To set all values of a C++ vector to zero, you can use the `std::fill` function from the `<algorithm>` header to fill the entire vector with zeros.
Here’s a code snippet demonstrating this:
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::fill(vec.begin(), vec.end(), 0);
return 0;
}
Understanding C++ Vectors
What Are C++ Vectors?
C++ vectors are a part of the Standard Template Library (STL) and represent an essential feature of modern C++ programming. They are dynamic arrays that allow for storing collections of items. Unlike primitive arrays, which have a fixed size, vectors can grow and shrink as needed.
The advantages of using vectors include:
- Dynamic Size: Vectors automatically resize themselves when you add or remove elements.
- Memory Management: C++ vectors handle memory allocation and deallocation automatically, reducing the risk of memory leaks.
- Ease of Use: With built-in functions for adding, removing, and accessing elements, vectors simplify data management.
How Vectors Work Internally
Internally, vectors maintain a contiguous block of memory to store their elements. They have a size (the number of elements they currently hold) and a capacity (the amount of allocated memory). When a vector's size exceeds its capacity, it reallocates memory to accommodate additional elements, copying over existing data. Understanding this dynamic allocation is crucial when working with vectors, as it can impact performance and memory usage.
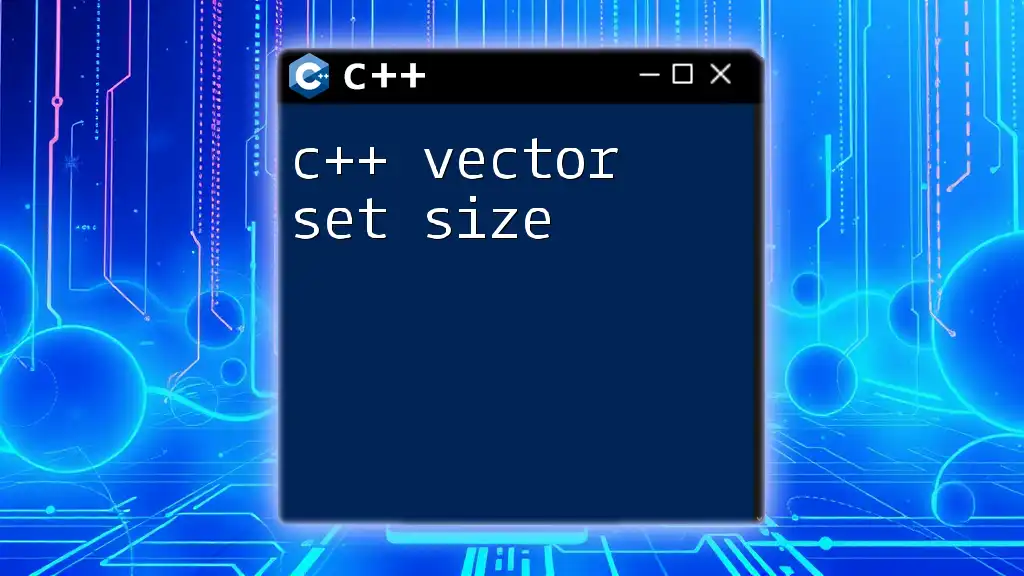
Methods to Set Vector Values to Zero
Using a Loop
One of the most straightforward methods for setting all values in a vector to zero is through a simple for-loop. This approach is clear, easy to understand, and widely used in C++ programming.
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
for (size_t i = 0; i < myVector.size(); ++i) {
myVector[i] = 0; // Setting each value to zero
}
// Output the modified vector
for (const auto& val : myVector) {
std::cout << val << " "; // Output: 0 0 0 0 0
}
return 0;
}
In this example, we iterate over the vector using its size and set each index to zero. This method is effective, but it can be a bit clunky compared to more concise alternatives.
Using `std::fill()`
The `<algorithm>` library provides a powerful function called `std::fill()`, which is ideal for bulk-setting values in a vector. This method enhances code readability and succinctness.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::fill(myVector.begin(), myVector.end(), 0); // Filling the vector with zeros
// Output the modified vector
for (const auto& val : myVector) {
std::cout << val << " "; // Output: 0 0 0 0 0
}
return 0;
}
In this case, `std::fill()` accepts two iterators—`begin()` and `end()`—and the value to fill, which in our case is zero. This method is not only shorter but also more expressive of the action being performed.
