In C++, a map is a collection of key-value pairs that allows for efficient data retrieval based on unique keys, as demonstrated in the following example:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << " is " << pair.second << " years old.\n";
}
return 0;
}
Understanding Maps in C++
What is a Map?
In C++, a map is a standard library container that stores key-value pairs where each key is unique. This data structure is part of the C++ Standard Template Library (STL) and allows for efficient retrieval, insertion, and deletion of data based on keys. Maps are particularly useful for associating one piece of data (the value) with another (the key) in a way that is easy to manage and access.
Characteristics of Maps
Maps have several unique characteristics:
-
Ordered vs. Unordered Maps: By default, a map maintains the order of keys. It is an ordered structure, meaning that the keys will be sorted in ascending order. If you need to work with unordered key-value pairs, you might consider using `unordered_map`, which offers average constant time complexity for search, insert, and delete operations.
-
Unique Keys: In a map, every key must be unique. If you try to insert a duplicate key, the existing value associated with that key will be overwritten.
-
Automatic Sorting: Maps automatically sort their keys based on the comparator used (by default, this is the `operator<`).
-
Complexity: The average time complexity for operations like insertion, deletion, and look-up in a map is \(O(\log n)\) due to the underlying balanced tree structure.
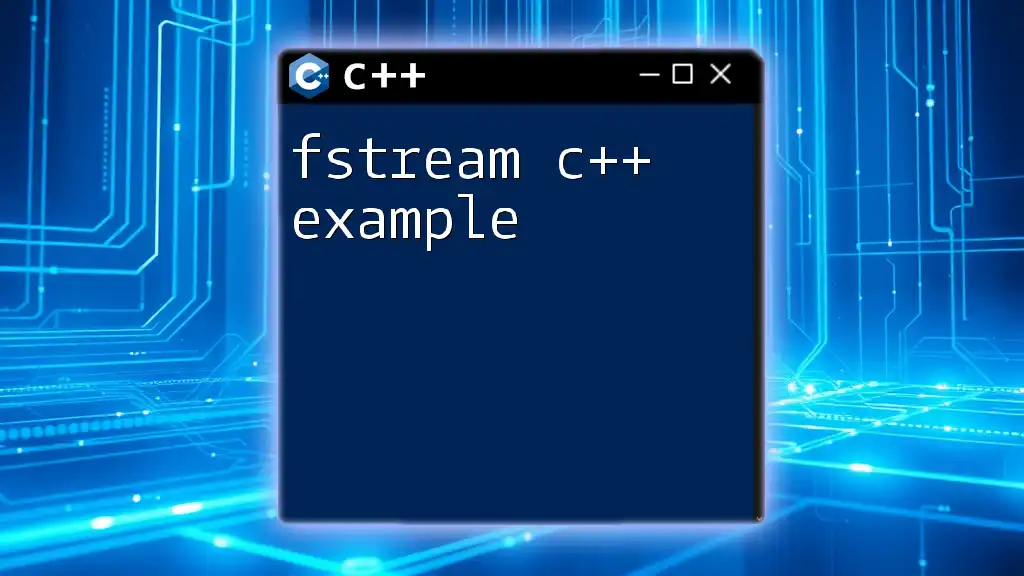
How to Use Map in C++
Including the Necessary Header
To use maps in your C++ program, ensure that you include the necessary header file:
#include <map>
Declaring a Map
Maps are declared using the following syntax:
std::map<KeyType, ValueType> mapName;
Example:
std::map<std::string, int> ageMap;
In this example, the keys are of type `std::string` (names), and the values are of type `int` (ages).
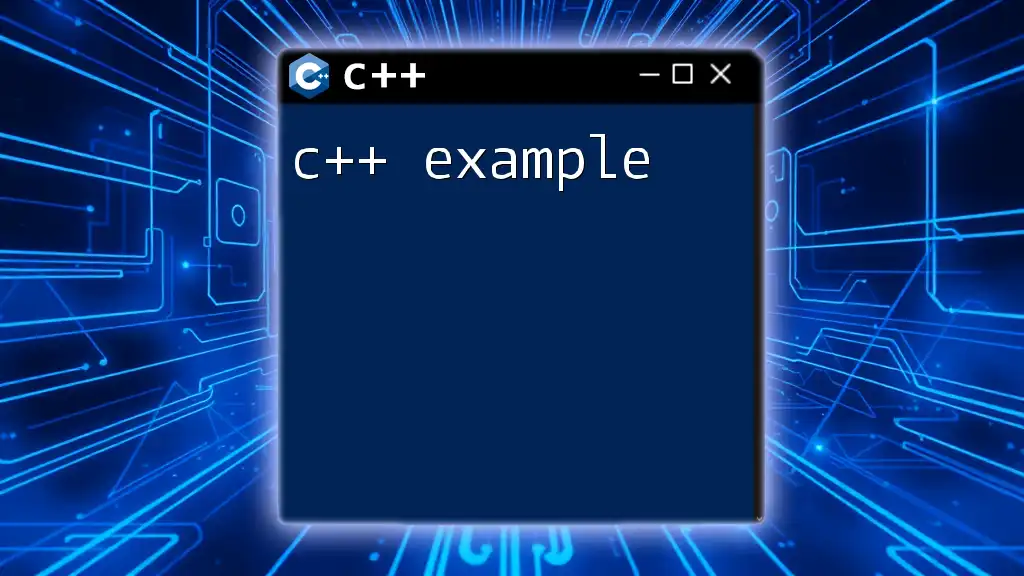
Example of Map in C++
Inserting Key-Value Pairs
The process of inserting key-value pairs into a map can be accomplished in multiple ways:
-
Using the `insert()` Method: This method adds a key-value pair to the map.
