In C++, the "identifier not found" error occurs when the compiler cannot recognize a variable, function, or class name, often due to typos or not having declared the identifier before use.
Here's a code snippet that demonstrates this error:
#include <iostream>
int main() {
std::cout << "Value: " << x << std::endl; // 'x' is not declared, which will cause an "identifier not found" error
return 0;
}
Understanding Identifiers in C++
What is an Identifier?
An identifier in C++ is a name used to identify variables, functions, classes, and other user-defined items. Identifiers serve as a way to refer to these elements when writing code. They must be chosen carefully, following specific rules and conventions to ensure clarity and to avoid potential errors.
Examples of Identifiers:
- Correct Identifiers: `myVariable`, `calculateSum()`
- Incorrect Identifiers: `2ndVariable` (can't start with a digit), `my-variable` (invalid character: hyphen)
Rules for Creating Identifiers in C++
When creating identifiers in C++, there are several rules to follow:
- Naming Conventions: Identifiers can include letters (uppercase and lowercase), digits, and the underscore character (_). However, they cannot start with a digit.
- Length and Case Sensitivity: Identifiers can be of any length and are case-sensitive. This means that `myVariable` and `myvariable` are considered different identifiers.
- Reserved Words: You cannot use reserved keywords (such as `int`, `return`, etc.) as identifiers, as they have specific meanings in C++.
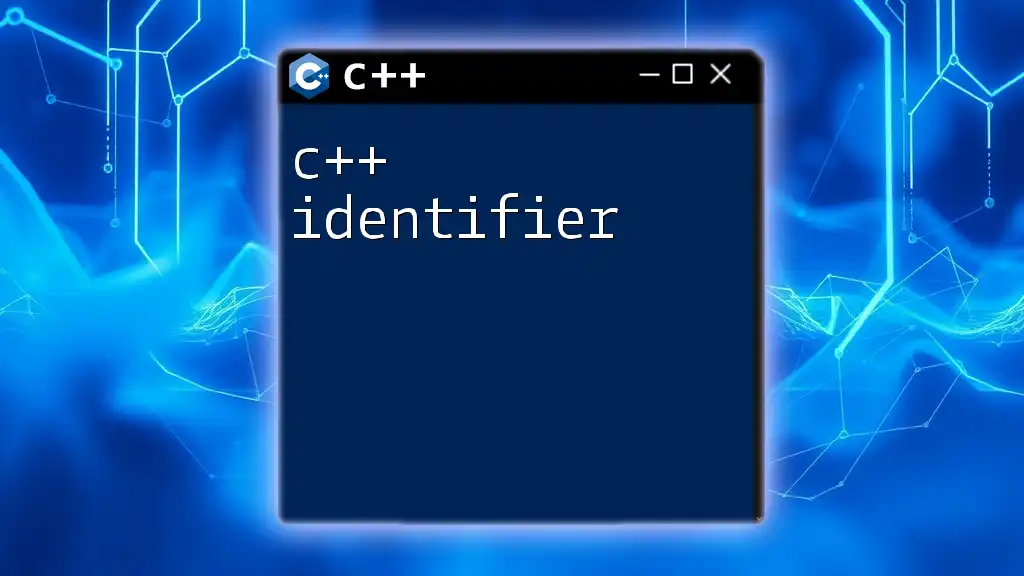
The "Identifier Not Found" Error Explained
What Does "Identifier Not Found" Mean?
The error "identifier not found" occurs when the C++ compiler is unable to resolve a name you have used in your code. This means that it does not recognize the identifier you referenced, which can lead to compilation failures.
Common Causes of the "Identifier Not Found" Error
Typographical Errors
A simple typographical mistake can lead to this error. If you misspell an identifier when referencing it, the compiler cannot find it.
Code Snippet:
int count;
std::cout << coint; // Error: "identifier not found"
Here, `coint` is a misspelling of `count`, which causes the compiler to throw the error.
Scope Issues
Identifying variables correctly based on their scope is crucial. If you try to access a variable that is not in the current scope (especially in functions), you'll encounter this error.
Code Snippet:
void myFunction() {
int localVar = 10;
}
std::cout << localVar; // Error: "identifier not found"
In this example, `localVar` is defined within `myFunction()`, making it inaccessible from outside this function, leading to an identifier not found error.
Uninitialized or Undefined Identifiers
If you attempt to use an identifier that has been declared but not defined, you'll also see this error.
Code Snippet:
extern int globalVar;
std::cout << globalVar; // Error: "identifier not found" if globalVar is not defined
In this example, `globalVar` is declared but never defined, resulting in the error when the program is run.
Missing Include Directives
Not including necessary header files can also result in the error when you try to use standard library identifiers.
Code Snippet:
// Missing #include <iostream>
std::cout << "Hello World"; // Error: "identifier not found"
In this case, because the `<iostream>` header is not included, `cout` is not recognized, leading to an identifier not found error.
