In C++, converting an infix expression (like `A + B`) to postfix notation (like `A B +`) can be done using the Shunting Yard algorithm, which utilizes a stack to reorder the operators and operands.
Here's a simple code snippet illustrating this conversion:
#include <iostream>
#include <stack>
#include <cstring>
#include <cctype>
int precedence(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
void infixToPostfix(const std::string& expression) {
std::stack<char> operators;
std::string postfix = "";
for (char c : expression) {
if (isalnum(c)) {
postfix += c; // Add operand to output
} else {
while (!operators.empty() && precedence(operators.top()) >= precedence(c)) {
postfix += operators.top(); // Add operator to output
operators.pop();
}
operators.push(c); // Push current operator onto stack
}
}
while (!operators.empty()) {
postfix += operators.top(); // Add remaining operators to output
operators.pop();
}
std::cout << "Postfix: " << postfix << std::endl;
}
int main() {
std::string infix = "A+B*C";
infixToPostfix(infix);
return 0;
}
This code defines a function `infixToPostfix` that converts an infix expression into postfix notation and prints the result.
Understanding Infix and Postfix Notations
What is Infix Notation?
Infix notation is the conventional way of writing expressions where operators are placed between their operands. For example, the expression `A + B` signifies that A is added to B. Infix expressions can also include multiple operations and use parentheses to denote the order of evaluation clearly. For instance, an expression like `(C * D) - E` indicates that C multiplied by D should be calculated first before subtracting E.
The primary challenge with infix notation arises from the need to consider operator precedence and explicit parentheses. The operator precedence dictates which operations are performed first, while parentheses can alter this default order, enhancing readability.
What is Postfix Notation?
Postfix notation, also known as Reverse Polish Notation (RPN), is an arithmetic notation in which every operator follows all of its operands. Thus, instead of writing `A + B`, you write `AB+`. In postfix, the order of operations is strictly enforced by the position of the operators and operands, eliminating the need for parentheses.
The advantages of using postfix notation are significant:
- Simplicity in Parsing: It allows for easier parsing by machines without needing to consider operator precedence.
- No Parentheses Needed: In postfix, there’s no ambiguity; the position defines how expressions are evaluated.
For example, the infix expression `(A + (B * C))` is represented in postfix form as `ABC*+`.
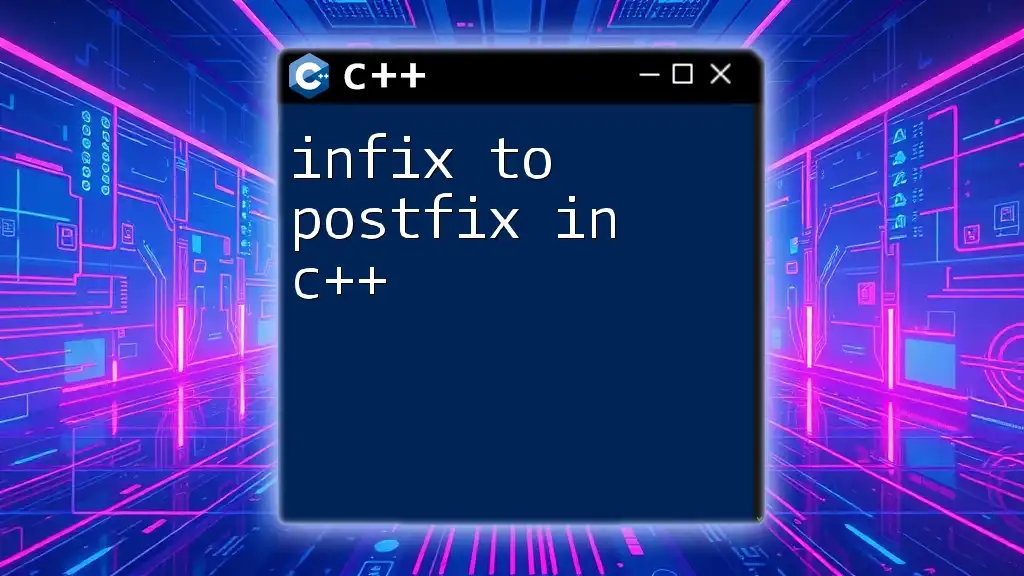
The Rules for Conversion
Operator Precedence
Understanding operator precedence is crucial when converting from infix to postfix. The basic precedence rules are as follows:
- Multiplication (`*`) and Division (`/`) take precedence over Addition (`+`) and Subtraction (`-`).
