Pattern matching in C++ can be efficiently achieved using regular expressions from the `<regex>` library to search, match, and manipulate strings based on specific patterns. Here's a simple example:
#include <iostream>
#include <regex>
int main() {
std::string text = "There are 123 apples and 456 oranges.";
std::regex pattern("\\d+"); // Matches one or more digits
std::smatch matches;
while (std::regex_search(text, matches, pattern)) {
std::cout << "Found number: " << matches[0] << std::endl;
text = matches.suffix().str(); // Update text to search in
}
return 0;
}
Understanding C++ Pattern Matching Concepts
What is Pattern Matching?
Pattern matching is a critical concept that allows developers to identify and manipulate strings or sequences based on specific patterns. Within programming, patterns represent certain conditions or structures, enabling developers to match inputs based on defined criteria.
Why Use C++ for Pattern Matching?
C++ offers a robust environment for pattern matching due to its versatility and performance-oriented design. The efficiency of compiled languages allows for rapid processing and handling of complex data. Furthermore, C++ provides various libraries, such as `<regex>`, that facilitate advanced pattern matching and string manipulation.
Basic Principles of Pattern Matching
At its core, pattern matching can be defined around two primary concepts: lexical patterns, which focus on the superficial structure of data, and semantic patterns, which concern the underlying meaning. C++ effectively supports both types through its rich feature set, enabling programmers to develop sophisticated algorithms for text processing.

Pattern Matching with Strings
Using Standard Library Functions
C++ provides various functions within the `<string>` library that enable simple pattern matching. Understanding and utilizing these functions can streamline many common tasks.
For instance, if you need to match a substring within a larger string, `std::string::find` is invaluable. Here’s a simple example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
if (text.find("World") != std::string::npos) {
std::cout << "Pattern found!" << std::endl;
}
return 0;
}
In this code, the `find` method checks for the occurrence of "World" in the string "Hello, World!". If the pattern is found, it returns the index of the first occurrence, while `std::string::npos` indicates no match.
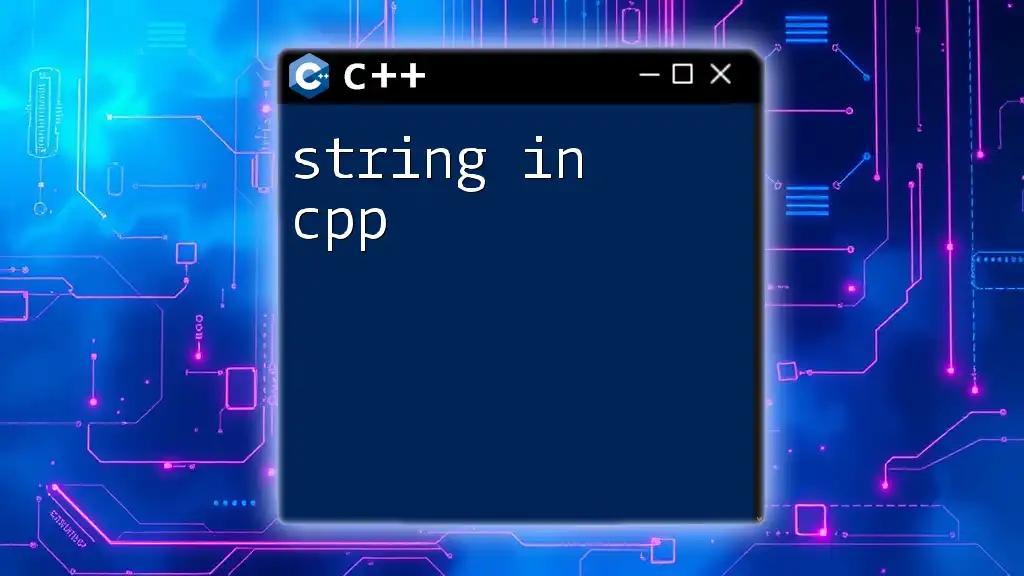
Regular Expressions in C++
Introduction to std::regex
The `<regex>` library in C++ introduces regular expressions, which are indispensable for complex pattern matching tasks. Regular expressions allow for versatile matching, searching, and manipulation operations, making them a powerful tool in any developer’s arsenal.