Example:
ageMap.insert({"Alice", 30});
-
Using the `[]` Operator: This operator allows you to either insert a new key-value pair or update an existing key.
Example:
ageMap["Bob"] = 25;
In both cases, if the key already exists, the associated value will be updated.
Accessing Values
To retrieve values from a map, you can use the key. The syntax is straightforward:
int alicesAge = ageMap["Alice"];
Important Note: If you try to access a value using a key that does not exist in the map, the `[]` operator will insert a new entry with the default value for the value type, which may not be the intended behavior. It's often safer to use the `.at()` method, which throws an exception if the key is not found:
try {
int age = ageMap.at("Charlie");
} catch (const std::out_of_range& e) {
std::cerr << "Key 'Charlie' not found!" << std::endl;
}
Iterating Over a Map
You can iterate through a map in various ways. The range-based for loop is one of the simplest methods, which allows you to access both the keys and the values directly:
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
Each iteration gives you a `pair`, where `pair.first` is the key and `pair.second` is the value.
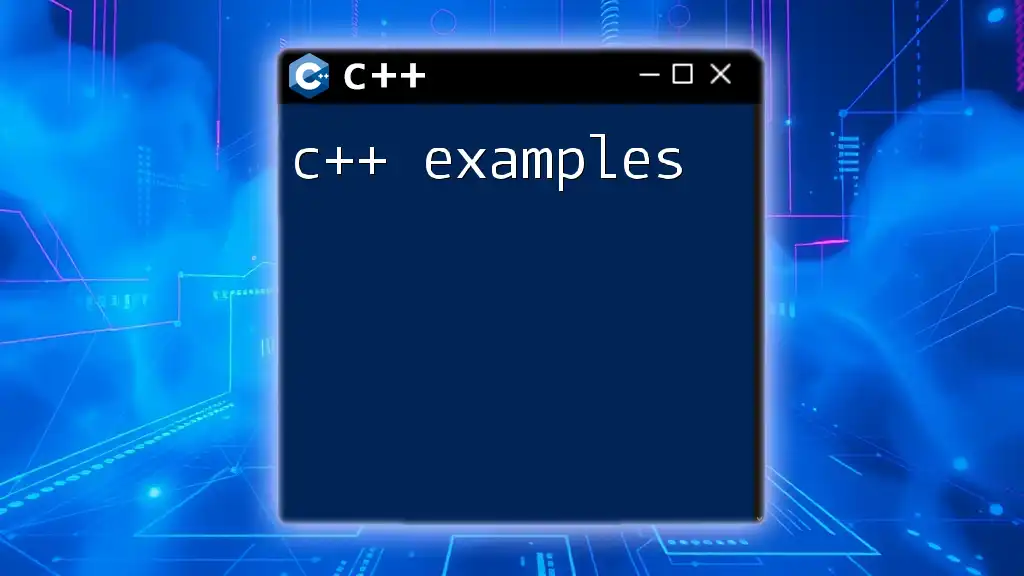
Advanced Usage of Map in C++
Using a Map with Custom Types
C++ allows you to utilize maps with user-defined types, making it a versatile container. Let’s say you have a `Person` structure:
struct Person {
std::string name;
int age;
};
std::map<int, Person> people;
In this case, the keys are integers (perhaps IDs), and the values are instances of the `Person` structure. This capability can help manage complex data more efficiently.
Sorting and Manipulating Maps
Sorting a map by its values rather than its keys can be achieved with a few extra steps. You would typically transfer the map elements to a vector and then sort that vector based on the values.
Example:
std::vector<std::pair<int, Person>> sortedPeople(people.begin(), people.end());
std::sort(sortedPeople.begin(), sortedPeople.end(), [](const auto& a, const auto& b) {
return a.second.age < b.second.age;
});
This code snippet creates a vector of pairs from the map and sorts it based on the age attribute of the `Person` structure.