Using `assign()`
Another method available to C++ vectors is the `assign()` member function. This function allows you to set all elements in a vector to a specific value with a single call.
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.assign(myVector.size(), 0); // Assigning all elements to zero
// Output the modified vector
for (const auto& val : myVector) {
std::cout << val << " "; // Output: 0 0 0 0 0
}
return 0;
}
Using `assign()`, you specify the size of the vector and the value to assign. This method is particularly useful when you want to change the size of an existing vector while also initializing the elements.
Using `std::vector::resize()`
The `resize()` function is another useful tool that can reset the elements of a vector. By resizing the vector, you can set all its values to zero if the new size is equal to the current size while specifying a default value.
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.resize(myVector.size(), 0); // Resetting the vector elements to zero
// Output the resized vector
for (const auto& val : myVector) {
std::cout << val << " "; // Output: 0 0 0 0 0
}
return 0;
}
Here, the vector remains the same size, but all existing values are replaced with zero. This can be particularly handy in scenarios where you may also want to change the size of the vector along with its contents.
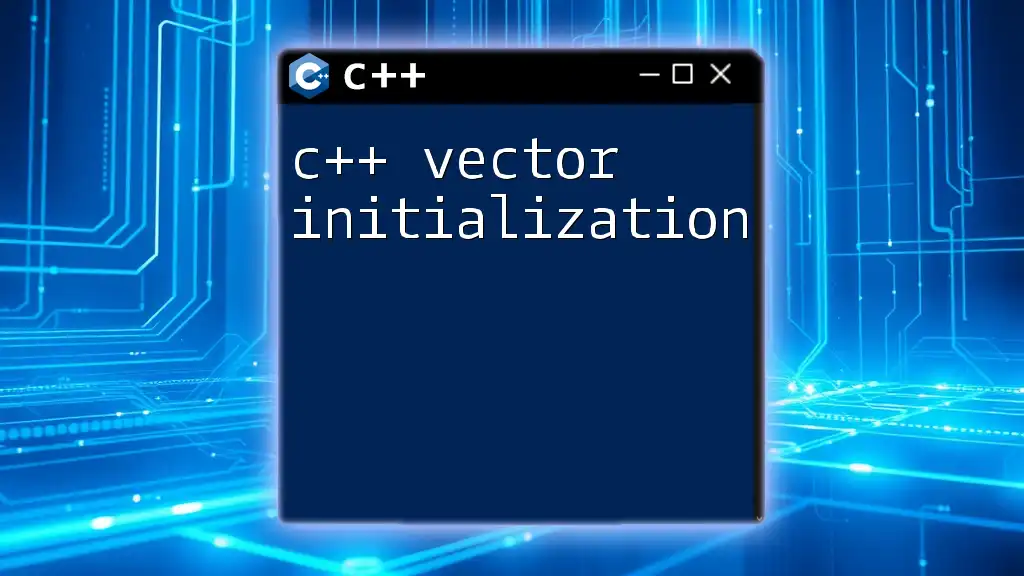
Performance Considerations
Time Complexity of Different Approaches
- For-loop: The time complexity is O(n) because we explicitly iterate through each element.
- std::fill(): The time complexity is also O(n), but it may perform faster in practice due to optimizations in the standard library.
- assign(): This method runs in O(n) time, as it needs to set each element individually.
- resize(): Similarly, resizing a vector also has a time complexity of O(n), especially if initializing new values.
In performance-critical code, understanding these complexities can help you choose the most efficient method for setting values to zero.
Practical Use Cases
Setting all values in a vector to zero can be useful in various scenarios:
- Resetting configurations or states in games or simulations.
- Clearing buffers or temporary storage before reuse to prevent uninitialized memory usage.
- Preparing an array for new computations where prior data might skew results.
