance, or resolving compilation errors to ensure smooth execution."
Here's a simple example of a common fix in C++ where a missing return statement could lead to undefined behavior:
#include <iostream>
int add(int a, int b) {
return a + b; // Ensure to return the result
}
int main() {
std::cout << "Sum: " << add(5, 3) << std::endl;
return 0;
}
Understanding Common C++ Issues
Types of Errors
Syntax Errors
Syntax errors are violations of C++ language rules and are typically caught at compile time. These errors often stem from simple mistakes, such as forgetting semicolons, curly braces, or using incorrect data types. For example, in the following code snippet, the absence of a semicolon leads to a syntax error:
int main() {
std::cout << "Hello, World!" // Missing semicolon
}
When you encounter a syntax error, carefully read the error message and identify the line number where the issue is reported. Fixing syntax errors is usually straightforward, as they can be resolved by ensuring the correct usage of syntax rules.
Runtime Errors
Runtime errors occur during program execution and are often less predictable. Common examples include dereferencing null pointers or accessing out-of-bounds elements in arrays. Consider this example, which results in a runtime error:
int* ptr = nullptr;
std::cout << *ptr; // Runtime error
To "fix C++" and prevent runtime errors, it’s essential to validate pointers and ensure they are properly initialized before dereferencing.
Logical Errors
Logical errors can be particularly tricky because they do not trigger compiler or runtime errors, yet they lead to incorrect program behavior. These mistakes occur when the logic of your code fails to implement the desired intention. For instance, mistakenly using a single equals sign (`=`) for assignment instead of the double equals sign (`==`) for comparison can result in unexpected behavior:
int a = 5, b = 10;
if (a = b) { // Assignment instead of comparison
std::cout << "Equal";
}
To address logical errors, careful inspection of your algorithm and the conditions involved is critical. Implementing unit tests can help verify that your functions behave as expected.
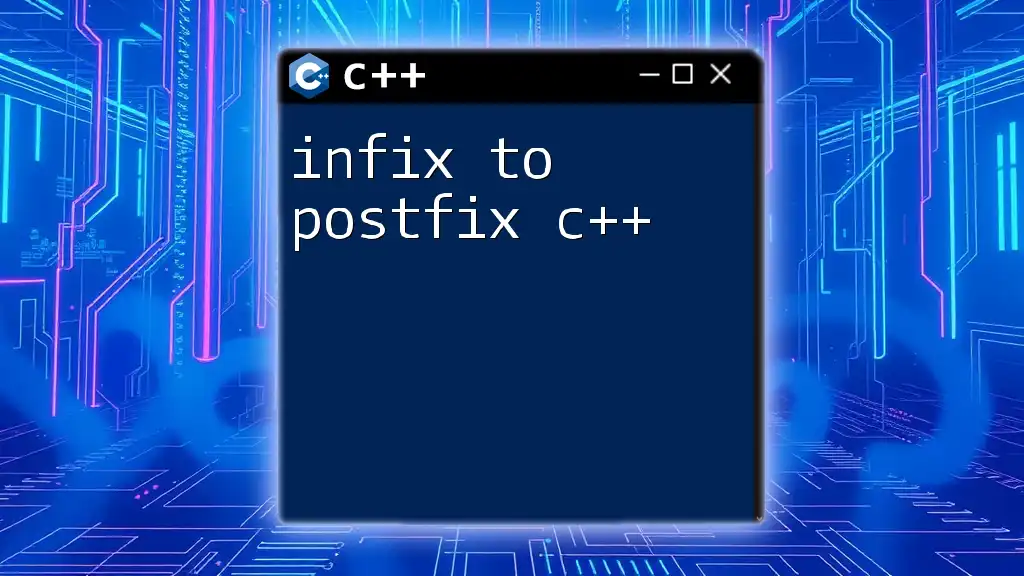
Fixing Common Errors
Using Compiler Feedback
Reading Error Messages
Compilers provide valuable feedback in the form of error messages when issues arise. Understanding these messages is crucial for efficient debugging. For example, if you encounter the error `error: expected ‘;’ before ‘return’`, it indicates that a semicolon is missing before the return statement. Reviewing the line and the surrounding context can help prevent future occurrences.