- Operators of the same precedence are usually evaluated from left to right, although some can be right associative.
Here’s a simple precedence table for reference:
Operator | Precedence |
---|---|
`+` `-` | 1 |
`*` `/` | 2 |
`^` | 3 (right associative) |
Associativity of Operators
In addition to precedence, associativity plays a critical role in how expressions are evaluated. For most operators, including `+`, `-`, `*`, and `/`, the associativity is left-to-right. This means that if two operators of the same precedence appear, the one on the left is evaluated first.
For example, in the expression `A - B + C`, the operation evaluates as `(A - B) + C` due to left-to-right associativity.
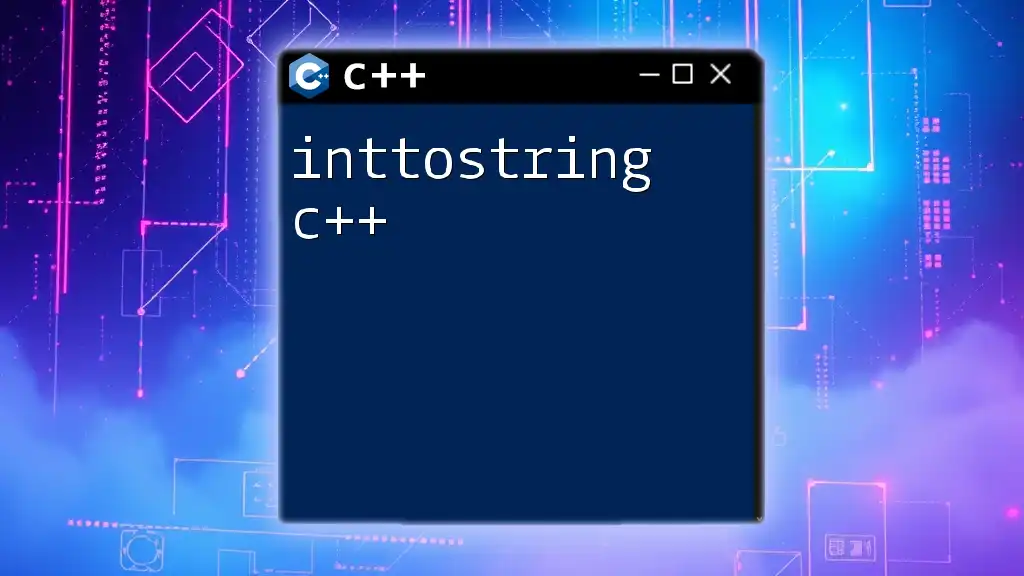
Algorithm for Infix to Postfix Conversion
Overview of the Shunting Yard Algorithm
The Shunting Yard algorithm, created by Edsger Dijkstra, is the most commonly used technique for converting infix expressions to postfix notation. The algorithm uses two primary structures—a stack for operators and an output queue for the final postfix expression.
Steps of the Conversion Process
Reading Tokens
When converting an expression, we read the input token by token. The tokens can include operators, operands (numbers or variables), and parentheses.
Handling Different Tokens
- Operands: If the token is an operand (variable or number), it is added directly to the output queue.
- Operators: When the token is an operator, check the stack and the operator precedence:
- Pop from the stack to the output queue until you find an operator of lower precedence or a left parenthesis.
- Push the current operator onto the stack.
- Left Parenthesis `(`: Push the left parenthesis onto the stack to indicate a sub-expression.
- Right Parenthesis `)`: Pop operators from the stack to the output queue until encountering a left parenthesis. Pop and discard the left parenthesis.
Finalizing the Output
Once the entire infix expression has been read, any operators that remain in the stack are popped to the output. This ensures all operations are accounted for in the postfix notation.
