The infix to postfix conversion in C++ can be accomplished using a stack to rearrange operators and operands for easier expression evaluation. Here’s a simple implementation:
#include <iostream>
#include <stack>
#include <string>
#include <cctype>
int precedence(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
std::string infixToPostfix(const std::string& infix) {
std::stack<char> operators;
std::string postfix;
for (char ch : infix) {
if (isalnum(ch)) {
postfix += ch; // Add operand to output
} else {
while (!operators.empty() && precedence(operators.top()) >= precedence(ch)) {
postfix += operators.top(); // Pop operator from stack
operators.pop();
}
operators.push(ch); // Push current operator to stack
}
}
while (!operators.empty()) {
postfix += operators.top(); // Pop all remaining operators
operators.pop();
}
return postfix;
}
int main() {
std::string infix = "a+b*c";
std::string postfix = infixToPostfix(infix);
std::cout << "Postfix: " << postfix << std::endl;
return 0;
}
Understanding Notation Systems
What is Infix Notation?
Infix notation is the most common way of writing expressions, where operators are placed between the operands. For example, the expression `A + B` indicates that A is to be added to B. While this format is intuitive for humans, it poses challenges for computation, especially with operator precedence and parentheses.
What is Postfix Notation?
Postfix notation, also known as Reverse Polish Notation (RPN), places the operator after the operands. Using the previous example, the equivalent postfix expression would be `A B +`. This method eliminates the need for parentheses to denote precedence, greatly simplifying the evaluation process for computers.
Comparison between Infix and Postfix
When comparing infix and postfix, it's essential to look at their strengths and weaknesses:
-
Infix Notation
- Advantages:
- Natural for human expression.
- Readable in everyday contexts.
- Disadvantages:
- Requires parentheses for specifying precedence.
- More difficult for machines to evaluate directly.
- Advantages:
-
Postfix Notation
- Advantages:
- No need for parentheses.
- Easier for computers to parse and evaluate.
- Disadvantages:
- Less intuitive for humans.
- Requires understanding of the postfix structure.
- Advantages:
Understanding these notations is crucial for programmers, particularly when dealing with expression evaluation and compiler design.
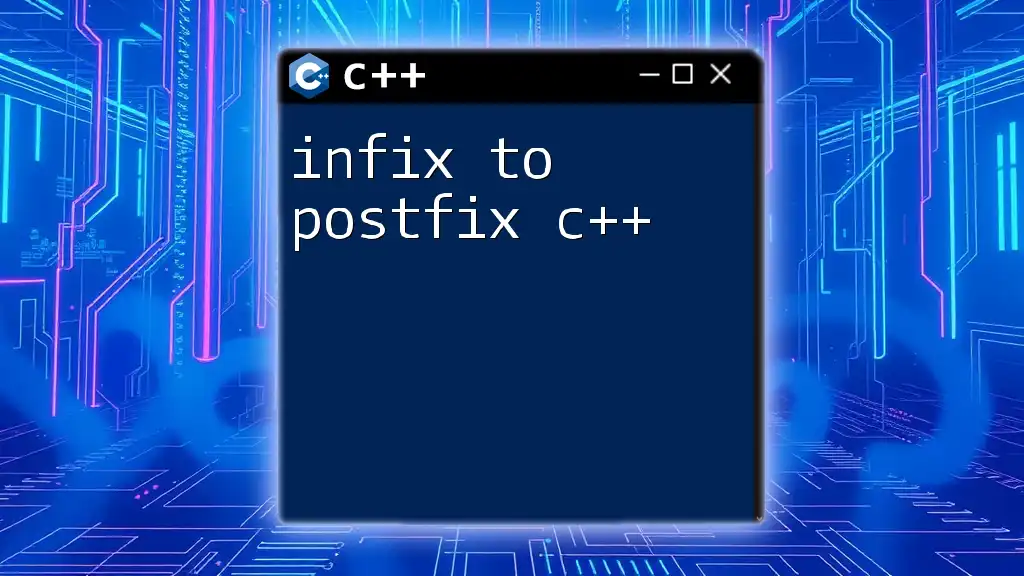
Algorithm for Converting Infix to Postfix
Overview of the Algorithm
The Shunting Yard Algorithm, developed by Edsger Dijkstra, provides a systematic approach to converting infix expressions to postfix notation. This algorithm adopts a stack-based approach, allowing the correct handling of operator precedence and associativity.
