The `push_back` function in C++ is used to add an element to the end of a vector, dynamically increasing its size.
Here's an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(10);
numbers.push_back(20);
numbers.push_back(30);
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding `push_back`
What is `push_back`?
The `push_back` function is a member of the C++ Standard Template Library (STL) vector class. This function allows you to add elements to the end of a vector, which serves as a dynamic array. By using `push_back`, you can easily manage a collection of objects without worrying about the allocation and deallocation of memory.
The Role of `push_back` in C++ STL
In the C++ STL, vectors are one of the most commonly used container types due to their ability to store a growing collection of elements. The `push_back` function plays a critical role in maintaining these collections by enabling dynamic resizing whenever new items are added.
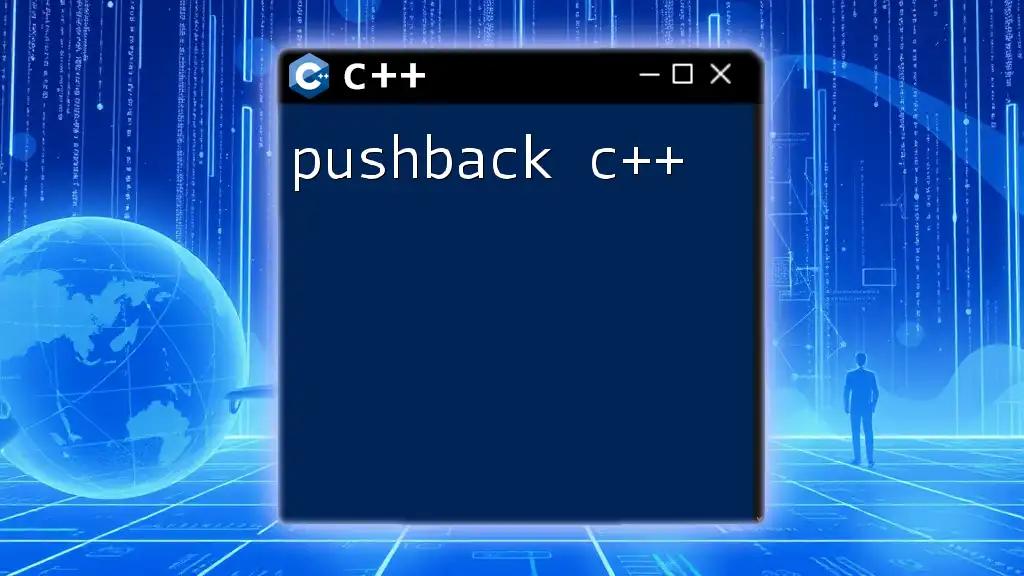
Using `push_back` in Vectors
What is a Vector in C++?
A vector is a sequence container representing an array that can change in size. Compared to static arrays, vectors can adjust their sizes dynamically, allowing for efficient data management. This characteristic makes vectors a preferred choice when the exact size of the data is not known upfront.
Syntax of `push_back`
The syntax for using the `push_back` method is straightforward:
vector<type> vec;
vec.push_back(value);
With this syntax, you instantiate a vector of a particular type and then use `push_back` to add a value to it.
Adding Elements to a Vector
Adding single elements to a vector with `push_back` is simple. Here’s a basic example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(10);
numbers.push_back(20);
std::cout << "Vector elements: ";
for (int n : numbers) {
std::cout << n << " "; // Output: 10 20
}
return 0;
}
In this example, we create an empty vector named `numbers` and then append the values 10 and 20 to it using `push_back`. The output illustrates that both elements were successfully added.
Inserting Multiple Elements Using `push_back`
You can also use a loop to insert multiple values into a vector. For instance:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
for (int i = 0; i < 5; ++i) {
numbers.push_back(i * 10); // Adds 0, 10, 20, 30, 40
}
std::cout << "Vector elements: ";
for (int n : numbers) {
std::cout << n << " "; // Output: 0 10 20 30 40
}
return 0;
}
This example demonstrates how to dynamically build a vector of integers using a loop to generate values. Each iteration calls `push_back` to add a new element to the vector.

Key Characteristics of `push_back`
Memory Management
One important aspect of `push_back` is memory management. When you add an element using `push_back`, the vector may need to allocate more memory if its current storage is full. This resizing process can lead to performance overhead, especially if you are adding a large number of elements.
Automatic Resizing of Vectors
Vectors automatically resize themselves to accommodate new elements. Initially, a vector allocates a certain amount of memory. If you exceed this limit, the vector will allocate more memory—typically, it doubles the previous size. This feature simplifies the programmer's job by handling memory allocation in the background.