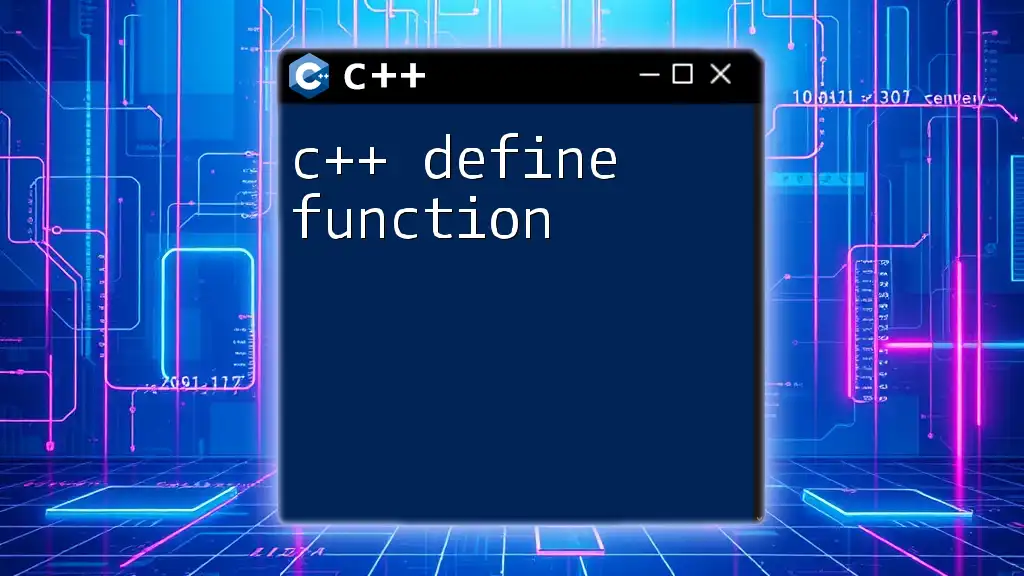
Debugging the "Identifier Not Found" Error
Tools and Techniques
To debug the "identifier not found" error effectively, utilize the compiler's error messages. They often point out the exact line and context in which the error occurs. Additionally, modern IDEs (Integrated Development Environments) provide debugging tools that highlight errors in real-time, making them easier to identify and resolve.
Best Practices to Avoid the Error
To minimize the chances of encountering the "identifier not found" error:
-
Consistent Naming Conventions: Adopting a consistent naming convention helps maintain clarity and reduces the likelihood of typographical errors.
-
Code Reviews and Pair Programming: Regularly reviewing code with peers can spotlight potential mistakes before compilation errors arise.
-
Documenting Code and Variable Usage: Keeping comments and documentation up to date ensures that the purpose and scope of identifiers are clear, helping to avoid confusion.
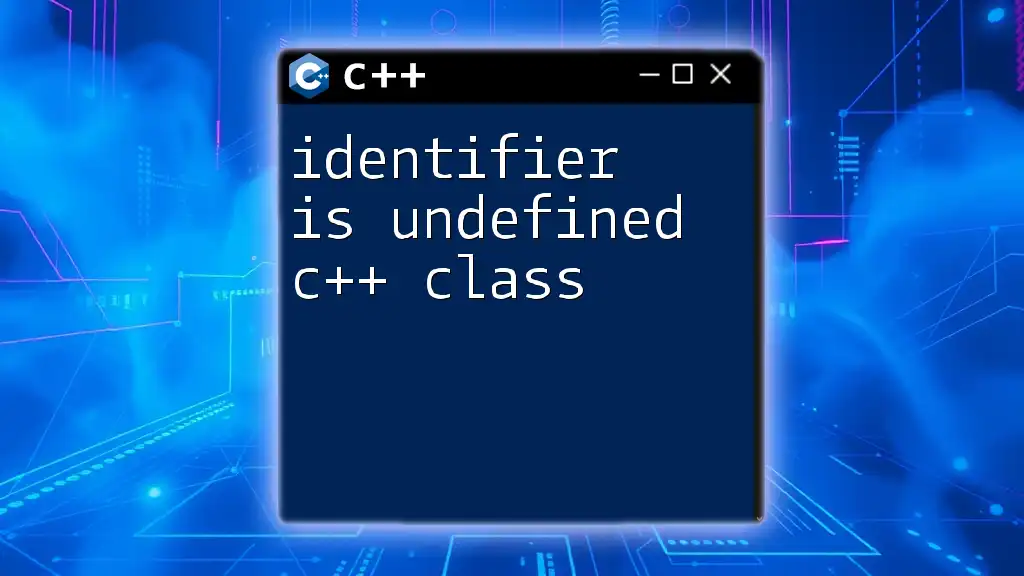
Real-World Example of Resolving "Identifier Not Found"
Step-by-Step Walkthrough
Let's consider a scenario where you encounter the "identifier not found" error, and resolve it step by step.
Original Code with Errors:
#include <iostream>
void displayValue() {
std::cout << value; // Error: "identifier not found"
}
int main() {
int value = 10;
displayValue();
return 0;
}
Analysis: In this code snippet, `value` is declared within the `main` function, making it local to that function. When `displayValue()` tries to access `value`, it results in the "identifier not found" error.
