In C++, the error "identifier is undefined" occurs when the compiler encounters a name (such as a variable or function) that it does not recognize, often due to missing declarations, namespaces, or incorrect spelling.
Here’s a code snippet illustrating this error:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass obj;
obj.show(); // Error: 'show' is undefined
return 0;
}
Understanding the Concept of Identifiers in C++
What is an Identifier?
An identifier is a name used in a program to identify a variable, function, class, or any other user-defined item. In C++, identifiers are essential because they provide a way to reference the various elements in the code, allowing developers to create understandable and maintainable programs.
Types of Identifiers
In C++, identifiers can be classified into several types:
- Variable names: Used for storing data.
- Function names: Represent actions that the program can perform.
- Class names: Define the blueprint for creating objects.
- Namespace names: Allow for organizing code into logical groups.
Understanding how identifiers work is crucial for preventing errors, including the "identifier is undefined c++ class" error.
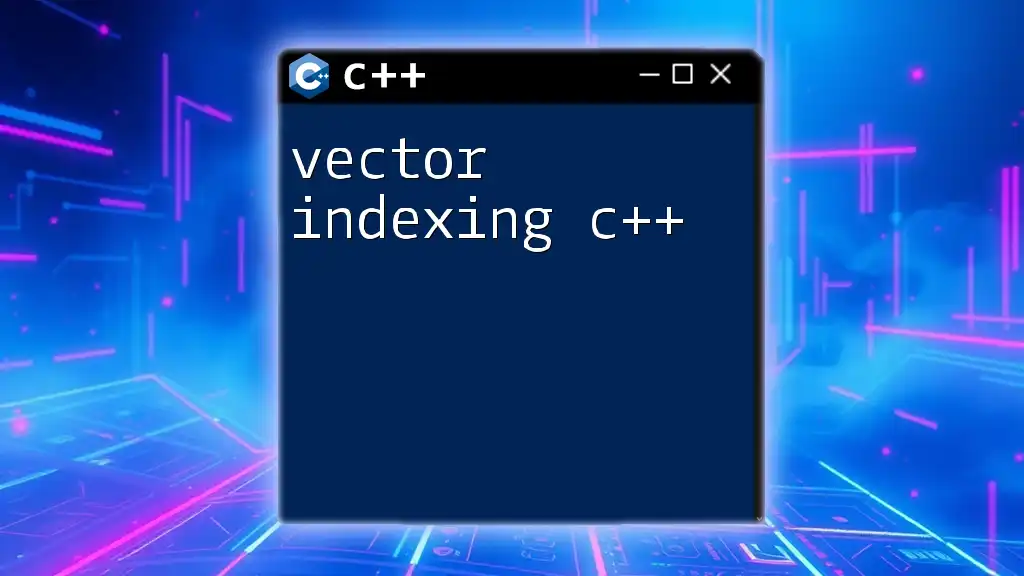
Common Causes of "Identifier is Undefined" Error
Missing Header Files
Header files in C++ contain declarations of functions, classes, variables, and other constructs. If you forget to include a necessary header file, your program may throw an error stating that an identifier is undefined.
For example, if we try to use the string class without including its header:
#include <iostream>
int main() {
std::string name; // "identifier is undefined" error occurs.
std::cout << name << std::endl;
return 0;
}
By including the correct header file with `#include <string>`, the issue can be resolved.
Scope Issues
The concept of scope refers to the accessibility of identifiers in a program. If an identifier is defined in a specific scope (like inside a function), it cannot be accessed outside that scope. This can lead to the "identifier is undefined c++ class" error.
Consider this example:
#include <iostream>
void show() {
int number = 42;
}
int main() {
std::cout << number; // Error: 'number' is undefined here
return 0;
}
To resolve this, make sure that the variable's scope covers the area where it is accessed.
Typographical Errors
Typos are a common source of errors. If an identifier is misspelled anywhere in the code, the compiler will not recognize it, resulting in an "identifier is undefined c++ class" error.
For instance:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass myClass;
myClas.display(); // Error: 'myClas' is undefined
return 0;
}
Here, fixing the typo (`myClass.display();`) resolves the issue.
Class Declaration and Definition Issues
In C++, it is essential to distinguish between class declarations and definitions. If only a declaration exists and you attempt to create an object or call a method before the class is fully defined, you will encounter an undefined identifier error.
