In C++, a `float` is a data type that allows you to store single-precision floating-point numbers, which are useful for representing decimal values.
float myNumber = 3.14f; // Declares a float variable and initializes it with a value
What is a Float in C++?
Definition of Float
In C++, a float is a data type that represents a floating-point number, commonly referred to as a decimal number. It allows the storage of numeric values that have a fractional component. For example, when you think of calculations involving real-world measurements like weight or temperature, the float type becomes essential.
Floats are particularly useful when you need to express numbers that can have decimals, distinguishing them from integer types, which can only represent whole numbers.
Characteristics of Float
Floats in C++ are typically represented in single precision, which means they occupy 4 bytes in memory. They can represent values ranging from approximately 1.5 × 10^−45 to 3.4 × 10^38. However, they come with a trade-off in precision: they can accurately represent about 6 to 7 decimal digits.
It's essential to differentiate between single precision (float) and double precision (double). A double can store much larger ranges and provides greater precision, using 8 bytes in memory.
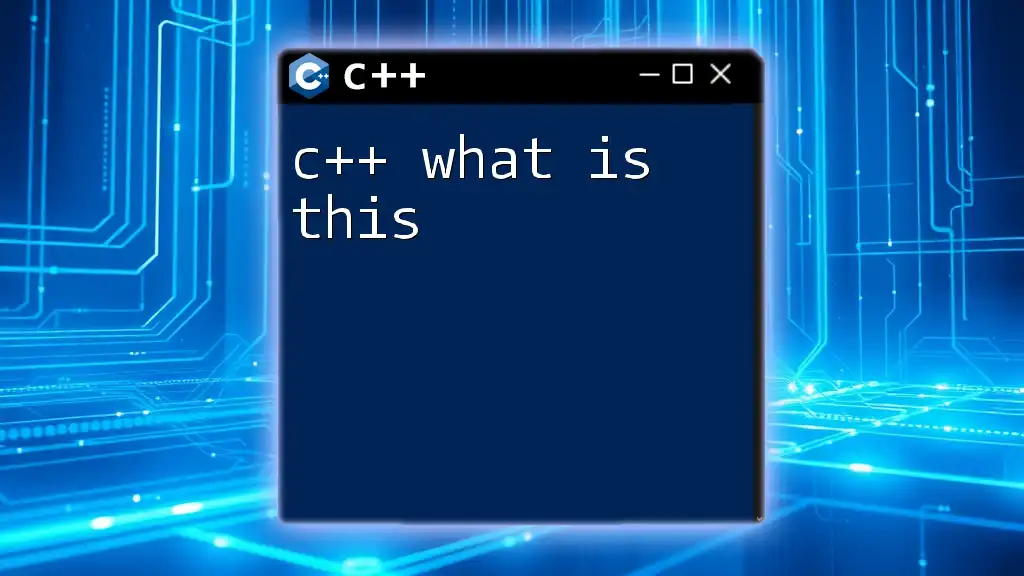
Why Use Float in C++?
Applications of Float
The float type is integral in numerous applications, including:
- Scientific Calculations: Many scientific disciplines require precise measurements that often involve fractions, such as physics and chemistry.
- Graphics Programming: In computer graphics, floats are extensively used for various calculations, including rendering images and representing coordinates.
Real-world examples include calculating gravitational forces, determining distances between graphical objects, or simulating physics in games.
Advantages of Using Float
The primary advantages of using float over integers are:
- Flexibility in calculations: Floats can represent a broader range of numbers, including fractions, which is crucial for accurate calculations.
- Performance: In scenarios involving a mix of integer and floating-point calculations, using floats can lead to more efficient algorithms, particularly when precision is less crucial.
