In C++, the "incomplete type" error occurs when you try to use a class or struct that hasn't been fully defined, such as attempting to create an object or access members before the class definition is completed.
Here’s a code snippet that demonstrates this error:
#include <iostream>
struct Incomplete; // Forward declaration
int main() {
Incomplete obj; // Error: 'Incomplete' is an incomplete type
return 0;
}
What is an Incomplete Type?
In C++, an incomplete type is a type that has been declared but not fully defined. This means that while the compiler knows that a certain type exists, it lacks the necessary information about the structure or size of that type to safely allocate memory or perform operations on it. For example, when you forward declare a class but do not define it, you create an incomplete type.
Understanding incomplete types is crucial for C++ programmers because they are commonly the source of compilation errors, specifically related to C++ incomplete type is not allowed issues.
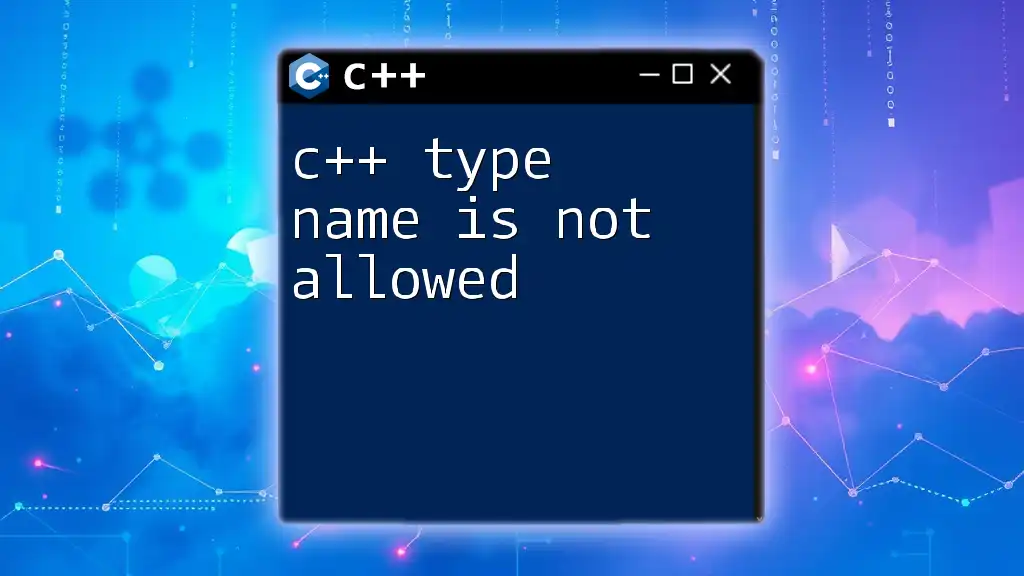
Understanding Types in C++
What Are Types in C++?
C++ supports various data types, which are classified into two main categories: built-in types (such as integers, floats, and characters) and user-defined types (such as classes, structs, and enums). Data types define the nature of the data a variable can hold and dictate how the program interacts with that data.
The Role of Type Checking
Type checking in C++ ensures that operations are performed on compatible types. The compiler checks that variable types conform to certain rules to avoid runtime errors, ensuring that data is manipulated correctly. This becomes particularly important when dealing with incomplete types.
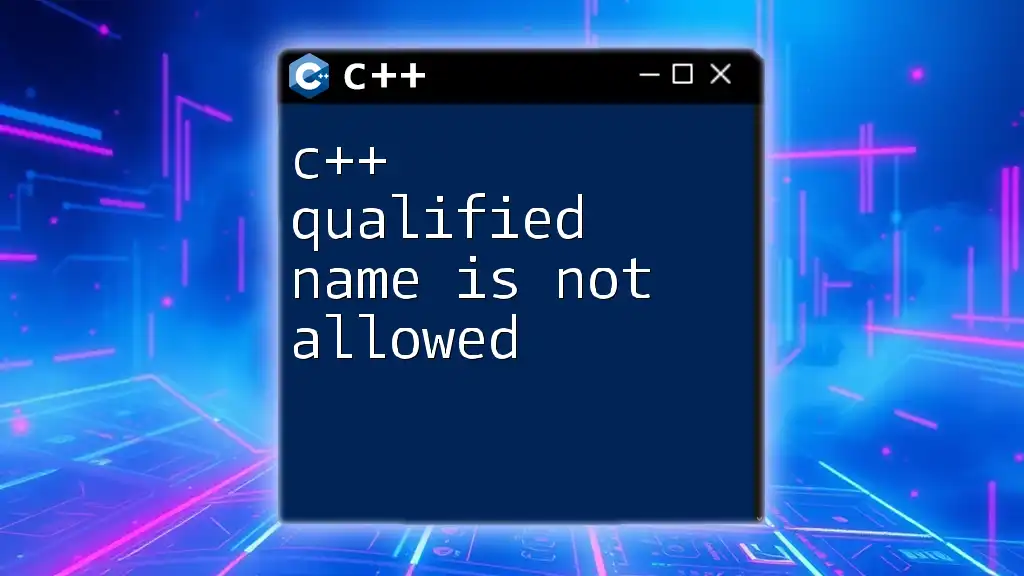
Incomplete Types in Detail
Definition of Incomplete Types
An incomplete type is essentially one that has been introduced but lacks a complete definition. When a type is declared as incomplete, the compiler cannot ascertain its size or allowed operations involving that type.