For example:
#include <iostream>
class MyClass; // Forward declaration
int main() {
MyClass obj; // Error: 'MyClass' is undefined
return 0;
}
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
To avoid this error, ensure that the class is fully defined before its use.
Namespace Conflicts
Namespaces allow developers to group related code and avoid naming conflicts. However, if identifiers from different namespaces conflict and are not handled properly, you may face the "identifier is undefined c++ class" error.
For example:
#include <iostream>
namespace A {
class MyClass {
public:
void display() {
std::cout << "A's MyClass" << std::endl;
}
};
}
namespace B {
class MyClass { // Conflict with A::MyClass
public:
void display() {
std::cout << "B's MyClass" << std::endl;
}
};
}
int main() {
A::MyClass objA;
B::MyClass objB;
objA.display();
objB.display(); // Here, names are resolved by namespaces
return 0;
}
To avoid conflicts, ensure you use the correct namespace or undefine ambiguities by fully qualifying your class names.
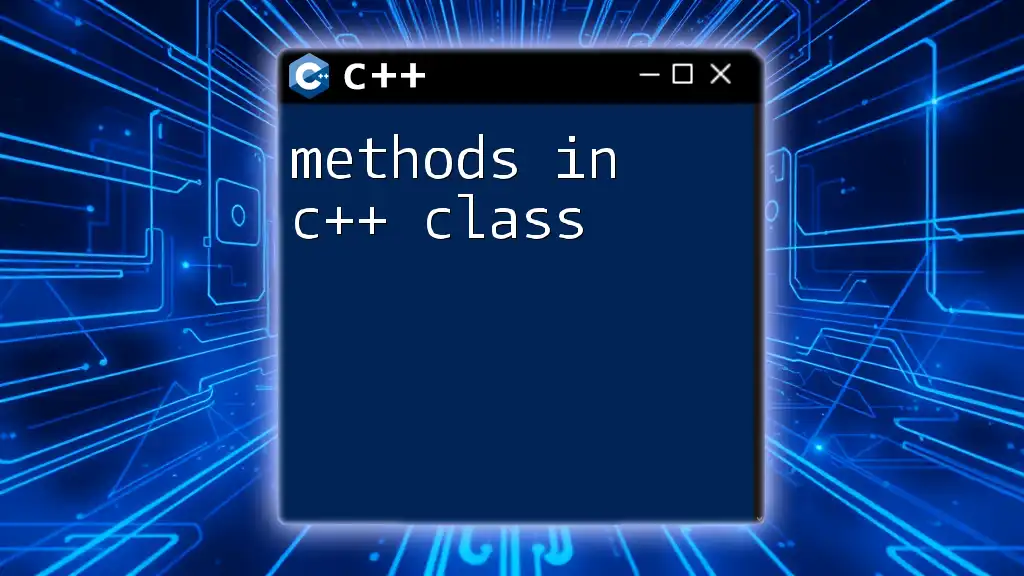
How to Resolve the "Identifier is Undefined" Error
Step-by-Step Debugging Process
-
Check for Typos: Even a small typo can lead to significant complications. Always verify the spelling of identifiers to ensure they match throughout your code.
-
Include Necessary Header Files: Confirm that all required header files are included at the top of your source file. If using STL components, ensure their headers are present.
-
Check Scope: Be aware of the scope of your identifiers. If an identifier is defined within a certain block, it will not be recognized outside it.
-
Review Class Declarations and Definitions: Ensure that all classes are declared and defined properly before their use. Forward declarations can prevent errors but must be managed carefully.
-
Use Namespaces Correctly: Frequently verify which namespaces are being used and ensure the identifiers are referenced correctly to avoid conflicts.
