In C++, a mutex (mutual exclusion) is used to prevent data races by ensuring that only one thread can access a resource at a time; here’s a simple example demonstrating its usage:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void print_numbers(int id) {
mtx.lock();
std::cout << "Thread " << id << ": ";
for (int i = 1; i <= 5; ++i) {
std::cout << i << " ";
}
std::cout << std::endl;
mtx.unlock();
}
int main() {
std::thread t1(print_numbers, 1);
std::thread t2(print_numbers, 2);
t1.join();
t2.join();
return 0;
}
What is a Mutex?
A mutex, short for "mutual exclusion," is a synchronization primitive that plays a critical role in multithreaded programming. It allows you to ensure that only one thread accesses a shared resource at a time, thereby preventing conditions where data may be corrupted or inconsistently read due to concurrent modifications.
Using a mutex allows for safe interaction with shared resources, which is essential when multiple threads are involved. Without proper synchronization, your application can run into race conditions, where the output or the state of shared data depends on the timing of the threads, leading to unpredictable behavior.
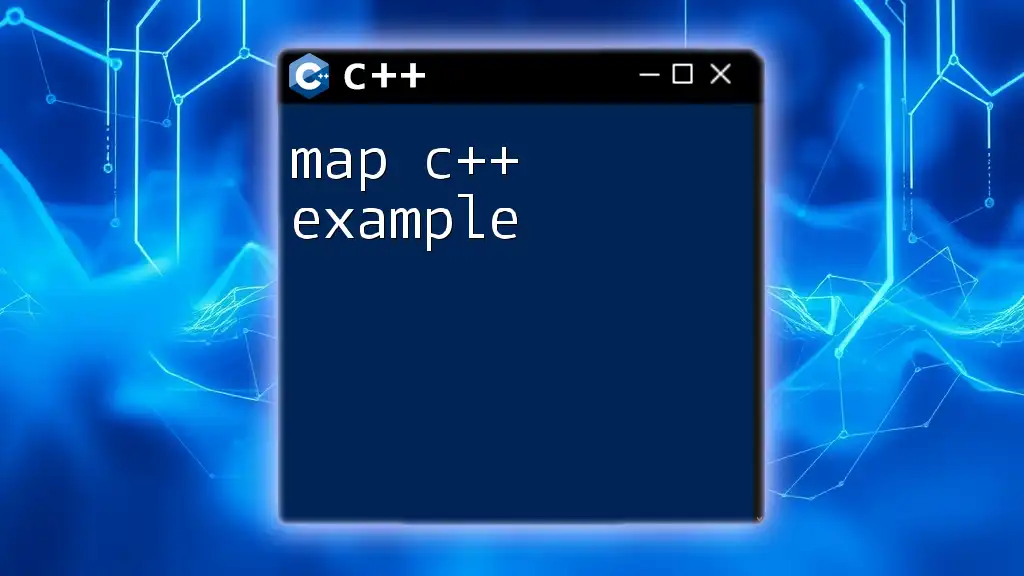
Why Use Mutex in C++?
With the rise of parallel programming, ensuring thread safety has never been more crucial. Here are some key reasons to use mutexes:
- Thread Safety: Protect shared data from concurrent access, preventing data races.
- Consistency: Maintain the integrity of your data, ensuring that no thread sees a corrupted state.
- Multi-threading Support: In a multithreaded application, mutexes provide the necessary tools for managing shared resources safely.
Consider a scenario where two threads try to update a counter at the same time without a mutex. The absence of synchronization may lead to lost updates, where one thread's changes override another's because they read the same value simultaneously. Mutexes mitigate this by locking the counter while it is being updated.