Benefits of Using `push_back`
Ease of Use
The `push_back` method provides a simple and intuitive way to append data to a container. It abstracts the complexities of memory management, allowing developers to focus on their application logic rather than worrying about underlying storage details.
Dynamic Data Management
Using `push_back`, you can easily manage dynamic collections of data. Whether you are processing user input, managing a list of items, or handling variable-length datasets, `push_back` gives you the flexibility to adapt your container to changing requirements.

Common Use Cases for `push_back`
Building Dynamic Lists
In real-world applications, dynamic lists of elements are frequently necessary. For example, when collecting user inputs during a program's execution—such as survey responses or menu selections—using `push_back` allows you to gather and store these inputs efficiently.
Practical Examples of Using `push_back`
You might find `push_back` useful in various scenarios, such as:
- Collecting data for a dynamic playlist in a music application.
- Storing computed values during iterative algorithms.
- Aggregating responses from a set of queries in database applications.
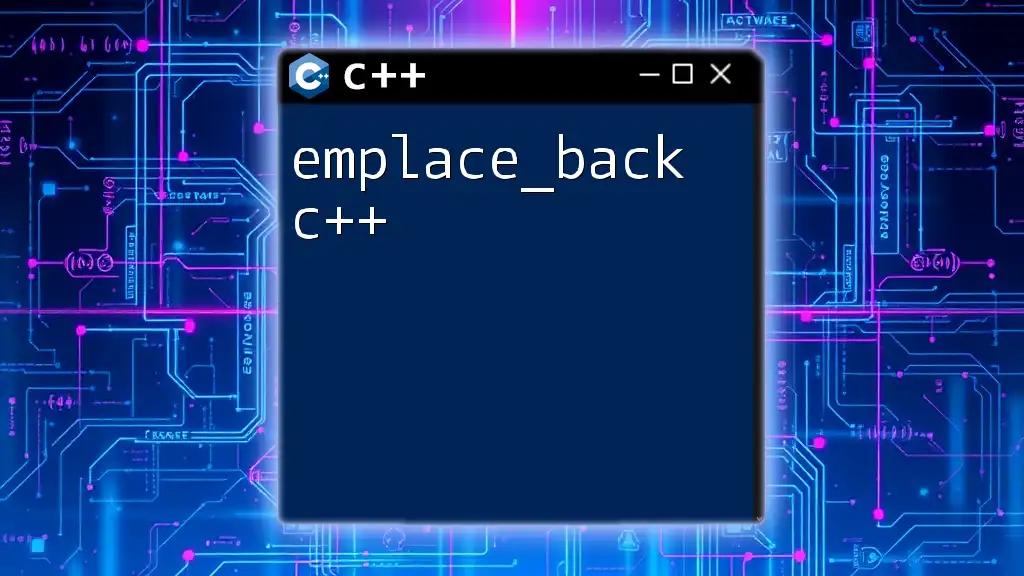
Limitations and Considerations
Performance Considerations
While `push_back` is convenient, using it excessively can lead to performance degradation due to frequent memory reallocations. Each time a vector resizes, it may involve copying existing elements to the new memory location.
Alternatives to `push_back`
In instances where performance is critical, consider using `reserve` to pre-allocate memory before repeatedly using `push_back`:
std::vector<int> numbers;
numbers.reserve(100); // Pre-allocate space for 100 elements
for (int i = 0; i < 100; ++i) {
numbers.push_back(i);
}
By using `reserve`, you minimize the number of memory reallocations, which enhances performance for large insertions.
When Not to Use `push_back`
In cases where the number of elements is known beforehand, it may be more efficient to use a fixed-size array or a pre-allocated vector rather than relying on `push_back` for incremental additions.

Conclusion
The `push_back` function is an essential tool in C++ for managing dynamic collections of data through vectors. By understanding its functionality and implications, you can leverage `push_back` effectively to streamline your programming tasks in C++. The ease of use, combined with the flexible management of dynamic data, makes `push_back` a fundamental component of C++ programming. As you progress in your C++ journey, practice using `push_back` to gain a deeper understanding of how it can enhance your code.

References and Further Reading
For more in-depth knowledge about vectors and the `push_back` function, consider consulting the official C++ documentation. Additionally, seek out books and online resources that delve into advanced C++ concepts, particularly those focused on the Standard Template Library and data structure management.