In C++, `typedef enum` is used to create a new type name for an enumeration, making it easier to reference the enum type in your code.
typedef enum Color { RED, GREEN, BLUE } Color;
Understanding Enums in C++
What is an Enum?
An enum (short for "enumeration") is a user-defined type that consists of a set of named integral constants. Enums are often used in programming to represent a collection of related values, typically for improving code readability and maintainability.
For example, here’s a simple declaration of an enum for traffic lights:
enum TrafficLight {
RED,
YELLOW,
GREEN
};
In this case, `TrafficLight` is an enum type representing three possible states of a traffic light.
Why Use Enums?
Using enums provides numerous benefits over simply using constants. One of the primary advantages is that enums enhance code readability by giving meaningful names to numeric constants. When you see `RED`, `YELLOW`, and `GREEN`, it's immediately clear that these correspond to traffic light states, whereas the numbers 0, 1, and 2 would require additional context.
Furthermore, enums help in minimizing errors by ensuring that only valid values from the set are used in the code. For example, trying to assign a value outside the defined range will result in a compile-time error.
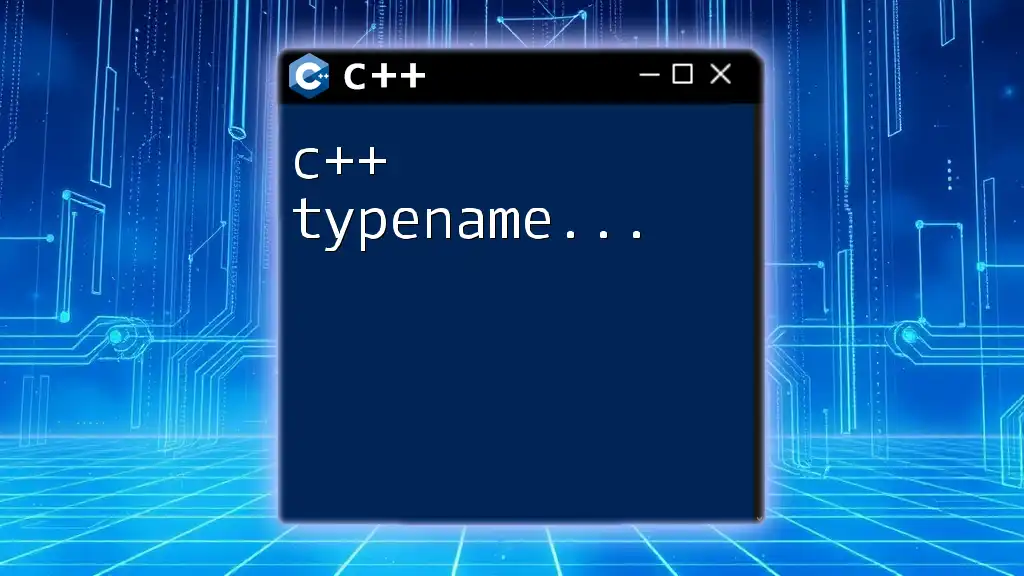
Introduction to typedef in C++
What is typedef?
The `typedef` keyword in C++ is used for creating an alias for an existing type, making code easier to read and maintain. This is particularly useful for complex data types such as pointers or structures.
Here’s an example of a simple typedef declaration:
typedef unsigned long ulong;
In this instance, `ulong` becomes an alias for `unsigned long`, allowing for cleaner and more straightforward code.
