In C++, passing by pointer allows a function to directly modify the variable's value in the calling context by passing the address of the variable instead of its value.
Here's a simple code snippet demonstrating this concept:
#include <iostream>
using namespace std;
void increment(int* ptr) {
(*ptr)++;
}
int main() {
int num = 5;
increment(&num);
cout << "Incremented value: " << num << endl; // Output: Incremented value: 6
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer is a variable that stores the memory address of another variable. In C++, pointers are powerful tools that allow developers to reference and manipulate variables directly in memory.
Basic Syntax for Declaring Pointers:
To declare a pointer, use the following syntax:
int* ptr; // Declaration of a pointer to an integer
Here, `ptr` is a pointer that can hold the address of an integer variable.
The Role of Pointers in C++
Pointers play a crucial role in memory management and data manipulation. They allow you to achieve:
-
Dynamic Memory Allocation: You can allocate and deallocate memory at runtime, giving you control over memory usage.
-
Function Interactions: When passing parameters to functions, pointers enable modifications to the original variables without the overhead of copying data.

Passing by Value vs. Passing by Pointer
What is Passing by Value?
When you pass a variable to a function by value, a copy of the variable is created. Changes made to the parameter inside the function do not affect the original variable outside of it.
Example Code Snippet:
void passByValue(int num) {
num = 20; // changes are not reflected in the original argument
}
What is Passing by Pointer?
On the other hand, when you pass a variable by pointer, you pass the address of the variable, allowing the function to modify the original variable's value directly. This mechanism is powerful for situations where you need to change the value of the input variable.
Example Code Snippet:
void passByPointer(int* ptr) {
*ptr = 20; // changes are reflected in the original argument
}

How to Pass by Pointer in C++
Function Definition with Pointer Parameters
To define a function that accepts pointers as parameters, you need to specify the pointer type in the function signature. This indicates that the function will receive the address of the variable.
Example of Passing by Pointer in C++
Here’s a simple yet potent demonstration of passing by pointer in C++:
#include <iostream>
using namespace std;
void passByPointer(int* ptr) {
*ptr = 42; // Directly modify the value of the original variable
}
int main() {
int number = 10;
cout << "Before: " << number << endl; // Outputs: Before: 10
passByPointer(&number);
cout << "After: " << number << endl; // Outputs: After: 42
return 0;
}
In this example, the `passByPointer` function modifies the original variable `number` by dereferencing the pointer. The `&` operator in `main` retrieves the address of `number`.

Advantages of Passing by Pointer
Memory Efficiency
Passing by pointer is memory efficient since passing large structures or arrays by reference avoids overhead in copying data. Instead of duplicating large data structures in function calls, pointers allow for direct manipulation of data in its original location in memory.
Modifying Original Data
Another advantage of passing by pointer is that it allows functions to modify the original data. This is particularly useful in scenarios where you need to update multiple variables or return multiple results from a function without using out parameters.

Potential Pitfalls of Passing by Pointer
Pointer Dereferencing and Null Pointers
Dereferencing a pointer means accessing the value stored at the pointer’s address. If a pointer is uninitialized or set to null, dereferencing it will lead to undefined behavior and can cause your program to crash.
Example Code Snippet:
int* ptr = nullptr;
*ptr = 10; // Dereferencing a null pointer results in undefined behavior
Ensure that a pointer is valid before dereferencing it. Always initialize pointers and check for null before use.
Memory Leaks and Management
When utilizing dynamic memory allocation, it’s crucial to manage allocated memory properly. If you allocate memory using `new`, you must free that memory using `delete` once it’s no longer in use.
Example Code Snippet:
int* ptr = new int;
*ptr = 5;
// ... use pointer ...
delete ptr; // Always release dynamically allocated memory
Failure to release memory can lead to memory leaks, causing your program to consume increasing amounts of memory over time.

Best Practices for Passing by Pointer in C++
Consistency in Code
Maintaining a consistent coding style is essential when using pointers. Always use clear and descriptive names for pointer variables, making it easier for others (and yourself) to read and understand your code.
Use of Smart Pointers (C++11 and beyond)
C++11 introduced smart pointers, which help manage memory automatically. Smart pointers like `unique_ptr` and `shared_ptr` offer automatic destruction of dynamically allocated memory, significantly reducing the chances of memory leaks.
Example Code Snippet:
#include <memory>
void function(std::unique_ptr<int> ptr) {
// Function that uses smart pointers
}
Using smart pointers not only enhances memory safety but also promotes cleaner and more maintainable code.
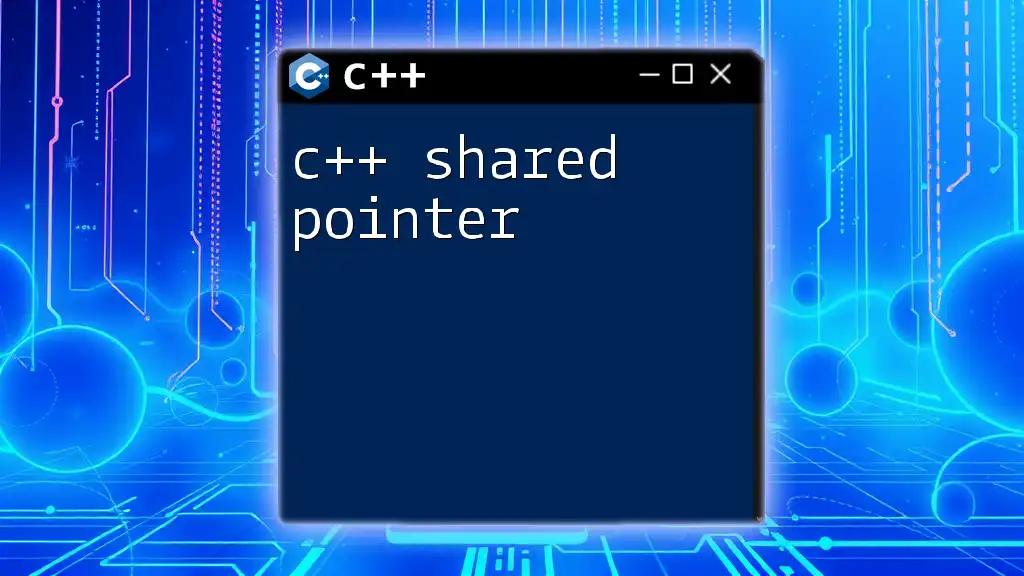
Conclusion
Understanding C++ passing by pointer is essential for efficient programming in C++. By mastering pointers, you can optimize memory usage, manipulate data directly, and implement powerful programming techniques. Embrace the complexities of pointers, as they open new horizons in your development journey. Remember to practice with hands-on examples, ensuring that you fully grasp the concepts discussed. For further enhancement of your knowledge, explore comprehensive resources dedicated to C++ pointers and memory management.