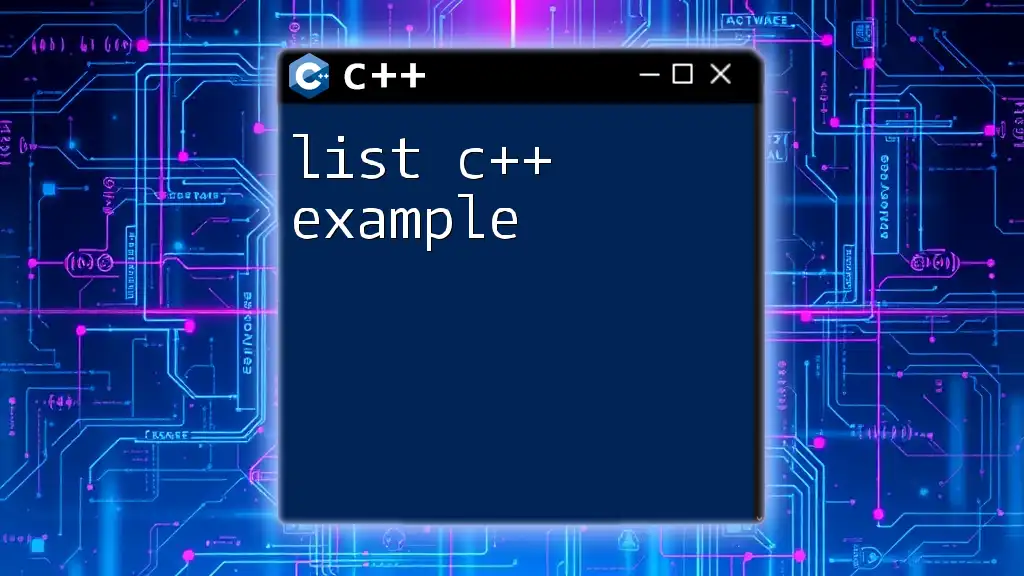
Example Map in C++: A Complete Application
In this section, we’ll develop a simple application that demonstrates the usage of maps. This program will create a map for looking up ages by name:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, int> ageMap;
// Inserting key-value pairs
ageMap.insert({"Alice", 30});
ageMap["Bob"] = 25;
// Accessing values
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl;
// Iterating through the map
std::cout << "All entries in ageMap:" << std::endl;
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
This program demonstrates inserting, accessing, and iterating over a map. The expected output will provide a clear representation of all entries in the `ageMap`.
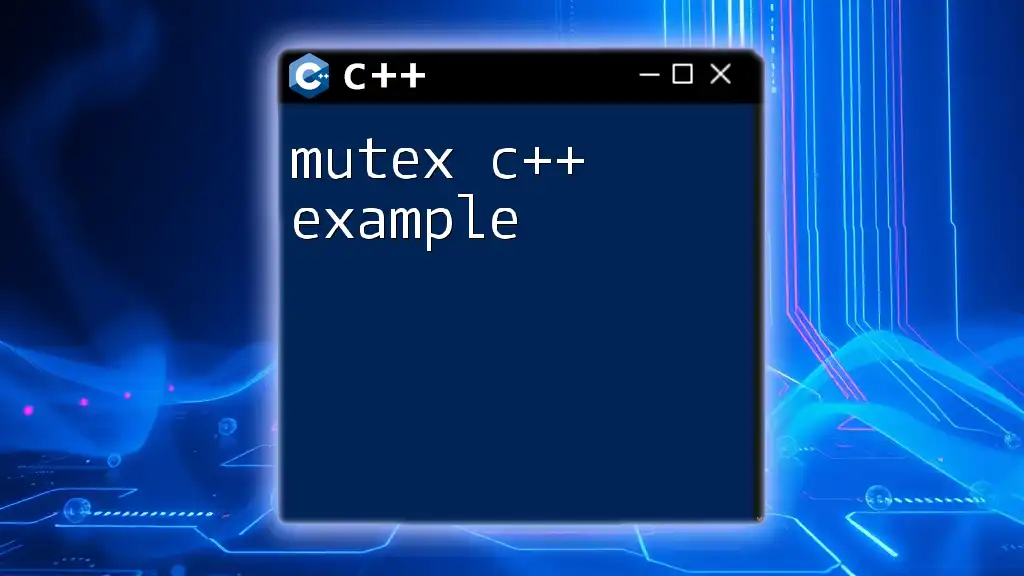
Common Mistakes When Using Maps
Forgetting to Include the Header
One common mistake new programmers make is forgetting to include the map header. Always ensure you include `#include <map>` to avoid compilation errors.
