C++ Runtime Type Information (RTTI) enables the identification of an object's type at runtime, typically through the use of the `typeid` operator and dynamic casting.
Here’s a code snippet demonstrating its use:
#include <iostream>
#include <typeinfo>
class Base {
virtual void dummy() {}
};
class Derived : public Base {};
int main() {
Base* b = new Derived();
if (Derived* d = dynamic_cast<Derived*>(b)) {
std::cout << "b is of type: " << typeid(*d).name() << std::endl;
} else {
std::cout << "b is not of type Derived." << std::endl;
}
delete b;
return 0;
}
Understanding RTTI in C++
What is RTTI?
C++ Runtime Type Information (RTTI) is a feature of the C++ programming language that allows the type of an object to be determined during runtime. This stands in contrast to compile-time type information, which is resolved when the code is compiled. RTTI is essential for scenarios involving polymorphism, where you might not know the exact type of an object until the program is executed.
Key Components of RTTI
RTTI consists mainly of two components: type identification and dynamic casting.
-
Type Identification: This is the process of determining the actual type of an object at runtime. This allows developers to ascertain an object's type even if they are working with a base class pointer or reference.
-
Dynamic Casting: This is a safe method to convert a base class pointer/reference into a derived class pointer/reference. If the conversion is invalid, `dynamic_cast` will return `nullptr`, avoiding potential errors and undefined behavior.
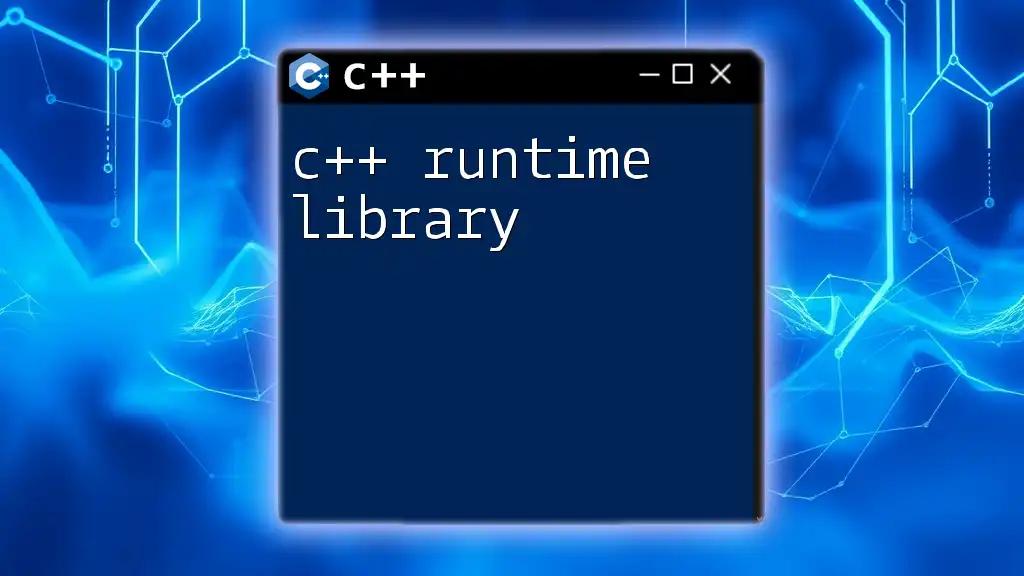
Enabling RTTI in C++
Compiler Options for RTTI
To utilize RTTI in your C++ programs, it's crucial that RTTI is enabled in your compiler settings. Most modern C++ compilers, including GCC and MSVC, have RTTI enabled by default. However, if you want to ensure that it's active, you may find specific flags:
- GCC: Use the `-frtti` flag.
- MSVC: RTTI is enabled by default, but you can toggle it in properties under "C/C++ > Code Generation > Enable Run-Time Type Info".
Requirements for RTTI to Work
For RTTI to be effective, certain conditions must be met:
-
The class must have at least one virtual function. This establishes the necessary infrastructure for RTTI to link type information with the intended type.
-
The class must adhere to inheritance. RTTI works best in class hierarchies where base and derived classes are present. Without these, RTTI can’t determine the type of the object dynamically.