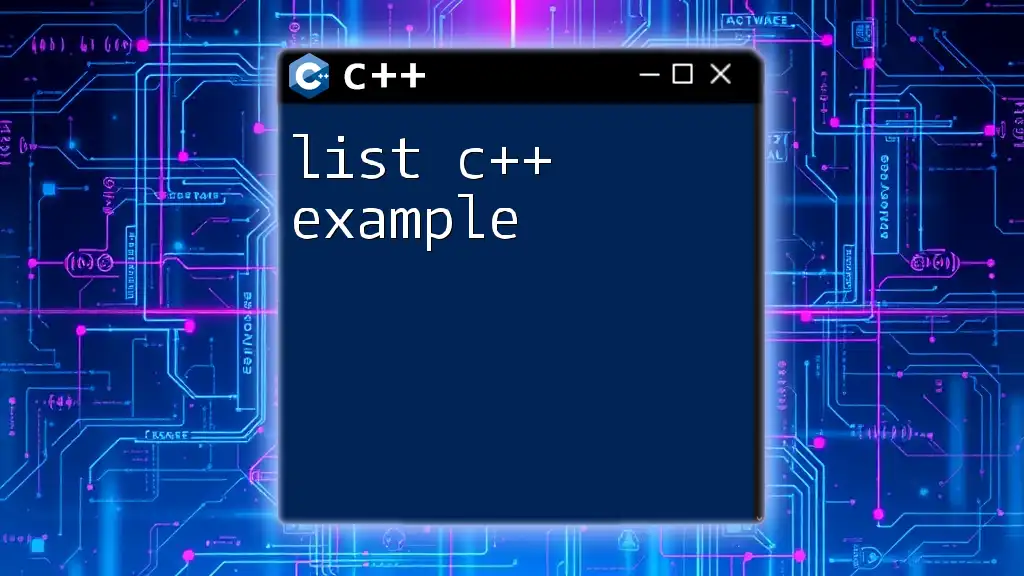
Setting Up Your Environment
Before diving into our `mutex c++ example`, ensure you have a suitable development environment set up. Here’s a quick guide:
- Compiler: Ensure you have a modern C++ compiler that supports C++11 or later, as mutex features are part of this version.
- IDE/Text Editor: Use a reliable Integrated Development Environment (IDE) such as Visual Studio, Code::Blocks, or any text editor that supports C++.
- Project Setup: Create a new C++ project and ensure the necessary compiler flags or settings are configured for C++11 support.
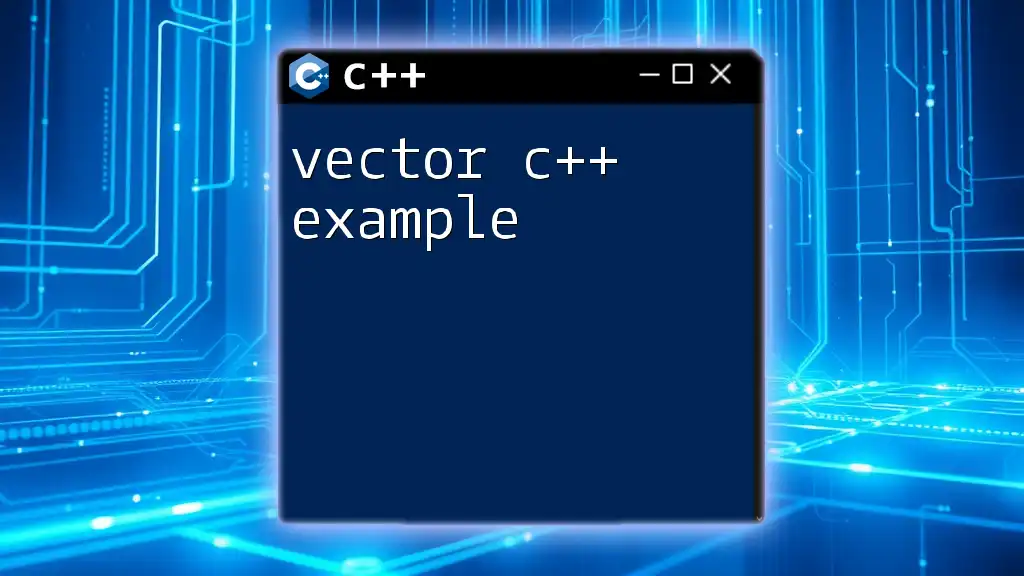
Basic Mutex Example in C++
Understanding the Basics
To implement a mutex in C++, you need to include the `<mutex>` header file. Here’s how to initialize a mutex object:
#include <mutex>
std::mutex myMutex; // Mutex declaration
With this setup, you can manage access to shared resources effectively.
Example Code Snippet
Here’s a simple `mutex c++ example` that demonstrates basic mutex usage:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void print_numbers(int id) {
mtx.lock(); // Lock the mutex before accessing shared resource
// Critical Section
std::cout << "Thread " << id << ": ";
for (int i = 0; i < 5; ++i) {
std::cout << i << " ";
}
std::cout << std::endl;
mtx.unlock(); // Unlock the mutex after accessing shared resource
}
int main() {
std::thread threads[3];
for (int i = 0; i < 3; ++i) {
threads[i] = std::thread(print_numbers, i);
}
for (int i = 0; i < 3; ++i) {
threads[i].join(); // Wait for all threads to finish
}
return 0;
}
Explanation of Code
In this example, we define three threads that print numbers. The `mtx.lock()` call ensures only one thread can execute the critical section (the printing operation) at a time. When a thread finishes printing, it releases the mutex with `mtx.unlock()`. This pattern protects the shared resource (standard output) from being accessed by multiple threads simultaneously.
