To iterate a vector in reverse in C++, you can use a reverse iterator or a traditional for loop with decrementing indices.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
// Using reverse iterator
for (auto it = vec.rbegin(); it != vec.rend(); ++it) {
std::cout << *it << ' ';
}
// Output: 5 4 3 2 1
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can grow and shrink in size as needed. It is part of the Standard Template Library (STL) and provides numerous advantages over traditional arrays, such as automatic memory management and built-in methods for various operations.
Basic Operations on Vectors
To leverage the full potential of vectors, it's crucial to understand some of their basic operations:
- Adding Elements: You can add elements using the `push_back` method, which appends an element to the end of the vector.
- Accessing Elements: Use the `operator[]` or the `at()` method to access elements. While `operator[]` does not perform bounds checking, `at()` does, making it a safer option.
- Removing Elements: The `pop_back()` method allows you to remove the last element from the vector.
Understanding these operations sets the stage for efficient manipulation, especially when it comes to iterating vectors in reverse.
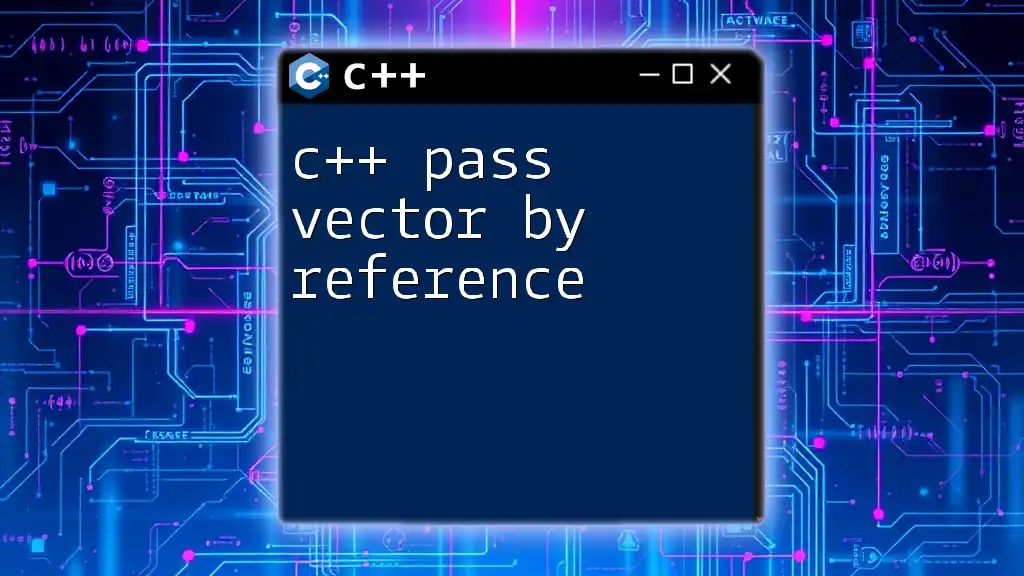
Methods to Iterate a Vector in Reverse
Using Reverse Iterators
Introduction to Iterators
Iterators are objects that allow you to traverse the elements of a container (like a vector) without exposing the underlying structure. Reverse iterators provide a way to iterate over the elements from the end to the beginning.
How to Use Reverse Iterators
In C++, using reverse iterators is intuitive and straightforward. You can achieve this with the `rbegin()` and `rend()` functions. Here’s how you can do it:
std::vector<int> vec = {1, 2, 3, 4, 5};
for (auto it = vec.rbegin(); it != vec.rend(); ++it) {
std::cout << *it << " ";
}
Explanation:
- `rbegin()` returns an iterator pointing to the last element, while `rend()` points to one position before the first element. This allows you to traverse the vector seamlessly from back to front.
Using Standard Loops with Indices
Reverse Indexing Technique
Another practical method for iterating a vector in reverse is to use reverse indexing. This approach involves simply decrementing the index during iteration. The code snippet below demonstrates this technique:
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int i = vec.size() - 1; i >= 0; --i) {
std::cout << vec[i] << " ";
}
Explanation:
- The `size()` method is called to get the total number of elements, and then we can access each element in reverse order by using `vec[i]`. This method is simple and effective for reverse iteration.
Using STL Algorithms
The `std::reverse` and `std::for_each`
C++ also provides robust facilities in the Standard Library to handle vector manipulation, such as `std::reverse` and `std::for_each`. The following example shows how to utilize these functions:
std::vector<int> vec = {1, 2, 3, 4, 5};
std::reverse(vec.begin(), vec.end());
std::for_each(vec.begin(), vec.end(), [](int num) {
std::cout << num << " ";
});
Explanation:
- The `std::reverse` function reverses the order of elements within the vector seamlessly, and then `std::for_each` applies a function to each element, in this case, a lambda that prints each element. This method is very powerful for applying actions to each element after rearranging them.
