In C++, you can iterate over struct members using a combination of reflection or by using helper functions to access each member, typically through a predefined list of member pointers.
Here's an example code snippet:
#include <iostream>
#include <tuple>
#include <string>
struct Person {
std::string name;
int age;
double height;
};
template<typename T, std::size_t... I>
void iterateStructMembers(const T& obj, std::index_sequence<I...>) {
((std::cout << obj.*std::get<I>(std::tie(&T::name, &T::age, &T::height)) << '\n'), ...);
}
int main() {
Person person = {"Alice", 30, 5.6};
iterateStructMembers(person, std::make_index_sequence<3>{});
return 0;
}
Understanding C++ Structs
What is a Struct?
A struct in C++ is a user-defined data type that allows grouping of different variables under a single name. It can contain multiple members, which can be of various data types. Unlike classes, structs have public members by default and are generally used to represent simple data structures.
Declaring and Initializing Structs
To declare a struct, you specify the `struct` keyword followed by the name of the struct and its members enclosed in curly braces. Here’s a straightforward example:
struct Person {
std::string name;
int age;
};
You can initialize a struct object as follows:
Person john = {"John Doe", 30};
Best practices suggest initializing struct members immediately upon creation for better readability and maintainability.

Methods to Iterate Over Struct Members
Using Reflection (C++20)
Reflection is a powerful feature introduced in C++20, allowing developers to inspect object structures at runtime. Although its implementation may vary by compiler, the concept is revolutionary for dynamically iterating over struct members.
In principle, you could use something like this:
// Example requires C++20 reflection capabilities
However, since this feature is still in flux, it’s essential to check compiler support or community libraries that provide reflection-like capabilities for older standards.
Manual Iteration Using `std::array` or `std::vector`
A common approach to iterate over struct members is to store them in an `std::array` or `std::vector`. Let’s say we want to create a structure for colors and iterate over them:
struct Color {
int r, g, b;
};
Color colors[] = { {255, 0, 0}, {0, 255, 0}, {0, 0, 255} };
for (const auto& color : colors) {
std::cout << "R: " << color.r << " G: " << color.g << " B: " << color.b << '\n';
}
This method is simple but effective. Pros include easy implementation and type safety. Cons arise if you have a large number of struct members, making this approach cumbersome.
Using `std::tuple` for Variadic Structs
