To get the current directory in a C++ program, you can use the `getcwd` function from the `<unistd.h>` header file on Unix-like systems or `_getcwd` from `<direct.h>` on Windows.
Here's a code snippet demonstrating this in C++:
#include <iostream>
#include <unistd.h> // For UNIX-like systems
#include <direct.h> // For Windows
int main() {
char cwd[1024];
#ifdef _WIN32
_getcwd(cwd, sizeof(cwd));
#else
getcwd(cwd, sizeof(cwd));
#endif
std::cout << "Current directory: " << cwd << std::endl;
return 0;
}
Understanding the Current Directory
The current working directory is the directory in which a program is executed. It serves as the base location for relative file paths and can greatly affect file operations like reading, writing, and executing files. Understanding how to access this information is crucial for effective file management in C++ programming.
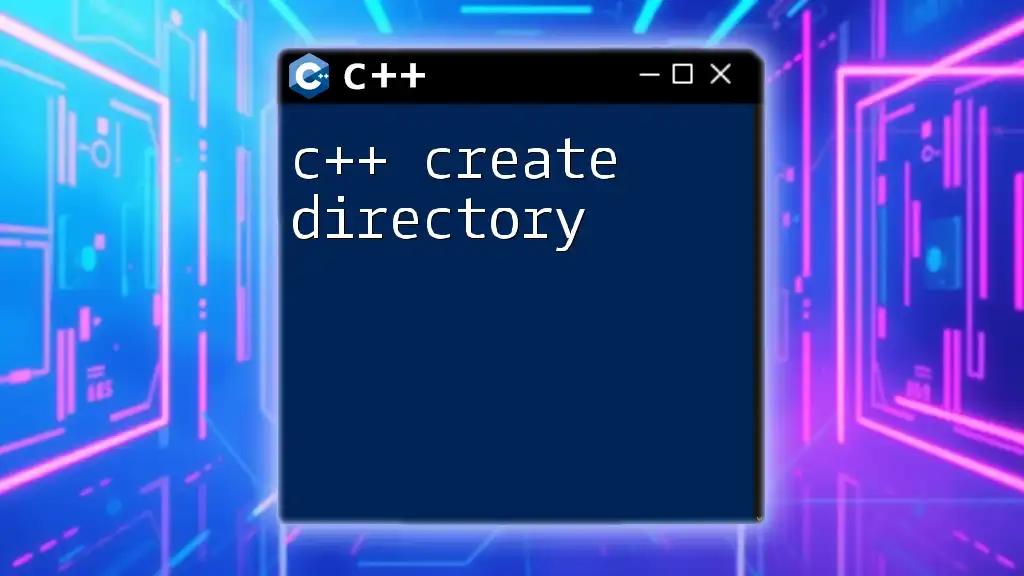
Methods to Get Current Directory in C++
There are several methods to retrieve the current directory in C++, ranging from modern standards introduced in C++17 to older methods suitable for legacy code.
Using `<filesystem>` Library
The `<filesystem>` library is introduced in C++17, providing a robust and intuitive way to handle filesystem paths and operations. This new addition simplifies many common tasks, such as getting the current directory.
Benefits of using `<filesystem>` include:
- Cross-Platform Compatibility: Code remains portable across different operating systems.
- Rich Functionality: Provides numerous utilities for handling file paths, checking directory existence, and iterating over directories.
Example Code: Using `<filesystem>`
#include <iostream>
#include <filesystem>
int main() {
std::filesystem::path currentPath = std::filesystem::current_path();
std::cout << "Current Directory: " << currentPath << std::endl;
return 0;
}
Explanation:
- The code begins by including the necessary headers.
- `std::filesystem::current_path()` fetches the current directory path and stores it in `currentPath`.
- Finally, it outputs the directory to the console. This method is straightforward and efficient, capitalizing on C++'s modern capabilities.
Alternative Methods to Get Current Directory in C++
For projects that don't support C++17, we can resort to traditional methods.
Using `getcwd` from `<unistd.h>`
The `getcwd` function is a POSIX standard function that retrieves the current working directory. Knowing how to use this function can be useful when you're working in a Linux or UNIX-like environment.
Example Code: Using `getcwd`
#include <iostream>
#include <unistd.h>
#include <limits.h>
int main() {
char currentPath[PATH_MAX];
if (getcwd(currentPath, sizeof(currentPath)) != nullptr) {
std::cout << "Current Directory: " << currentPath << std::endl;
} else {
std::cerr << "Error getting current directory!" << std::endl;
}
return 0;
}
Explanation:
- In this code snippet, the header `<unistd.h>` is included to access the `getcwd` function.