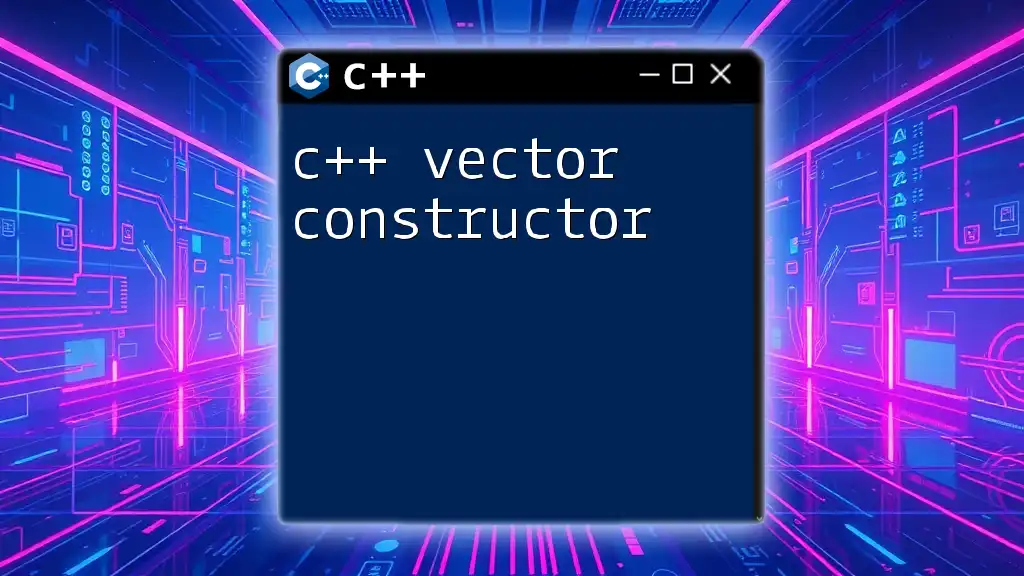
Tips for Working with Vectors in C++
Best Practices for Using Vectors
- Initialization: Always initialize your vectors. This helps prevent accessing uninitialized data.
- Capacity Management: Be aware of your vector's capacity and size, especially when handling large datasets to avoid unnecessary reallocations.
- Use Algorithms: Leverage the power of the STL (like `std::fill`) instead of writing custom loops; it leads to cleaner and more maintainable code.
Resources for Further Learning
For those looking to deepen their understanding of C++ and vectors, consider these resources:
- Online tutorials and courses that specifically focus on C++ STL.
- Books like "The C++ Programming Language" by Bjarne Stroustrup.
- C++ community forums and discussion groups for practical advice and coding examples.
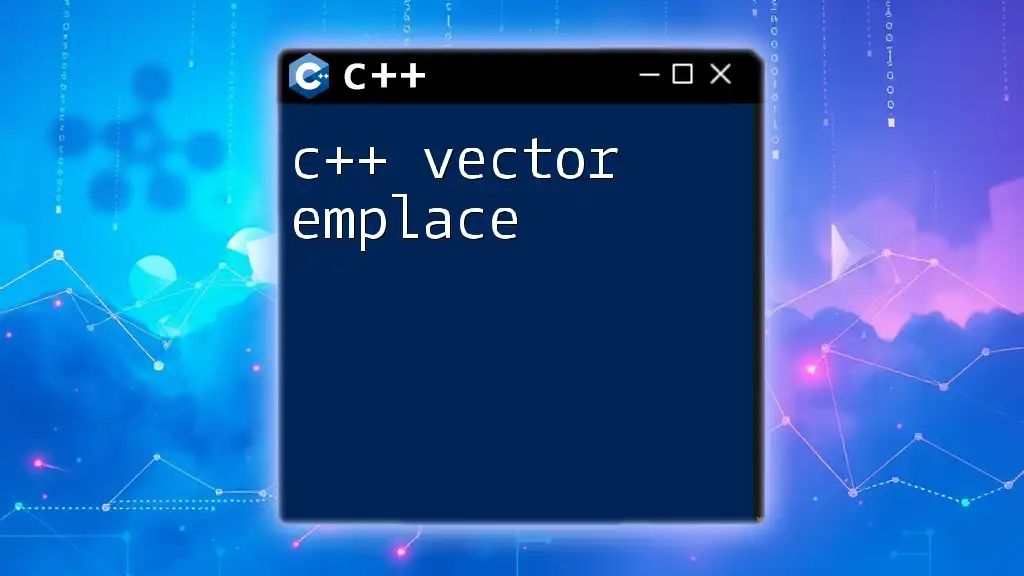
Conclusion
In this article, we've explored the various methods for c++ vector set all values to zero. From simple loops to algorithmic solutions like `std::fill()` and `assign()`, each method has its distinct advantages and use cases. Mastering these techniques not only enhances your coding efficiency but also contributes to your overall proficiency as a C++ programmer.
Experiment with these methods, and familiarize yourself with vectors to harness their power in your programming projects. Your journey with C++ vectors has just begun!