Order of precedence in C++ determines the sequence in which operators are evaluated in expressions, impacting the final result.
Here’s a code snippet showcasing order of precedence:
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 10, c = 20;
int result = a + b * c; // b * c is evaluated first
cout << "Result: " << result; // Output will be 205
return 0;
}
Understanding Operators in C++
In C++, operators are special symbols that perform operations on variables and values. Here are the main categories of operators you will encounter:
- Arithmetic Operators: Used to perform basic mathematical operations such as addition, subtraction, multiplication, and division.
- Relational Operators: Used to compare two values, resulting in a boolean value (true or false). Examples include `==`, `!=`, `<`, and `>`.
- Logical Operators: Used to combine multiple boolean expressions. The common logical operators are `&&` (AND), `||` (OR), and `!` (NOT).
- Bitwise Operators: Perform operations on bits. E.g., `&` (AND), `|` (OR), and `^` (XOR).
- Assignment Operators: Used to assign values to variables, including `=`, `+=`, `-=`, `*=`, and `/=`.
- Miscellaneous Operators: Includes the ternary operator (`?:`), sizeof operator, and comma operator.
Each operator has a defined precedence level, which dictates in what order operations are performed in expressions.
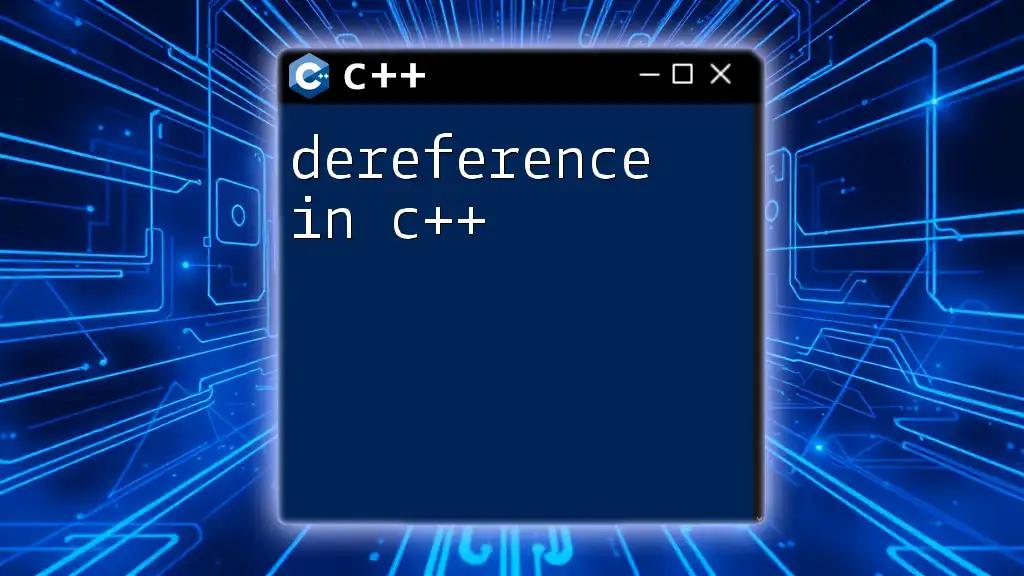
The Order of Precedence in C++
Understanding the order of precedence in C++ is crucial for interpreting how expressions are evaluated. Each operator is assigned a precedence level that determines how and when it is applied during computation.
Here’s a simple table of operators and their precedence (from highest to lowest):
Operator | Type |
---|---|
`()` | Parentheses |
`++`, `--` | Unary Operators |
`*`, `/`, `%` | Multiplication/Division |
`+`, `-` | Addition/Subtraction |
`<<`, `>>` | Bitwise Shift |
`<`, `<=`, `>`, `>=` | Relational Operators |
`==`, `!=` | Equality Operators |
`&` | Bitwise AND |
`^` | Bitwise XOR |
` | ` |
`&&` | Logical AND |
` | |
`?:` | Ternary Operator |
`=`, `+=`, `-=`, `*=`, `/=` | Assignment Operators |
Precedence is not the only factor to consider; associativity defines the order in which operators of the same precedence are evaluated. Operators can be either left to right or right to left. For instance, `+` and `-` have left-to-right associativity, while the assignment operator `=` has right-to-left associativity.
