In C++, `cout` is used to output data to the standard output stream, typically the console, with an example syntax shown below:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is `cout`?
`cout`, short for character output stream, is an essential part of the C++ Standard Library utilized for outputting data to the console. It plays a key role in interacting with users by displaying text, numbers, and other information as part of the program's functionality.
The Role of `std::cout`
The `cout` object resides within the `std` namespace. The significance of using `std::cout` is to prevent naming conflicts in larger programs and to clarify that `cout` is part of the C++ standard library. In order to utilize this feature, you must include the `<iostream>` header file in your program.
Example:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to C++ programming!" << endl;
return 0;
}
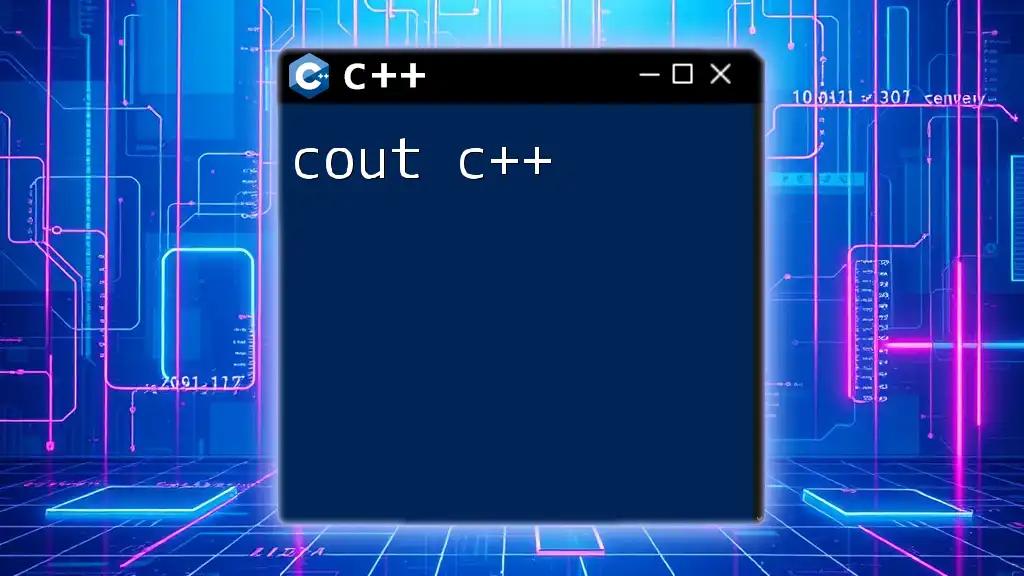
Getting Started with `cout`
Setup Your Environment
Before diving into coding, ensure that you have a proper C++ development environment set up on your computer. Popular options include IDEs such as Code::Blocks, Visual Studio, and Eclipse, or using text editors with command-line tools like g++.
Basic Syntax of `cout`
The syntax for using `cout` is straightforward. To print output to the console, you use the stream insertion operator `<<`. Here's a simple illustration:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Here, `Hello, World!` is sent to the console, and `endl` is used to insert a line break, flushing the output buffer.
Multiple Outputs
One of the strengths of `cout` is the ability to concatenate multiple values in a single output line. You can seamlessly mix strings and variables as demonstrated below:
int age = 25;
cout << "I am " << age << " years old." << endl;
This statement will print `I am 25 years old.` to the console, showcasing how `cout` can handle both string literals and variables.

Formatting Output
Basic Formatting Techniques
Formatting allows for improved readability of console output. Using `endl` is common for line breaks; however, you can also use the `'\n'` character for new lines, which is slightly more efficient:
cout << "First Line\nSecond Line" << endl;
Manipulators
C++ provides several manipulators that enhance the formatting of output values. Two commonly used manipulators are `std::fixed` and `std::setprecision`.