When you want to group different types and iterate through them, consider using `std::tuple`. This structure allows you to bundle different types together and use structured binding to access the members easily. Here’s how you might do this:
#include <tuple>
struct Employee {
std::string name;
int id;
};
std::tuple<std::string, int> emp{"Alice", 101};
auto [name, id] = emp;
std::cout << "Name: " << name << ", ID: " << id << '\n';
Using `std::tuple` can offer significant type safety and allows for various data types, but be aware that accessing each member requires you to know their order and type upfront.
Utilizing Macros for Struct Member Access
C++ macros can be a double-edged sword. While they can potentially simplify syntax, they may also lead to maintenance nightmares if used excessively. Here’s a basic implementation of a macro that iterates over struct members:
#define ITERATE_MEMBERS(STRUCT) \
for (const auto &member : {STRUCT.name, STRUCT.age})
struct Student {
std::string name;
int grade;
};
Student student{"Bob", 90};
ITERATE_MEMBERS(student) {
std::cout << member << ' ';
}
While this could save time, be cautious: macros can obscure code meaning and produce unexpected behaviors if not managed carefully.
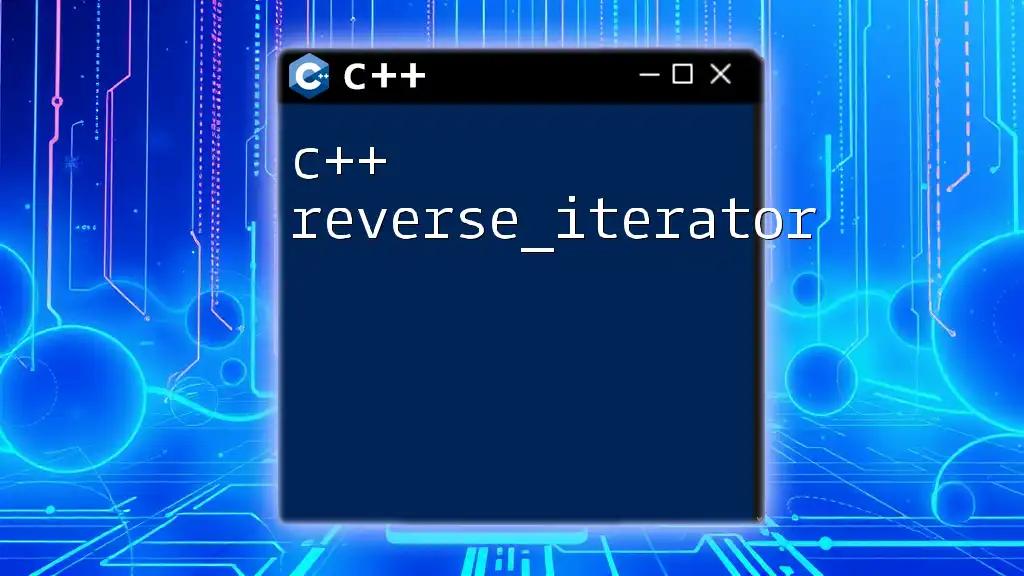
The Role of Templates in Iterating Struct Members
Template Specialization
Templates provide a level of flexibility that can be beneficial in various contexts. By using templates, you can write function signatures that can accept any struct type.
Here’s an example of applying templates for struct iteration:
template<typename T>
void printStructMembers(const T& structObj) {
// Your custom print logic here
}
Using templates means you can craft functions that work for various struct types without rewriting code.
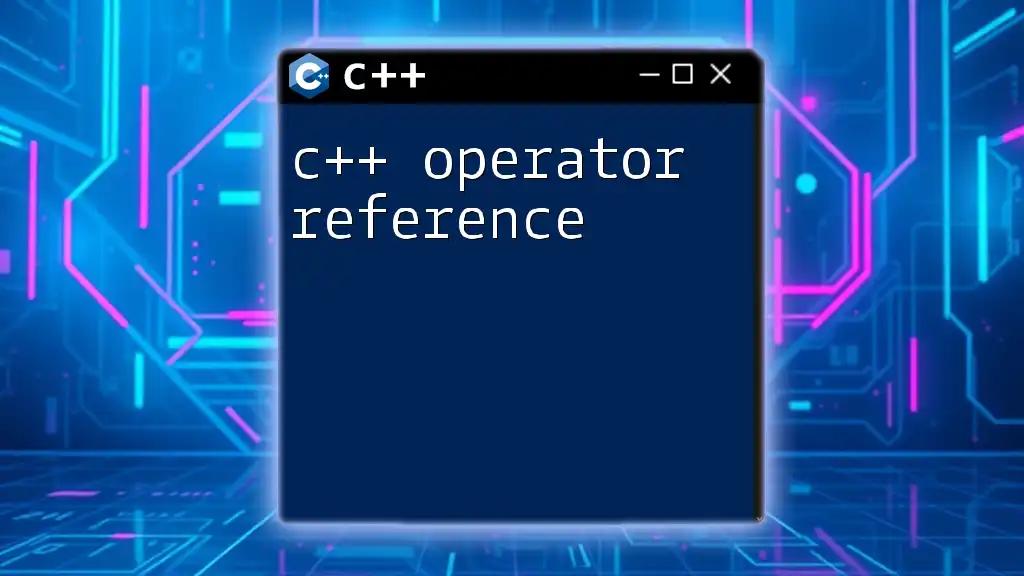
Conclusion
In this guide, we explored several ways to c++ iterate over struct members, each with its potential advantages and drawbacks. From traditional manual iteration using containers like `std::array` to more advanced techniques using templates and macros, the choice largely depends on your specific needs and the nature of the struct.
Experimenting with different methods offers insights into which approach suits your coding style and application requirements. Mastering struct member iteration is not just about syntax but understanding how to leverage the power of C++ in ways that enhance your programming efficiency.

Additional Resources
For more in-depth studies, explore resources on C++ structs and iteration techniques. Books and online courses on C++ programming can also provide valuable insight into struct usage and advanced programming topics.

Call to Action
We invite you to share your thoughts or any questions regarding iterating over struct members in C++. What methods do you prefer, and why? Join our community for more tips on mastering C++ commands!