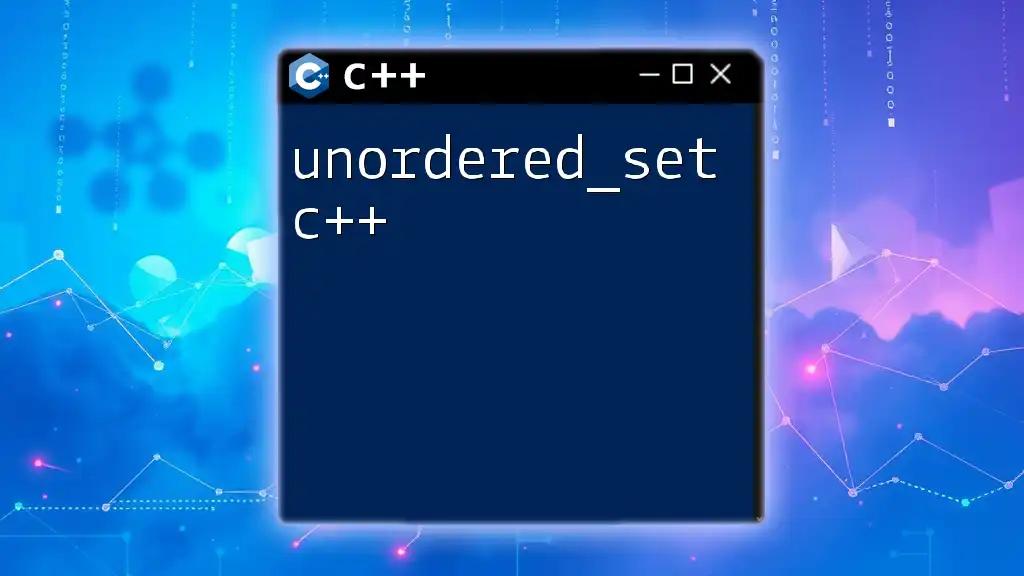
Examples of Order of Precedence
Simple Example with Arithmetic Operations
Consider the following expression:
int result = 3 + 4 * 2;
In this case, due to the order of precedence, the multiplication operator `*` has a higher precedence than addition `+`. Therefore, the evaluation occurs as follows:
- `4 * 2` is calculated first, resulting in `8`.
- Then, `3 + 8` results in `11`.
Complex Example Mixing Different Operator Types
Now, examine a more complex expression:
bool flag = (3 + 4 < 10) && (5 > 2);
Here's how this will be evaluated:
- The sub-expression `3 + 4` is evaluated first (due to the precedence of addition).
- This results in `7`, leading to `7 < 10`, which is `true`.
- The second sub-expression `5 > 2` is evaluated next, resulting in `true`.
- Finally, since both sub-expressions are `true`, the logical AND `&&` evaluates to `true`.