Example of `std::setprecision`
Here's how you can control the precision of floating-point numbers displayed:
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
double pi = 3.14159;
cout << fixed << setprecision(2) << pi << endl; // Outputs: 3.14
return 0;
}
The output of this code snippet limits the decimal display of `pi` to two places, showing how `cout` can produce professional-looking numerical outputs.
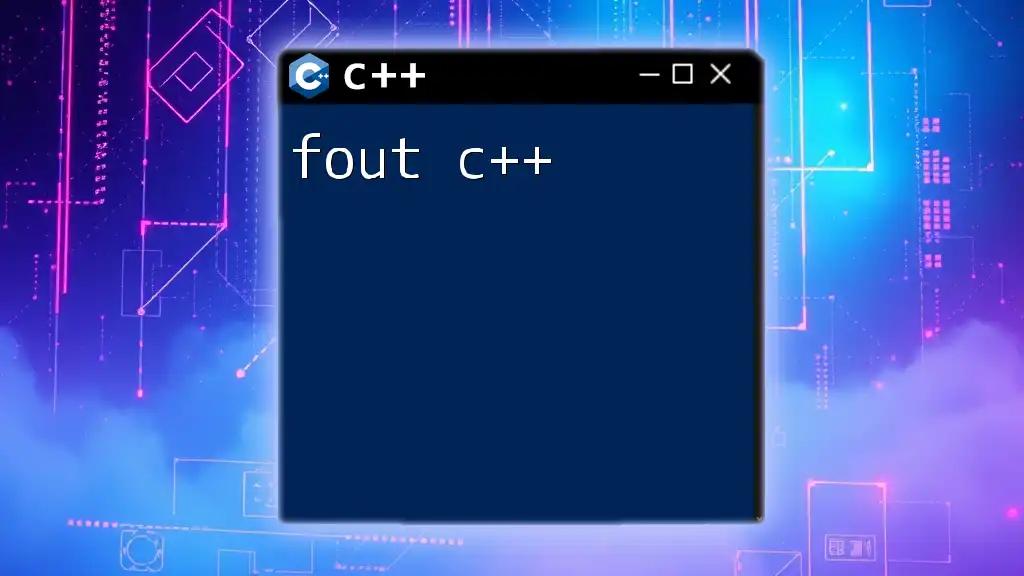
Advanced Output Techniques
Outputting Different Data Types
`cout` is versatile in that it can handle a variety of data types seamlessly—integers, floating-point numbers, characters, and strings can all be displayed:
int x = 10;
float y = 5.5;
char z = 'A';
string name = "Alice";
cout << "Integer: " << x << ", Float: " << y << ", Character: " << z << ", String: " << name << endl;
In this code, each variable's type is demonstrated, showcasing the flexibility of `cout` in handling mixed data types in a clean and readable format.
Custom Output with `cout`
Creating custom output functions allows for more complex formatting or repetitive tasks. For instance, consider the following function that formats user information:
void displayInfo(string name, int age) {
cout << "Name: " << name << ", Age: " << age << endl;
}
You can call this function from `main` to display information dynamically, reinforcing how `cout` can enhance custom implementations.