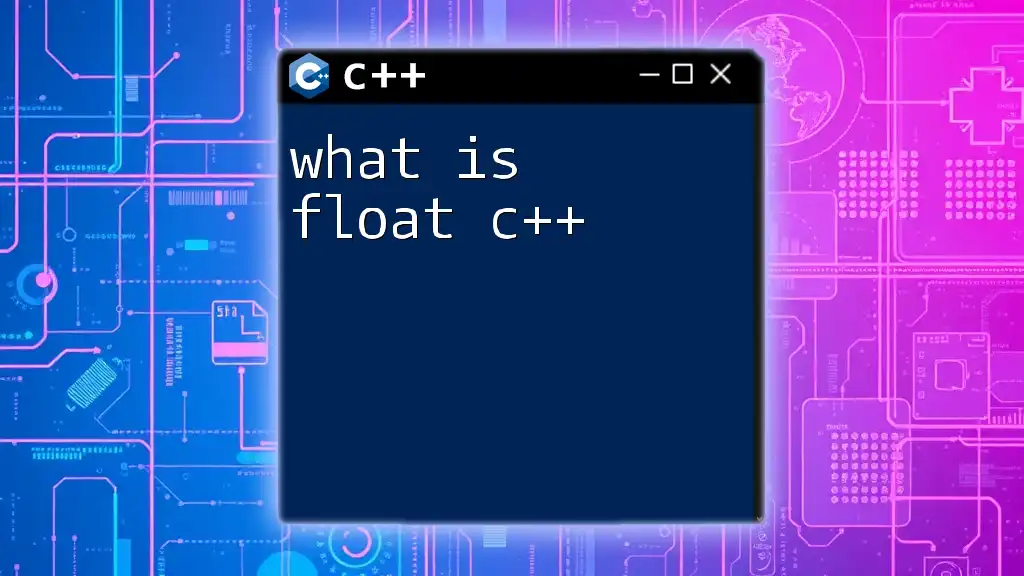
Declaring and Initializing Float Variables
Syntax of Float Declaration
Declaring a float variable in C++ is straightforward and uses the `float` keyword followed by the variable name. Here’s the basic syntax for declaring a float variable:
float variableName;
Initializing Float Variables
You can initialize float variables in different ways—through direct initialization or by using a constructor. Here are examples of both:
Direct Initialization
float pi = 3.14f;
Constructor Initialization
float gravity(9.81f);
Alternatively, you can also initialize a float variable as follows:
float temperature = 25.0f; // Direct initialization with decimal
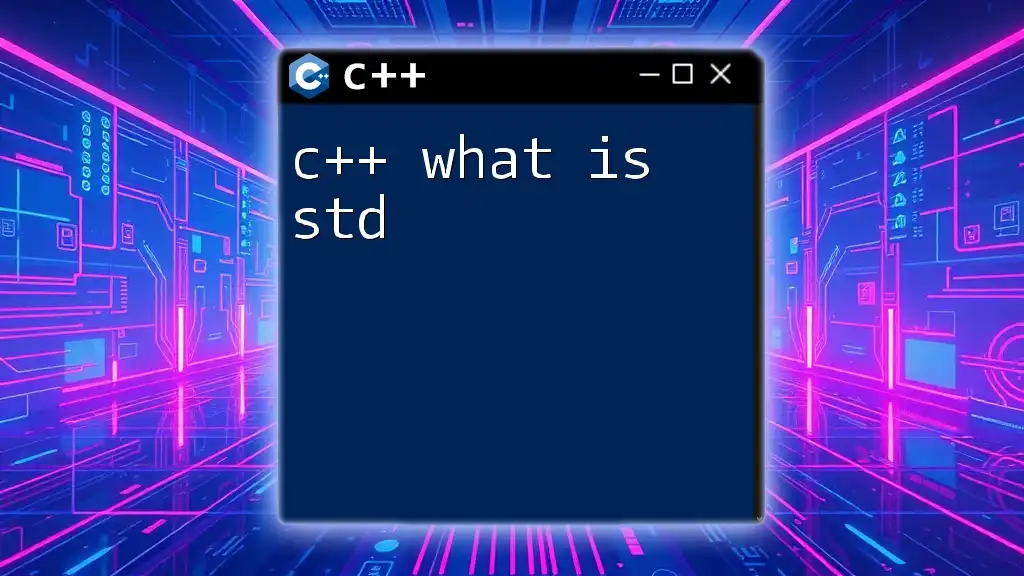
Operations with Float in C++
Arithmetic Operations
Floats support all standard arithmetic operations, including addition, subtraction, multiplication, and division. Here are simple examples demonstrating each operation:
Addition
float a = 5.5f;
float b = 2.2f;
float sum = a + b; // Result: 7.7
Subtraction
float difference = a - b; // Result: 3.3
Multiplication
float product = a * b; // Result: 12.1
Division