Common Examples of Incomplete Types
-
Forward Declarations of Classes: A forward declaration is a promise that a class will be defined later in the code.
class MyClass; // Forward declaration void processClass(MyClass obj); // This is an error; MyClass is incomplete here
-
Incomplete Structs and Unions: When a struct or union is declared but not defined, it is also considered incomplete.
struct MyStruct; // Incomplete struct declaration MyStruct instance; // This is an error: MyStruct is incomplete
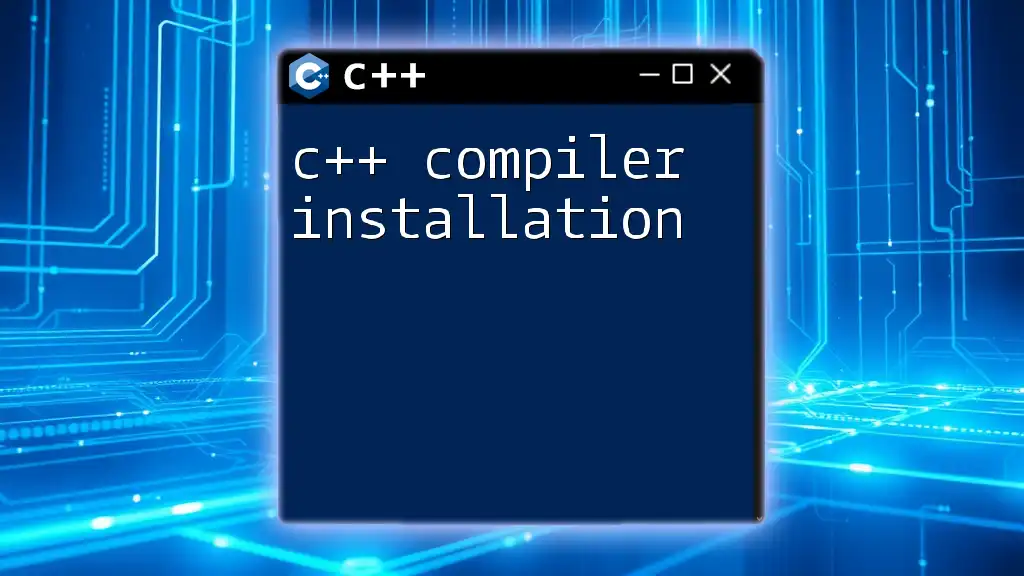
Why C++ Does Not Allow Incomplete Types
The Importance of Complete Types
C++ demands complete types during compilation because the compiler needs to know the size and layout of objects in memory. When dealing with functions or variables of a type, you cannot invoke methods or access members of an incomplete type.
Consequences of Using Incomplete Types
If you attempt to use an incomplete type, you will encounter compilation errors, as the compiler cannot allocate the necessary memory. For instance, trying to create an instance of an incomplete type leads to a compilation error.