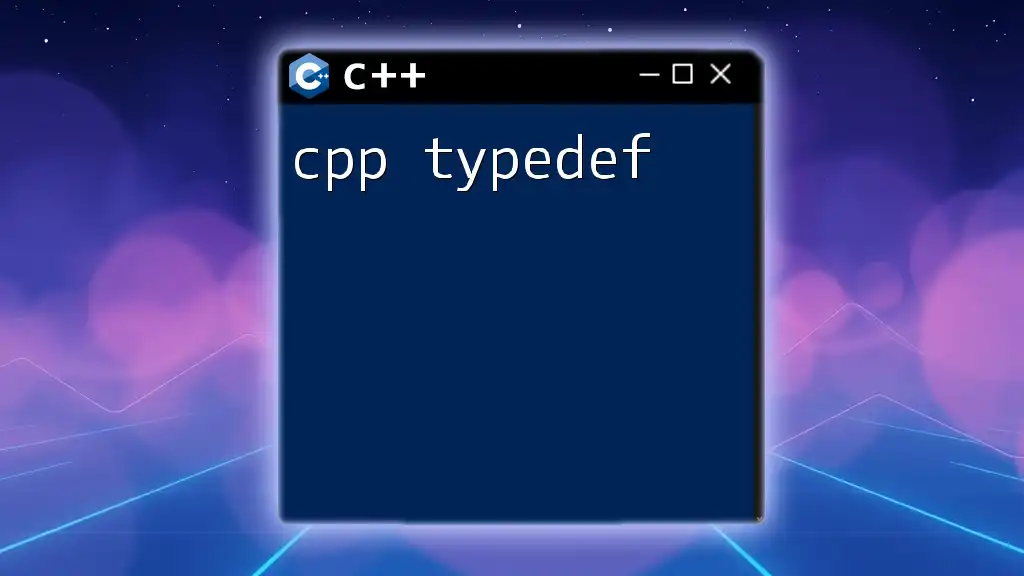
Combining typedef and enum
What Does typedef enum Mean?
The construct `typedef enum` combines the benefits of both `typedef` and `enum`. By using `typedef` with `enum`, you can create a type alias that makes it easy to refer to an enumeration type without repeatedly typing the `enum` keyword. This combination enhances code clarity and brevity.
Syntax of typedef enum
The syntax for declaring a `typedef enum` is as follows:
typedef enum {
VALUE1,
VALUE2,
VALUE3
} AliasName;
Breakdown of the syntax:
- typedef indicates we’re creating a new alias for a type.
- enum {...} defines the enumeration, where you will list the possible values.
- AliasName is the name you will use in your code to refer to this enum.
For illustrative purposes:
typedef enum {
RED,
GREEN,
BLUE
} Color;
How to Use typedef enum
To effectively utilize `typedef enum`, one can create instances of them just like any other type. Consider the example below:
typedef enum {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY
} DayOfWeek;
DayOfWeek today = FRIDAY;
if (today == FRIDAY) {
// Logic specific to Friday
}
In this code, we define an enum `DayOfWeek` to represent days of the week. The variable `today` is then assigned the value `FRIDAY`. This approach makes it easy to compare and manage days in our logic.
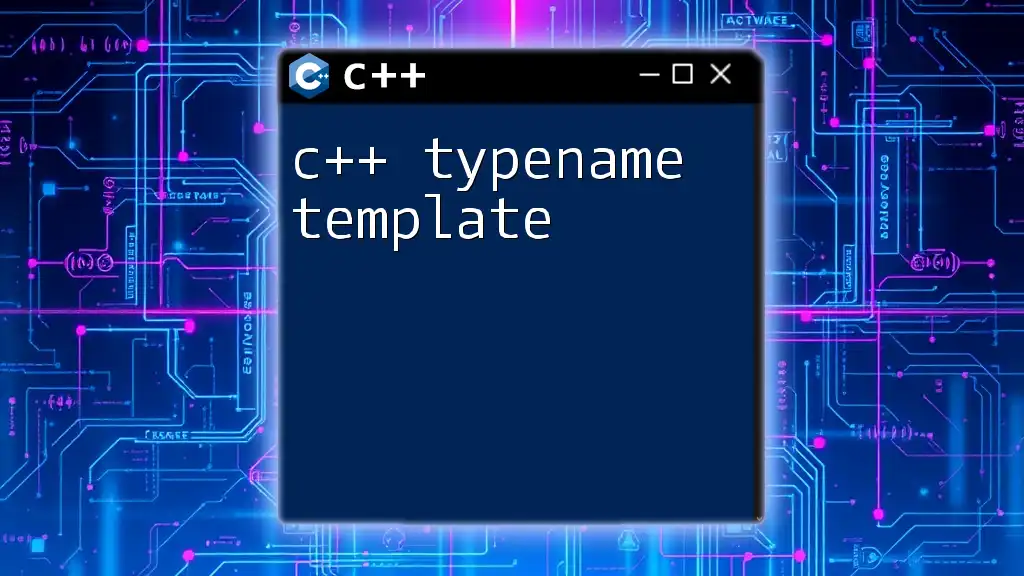
Advantages of Using typedef enum
Improved Code Clarity
Using `typedef enum` greatly improves the clarity of a program. It provides clear definitions for specific values that belong to a logical group. Code that uses clear enumerations can be easier to understand at a glance, particularly for someone new to the codebase.