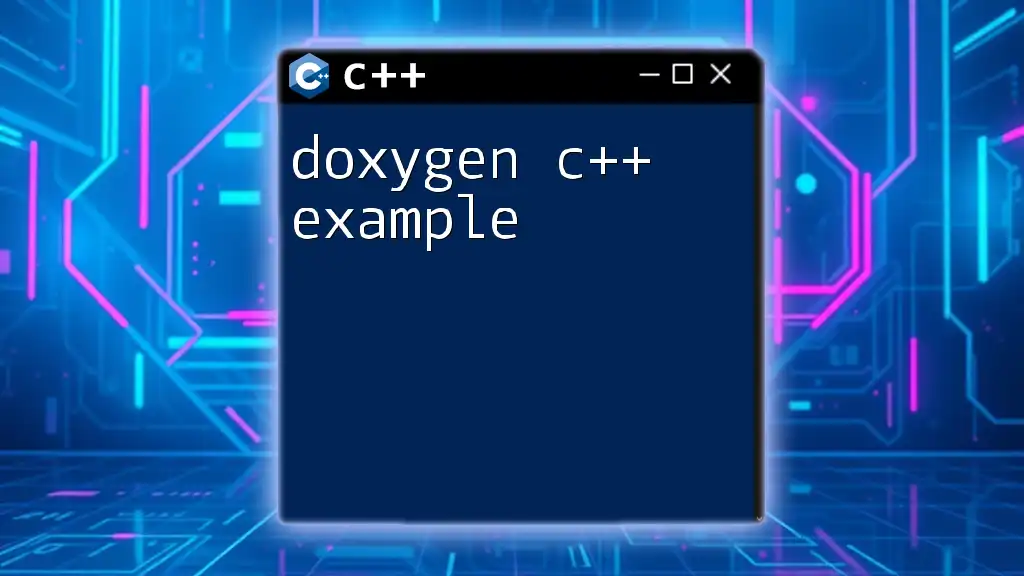
Advanced Mutex Example
Using `std::lock_guard`
As your application grows, managing locks and unlocks manually can introduce bugs, especially if exceptions occur. A better approach is to use `std::lock_guard`, a convenient RAII class that automatically locks the mutex when created and unlocks it when it goes out of scope.
Example Code Snippet
Here’s an improved version of our previous example using `std::lock_guard`:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void print_numbers(int id) {
std::lock_guard<std::mutex> lock(mtx); // Automatically locks the mutex
std::cout << "Thread " << id << ": ";
for (int i = 0; i < 5; ++i) {
std::cout << i << " ";
}
std::cout << std::endl;
}
int main() {
std::thread threads[3];
for (int i = 0; i < 3; ++i) {
threads[i] = std::thread(print_numbers, i);
}
for (int i = 0; i < 3; ++i) {
threads[i].join(); // Wait for all threads to finish
}
return 0;
}
Explanation of Code
In this revised example, the `std::lock_guard` manages the mutex automatically. Upon entering the `print_numbers` function, the mutex is locked, and as soon as the function exits—whether normally or through an exception—the mutex is released. This approach significantly reduces error chances by preventing deadlocks that may arise from forgetting to unlock a mutex.
