The Microsoft C++ Visual Runtime is a set of libraries that provide essential functionality for C++ applications, ensuring they run smoothly on Windows systems.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Microsoft C++ Visual Runtime
What is Microsoft C++ Visual Runtime?
Microsoft C++ Visual Runtime, often referred to as the MSVC runtime, is a collection of dynamic link libraries (DLLs) necessary for running applications developed with Microsoft Visual C++. These libraries include essential components that provide runtime support for C++ applications, such as memory management, exceptions handling, file input/output, and much more.
Importance of Microsoft C++ Visual Runtime
Understanding the importance of the MSVC runtime is crucial for developers. It ensures that applications developed in C++ can execute without needing to compile code every time it runs. The runtime environment abstracts many complex processes, allowing developers to focus on their application's functionality rather than the underlying system intricacies.
Common use cases for the MSVC runtime include:
- Running video games created with C++ using Microsoft development tools.
- Executing productivity software like Microsoft Office that rely on C++ libraries.
- Supporting any third-party applications developed in C++.
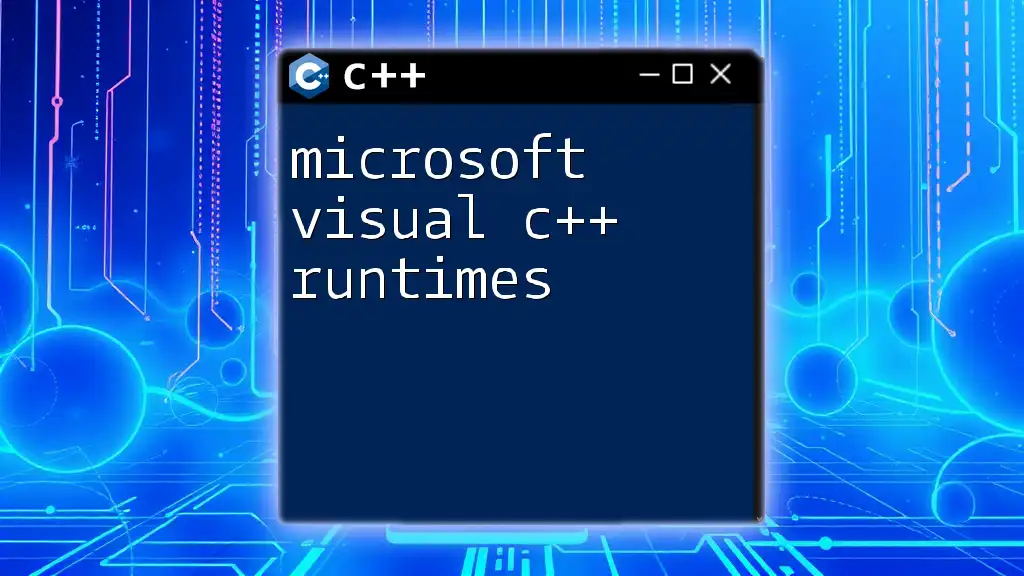
Installation of Microsoft C++ Visual Runtime
System Requirements
Before installing the Microsoft C++ Visual Runtime, it is essential to meet specific hardware and software requirements:
- Operating Systems: Compatible with Windows 7, Windows 8, 10, and later versions.
- Architecture: Both 32-bit and 64-bit versions are available, so ensure to select based on the OS architecture.
Steps to Install the C++ Runtime
To install the MSVC runtime:
- Visit the official Microsoft website to download the Visual C++ Redistributable packages.
- Select the appropriate version based on your application's requirements.
- Click on the download link and run the installer.
- Follow the installation wizard prompts until the installation completes.
Verifying Installation
To confirm that the Microsoft C++ Visual Runtime is correctly installed:
- Open the Control Panel, navigate to Programs and Features, and look for entries titled "Microsoft Visual C++ Redistributable" followed by the version number. This confirms successful installation.