Debugging Techniques
Using Debuggers
Debuggers are powerful tools that allow developers to pause program execution, set breakpoints, and inspect variables. Setting a breakpoint at a critical juncture enables you to analyze the current state of the program, checking variable values and the flow of execution. Learning to navigate through your code with a debugger can drastically improve the efficiency of your debugging process.
Logging and Print Statements
Utilizing `std::cout` to insert print statements at various points in your code can help track program flow and object states. For instance, if you’re unsure whether a loop is executed correctly, you might add:
std::cout << "Value of x: " << x << std::endl;
Such statements provide insights into variable values and can lead you to the root of the problem.
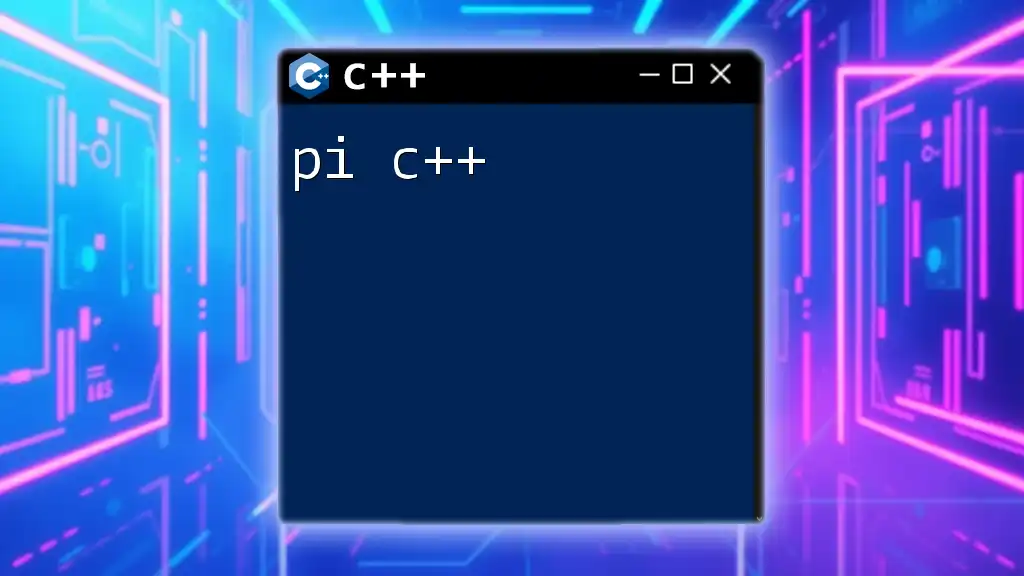
Performance Optimization
Identifying Bottlenecks
Performance bottlenecks can significantly degrade the responsiveness of your C++ programs. Employ profiling tools like Valgrind or gprof. These tools analyze your code and identify functions that consume excessive CPU time or memory. By identifying these bottlenecks, you can focus on optimizing these problem areas to ensure your application runs smoothly.
Common Optimization Techniques
Memory Management
Understanding memory management is essential in C++. Knowing when to use dynamic memory allocation (using `new`) versus automatic variables can prevent memory leaks and ensure proper resource usage:
int* arr = new int[10]; // Dynamic memory allocation
delete[] arr; // Clean up to avoid memory leaks
Always remember to free dynamically allocated memory using `delete` or `delete[]`. Missing this cleanup can lead to severe memory leaks.
Efficient Algorithms
Selecting the right data structures plays a crucial role in performance. For example, `std::vector` is often more efficient than linked lists for random access. Using the appropriate algorithm can greatly improve the efficiency of your program. Understanding the time and space complexity of different algorithms will allow you to make informed decisions when it comes to optimizing your code.