float quotient = a / b; // Result: 2.5
Comparison of Float Values
Comparing float values involves using comparison operators. However, due to precision limitations, be cautious with direct comparisons. Here’s how you can compare two float values:
if (a > b) {
// logic when a is greater than b
}
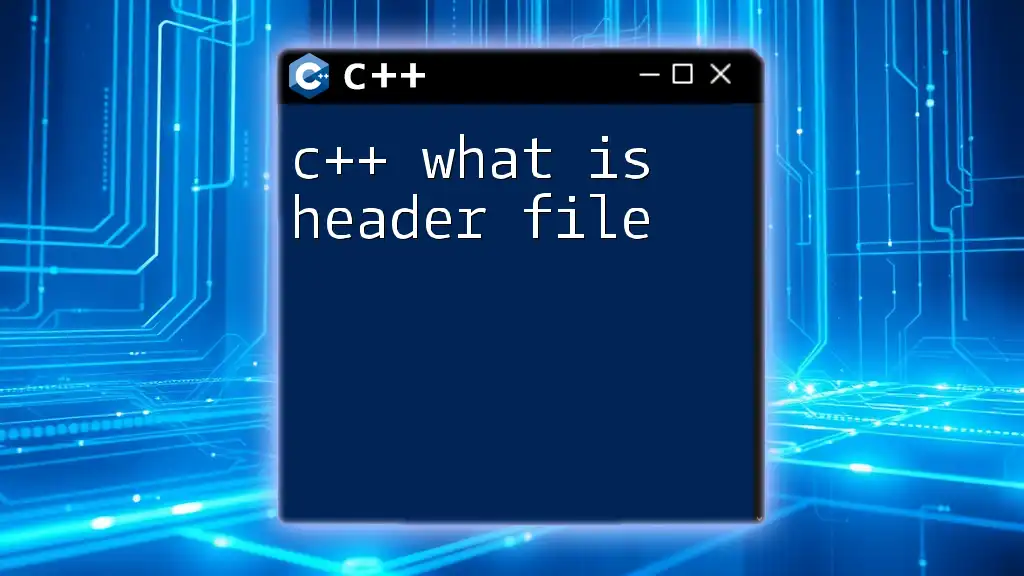
Common Functions Used with Float
Standard Library Functions
C++ provides various mathematical functions that work seamlessly with floats. A few essential functions include `sin()`, `cos()`, and `pow()`. Here’s an example of using the sine function:
#include <cmath>
float pi = 3.14159265f;
float sinValue = sin(pi / 2); // Result: 1.0
Type Conversion Functions
Sometimes you may need to convert a float to an integer (or vice versa). This is where type casting comes in handy. Here’s an example of converting a float to an integer using `static_cast`:
float pi = 3.14f;
int intValue = static_cast<int>(pi); // Converts float to int, Result: 3
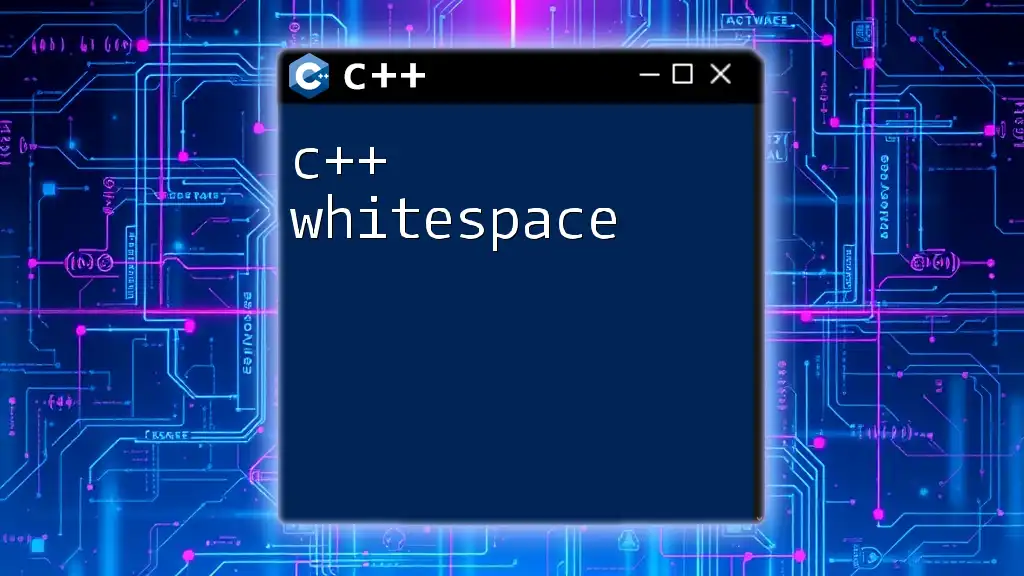
Precision and Rounding Issues with Float
Understanding Precision Issues