Key Terminology
Before diving deeper, it's crucial to define some key terms that will be used throughout the conversion process:
- Operators: Symbols that specify the operation, like `+`, `-`, `*`, and `/`.
- Operands: Variables or constants such as `A`, `B`, `5`, etc.
- Precedence: Indicates the priority of operators—higher precedence operators are evaluated first.
- Associativity: Dictates the order of operations when operators of the same precedence appear (left-to-right or right-to-left).
Operator Precedence and Associativity Rules
To successfully implement the conversion algorithm, understanding operator precedence and associativity is crucial. For instance:
- `*` and `/` have higher precedence than `+` and `-`.
- Operators like `+` and `-` associate from left to right, while `^` associates from right to left.
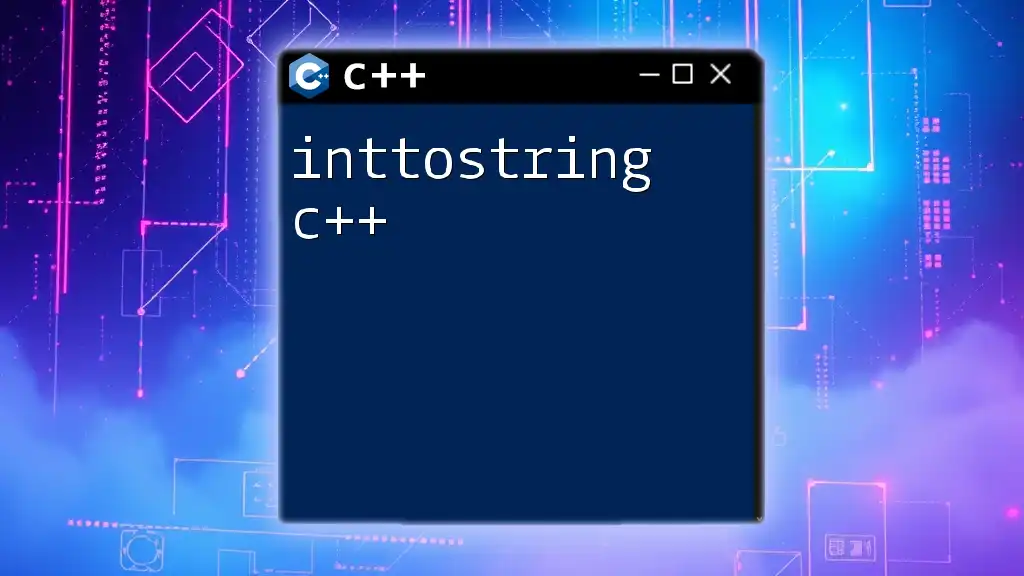
Implementing the Conversion in C++
Setting Up the Environment
To begin coding, you will need a C++ development environment set up on your system. This usually involves a C++ compiler (like GCC or Clang), a text editor or IDE (such as Visual Studio, Code::Blocks, or Sublime Text), and the Standard Template Library (STL) for data structures.
Code Structure
A well-organized C++ program consists of clearly defined functions and classes. For this implementation, the main components will include:
- A function to define operator precedence.
- The main conversion function from infix to postfix.
Implementation Code
To illustrate the process, here’s a fully functioning implementation of the infix to postfix conversion.
Step 1: Include Necessary Libraries
First, include the necessary libraries required for the code:
#include <iostream>
#include <stack>
#include <string>
#include <cctype>
Step 2: Define Precedence Function
This function determines the precedence of different operators:
int precedence(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
Step 3: Convert Infix to Postfix Function
Now, let’s write the function that converts infix expressions to postfix:
std::string infixToPostfix(const std::string& infix) {
std::stack<char> operators;
std::string postfix = "";
for (char c : infix) {
if (isalnum(c)) {
postfix += c; // Add operand to postfix
} else if (c == '(') {
operators.push(c); // Push '(' to stack
} else if (c == ')') {
while (!operators.empty() && operators.top() != '(') {
postfix += operators.top();
operators.pop();
}
operators.pop(); // Remove '(' from the stack
} else { // Operator
while (!operators.empty() && precedence(operators.top()) >= precedence(c)) {
postfix += operators.top();
operators.pop();
}
operators.push(c); // Push current operator to stack
}
}
// Pop all remaining operators
while (!operators.empty()) {
postfix += operators.top();
operators.pop();
}
return postfix;
}
Code Explanation
In this implementation:
- The function initializes a stack to hold operators and a string to build the postfix expression.