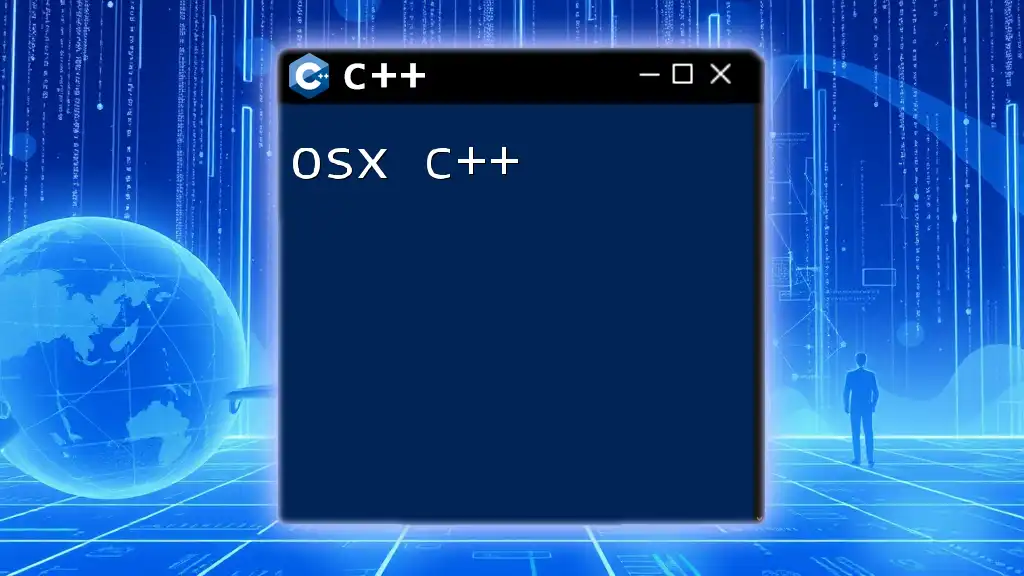
Best Coding Practices
Code Style and Organization
Consistency is key in coding. Following established naming conventions makes your code easier to read and maintain. Use meaningful variable names and adhere to a standard format for declarations and definitions. This practice not only helps you but also benefits others who may read your code in the future.
Comments and Documentation
While code should be as self-explanatory as possible, effective comments can clarify complex logic. Focus on adding comments where necessary to explain the "why" behind your choices, not just the "what." Rather than saying, "increments i," consider using a comment like, "Increment i to track the number of iterations."
Code Reviews
Participating in code reviews is vital for improving both your and your colleagues' code quality. Such reviews often uncover potential issues and provide insights you may not have considered. Regular review practices foster a culture of collaboration and continuous learning.
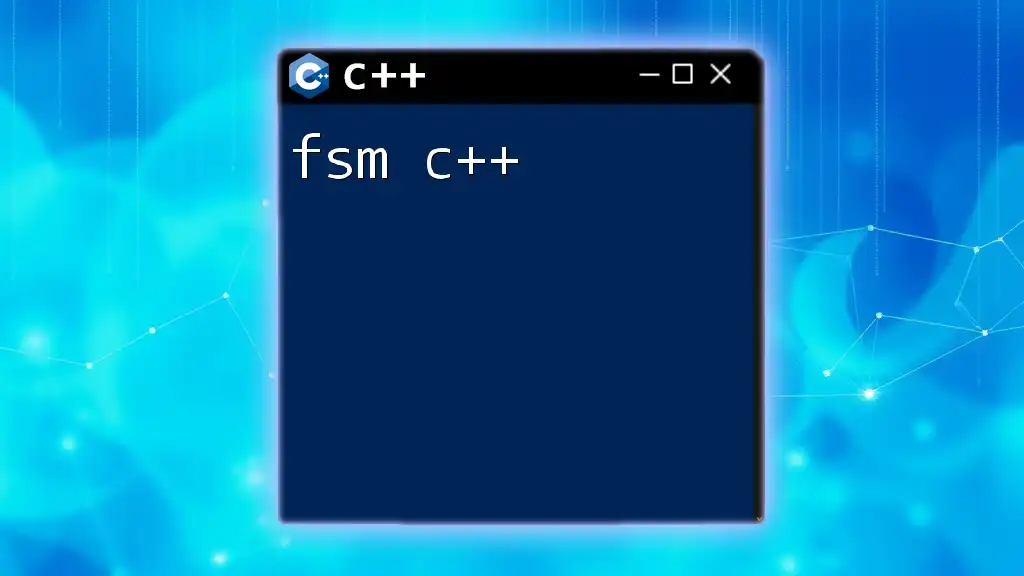
Advanced Fixing Techniques
Using Tools and Libraries
Static analysis tools such as Clang-Tidy or CPPCheck can help you detect potential issues in your code before it's compiled. These tools analyze your code for errors, style issues, and bugs, providing a comprehensive report. Integrating these tools into your development workflow can significantly ease the troubleshooting process.