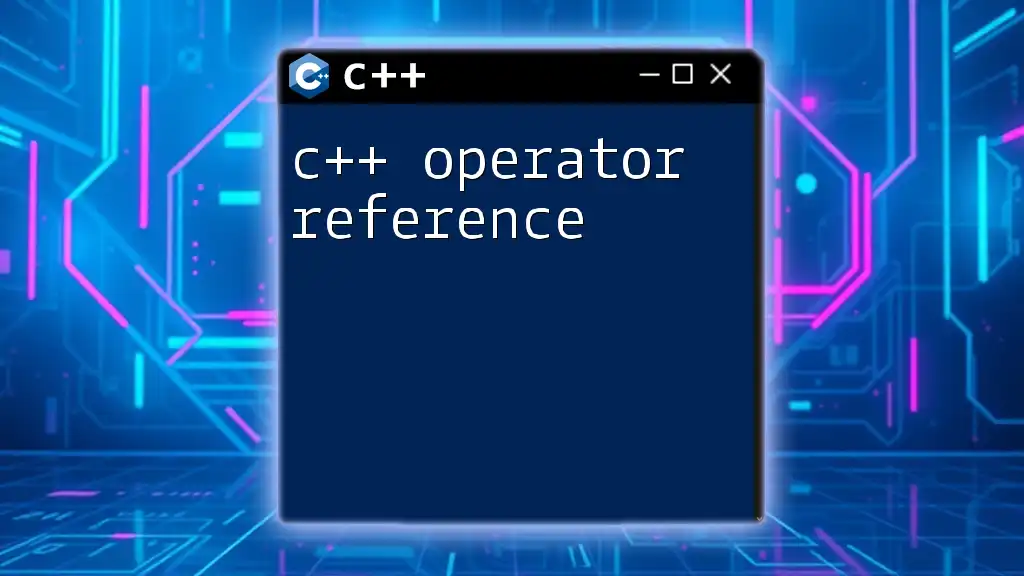
Performance Considerations
Time Complexity of Reverse Iteration
When considering performance, both reverse iterators and index-based methods exhibit O(n) time complexity for a complete traversal, where n is the number of elements in the vector. However, using STL algorithms like `std::reverse` may have additional overhead associated with the construction of iterators and the lambda function called within `std::for_each`.
Choosing the right method depends on the context—if your application requires frequent access to the elements in reverse order, it is helpful to explore which method fits best.
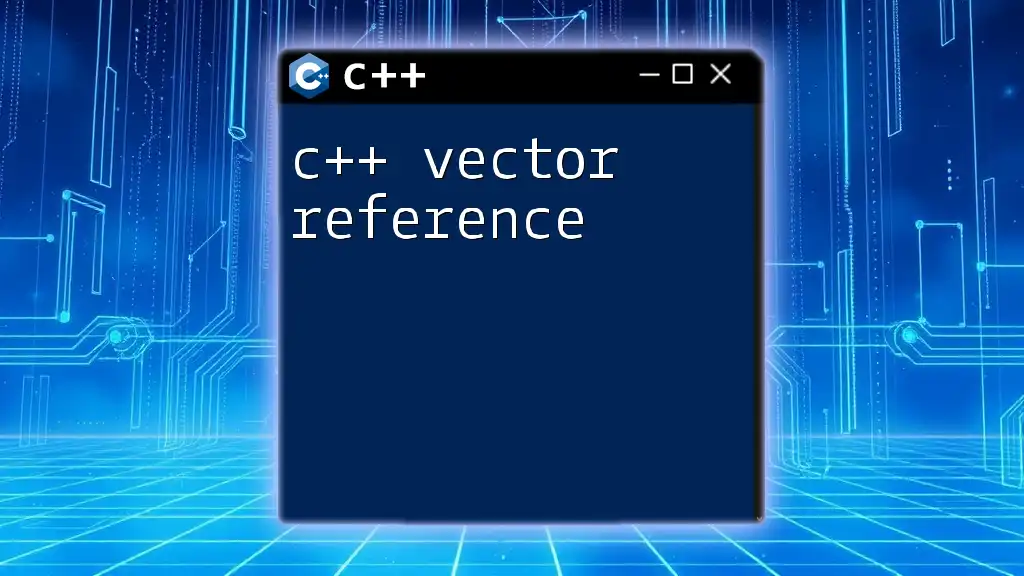
Practical Applications
Use Cases for Reverse Iteration
Reverse iteration is particularly useful in scenarios like:
- Game development: where you may need to delete or process items from the end of a list.
- Data processing tasks: such as backtracking algorithms or undo features.
- Handling user input: often requires scanning user inputs from the most recent to the oldest.
Real-World Example
For instance, if you're developing a text-based user interface where you want to display the most recent commands input by the user, you can utilize reverse iteration to present the last inputs first:
std::vector<std::string> commands = {"open", "save", "close", "quit"};
for (auto it = commands.rbegin(); it != commands.rend(); ++it) {
std::cout << *it << std::endl;
}
This code effectively showcases the commands in the reverse order they were inputted, giving users context about their recent actions.