- It iterates through each character of the infix expression:
- If the character is an operand, it gets appended directly to the postfix output.
- If it encounters a left parenthesis `(`, it pushes it onto the stack.
- For a right parenthesis `)`, it pops from the stack until it finds the corresponding `(`.
- When an operator is encountered, it checks the precedence of operators in the stack, popping them to the output as long as they have equal or higher precedence than the current operator, before pushing the current operator onto the stack.
- After processing all characters, any remaining operators in the stack are popped into the postfix expression.
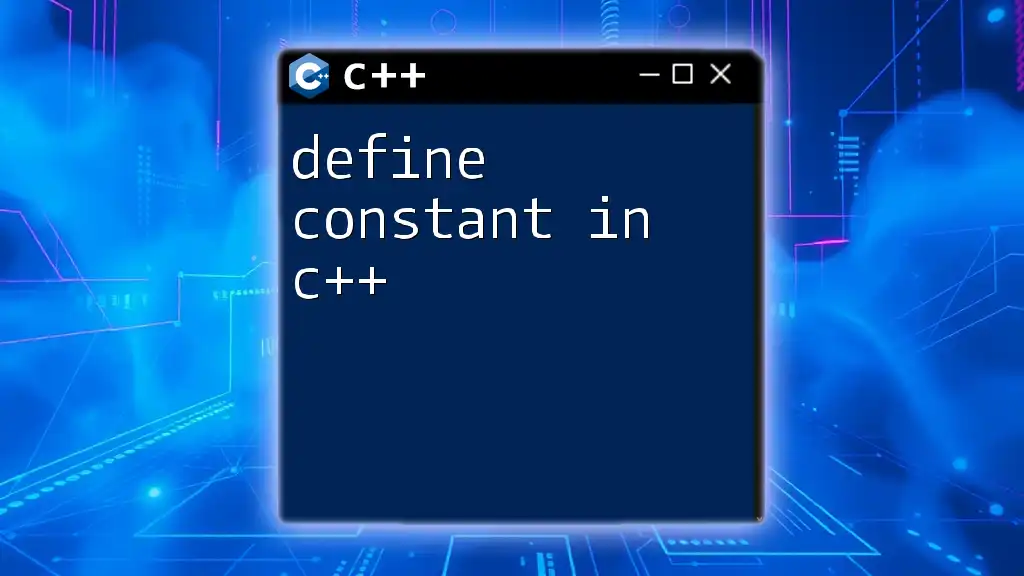
Example of Infix to Postfix Conversion
Example Expression
Consider the infix expression `(A + B) * C - D`.
Step-by-Step Conversion
- Read `(`: Push onto stack.
- Read `A`: Add to postfix: `A`.
- Read `+`: Push onto stack.
- Read `B`: Add to postfix: `AB`.
- Read `)`: Pop until `(`: Postfix becomes `AB+`.
- Read `*`: Push onto stack.
- Read `C`: Add to postfix: `AB+C`.
- Read `-`: Pop `` (higher precedence), then pop `(`: Postfix becomes `AB+C`.
- Read `D`: Add to postfix: `AB+C*D`.
- End of expression: Pop remaining operators: Final postfix becomes `AB+C*D-`.