Despite their advantages, floats can exhibit precision issues due to their representation in memory. For example, adding `0.1f` and `0.2f` could yield a value that is not precisely equal to `0.3f` due to how floating-point arithmetic works:
float result = 0.1f + 0.2f; // This may not exactly equal 0.3
Solutions for Managing Precision
To address precision errors often associated with floats, consider using `double` for calculations requiring higher precision. Alternatively, you can utilize rounding functions to format the output. For instance:
#include <iomanip>
std::cout << std::fixed << std::setprecision(2) << result; // Rounds to two decimal places
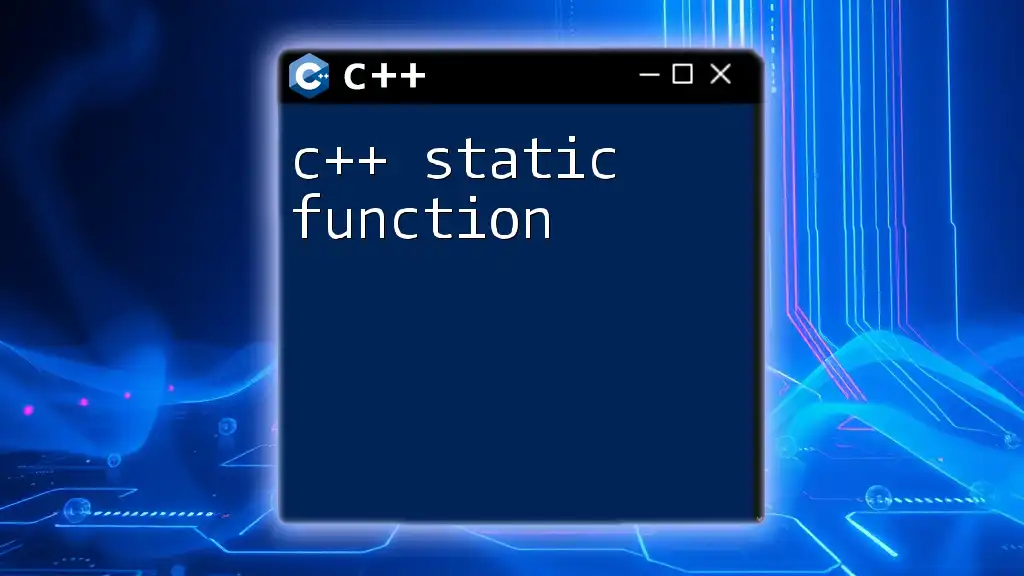
Conclusion
In summary, C++ floats are a crucial data type for handling decimal numbers in programming. They offer flexibility and the ability to perform a range of mathematical operations crucial for various applications. While using floats, keep in mind their limitations regarding precision, and consider best practices to mitigate any potential issues.
By understanding the fundamental aspects of C++ what is float, you're now better equipped to use this essential data type effectively in your programming projects.