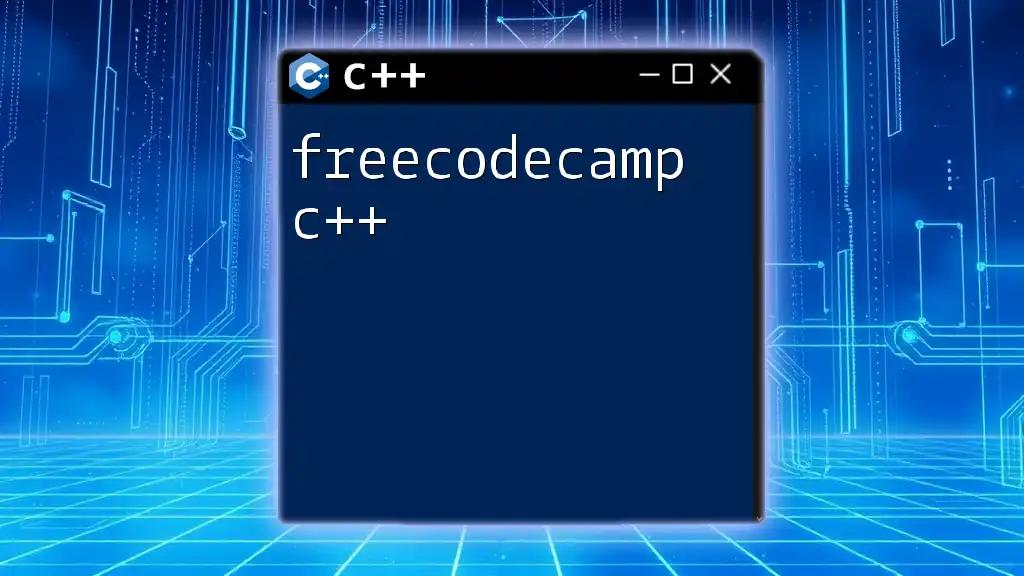
Parentheses and Their Role
Parentheses play a pivotal role in overriding default precedence. They allow you to group operations, ensuring that expressions are evaluated in a specific order.
For instance, consider the following code:
int result = (3 + 4) * 2;
Using parentheses, the expression `3 + 4` will be evaluated first, resulting in `7`, before being multiplied by `2`. Hence, the result is `14`.
In contrast, without parentheses, the expression would default to:
int result = 3 + (4 * 2);
Here, `4 * 2` is computed first, yielding `8`, and the final result would be `11`.
Tip: Use parentheses to enhance the clarity of your code. They can significantly reduce ambiguity and ensure the intended order of operations.

Common Mistakes and Misunderstandings
Even experienced developers sometimes fall prey to common mistakes concerning operator precedence:
- Ambiguous Expressions: For example, in evaluating `x + y * z`, many might mistakenly think addition occurs first, which leads to incorrect results.
int x = 2, y = 3, z = 4;
int result = x + y * z; // This evaluates as 2 + (3 * 4) = 14
- Misusing Assumptions: Another common issue arises when combining different types of operators without attention to precedence. An expression like `a || b && c` should be treated carefully as `a || (b && c)`.
To avoid these pitfalls, always review expressions before finalizing your code and consider using parentheses for clarity.

Practical Applications of Order of Precedence
A strong grasp of the order of precedence in C++ is tremendously beneficial during algorithm development or debugging. Let's say you’re designing a function that calculates discounts:
double calculateTotal(double price, double discount, double tax) {
return price * (1 - discount) + tax; // Precedence clarifies your intention here
}
Understanding the precedence ensures your calculations reflect the intended logic.
Additionally, practice is crucial. Consider implementing small coding exercises that involve expressions with varying operator types to build proficiency in operator precedence.
