In C++, you can determine the type of an object at runtime using the `typeid` operator, which returns a reference to a type_info object representing the type of the expression.
#include <iostream>
#include <typeinfo>
class MyClass {};
int main() {
MyClass myObject;
std::cout << "The type of myObject is: " << typeid(myObject).name() << std::endl;
return 0;
}
Understanding Object Types
What is an Object Type?
In C++, an object type defines the properties and behaviors of an instance of a class, struct, or a built-in type. Every variable and object in your program belongs to a specific type, which determines how they can be used and what operations can be performed on them.
Understanding object types is crucial as it impacts the efficiency and safety of your code. For example, knowing whether a variable is of type `int`, `float`, or a user-defined class helps in enforcing type safety, which prevents unintended operations that can lead to errors.
Why Knowing the Object Type Matters
Knowing the type of an object plays a pivotal role in several aspects of programming in C++. This information:
- Ensures type safety, avoiding operations that are not defined for a specific type.
- Enables function overloading, allowing you to define multiple functions with the same name but different parameter types.
- In templates, understanding object types allows for generic programming, where you can create functions or classes that work for any data type.
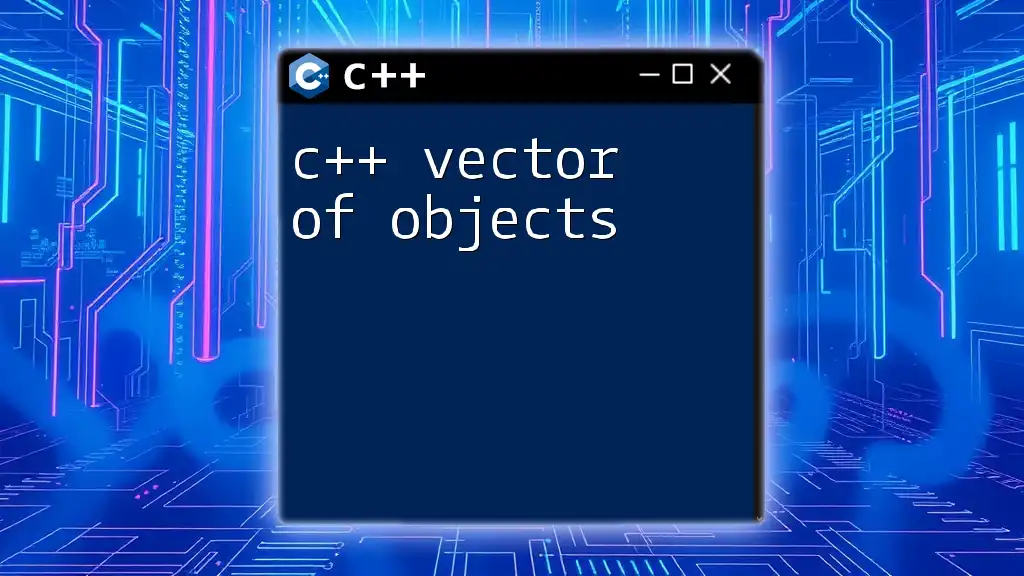
Getting the Type of an Object in C++
Using `typeid`
Overview of `typeid`
The `typeid` operator in C++ allows you to query the type of an object at runtime. It is part of the Run-Time Type Information (RTTI) system. The operator returns a `type_info` object, which holds information about the object's type.
The syntax is straightforward:
typeid(expression).name();
Here's a basic example demonstrating the use of `typeid`:
#include <iostream>
#include <typeinfo>
class Base {};
class Derived : public Base {};
int main() {
Base b;
Derived d;
std::cout << typeid(b).name() << std::endl; // Outputs type of Base
std::cout << typeid(d).name() << std::endl; // Outputs type of Derived
return 0;
}
In this example, `typeid` retrieves the types of `b` and `d`. It's worth noting that the exact string output may vary depending on the compiler used.