For instance, instead of seeing obscure constants referred by numeric values, a developer would see meaningful identifiers such as `FRIDAY`, which conveys the intent without needing extensive comments.
Error Reduction
When using simple constants, it’s easy to mistakenly assign an incorrect value. For example, using plain integers like `1` for `FRIDAY` may lead to accidental misuse. Conversely, with `typedef enum`, you enforce proper usage as shown below:
DayOfWeek today = 5; // Error-prone
The compiler will help catch incorrect assignments if we try to assign non-allowed values to the variable of type `DayOfWeek`.
Efficiency in Code Maintenance
Maintaining code becomes significantly leaner when using `typedef enum`. If an enumeration needs changes, such as adding or removing values, it can be done in one place without having to sift through the entire codebase. This creates a single source of truth, leading to easier updates and fewer chances for failure.
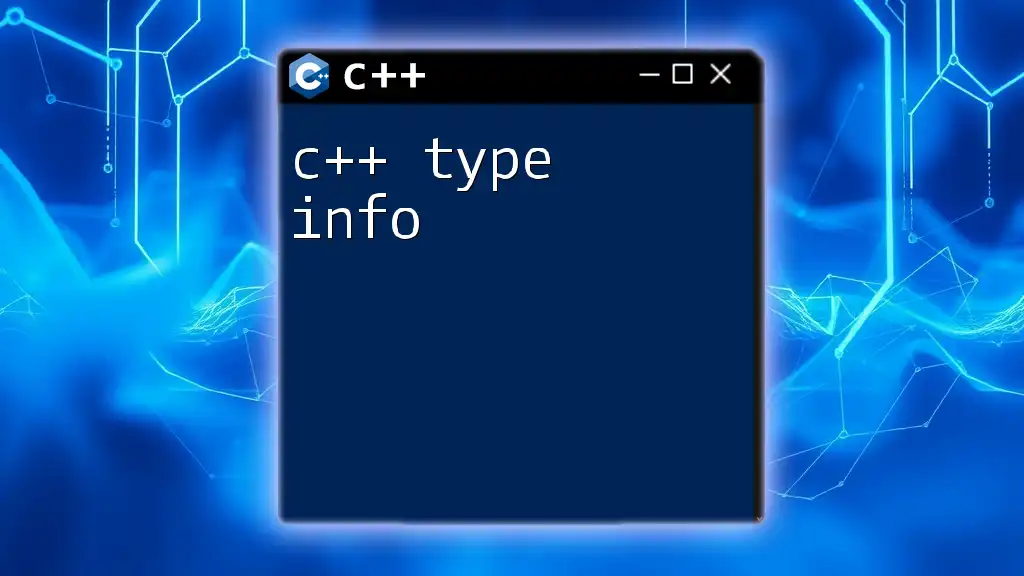
Potential Pitfalls of using typedef enum
Common Mistakes
One common mistake that beginners make is misunderstanding scope. Declaring a `typedef enum` multiple times can lead to complications. For example, redeclaring the same type in multiple files can lead to conflicts if not properly managed.
typedef enum {
CAT,
DOG
} Animal; // Declaring Animal twice in different files will cause an error
Scope Issues
Understanding the scope of enums can also pose challenges. When you declare an enum within a function scope, it isn't visible outside of it unless explicitly declared globally. This can lead to confusion if developers expect enumerations to be globally available when they are not.
Here is an illustration of scope issues:
typedef enum {
APPLE,
ORANGE
} Fruit;
// This Fruit enum won't be accessible outside the scope it was declared.
