C++ is a high-level programming language that combines features of both low-level and high-level programming, enabling developers to write efficient and effective software.
Here’s a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a high-level programming language, developed by Bjarne Stroustrup in the early 1980s. It is an extension of the C programming language, incorporating features that enable object-oriented programming (OOP). C++ has grown to be one of the most widely-used languages, known for its versatility and performance.
Key features that define C++ include:
- Object-oriented programming: C++ facilitates the use of classes and objects, which allows developers to model real-world entities and their interactions efficiently.
- Low-level memory manipulation: C++ provides functionalities for direct memory address manipulation, making it suitable for system programming.
- Performance and efficiency: It allows for both high-level abstractions and low-level operations, giving developers fine control over resource usage.

Why Learn C++?
Learning C++ is beneficial for several reasons. First, it is a versatile language utilized across various application domains, including system software, game development, embedded systems, and even high-performance applications like graphics engines.
When compared to other programming languages, C++ has distinct advantages:
- Performance: C++ typically offers faster execution times than languages like Python or Java due to its compiled nature and lower-level operations.
- Control: Developers have more control over system resources and memory, allowing for optimization that can drastically enhance performance.

Key Concepts of C++
Understanding C++ requires a familiarity with its syntax and core concepts.
Syntax Overview
C++ syntax is similar to that of C and is fairly intuitive for those who have experience with programming. A basic structure usually includes defining a `main` function, which is the entry point for execution.
For example, creating a simple variable:
int main() {
int number = 10;
return 0;
}
In this snippet, we declare an integer variable called `number` and assign it a value of 10.
Object-Oriented Programming in C++
Object-oriented programming is a fundamental concept in C++. It revolves around the use of classes and objects.
Classes and Objects
A class serves as a blueprint for creating objects. Objects are instances of classes, encapsulating properties (attributes) and behaviors (methods).
Here’s a straightforward example of defining a class and creating an object:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Outputs: Woof!
return 0;
}
In this code, we define a `Dog` class that has a `bark` method. When we create an object `myDog` of the class, we can call its `bark` method to produce output.
Inheritance and Polymorphism
C++ also supports inheritance, allowing a class to derive properties and behaviors from another class. This creates a hierarchical relationship. Polymorphism provides a way to call derived class methods through base class pointers or references.
For example, consider this code demonstrating inheritance:
class Animal {
public:
virtual void sound() {
std::cout << "Some generic sound" << std::endl;
}
};
class Cat : public Animal {
public:
void sound() override {
std::cout << "Meow!" << std::endl;
}
};
int main() {
Animal* myAnimal = new Cat();
myAnimal->sound(); // Outputs: Meow!
delete myAnimal;
return 0;
}
In this scenario, `Cat` inherits from `Animal`, and the `sound` method is overridden to provide specific behavior. This showcases the power of polymorphism, allowing us to use pointers of the base class type to call derived class methods.
Memory Management in C++
Memory management is a critical aspect of C++ programming, distinguished by its use of pointers and references.
Pointers and References
Pointers enable the manipulation of memory addresses directly, offering significant control over how memory is allocated and accessed. Here’s an example illustrating how pointers are used:
int main() {
int value = 42;
int* ptr = &value; // ptr holds the address of value
std::cout << *ptr; // Outputs: 42
return 0;
}
In this code, we declare an integer and create a pointer that points to its address. By dereferencing the pointer using `*`, we access the value it points to.
Control Structures in C++
Control structures in C++ are vital for directing the flow of execution within a program.
Conditionals
Conditionals such as `if-else` statements help manage decision-making in a program.
Loops
Loops allow repetitive execution of code blocks. Here’s a demonstration of a simple `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << i << " "; // Outputs: 0 1 2 3 4
}
This loop iterates from 0 to 4, printing each number.

Writing Your First C++ Program
Getting started with C++ requires setting up the appropriate development environment. Once configured, you can write your first program.
A classic beginner example is the "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` allows us to use the input and output stream. The `main` function executes and prints "Hello, World!" to the console.

Common Misconceptions About C++
There are numerous misconceptions surrounding C++.
- C++ is only for systems programming: While it excels in systems programming, its utility spans across different domains, including game and application development.
- C++ is too complex for beginners: While its rich feature set may initially seem daunting, many beginners find it manageable with practice and guidance.
- C++ and C are the same: While C++ is derived from C, they have significant differences, particularly in how they handle abstraction and object-oriented paradigms.

Resources for Learning C++
To further your understanding of C++, numerous resources are available:
Online Courses
There are several platforms that offer comprehensive C++ courses, such as Coursera, Udacity, and edX. They cater to learners of all levels, from beginners to advanced programmers.
Books
Some of the recommended readings include "C++ Primer" by Stanley B. Lippman and "Effective C++" by Scott Meyers. These texts provide in-depth knowledge and best practices in C++ programming.
Community and Forums
Engaging with the community can significantly enhance your learning journey. Websites like Stack Overflow, Reddit, or dedicated C++ forums are great places to ask questions, share knowledge, and find helpful resources.
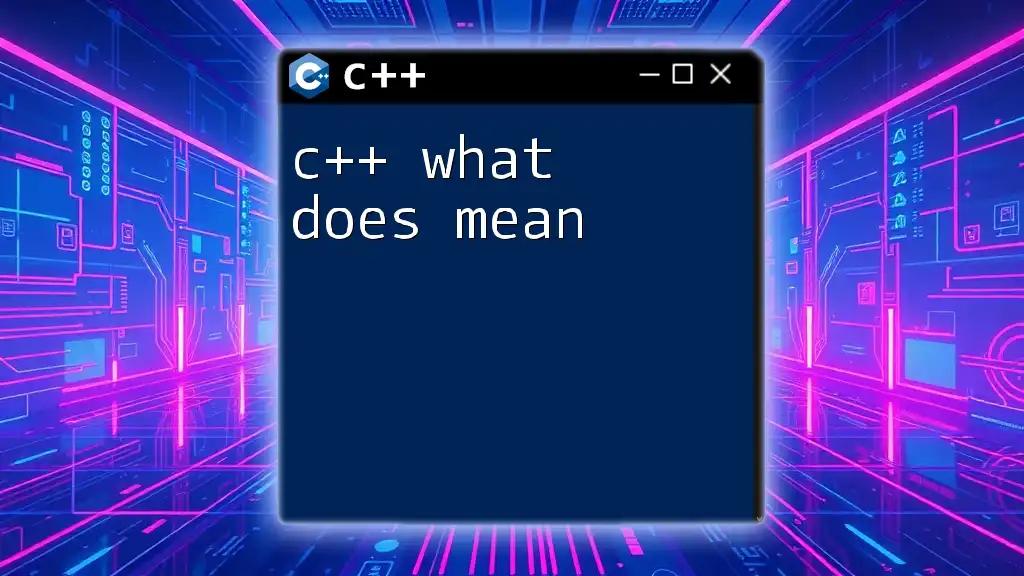
Conclusion
In summary, C++ is a powerful and versatile programming language that embodies essential computing concepts and practices. The journey of learning C++ can be both rewarding and enjoyable. With its combination of performance, control, and abstraction capabilities, C++ remains relevant in various fields of software development. Starting to learn C++ now will put you on a path to mastering one of the most sought-after skills in the tech industry.