Limitations of `typeid`
While `typeid` is powerful, it comes with some limitations. One notable point is its behavior with polymorphism. If you use `typeid` on a base class pointer or reference to determine the derived class type, you must ensure that the class has at least one virtual function. Otherwise, it will return the type of the base class instead of the derived class.
For example:
#include <iostream>
#include <typeinfo>
class Base {
public:
virtual ~Base() {} // Adding virtual destructor
};
class Derived : public Base {};
int main() {
Base* b = new Derived();
std::cout << typeid(*b).name() << std::endl; // Correctly identifies as Derived
delete b;
return 0;
}
If the destructor was not virtual, `typeid(*b)` would return the type of `Base`, leading to possible errors in type handling.
Using `decltype`
Overview of `decltype`
The `decltype` specifier allows you to query the type of an expression at compile time, which is extremely useful in various contexts, including templates, type deduction, and function return types.
Its syntax is:
decltype(expression);
Here's a simple illustration of `decltype`:
#include <iostream>
int main() {
int x = 5;
decltype(x) y = 10; // y is of type int
std::cout << "Type of y: " << typeid(y).name() << std::endl; // Outputs type of int
return 0;
}
In this code, `decltype(x)` deduces the type of `x` (which is `int`) and applies it to declare `y`.
Use Cases for `decltype`
`decltype` shines in template programming where you want to ensure that your function or class can accept any type while maintaining its original type properties.
For instance:
template <typename T>
decltype(auto) add(T a, T b) {
return a + b; // Uses the same type as its parameters
}
The use of `decltype(auto)` in this template ensures that the return type of the `add` function matches the type of its parameters, allowing it to return `int`, `float`, or any other compatible type based on the inputs.
Combined Use of `typeid` and `decltype`
Combining `typeid` and `decltype` can enhance type deduction and clarity in complex generic programming scenarios. They work together to let you manipulate types flexibly and reliably.
Consider the following example:
#include <iostream>
#include <typeinfo>
template <typename T>
void printType(T t) {
std::cout << "Type of T: " << typeid(T).name() << std::endl;
std::cout << "Type of decltype(t): " << typeid(decltype(t)).name() << std::endl;
}
int main() {
int a = 0;
printType(a); // Outputs type information for int
return 0;
}
In this function, `printType` uses both operators to convey a clear picture of the types involved. This kind of utility is particularly valuable in code bases with extensive use of templates or polymorphic types.
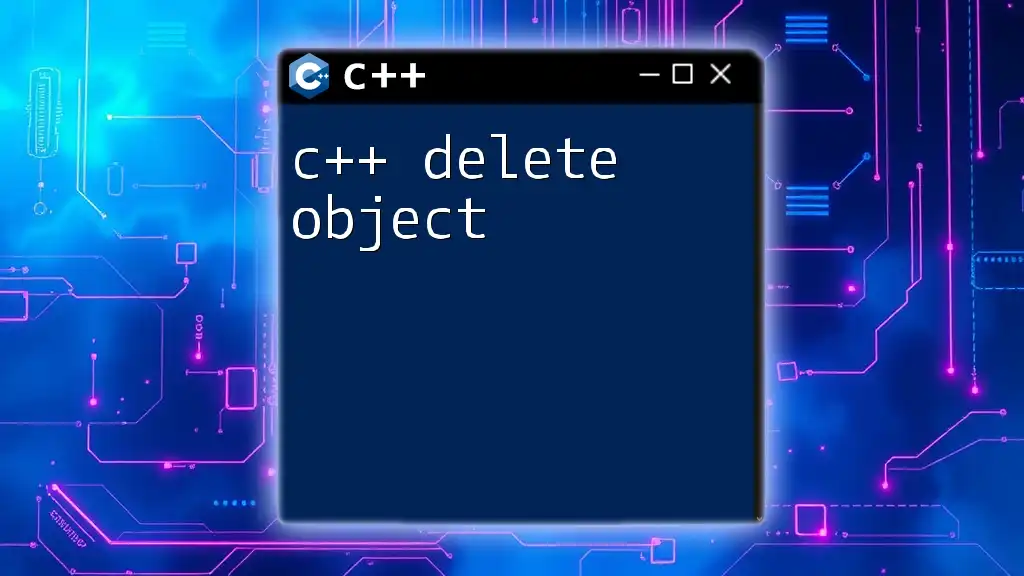
Best Practices for Getting Object Types
Avoiding Common Pitfalls
When using `typeid`, always remember to use it with polymorphic types to avoid unexpected results. Additionally, be cautious with `decltype` in complex expressions; it can yield surprising results if the original expression involves more than just a simple variable.