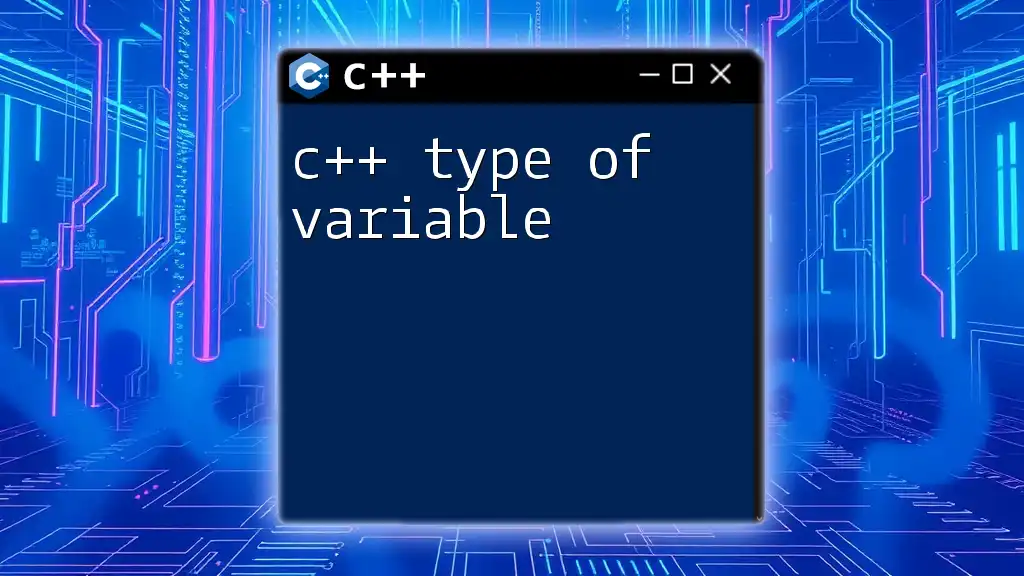
Best Practices for using typedef enum
Naming Conventions
When defining `typedef enum`, adopting consistent naming conventions enhances readability and maintainability. It’s advisable to use PascalCase for enum names and uppercase letters for the enumeration values, which clearly distinguishes them from regular variables.
For example:
typedef enum {
RED,
GREEN,
BLUE
} Color;
When to Utilize
Determining when to use `typedef enum` comes down to understanding the cohesion of related constants. If you find yourself using a group of constants that logically belong together, it is an ideal candidate for an enumeration.
For instance, if you frequently reference the days of the week, implementing a `DayOfWeek` enum is suitable. Avoid using enums for unrelated values, as this could lead to confusion and misuse.
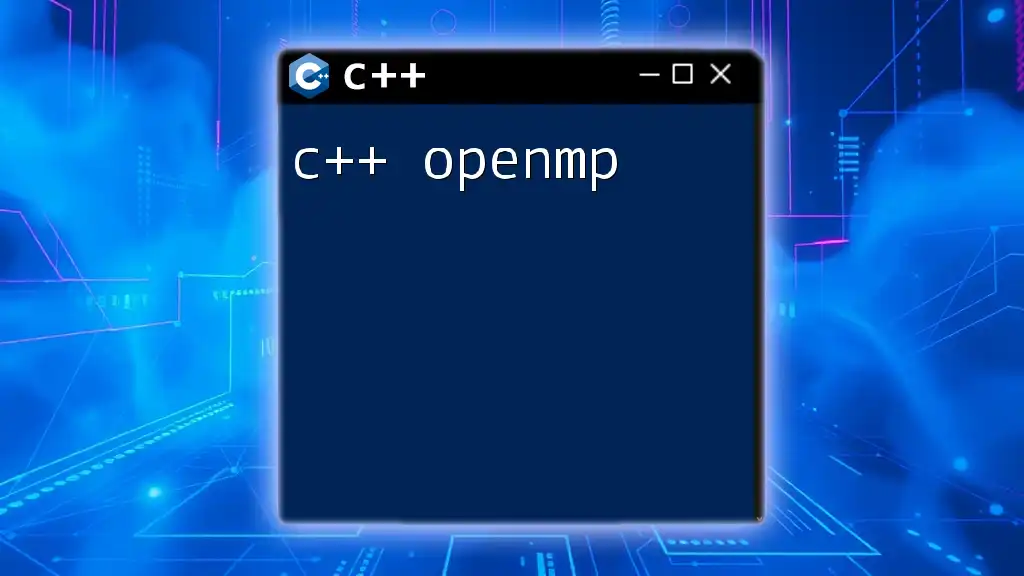
Conclusion
In summary, c++ typedef enum offers a robust method for defining enumerations within your code, enhancing readability, maintainability, and reducing errors. By leveraging this construct, you can cultivate cleaner, more understandable code, which is essential for successful software development. Adopting best practices such as coherent naming conventions and judiciously using enums can lead to a more efficient coding experience.