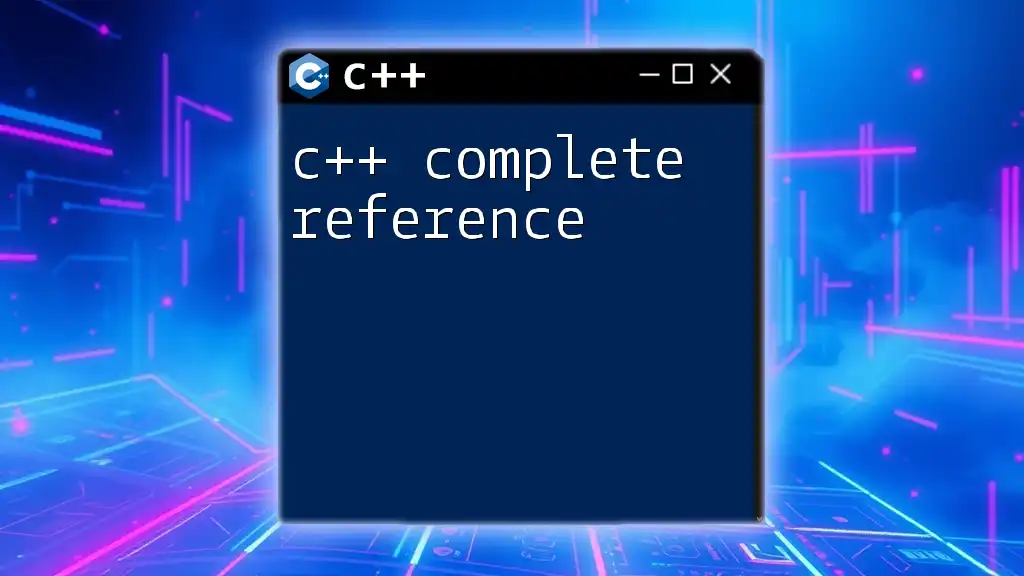
How to Resolve Incomplete Type Errors
Strategies for Handling Incomplete Types
To avoid the C++ incomplete type is not allowed errors, it's essential to adopt proper coding strategies.
Use Complete Type Declarations
Always ensure that you are using complete types in areas of your code where the type is required. This often involves defining classes and structs before using them:
class MyClass { // Complete definition
public:
void display() { /* implementation */ }
};
void processClass(MyClass obj) {
obj.display(); // Now this works, as MyClass is fully defined
}
Proper Preparation of Class Definitions
Ensure that the definition of any class or struct is provided before it is instantiated or used. This prevents type-related errors and ensures seamless compilation.
Utilizing Forward Declarations Appropriately
While forward declarations are useful, they must be applied judiciously. Only use them when you know the definition will be provided later in your code, and ensure that function signatures do not require access to the incomplete type.
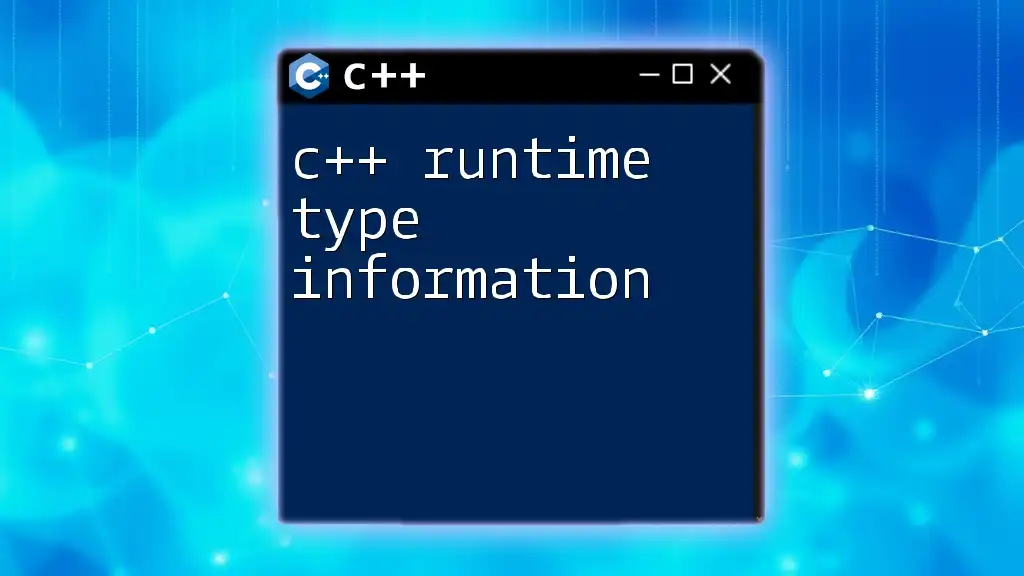
Common Errors Related to Incomplete Types
Compilation Errors Due to Incomplete Types
When you incorrectly try to instantiate or access members of an incomplete type, the compiler will generate an error. Here is an example:
class MyClass; // Forward declaration
MyClass obj; // Error: MyClass is an incomplete type
Debugging Incomplete Type Issues
To diagnose these issues:
- Look for places where you might have forgot to include complete type definitions.
- Consider using compiler flags that provide more verbose output, helping identify incomplete type errors quickly.