Enhancing Readability and Maintainability
To maintain clean and understandable code, it’s advisable to comment on types where ambiguity may arise. For example, if you are deducing a type in templates, a brief comment explaining the expected type or behavior can guide future readers.
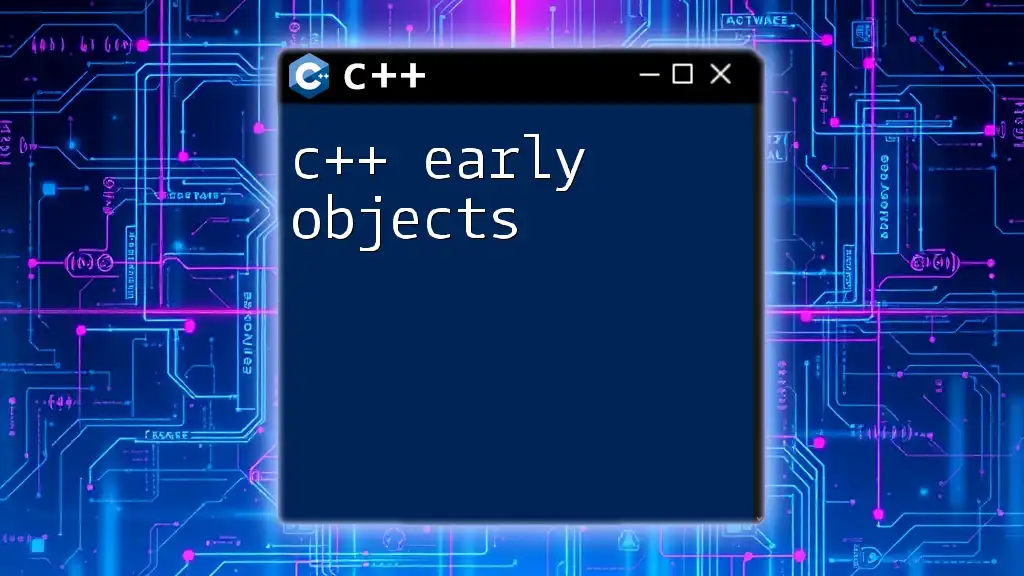
Conclusion
In conclusion, understanding how to get the type of an object in C++ using `typeid` and `decltype` is crucial for developing robust and flexible applications. Mastering these tools enables better type safety, efficient overload resolution, and clearer template programming, ultimately leading to better-maintained code. Practicing with examples and incorporating these methods into your everyday coding will help solidify your understanding and ability in managing types effectively.