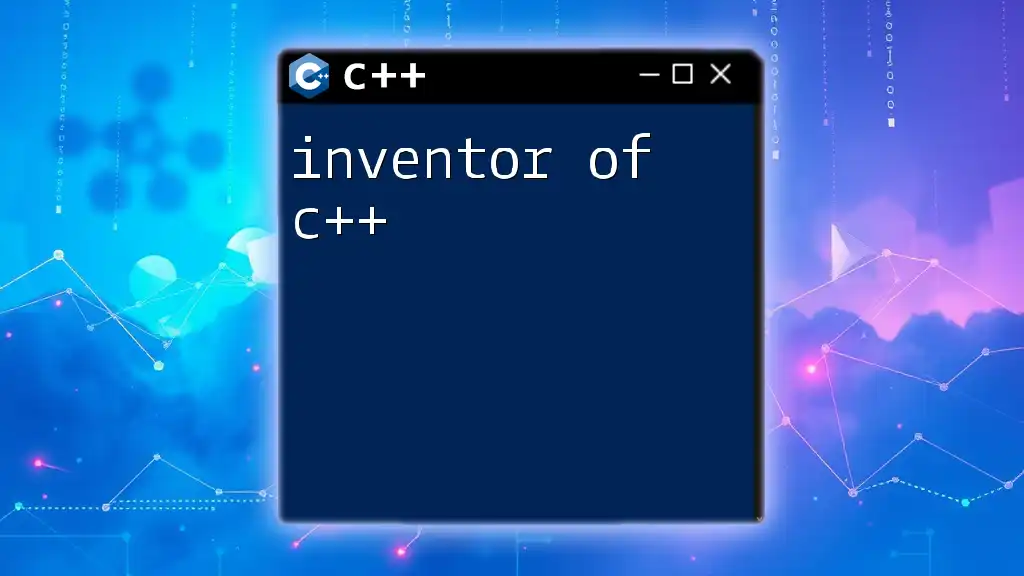
Implementing Infix to Postfix Conversion in C++
Setting Up the Environment
To implement the conversion algorithm in C++, you will need a standard development environment. IDEs like Visual Studio, Code::Blocks, or online compilers can be used. Ensure you include the necessary libraries for handling stacks and strings:
#include <iostream>
#include <stack>
#include <string>
Sample Code Implementation
Structure of the Code
Breaking down the code into functions enhances readability. Here’s how you can structure it:
Function to Check Precedence:
int precedence(char op) {
if(op == '+' || op == '-') return 1;
if(op == '*' || op == '/') return 2;
return 0; // for parentheses
}
Complete Code Example
Below is a complete implementation of infix to postfix conversion:
#include <iostream>
#include <stack>
#include <string>
using namespace std;
string infixToPostfix(const string& expression) {
stack<char> operators;
string output;
for (char token : expression) {
if (isalnum(token)) { // Operand
output += token;
} else if (token == '(') {
operators.push(token);
} else if (token == ')') {
while (!operators.empty() && operators.top() != '(') {
output += operators.top();
operators.pop();
}
operators.pop(); // Pop the '('
} else { // Operator
while (!operators.empty() && precedence(operators.top()) >= precedence(token)) {
output += operators.top();
operators.pop();
}
operators.push(token);
}
}
while (!operators.empty()) {
output += operators.top();
operators.pop();
}
return output;
}
Running the Code
To see the conversion in action, you can write a simple `main` function:
int main() {
string infix = "A+(B*C)";
string postfix = infixToPostfix(infix);
cout << "Postfix: " << postfix << endl; // Output: ABC*+
return 0;
}
This code effectively reads an infix expression and converts it into postfix notation, illustrating the power and efficiency of this algorithm.