Key components of this library include:
- `std::regex`: Represents the regex pattern.
- `std::smatch`: Used for storing match results.
- `std::regex_match`: Evaluates whether the entire string matches a pattern.
Basic Regular Expression Syntax
Regular expressions consist of various syntax elements that define the patterns you want to match. Here are a few common components:
- Literals: Regular characters.
- Metacharacters: Special characters with specific meanings (e.g., `\d` for digits, `\w` for alphanumeric characters).
Practical Examples Using std::regex
Let’s see how to perform pattern matching with regex included in C++. Below is an example that checks whether a string has a valid email format:
#include <iostream>
#include <regex>
int main() {
std::string email = "example@gmail.com";
std::regex pattern(R"(\w+@\w+\.\w+)");
if (std::regex_match(email, pattern)) {
std::cout << "Valid email format." << std::endl;
} else {
std::cout << "Invalid email format." << std::endl;
}
return 0;
}
In this demonstration, the regex pattern checks for a simplistic email structure, ensuring it contains a username, an "@" symbol, and a domain format.
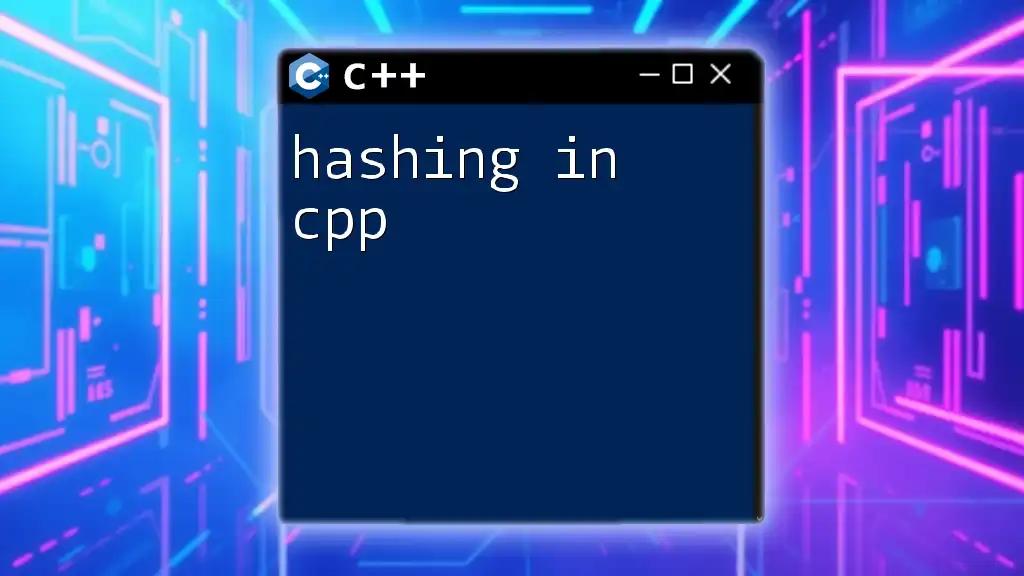
Advanced Pattern Matching Techniques
Capture Groups and Backreferences
Capture groups allow you to extract specific portions of a string that match certain patterns, enhancing your ability to process data dynamically. Here's an example of how to utilize capture groups:
#include <iostream>
#include <regex>
int main() {
std::string text = "John Doe, age 30";
std::regex pattern(R"((\w+) (\w+), age (\d+))");
std::smatch matches;
if (std::regex_search(text, matches, pattern)) {
std::cout << "First Name: " << matches[1]
<< ", Last Name: " << matches[2]
<< ", Age: " << matches[3] << std::endl;
}
return 0;
}
In this example, we use a regex to match a name and age format. The results are captured into a `std::smatch` object, allowing for easy access to each component of the pattern.