- The character array `currentPath` is predefined with the maximum possible path length.
- The `getcwd` function attempts to populate `currentPath` with the current directory.
- An if-statement checks for success; if it fails, an error message is displayed.
Using `_getcwd` on Windows Systems
If you are developing applications that run exclusively on Windows, the `_getcwd` function is the Windows equivalent of `getcwd` and is defined in `<direct.h>`.
Example Code: Using `_getcwd`
#include <iostream>
#include <direct.h> // _getcwd
int main() {
char currentPath[_MAX_PATH];
if (_getcwd(currentPath, sizeof(currentPath))) {
std::cout << "Current Directory: " << currentPath << std::endl;
} else {
std::cerr << "Error getting current directory!" << std::endl;
}
return 0;
}
Explanation:
- The `_getcwd` function functions similarly to `getcwd`. It requires including the `<direct.h>` header.
- `currentPath` is again defined with `_MAX_PATH` to ensure it can store the long path name.
- The application checks if the path retrieval is successful, and outputs the current directory, or displays an error if there is a problem.
Best Practices for Getting the Current Directory
When choosing a method to get the current directory, consider the following best practices:
- Use the `<filesystem>` Library: If you are using a C++ version that supports it, this is the recommended approach due to its simplicity and versatility.
- Error Handling: Always incorporate error handling when calling these functions. It allows your program to respond appropriately to unexpected situations such as permission issues or non-existent paths.
- Compatibility: Be mindful of the environment your code will run in. Using `getcwd` or `_getcwd` can lead to portability issues between UNIX-like systems and Windows.
Common Use Cases for Retrieving the Current Directory
Knowing how to retrieve the current directory can greatly facilitate various tasks in your applications:
- File I/O Operations: When reading from or writing to files, the program often needs to determine where to look or where to save files relative to the current directory.
- Logging: Applications frequently log their activities or errors to files, and knowing the current directory can help manage log files effectively.
- Resource Management: Many applications depend on external resources, such as configuration files or assets. Retrieving the current directory allows developers to locate these resources easily.
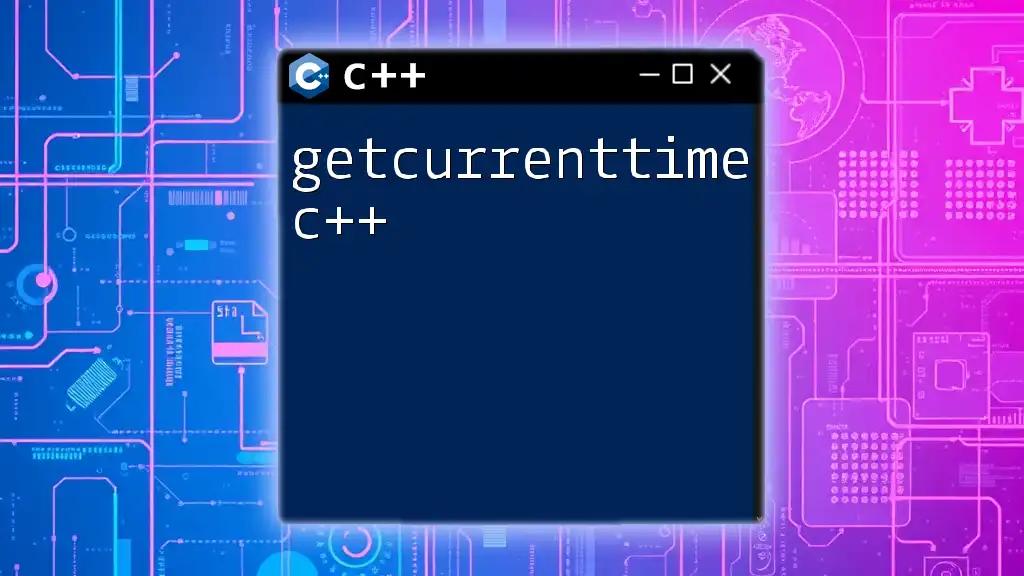
Conclusion
Obtaining the current directory in C++ is fundamental for effective file management and general application functionality. Whether using the modern `<filesystem>` approach or the traditional `getcwd` and `_getcwd` methods, understanding the options available empowers you to write more robust code.
Practice using these methods in small projects to solidify your understanding and explore how they can enhance your C++ programming skills. Don't hesitate to experiment with error handling and adapting the methods to your specific application needs.