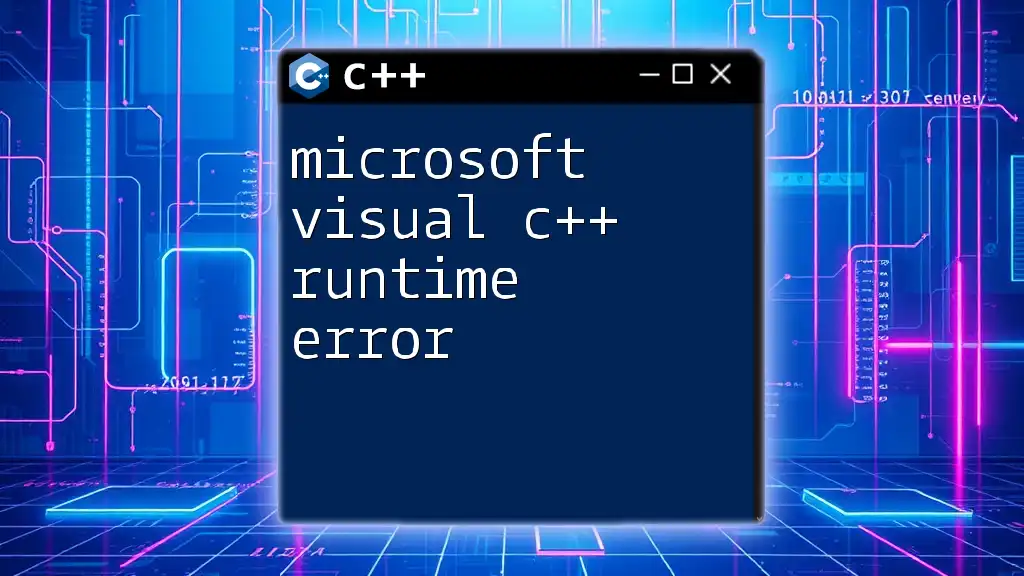
Components of Microsoft C++ Visual Runtime
Key Components Explained
The MSVC runtime comprises several key components that serve distinct functionalities in application development:
-
CRT (C Runtime Library): This library provides fundamental routines that allow C and C++ programs to perform basic functions, such as memory allocation, string manipulation, and math operations. For example:
#include <cstdlib> int main() { int* arr = (int*)malloc(10 * sizeof(int)); // allocates memory // Remember to free allocated memory free(arr); return 0; }
-
C++ Standard Library: This library includes powerful tools and functions that facilitate complex data handling such as containers (like vectors, lists), algorithms (sort, search), and streams for input/output operations, enhancing developer efficiency.
-
MFC (Microsoft Foundation Classes): MFC is a set of C++ classes designed to create Windows applications. Utilizing MFC simplifies the process of integrating with Windows API and GUI development.
-
ATL (Active Template Library): ATL offers a framework for creating lightweight COM (Component Object Model) components. It helps in developing add-ins and other custom components which can interface with Microsoft applications or other third-party software.
Version Differences
The evolution of the Microsoft C++ Visual Runtime has led to significant changes over the years. For instance, the shift from Visual Studio 2015 to Visual Studio 2019 saw improvements in performance, additional features, and better support for modern C++ standards. Awareness of these differences is essential for developers to ensure compatibility with existing projects.