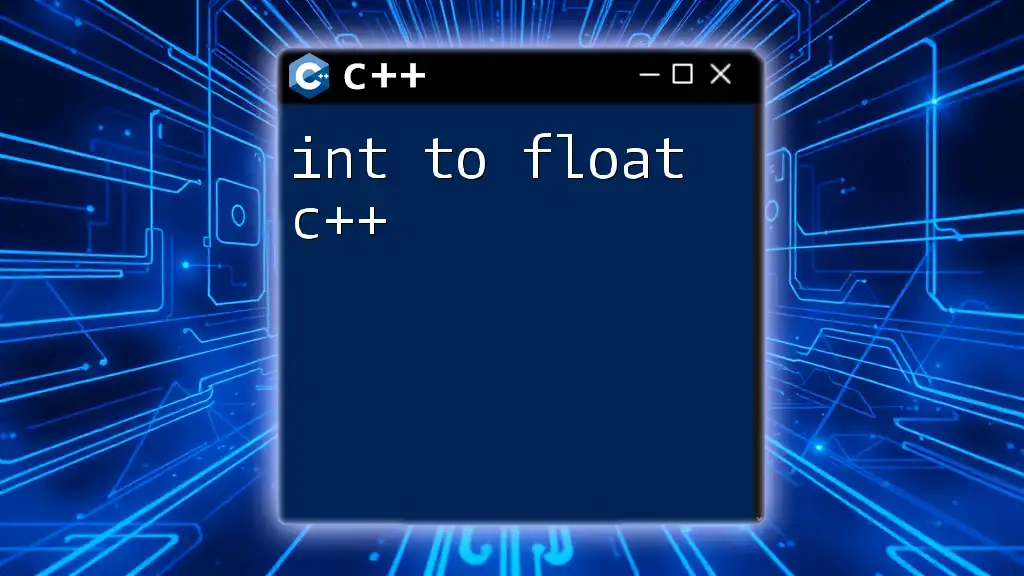
Handling Edge Cases and Errors
Common Mistakes in Conversion
While converting infix to postfix notation, some common issues can arise. For example, failing to respect the operator precedence can lead to incorrect calculations. Additionally, mismatched parentheses in the input can cause the algorithm to produce erroneous outputs.
Debugging Tips
To troubleshoot issues in your implementation:
- Use print statements to visualize the current state of the stack and output after processing each token. This can help identify where the algorithm is deviating from expectations.
- Test with various expressions, including edge cases such as empty expressions or those with only operators, to ensure robustness.
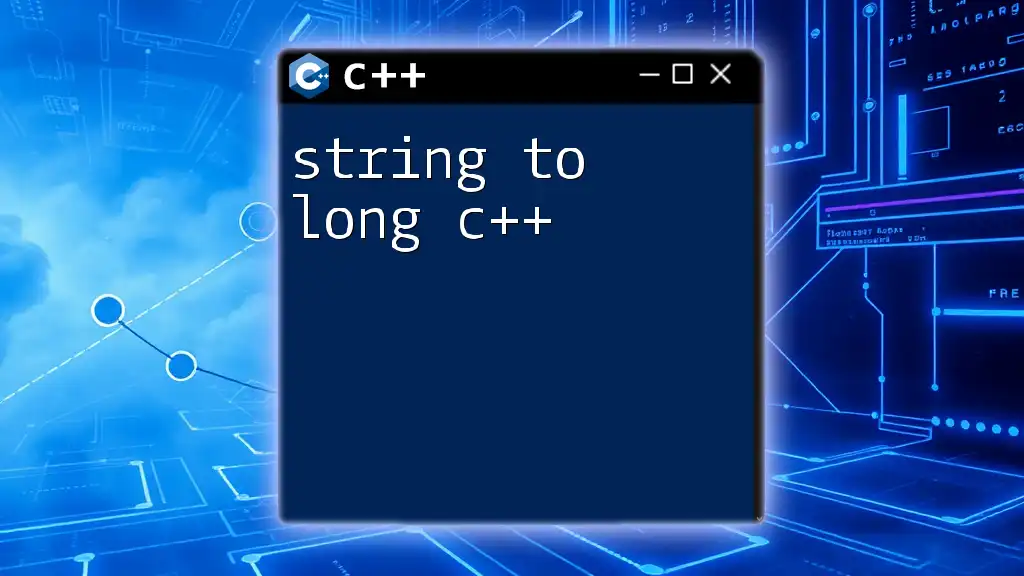
Conclusion
The conversion of infix expressions to postfix notation using C++ is an essential skill for programmers dealing with expression parsing and evaluation. By understanding the operator precedence, associativity, and implementing the Shunting Yard algorithm, you can effectively handle a variety of mathematical expressions without ambiguity.
With practice, you can tackle more complex expressions, enhancing your relevant skills in C++ development. Remember to explore additional resources for deeper learning and mastery of expression parsing.