Revised Code to Fix the Error:
#include <iostream>
int value = 10; // Moved declaration to global scope
void displayValue() {
std::cout << value; // Now works
}
int main() {
displayValue();
return 0;
}
Resolution: By moving the declaration of `value` to the global scope, we ensure that it is accessible to both the `main` function and `displayValue()`, thus resolving the error.
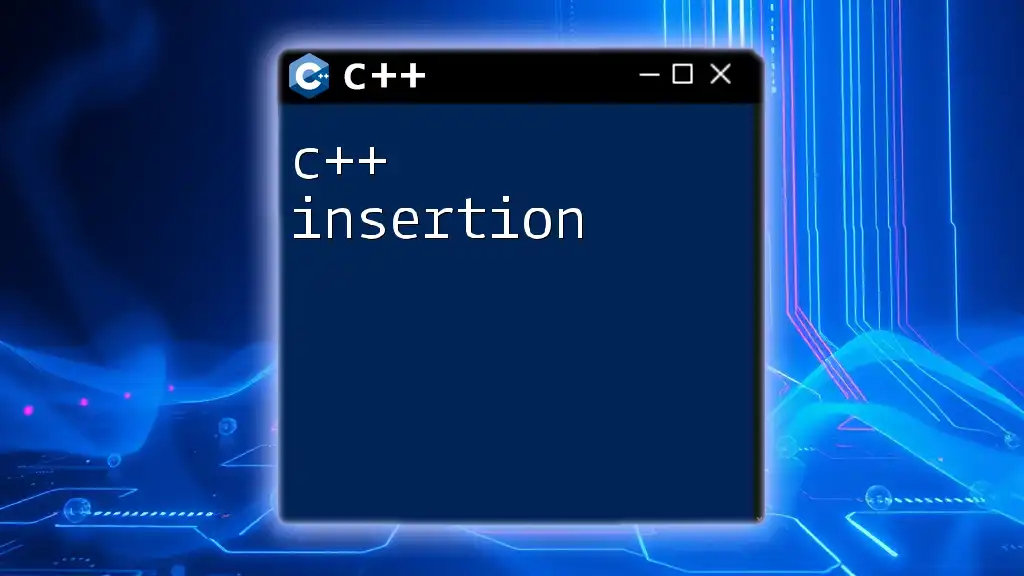
Conclusion
Understanding the nuances of identifiers in C++ and the common error of "identifier not found" is vital for every programmer. By identifying typographical errors, addressing scope issues, initializing identifiers correctly, and ensuring the inclusion of necessary headers, developers can effectively mitigate this error. Consistent naming conventions, thorough documentation, and utilizing tools for debugging will further enhance code quality in C++ programming. By practicing these strategies, you will build a strong foundation in C++ and become adept at handling and resolving errors efficiently.
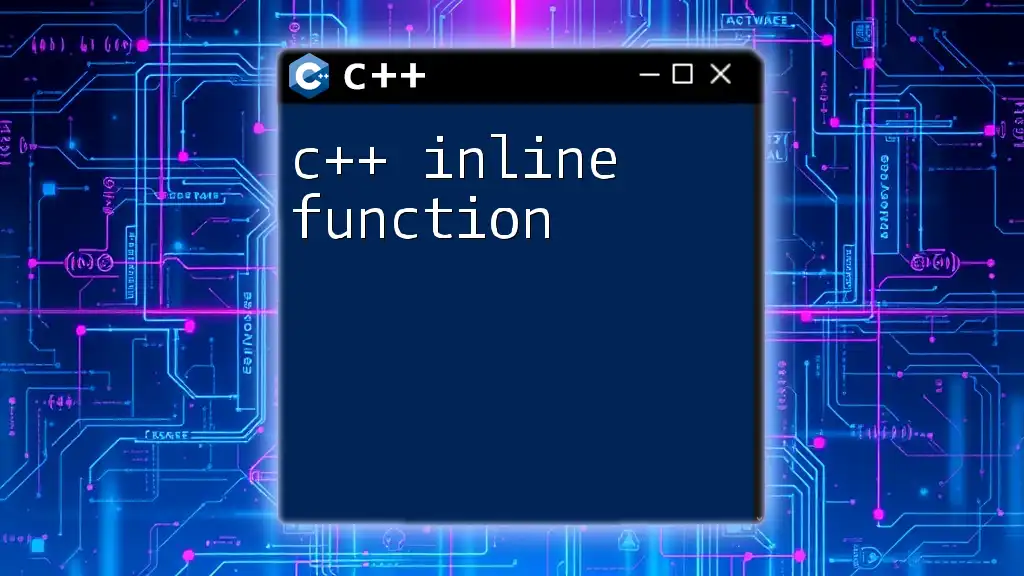
Additional Resources
For further reading, consider exploring C++ tutorials, official documentation, and practice exercises that can help solidify your understanding of identifiers and error handling in C++. By investing time in learning and practicing, you will gain a more profound command over C++ and its intricacies.