Refactoring
Refactoring involves improving the structure of your code without altering its functionality. This process can lead to cleaner, more efficient code. Breaking down lengthy functions into smaller, modular components not only enhances readability but also improves maintainability. For example:
// Before refactoring
void processUserData() {
// multiple responsibilities in a single function
}
// After refactoring
void validateUserData();
void saveUserData();
void notifyUser();
By clearly defining different responsibilities, your code becomes easier to understand and maintain.
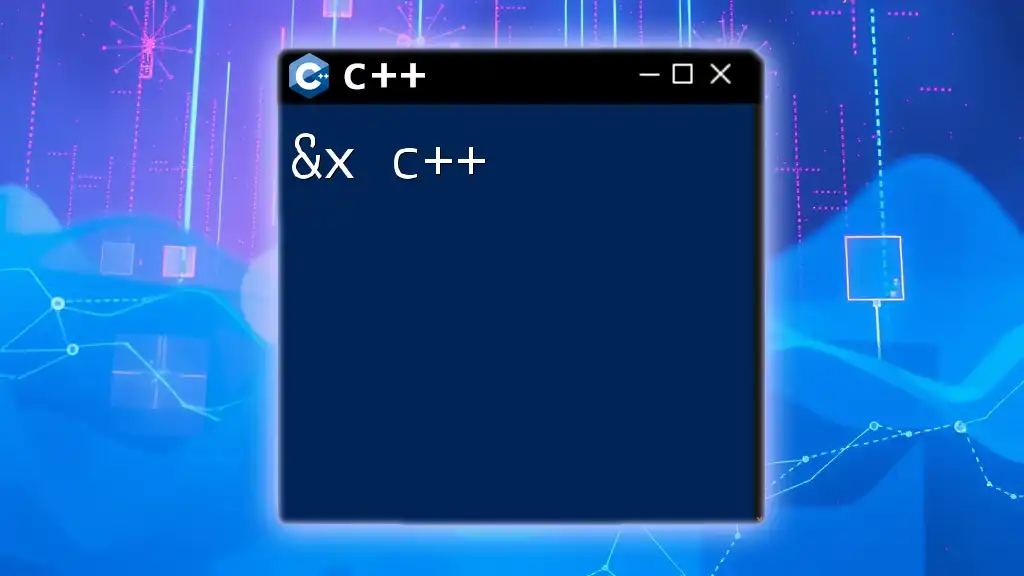
Conclusion
Understanding how to effectively "fix C++" issues can drastically improve the quality and performance of your applications. By familiarizing yourself with common errors, adopting robust debugging techniques, optimizing performance, and following best coding practices, you lay down a solid foundation for writing efficient and maintainable code. Remember, programming is an iterative process; embrace the learning curve and seek continuous improvement in your skills.