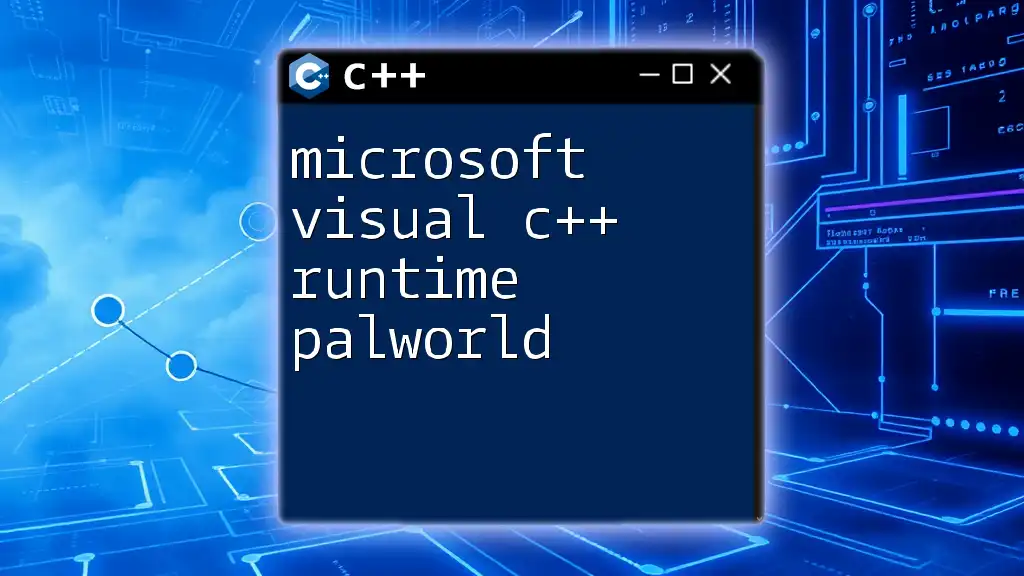
Common Issues with Microsoft C++ Visual Runtime
Runtime Errors
While using Microsoft C++ Visual Runtime, developers often encounter common runtime errors:
-
“xxx.dll not found”: This error typically indicates that an application is missing a required DLL file. A solution involves reinstalling the respective Visual C++ Redistributable package.
-
“R6025: pure virtual function call”: This error arises when a pure virtual function is called but has not been properly implemented. Careful code checks and implementing all pure virtual functions can resolve this issue.
Incompatibility Problems
Incompatibility between different versions of the MSVC runtime can cause applications to fail. To manage this:
- Ensure that all necessary redistributables are installed. When distributing applications, include the appropriate runtime versions.
- Utilize manifest files to specify the required versions of the runtime libraries for your application.
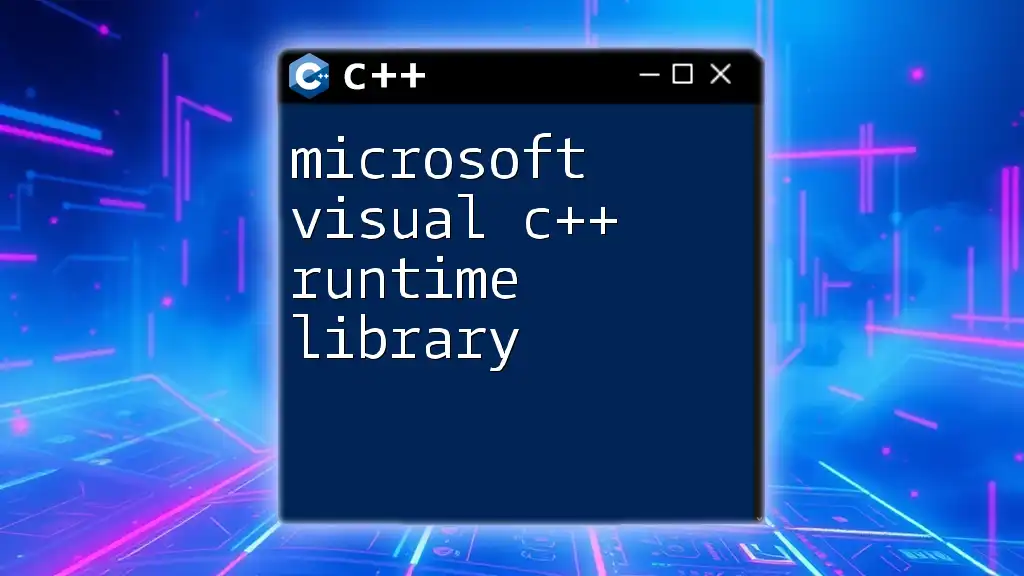
Updating Microsoft C++ Visual Runtime
Why Update?