Using std::regex_replace for String Manipulation
The power of regex extends beyond just matching; `std::regex_replace` enables you to modify strings based on patterns. This can be particularly useful for text transformation tasks. Here’s an example where we replace a specific word in a sentence:
#include <iostream>
#include <regex>
int main() {
std::string text = "The sun is shining";
std::regex pattern(R"(sun)");
std::string result = std::regex_replace(text, pattern, "moon");
std::cout << result << std::endl; // Output: The moon is shining
return 0;
}
In the code above, every instance of "sun" is replaced with "moon", demonstrating how to effectively manipulate string content through pattern matching.

Performance Considerations in Pattern Matching
Efficiency of std::regex vs Other Methods
While regular expressions are powerful, they may incur performance overhead compared to straightforward string manipulation techniques. Understanding when to utilize regex versus simple string functions is essential for writing efficient C++ programs. Regular expressions excel in complex matching scenarios, while direct string functions suffice for simpler tasks.
Using Compile-time Regular Expressions (C++20)
Beginning with C++20, developers can take advantage of compile-time regular expressions, which offer improved performance by evaluating regex patterns during compilation rather than runtime. This advancement can lead to significant performance increases in applications where regex use is prevalent.
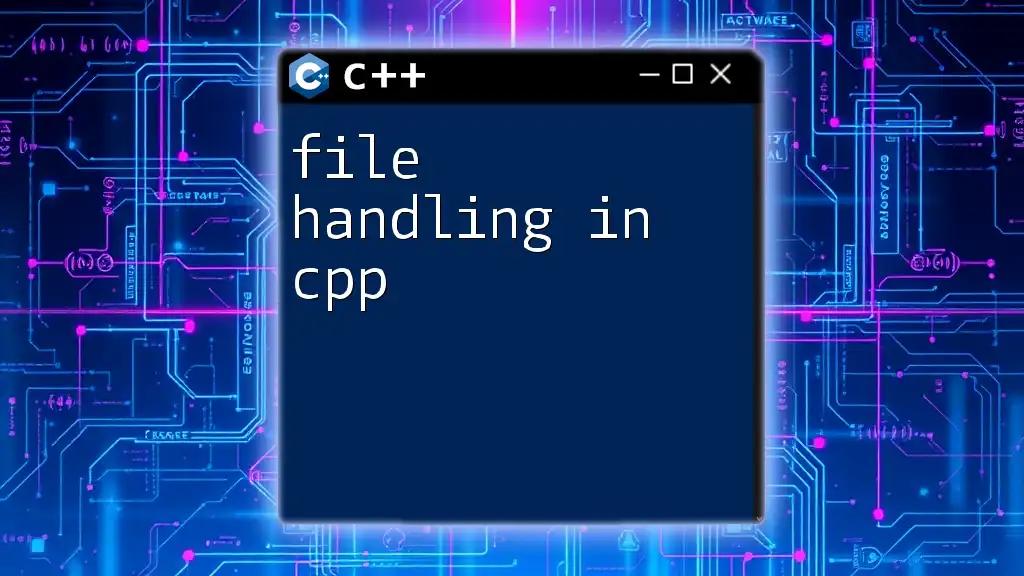
Conclusion
Recap of Key Takeaways
This guide has explored the fundamentals and applications of pattern matching in C++. By understanding the tools provided—specifically the capabilities of the `<regex>` library—you can handle a wide range of text processing tasks efficiently and effectively.
Further Resources and Next Steps
To enhance your knowledge and skills further, seek out additional resources, participate in community discussions, and engage with tutorials focused on regex and general string manipulation in C++. The journey towards mastering C++ pattern matching opens up myriad opportunities for elegant and efficient coding practices.

Call to Action
Engage with Us
We invite you to share your thoughts and experiences with pattern matching in C++. If you have questions or insights, feel free to comment below. Don't forget to share this article with fellow developers interested in improving their C++ skills!