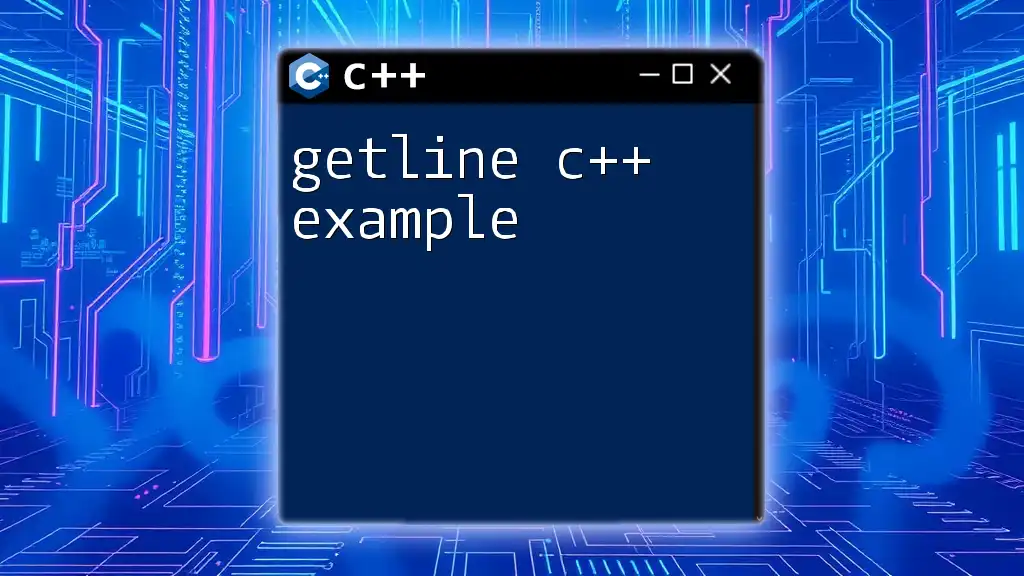
Best Practices for Using Mutexes
To ensure efficient and effective use of mutexes in C++, consider the following best practices:
- Minimize Lock Duration: Keep the code within a lock to a minimum. This reduces the time a mutex is held, allowing other threads to access shared resources sooner.
- Avoid Nested Locks: Nested locking can lead to deadlocks. If multiple threads hold locks in a different order, they can end up waiting on each other indefinitely.
- Lock on the Smallest Scope: Lock only the sections of code that need protection. The more you can restrict the use of locks, the better your application will perform.
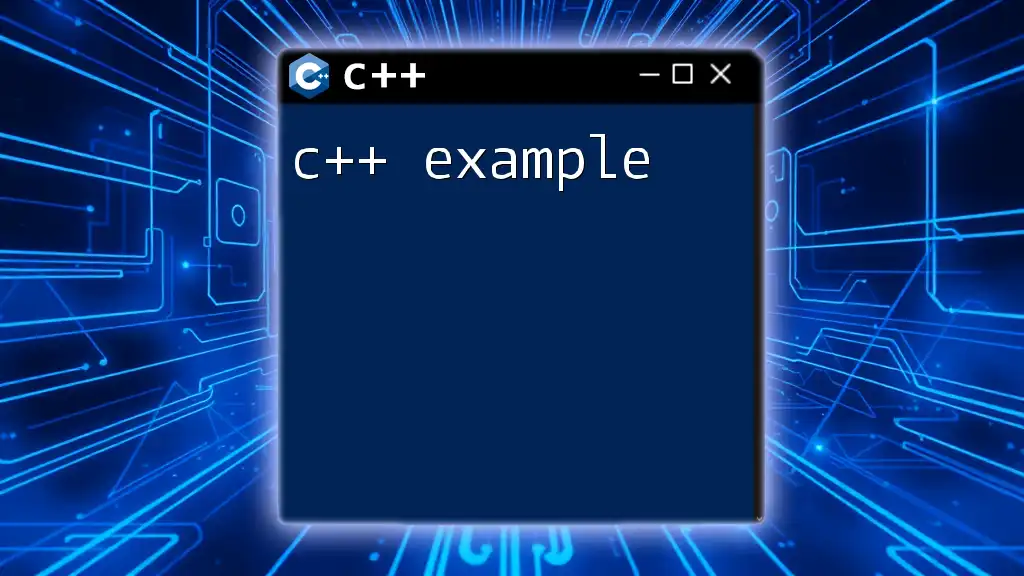
Common Issues with Mutex
Despite their advantages, mutexes can introduce complications if not managed correctly:
- Deadlocks: This occurs when two or more threads are waiting for each other to release the mutexes they hold. It can happen if you lock mutexes in different orders.
- Performance Bottlenecks: Frequent or long-held locks can degrade performance, especially in high-contention scenarios where many threads are competing for the same resources.
- Priority Inversion: A lower-priority thread holding a mutex may block a higher-priority thread, leading to unacceptable latencies.