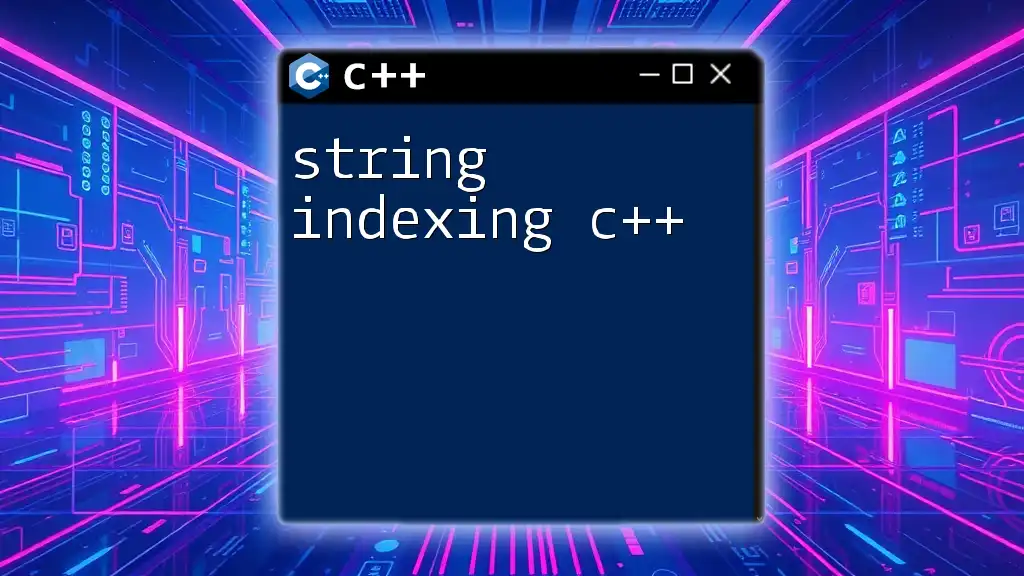
Best Practices in C++ Programming
Use Meaningful Identifiers
Choosing descriptive names for variables, functions, and classes can significantly enhance code readability and maintainability. Instead of using vague names like `x`, `y`, or `var`, opt for more explicit names such as `studentGrade` or `calculateSum`.
Regular Code Review
Implementing a code review process helps catch errors early, including those related to undefined identifiers. Peer review can provide fresh perspectives on identifying potential issues before they escalate.
Leverage Integrated Development Environments (IDEs)
Consider using an IDE that provides tools for debugging, syntax highlighting, error detection, and even code suggestions. These features can help you spot issues related to undefined identifiers quickly and efficiently.
Commenting Your Code
Adding meaningful comments can clarify the purpose of your identifiers, making it easier to understand the code logic and preventing the misuse of identifiers.
// This function calculates the area of a rectangle
double calculateArea(double length, double width) {
return length * width; // Return area
}
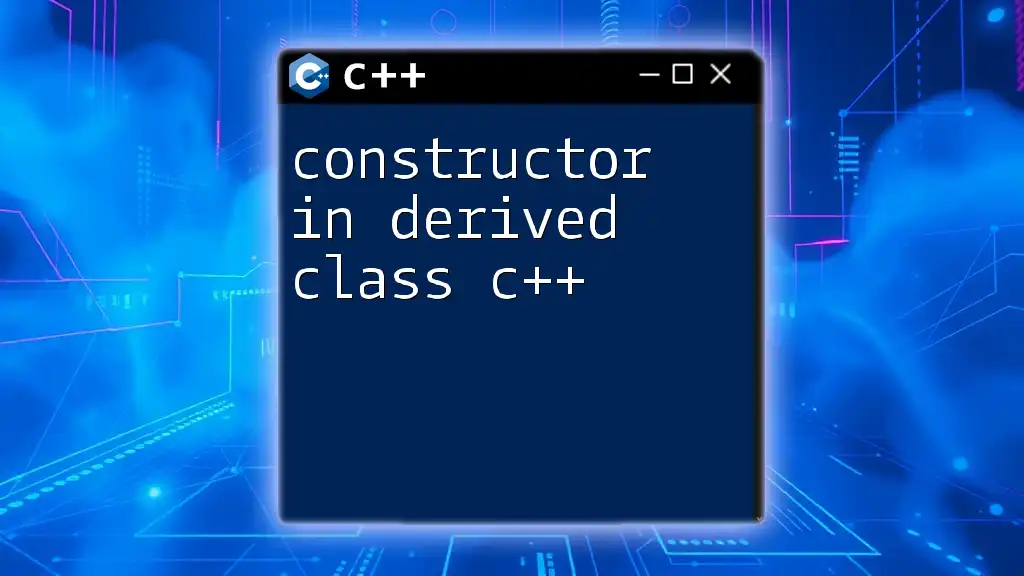
Conclusion
Understanding why an identifier is undefined in a C++ class is pivotal for debugging and writing effective code. By following the outlined solutions, you can avoid and resolve these issues, enhancing code quality and developer productivity. Emphasizing best practices will foster a more efficient programming environment, enabling you to tackle C++ challenges with confidence.