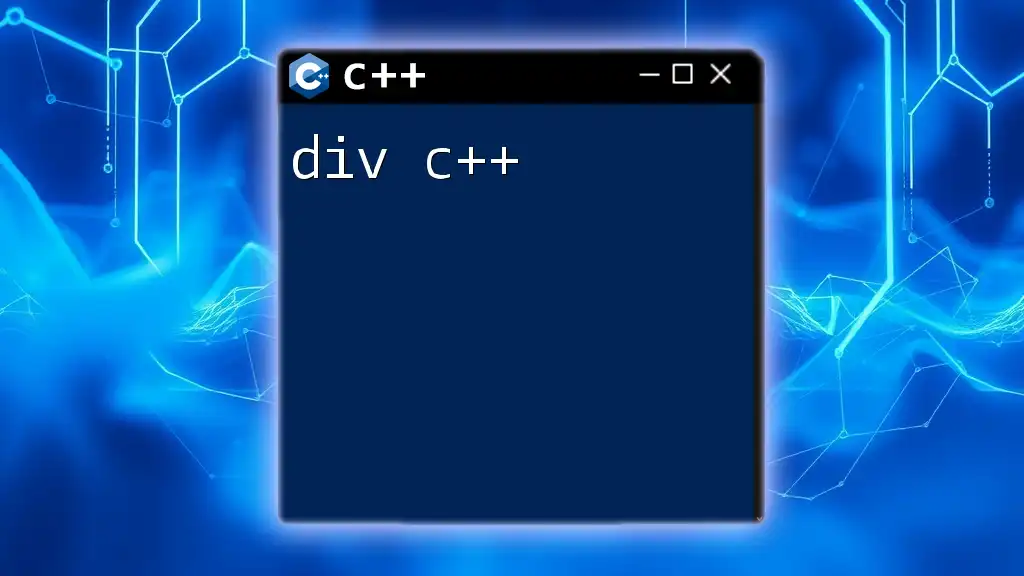
Additional Resources
For readers eager to dive deeper, consider exploring books, websites, or tutorials focused on advanced C++ topics. Engaging with community forums can also provide valuable insights and support as you continue to enhance your C++ proficiency.
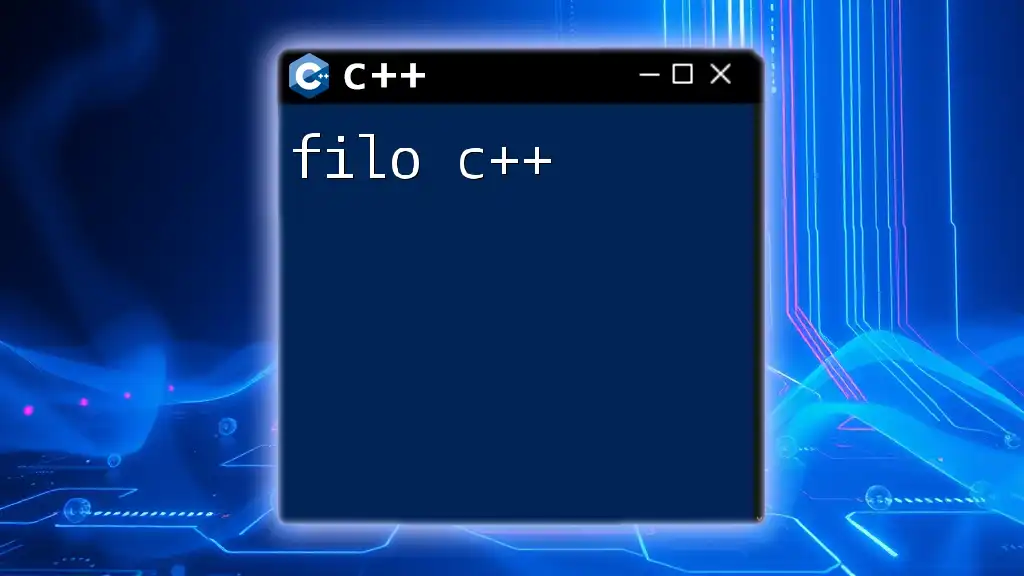
FAQ Section
Common Questions About Fixing C++
-
How can I ensure my code is bug-free?
Implement unit testing, use static analysis tools, and practice thorough code reviews. -
What should I do if I don’t understand an error message?
Consult documentation or community forums, and consider breaking down the code to locate the issue. -
How do I optimize a slow piece of code?
Profile your code to identify bottlenecks, refactor for efficiency, and explore appropriate data structures and algorithms.
By utilizing the strategies and insights shared in this guide, you can effectively fix issues in your C++ code and become a more proficient developer. Happy coding!