Keeping the Microsoft C++ Visual Runtime up to date is pivotal. Regular updates patch security vulnerabilities, improve stability, and enhance performance—thereby ensuring that applications function seamlessly in newer operating systems and environments.
How to Update
To update your Microsoft C++ Visual Runtime:
- Within the Control Panel, check under Programs and Features to see which versions are installed.
- Visit Microsoft's official download page for Visual C++ Redistributable packages to manually download and install the latest versions.
- Automated updates via Windows Update can also provide the latest improvements, so ensure your system settings allow for such updates.
Handling Multiple Versions on a System
Sometimes, applications require specific versions of the MSVC runtime. To manage various installations:
- Install the necessary versions as different applications require, as modern Windows allows multiple redistributables to coexist without issues.
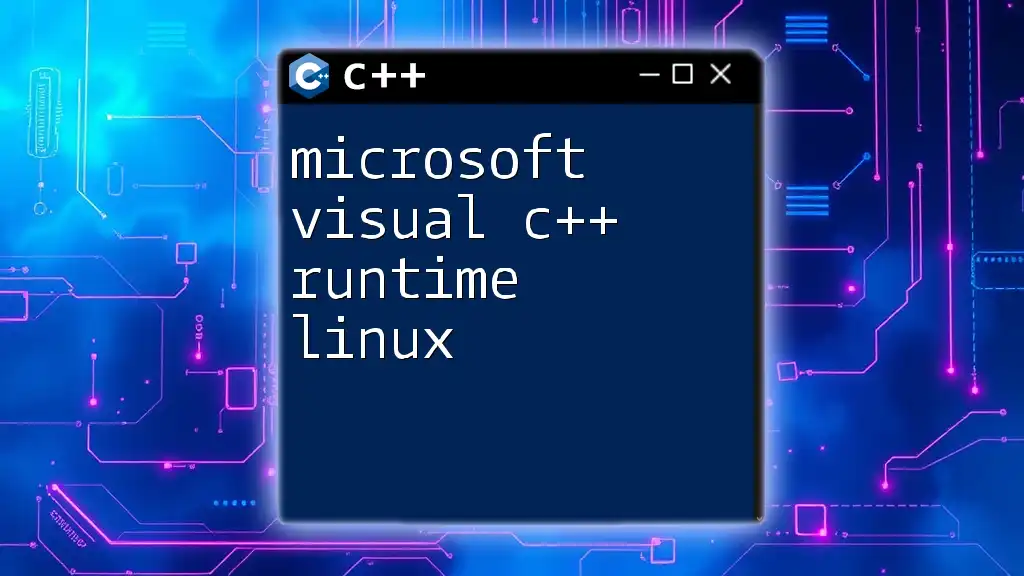
Advanced Topics
Debugging with Microsoft C++ Visual Runtime
Debugging is a crucial step in the development process. To effectively debug C++ applications using MSVC runtime:
- Utilize tools such as Visual Studio's built-in debugger, which provides insights into memory usage and points out runtime issues.
- Implement thorough logging practices to help identify where errors occur during runtime.
Performance Optimization
Optimizing C++ applications can lead to significant performance improvements. Consider the following:
- Use appropriate data structures from the C++ Standard Library, such as `std::vector` for dynamic arrays which manage memory allocation efficiently.
- Profile your application using tools provided in Visual Studio or third-party profilers to identify bottlenecks in code execution.