Output of the Example Code
When you run the function with the given expression, it yields:
Infix: (A + B) * C - D
Postfix: AB+C*D-
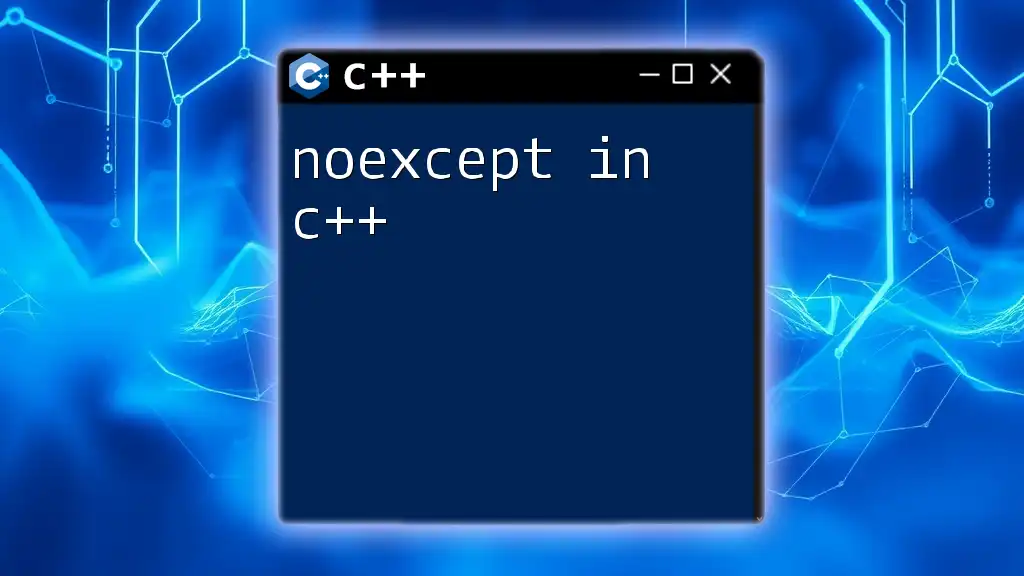
Testing the Implementation
Sample Test Cases
Testing the implementation with various expressions is vital to ensure correctness. Examples include:
- Infix: `A * (B + C)` ➔ Postfix: `ABC+*`
- Infix: `X + (Y - Z) / W` ➔ Postfix: `XYZ-W/+`
- Infix: `(P * Q) - (R + S)` ➔ Postfix: `PQ*RS+ -`
Validating the Output
For each conversion, ensure the output matches the expected postfix expression. This validation process strengthens the implementation's reliability.
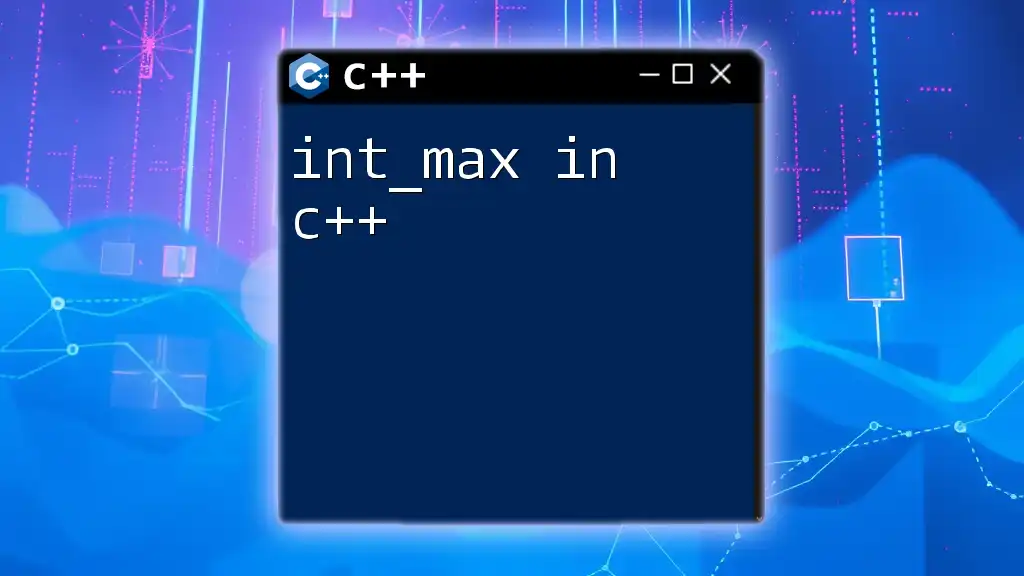
Conclusion
Understanding how to convert infix to postfix in C++ is a fundamental skill for programmers, especially when handling expression evaluations or designing compilers. The Shunting Yard Algorithm provides an efficient means of converting various mathematical expressions into a format that's simpler for machines to execute.