Using Non-Unique Keys
Another frequent error is attempting to insert a non-unique key. Remember that if you insert a duplicate key, the old value will be overwritten without any warning.
Error Handling in Accessing Non-Existent Keys
When accessing keys, be cautious about using the `[]` operator with keys that might not exist in the map. Instead, consider using the `.at()` method for safer key access, as it will throw an exception if the key is absent.
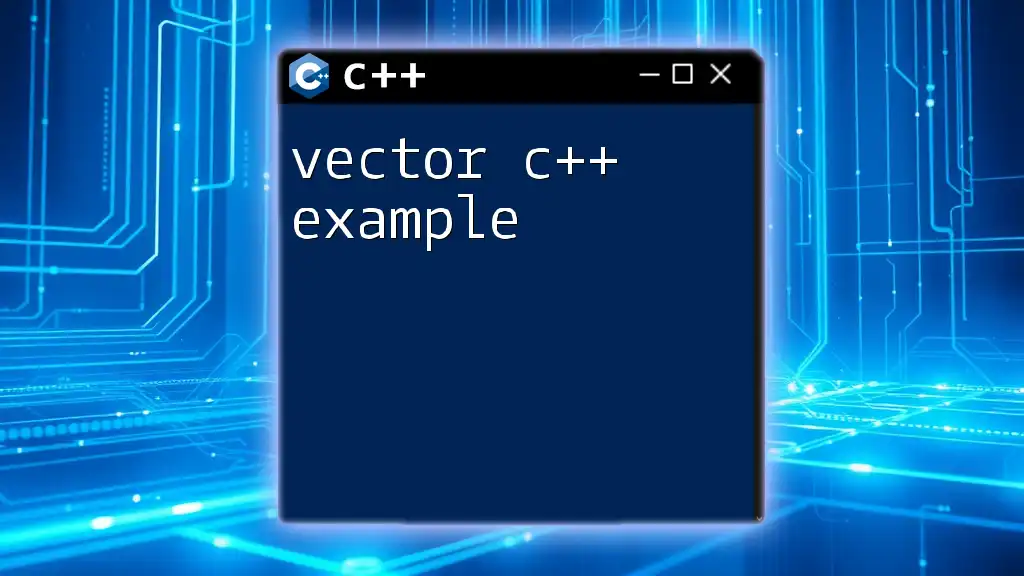
Conclusion
Throughout this article, we have explored the concept of maps in C++, from what a map is to its practical applications, including inserting, accessing, iterating, and working with custom types. Understanding and mastering maps can greatly enhance your ability to manage and retrieve data efficiently in your C++ applications. Practice these concepts in different scenarios to solidify your knowledge.
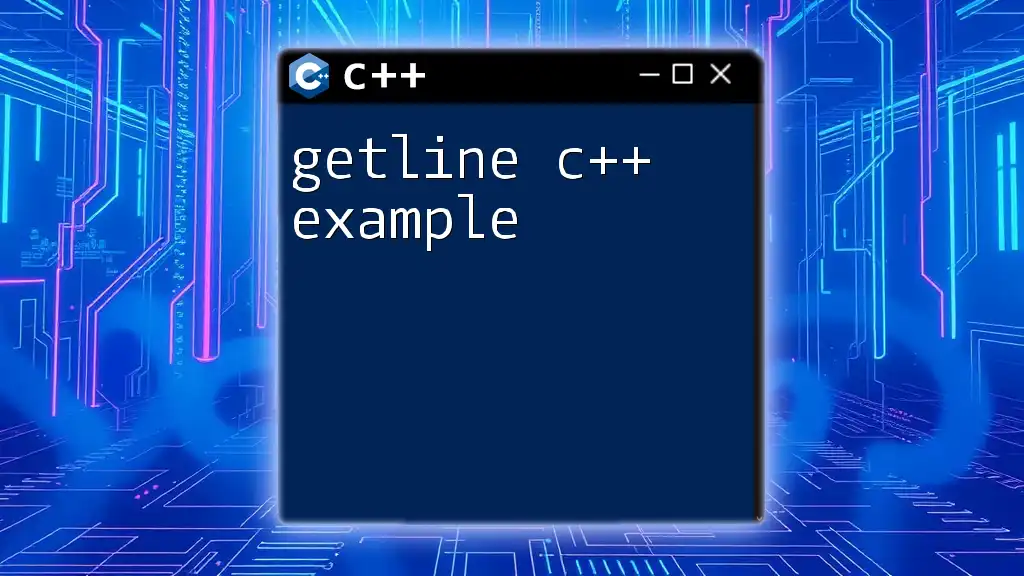
Call to Action
If you found this article helpful, consider subscribing for more C++ tutorials and tips! Share your experiences with maps in the comments, and let’s discuss how you can implement them in your projects.