How to Use RTTI in C++
Using `typeid`
Introduction to `typeid`
The `typeid` operator is a powerful tool in RTTI. It is used to retrieve the type information of an object or a type. Understanding the syntax and various use cases enhances its practicality.
Examples of `typeid`
Here’s a code snippet demonstrating how to use the `typeid` operator:
#include <iostream>
#include <typeinfo>
class Base {
public:
virtual ~Base() {}
};
class Derived : public Base {};
int main() {
Base* b = new Derived();
std::cout << typeid(*b).name() << std::endl; // Outputs the type of object
delete b;
return 0;
}
In the example above, `typeid(*b).name()` will give you the name of the actual derived class object `b` is pointing to, showcasing how RTTI assists in identifying the object’s type during runtime.
Implementing Dynamic Cast
The `dynamic_cast` operator
The `dynamic_cast` keyword plays a pivotal role in safely converting pointers or references of a base class to a derived class. It comes with built-in runtime checks to ensure that the conversion is valid.
Using `dynamic_cast` in Practice
Here’s how to implement `dynamic_cast` effectively:
#include <iostream>
class Base {
public:
virtual ~Base() {}
};
class Derived : public Base {};
int main() {
Base* b = new Derived();
Derived* d = dynamic_cast<Derived*>(b);
if (d) {
std::cout << "The cast was successful!" << std::endl;
} else {
std::cout << "The cast failed!" << std::endl;
}
delete b;
return 0;
}
In this code, the `dynamic_cast` checks whether `b` can safely be cast to `Derived*`. If the cast is successful, it continues; otherwise, you avoid potential runtime errors.
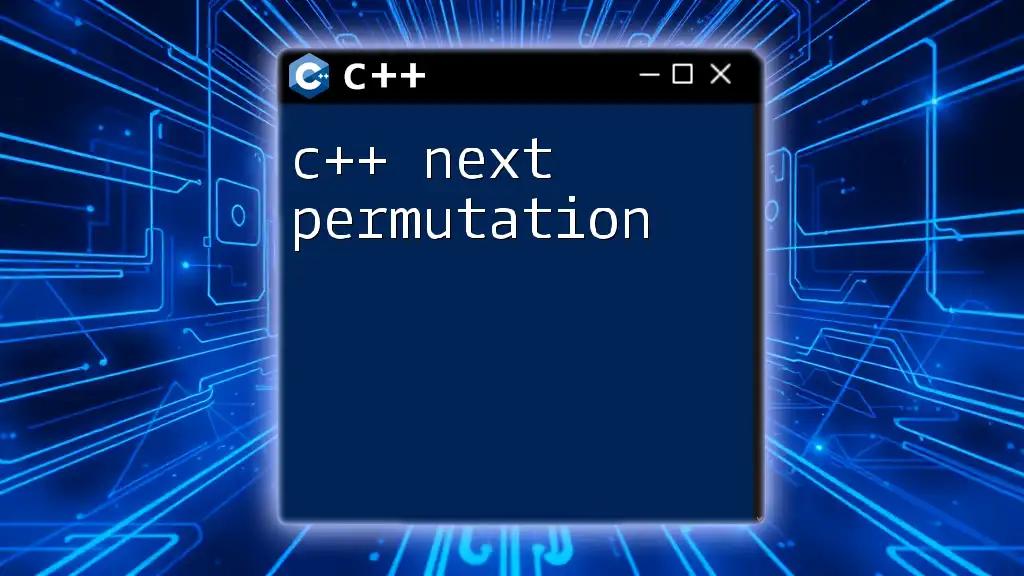
Best Practices for RTTI
When to Use RTTI
Using RTTI can be extremely beneficial when dealing with complex class hierarchies or when writing generic code that needs to handle various derived types. Common scenarios include plugin designs, factory patterns, and event handling systems.
Alternatives to RTTI
While RTTI is invaluable, it is not always necessary or optimal. Alternatives such as static polymorphism and template metaprogramming can often be used, especially when type determination needs to be resolved at compile time, ensuring faster performance and type safety.

Common Pitfalls and Misunderstandings
Misuse of RTTI
One common mistake is overusing RTTI in situations where simpler solutions exist. Relying on RTTI can lead to increased coupling and decreased maintainability. It's crucial to evaluate whether polymorphism or `dynamic_cast` is genuinely necessary.
Performance Considerations
Although RTTI is powerful, it can incur a non-negligible performance overhead. Use it cautiously in performance-critical paths. Always consider whether an alternative approach can provide better efficiency without the need for RTTI.

Conclusion: Embracing RTTI in Your C++ Projects
C++ Runtime Type Information is a robust feature that enhances the flexibility of your code through dynamic type identification and safe conversions. As you embrace RTTI in your projects, keep in mind its best practices, common pitfalls, and performance considerations to leverage its capabilities effectively.

Additional Resources
To further explore C++ runtime type information, consider diving into reputable book recommendations such as "Effective C++" by Scott Meyers, and engaging with online communities focused on C++ programming. Additional documentation and tutorials from the C++ Standard Library are invaluable resources for enhancing your understanding of RTTI and its applications.