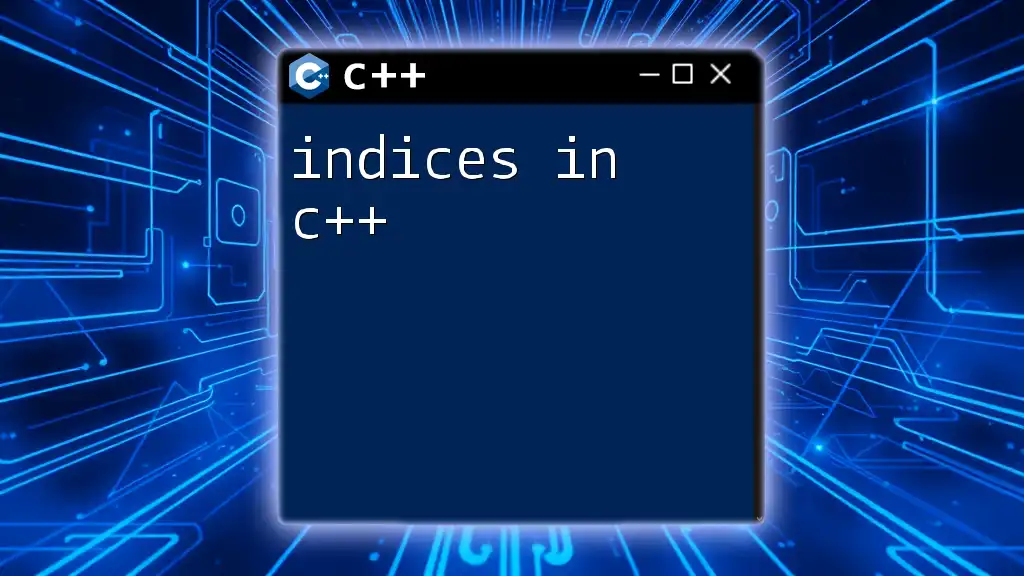
Additional Resources
Books and References
- “Compilers: Principles, Techniques, and Tools” by Alfred V. Aho, Monica S. Lam, Ravi Sethi, and Jeffrey D. Ullman
- “The Art of Computer Programming” by Donald E. Knuth
Online Tutorials
- Explore platforms such as Codecademy or Coursera for additional programming courses focusing on data structures and algorithms.
Join the Community
Engaging with programming communities on platforms like Stack Overflow or Reddit can enhance your learning. Sharing knowledge and solutions with peers can deepen your understanding of algorithms and their applications.
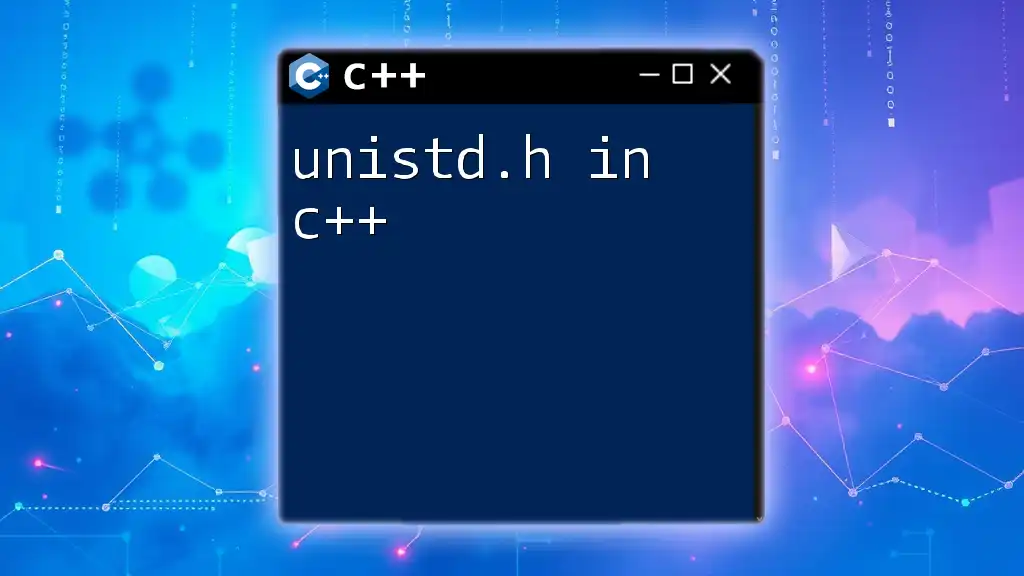
Call to Action
Are you ready to master C++ commands and develop your programming skills? Sign up for your course now to learn how to achieve proficiency quickly and concisely!