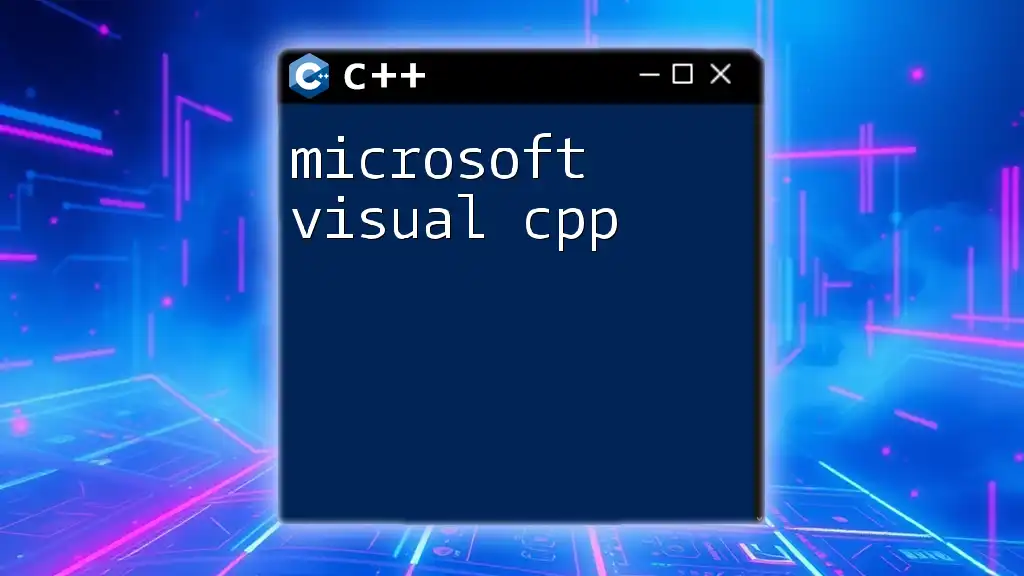
Conclusion
The Microsoft C++ Visual Runtime is indispensable for developers working with C++ applications on Windows. Its various components provide essential functionalities, while understanding how to install, update, and troubleshoot can significantly enhance development workflows. By staying informed about the runtime, developers can leverage its capabilities to create robust applications with fewer issues, ensuring a smooth user experience.
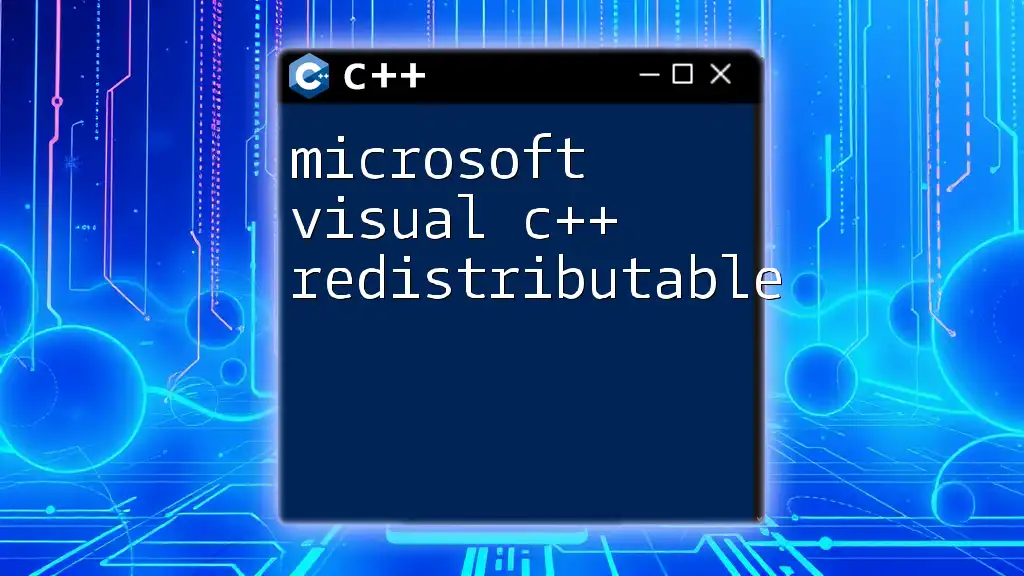
Additional Resources
For further exploration, be sure to check out the following valuable resources:
- Official Microsoft documentation on Visual C++ and runtime libraries.
- Community forums for peer support and knowledge sharing.
- Tutorials and courses on advanced C++ programming concepts and runtime management.
By mastering the Microsoft C++ Visual Runtime, you'll be better equipped to develop high-performance applications that stand out in today’s software landscape.