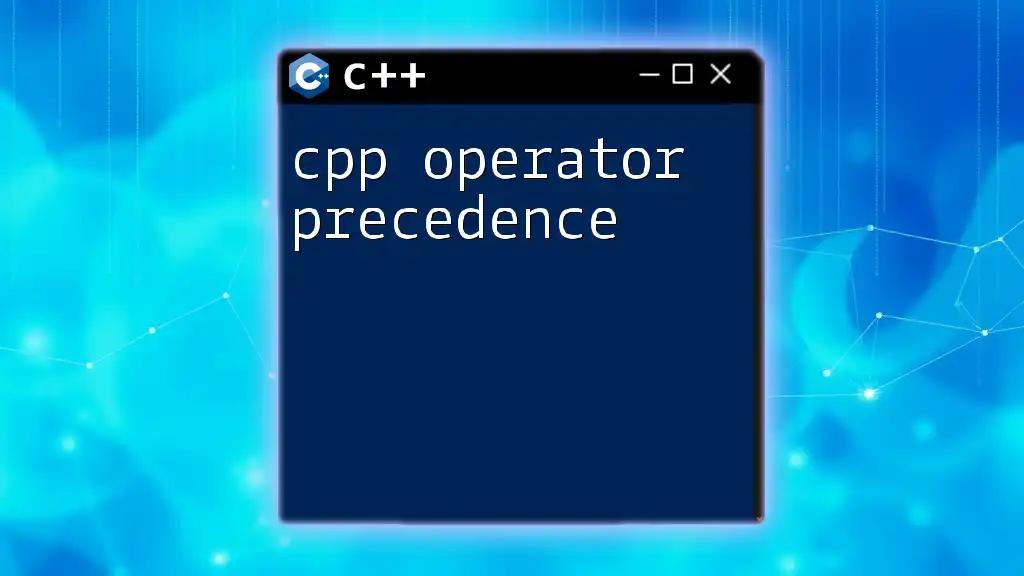
Summary
Understanding the order of precedence in C++ is vital for proper expression evaluation and accurate code writing. Remember that small mistakes can lead to unexpected behavior, making knowledge of operator precedence not just a best practice but a necessity.
As you continue your coding journey, focus on mastering these principles through consistent practice with various examples.
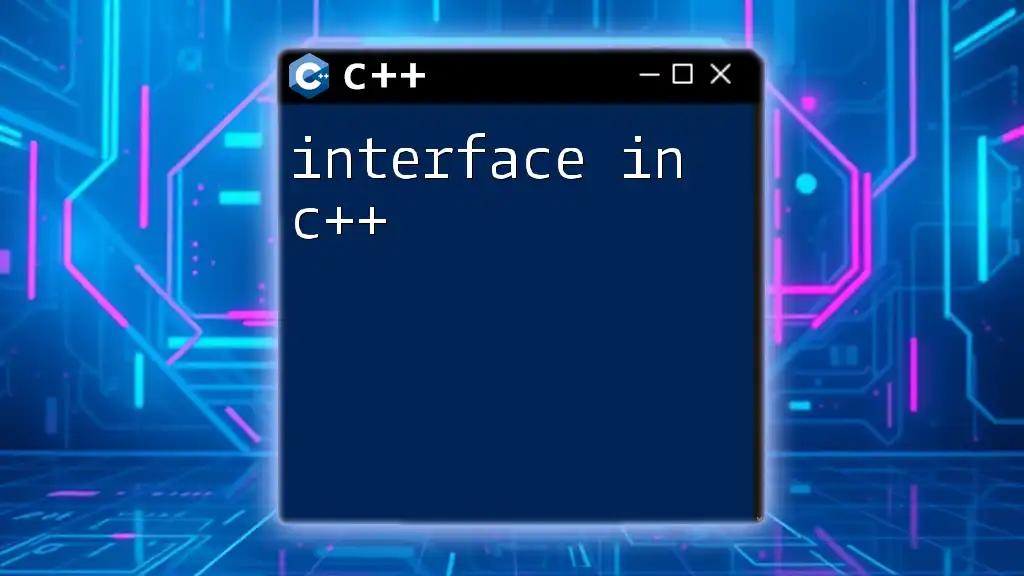
Frequently Asked Questions
What is the difference between order of precedence and order of operations?
Order of precedence refers to the rules that dictate which operators are applied first in an expression, while order of operations is a broader concept generally associated with mathematical expressions and includes rules like PEMDAS (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction).
How can I remember operator precedence rules?
A useful approach is to memorize the precedence ranking, possibly using mnemonics. Alternatively, maintaining a reference table nearby as you code can be immensely helpful.
Are there any exceptions to the general rules of precedence?
While precedence rules are standardized, always refer to the language documentation to be aware of any potential exceptions or changes in future iterations of C++.