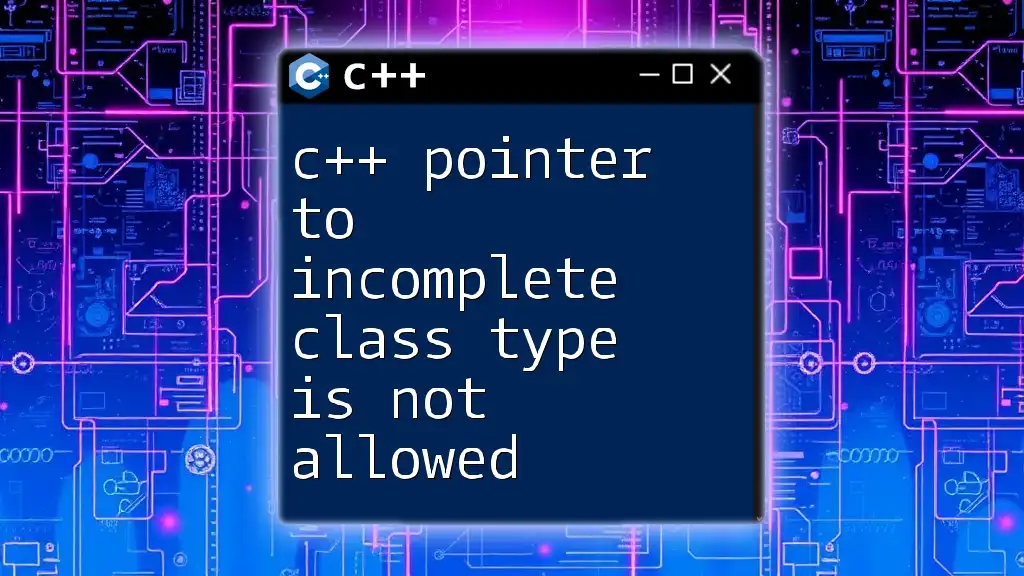
Best Practices
General Tips for Avoiding Incomplete Type Issues
- Organize Code Properly: Keep declarations and definitions organized so that types are fully defined before they are used.
- Minimize Forward Declarations: Use them sparingly and only when necessary; overuse can lead to complexities in code management.
Importance of Code Organization
A well-structured codebase enhances readability and can help prevent issues related to incomplete types. By grouping related declarations and definitions, you can ensure that types are fully recognized by the compiler before they are employed.
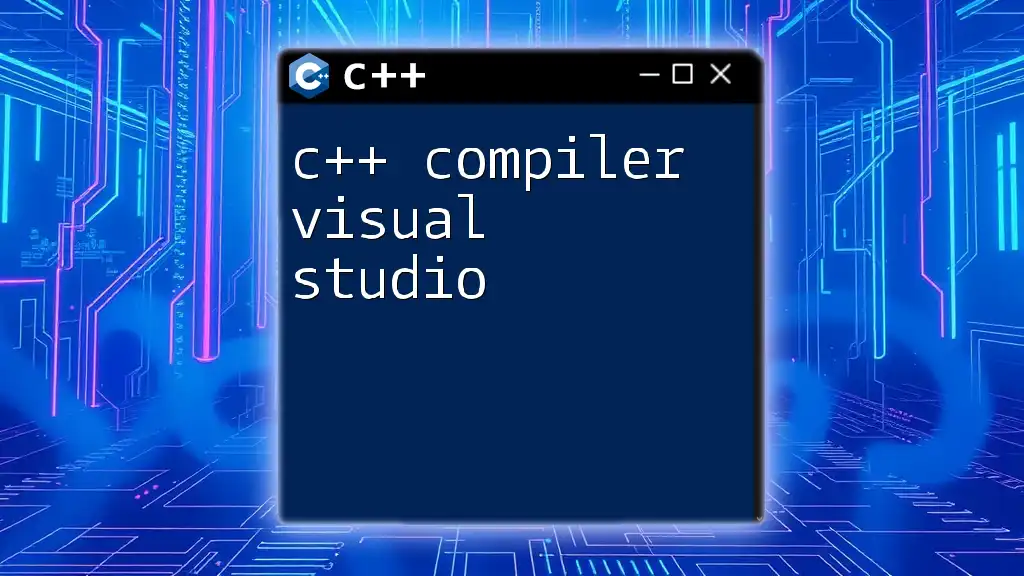
Conclusion
Understanding that the C++ incomplete type is not allowed error arises from incomplete type definitions is essential for effective programming. By maintaining a clear structure in your code, ensuring complete definitions, and managing your type declarations carefully, you can mitigate these issues and streamline your development process.