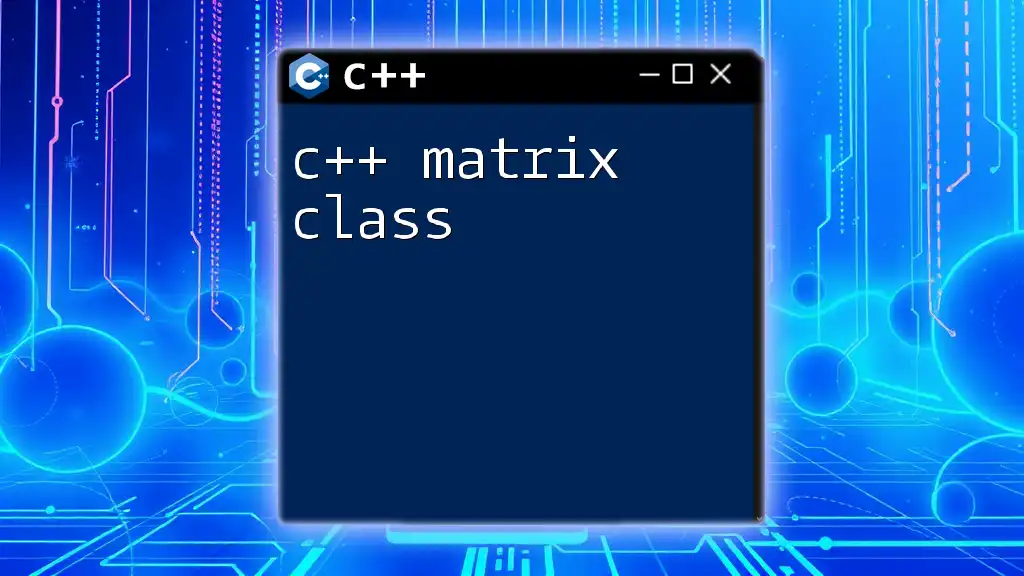
Additional Resources
Books and Tutorials
For further exploration of floats in C++, consider reputable books or online resources that delve deeper into the fundamentals of C++ programming.
Online Courses
Enrolling in courses specifically focused on C++ can also provide practical insights into working with various data types, including floats.
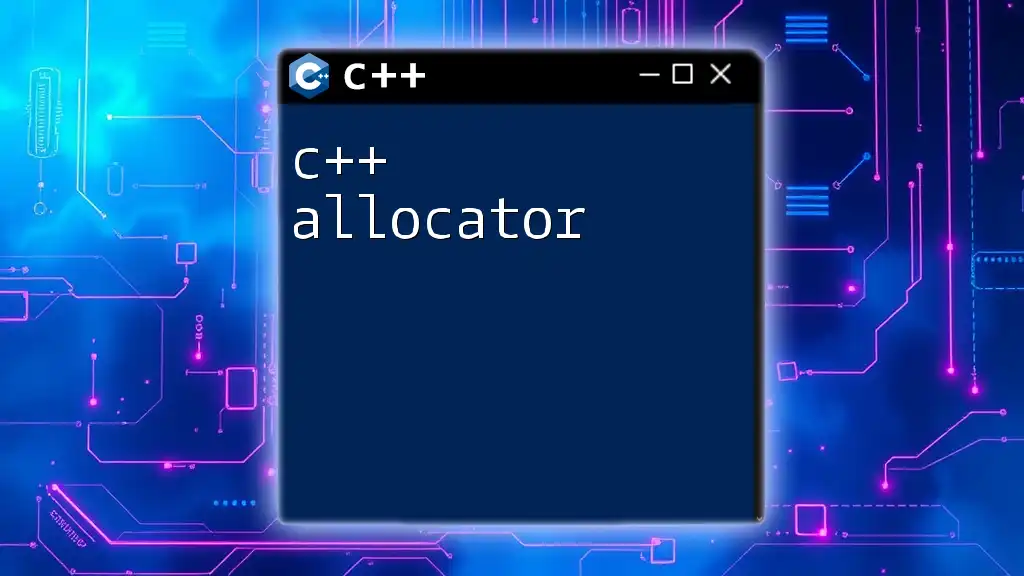
FAQs
What is the difference between float and double in C++?
The primary distinction between `float` and `double` lies in their precision and storage: `float` is single precision (4 bytes) offering limited accuracy, while `double` provides double precision (8 bytes) allowing for larger values and more accurate calculations.
Can float values be negative in C++?
Yes, float values in C++ can indeed be negative, just like integers. They can represent both positive and negative values, making them versatile for various mathematical scenarios.