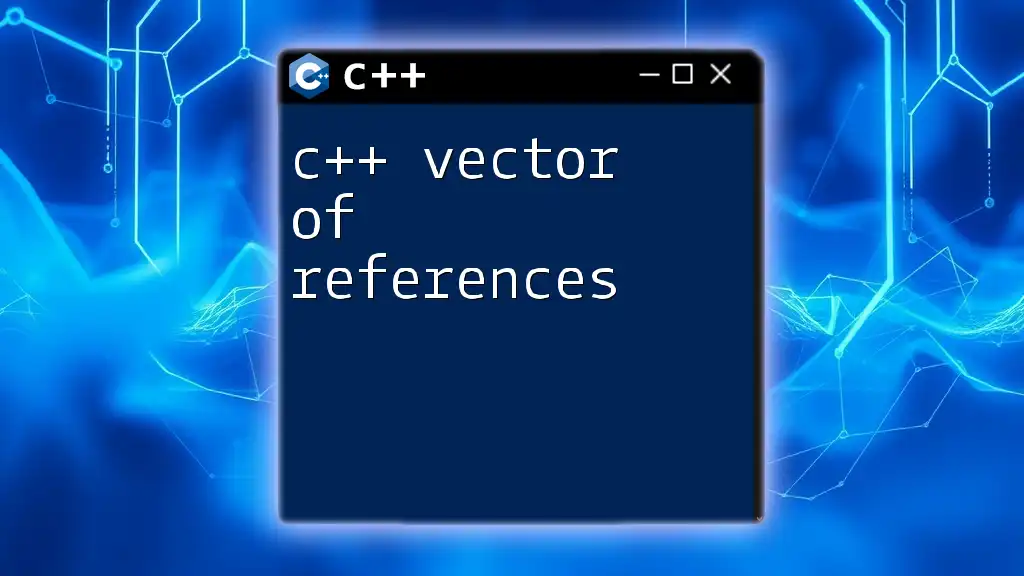
Common Mistakes to Avoid
Index Out of Bounds
A common mistake while iterating a vector in reverse is accessing elements outside the bounds. Always ensure you check that your index does not drop below zero:
// Oftentimes, forget to check size() can lead to:
std::vector<int> vec = {1, 2, 3};
for (int i = vec.size(); i >= 0; --i) { // Error: will access vec[-1]
std::cout << vec[i] << " ";
}
Misunderstanding Iterators
It's also vital to understand the concept of iterator invalidation. Changing the structure of the vector (like adding or removing elements) can invalidate iterators, leading to undefined behavior.
To avoid issues, always ensure you’re not modifying the vector within the same scope where iterators are being used.
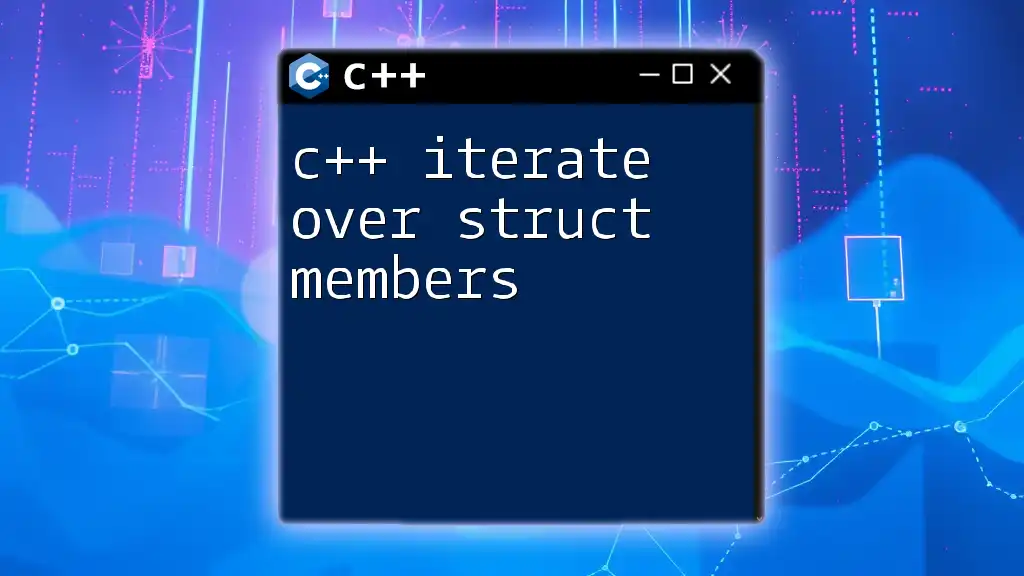
Conclusion
By mastering how to c++ iterate vector in reverse, you can enhance your programming skills and improve the efficiency of your applications. Whether using reverse iterators, indexing techniques, or leveraging the powerful STL algorithms, each method has its unique advantages and use cases.
As you practice these techniques, you'll gain confidence in managing vectors effectively in your C++ projects. Happy coding!