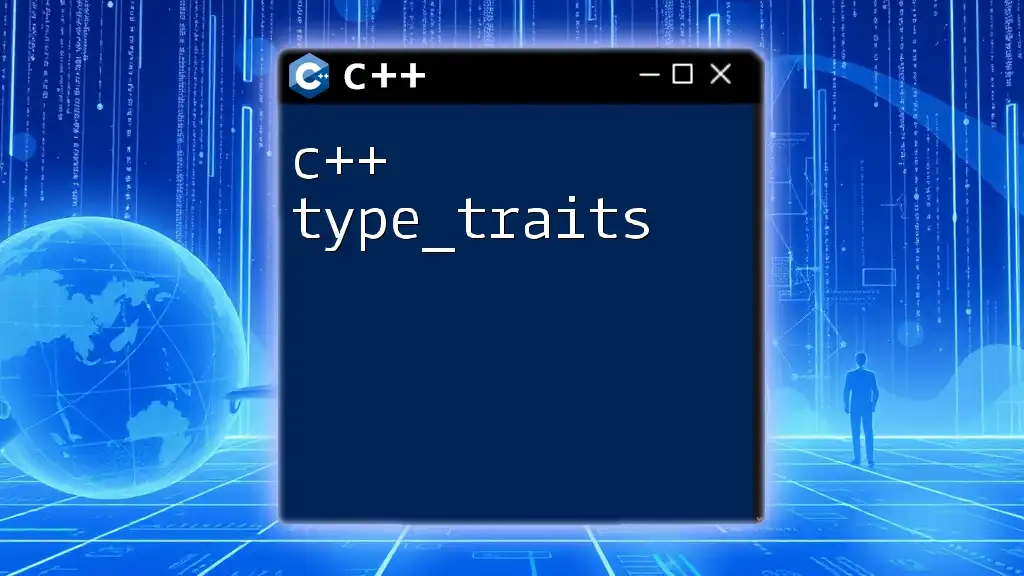
Additional Resources
For additional learning on enums and C++ programming, consider exploring reputable books, instructional websites, and video tutorials that delve deeper into the nuances of C++ development.
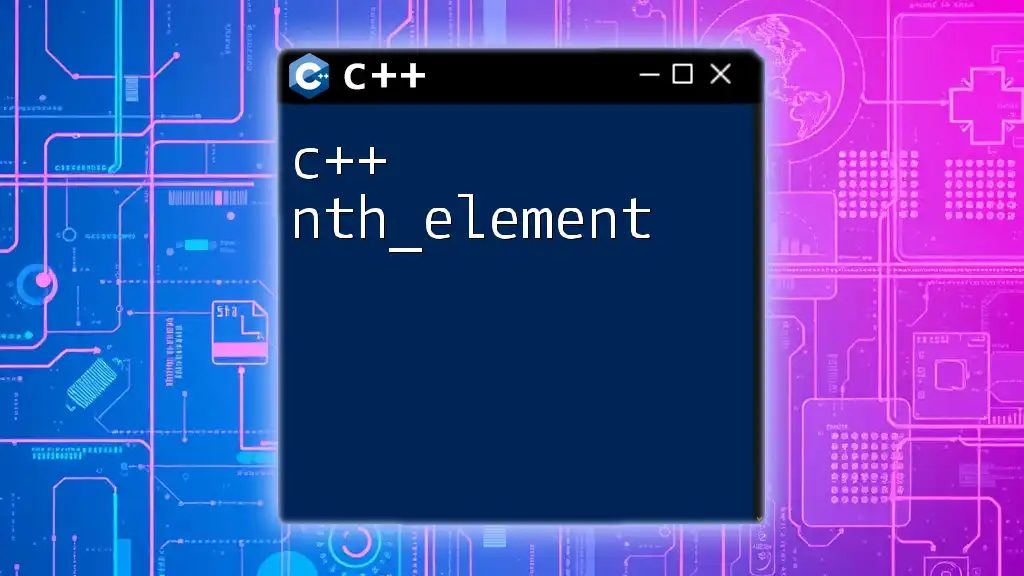
Call to Action
We encourage you to implement `typedef enum` in your projects and experience firsthand how it improves your coding workflow. Share your experiences, questions, or insights on this topic in the comments below!