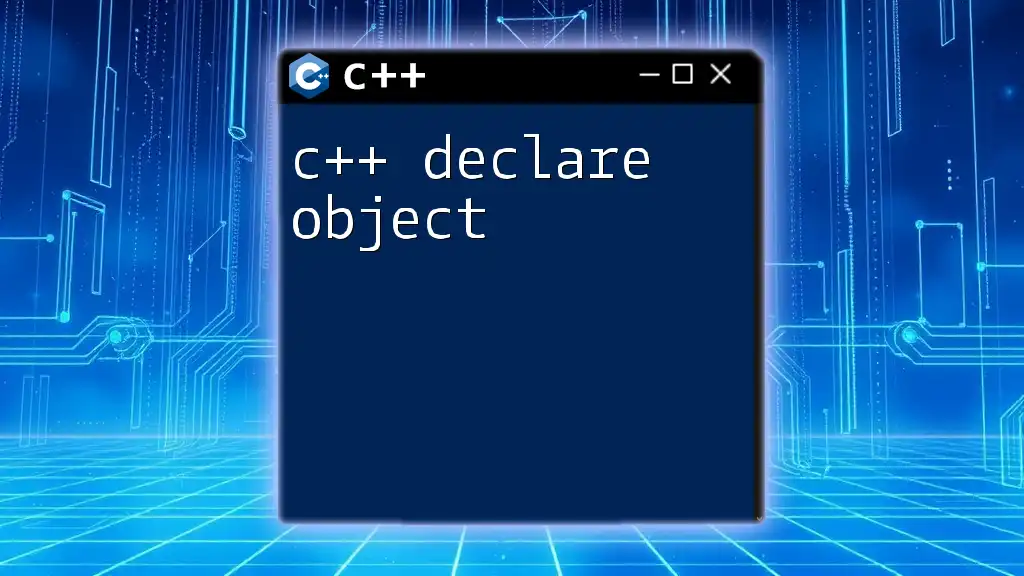
Additional Resources
- Check C++ documentation for deeper insights into RTTI and detailed explanations of `typeid` and `decltype`.
- Engage in online courses that delve into advanced C++ programming and its nuances.
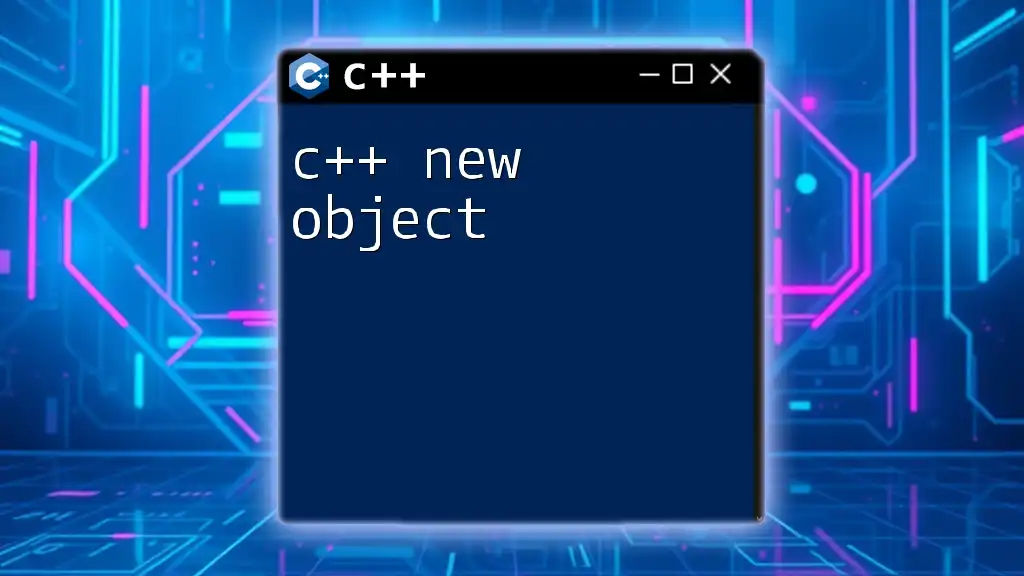
FAQs
What is `typeid` and when would I use it?
`typeid` is used for querying the runtime type of an object, particularly in scenarios dealing with inheritance and polymorphism. It helps identify actual object types in inherited classes.
Can `decltype` be used with function pointers?
Yes, `decltype` can be employed to define function pointers; it will deduce the signature of the function the pointer points to, thus maintaining type correctness.
Is it possible to get the type of an object at compile time?
Yes, using `decltype`, you can determine the type of an expression at compile time, making it highly useful in templates and generic programming workflows.