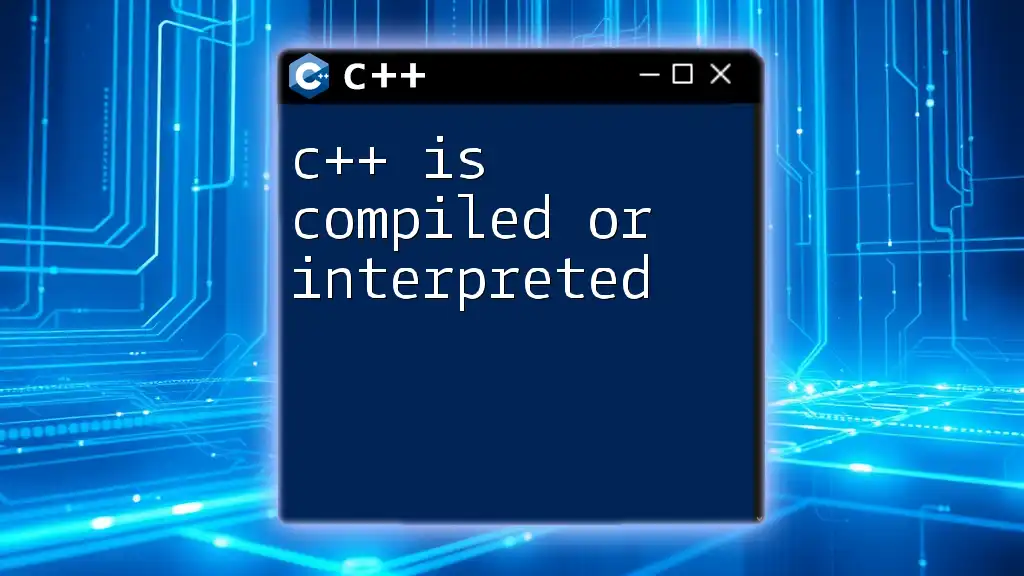
Additional Resources
For further exploration of C++ and to deepen your understanding, consider referencing materials like books focused on advanced C++ programming or engaging with online courses offering deeper dives into type management and other nuances of C++. Websites with comprehensive documentation and community support can also be invaluable.
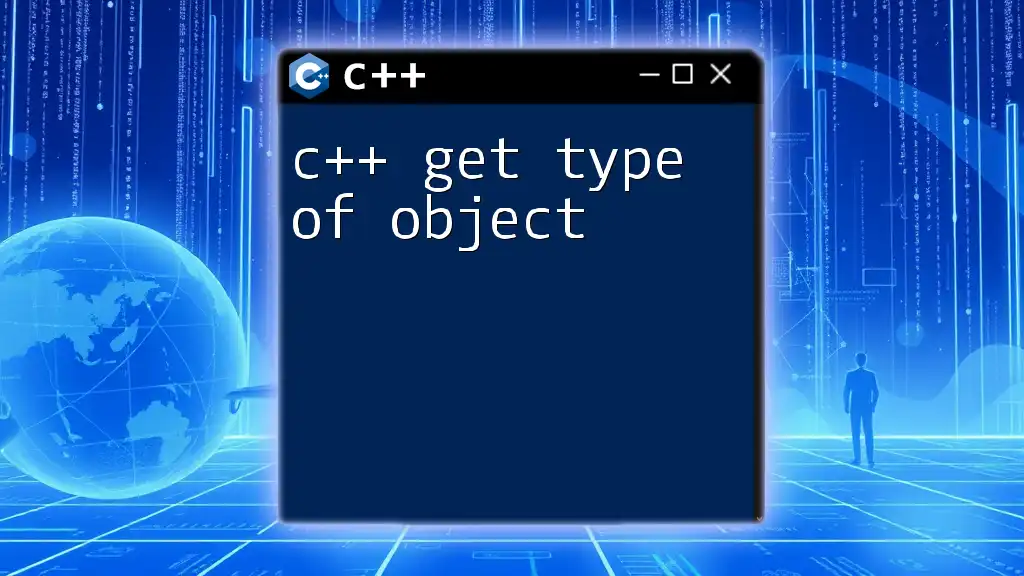
FAQs
What is the difference between a complete and an incomplete type?
A complete type has a full definition, allowing the compiler to determine its size and layout, while an incomplete type is declared but lacks sufficient information.
Can I use pointers to incomplete types?
Yes, you can use pointers to incomplete types as pointers are not concerned with the size of the type, but you cannot dereference them without a complete type definition.
How do templates interact with incomplete types?
Templates can be defined with incomplete types as long as the complete type is provided before instantiation. However, the complete type is necessary when you instantiate or use the template with that type.