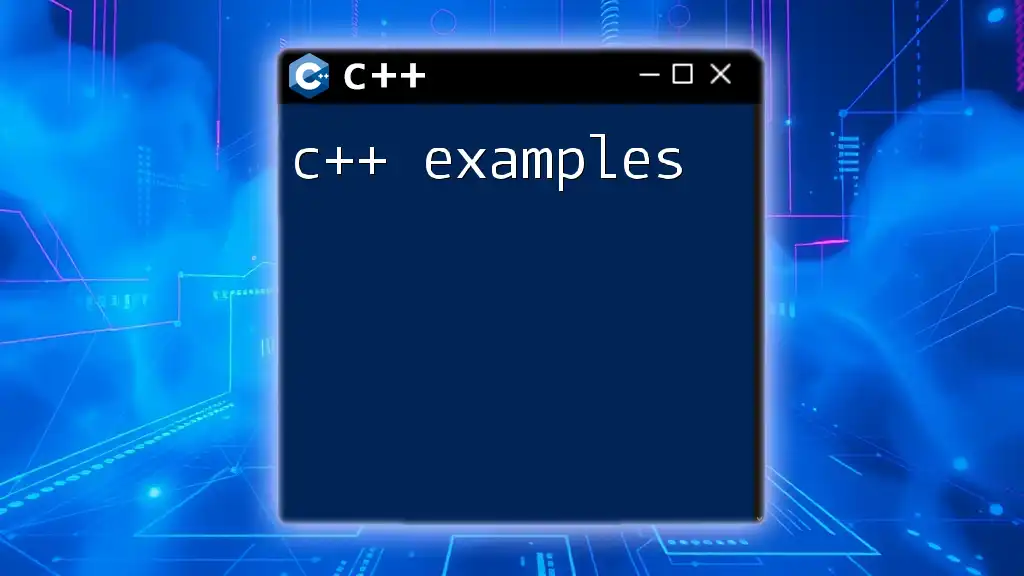
Conclusion
Mutexes are an essential tool in C++ for ensuring thread safety and managing access to shared resources. They protect against race conditions and maintain data integrity when multiple threads are involved.
By using `std::lock_guard`, you can simplify mutex management and reduce the risk of errors in your code. As you explore multithreading in C++, integrating these practices will help you create more robust and efficient applications.
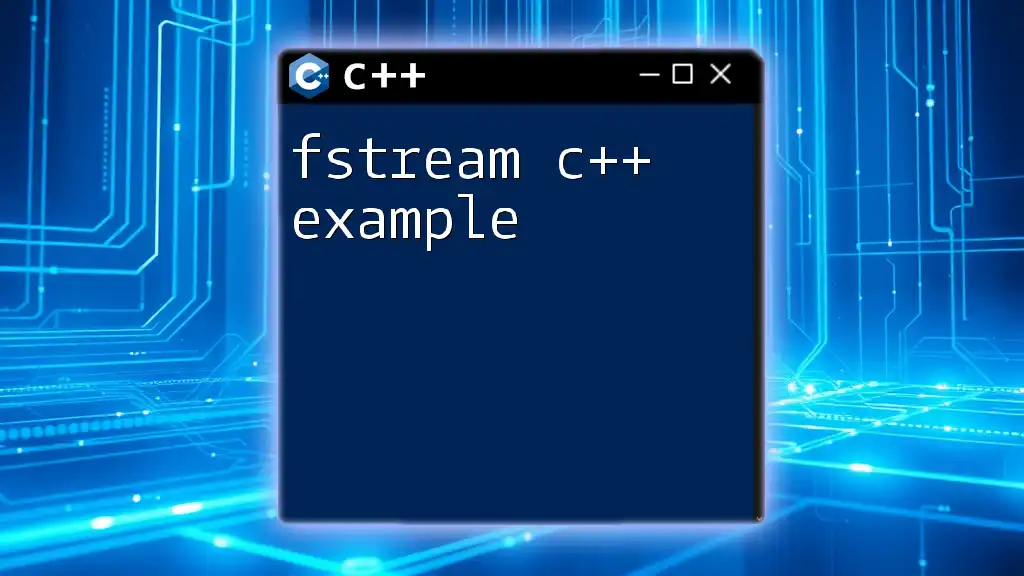
Additional Resources
For further learning on mutexes and multithreading in C++, consider the following resources:
- Visit the official [C++ documentation](http://en.cppreference.com/w/) for an in-depth look at threading capabilities.
- Explore books like "C++ Concurrency in Action" for comprehensive coverage of multithreaded programming.
- Join online communities such as Stack Overflow or Reddit's r/cpp for discussions and support from fellow developers.
With a solid understanding of mutexes, you’ll be well-equipped to tackle the complexities of multithreaded programming in C++.