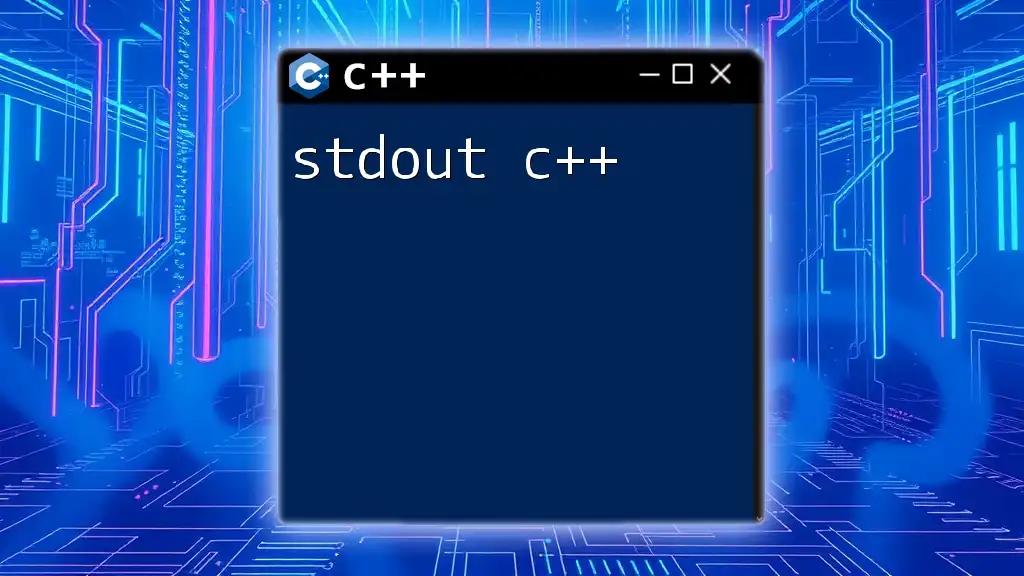
Common Errors with `cout`
Compile-time Errors
A common point of error occurs when omitting the necessary header file `#include <iostream>`. Without this, your program will fail to compile, producing error messages indicating an unrecognized identifier for `cout`.
Run-time Errors
Run-time errors can arise unexpectedly, often arising from incorrect variable types during output or trying to manipulate uninitialized variables. Ensuring that the variables you are attempting to output are correctly initialized is crucial for preventing these errors.
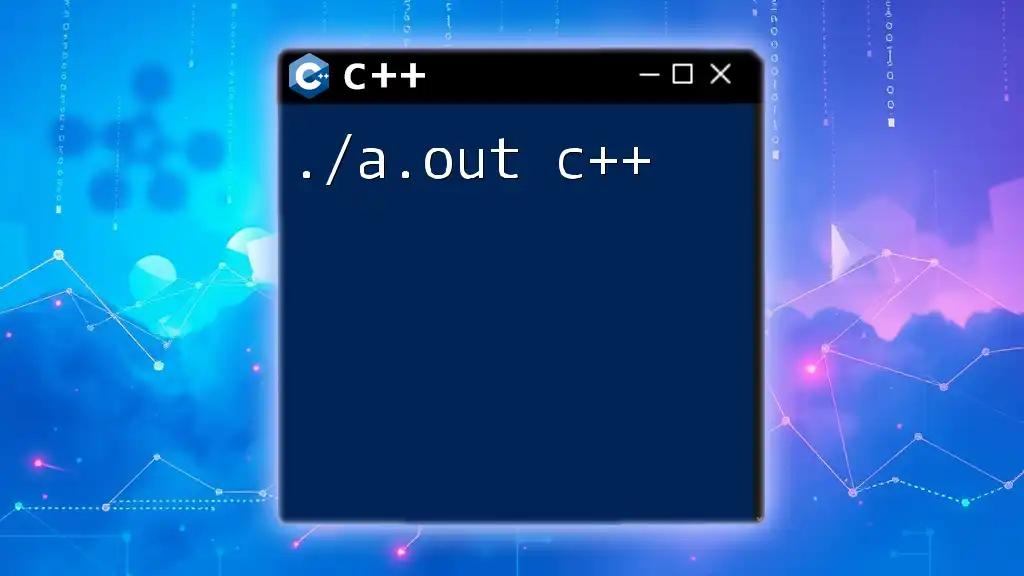
Conclusion
In summary, understanding `cout` is fundamental to mastering C++ programming. It facilitates user interaction through console output, allowing the display of strings, numbers, and more, while offering formatting control for better presentation.
Practicing various output functionalities with exercises will enhance your skills, helping you become a more proficient C++ programmer.

Additional Resources
For further learning, consider exploring online resources, textbooks dedicated to C++, or engaging in community forums where you can share experiences and gain insights from others.
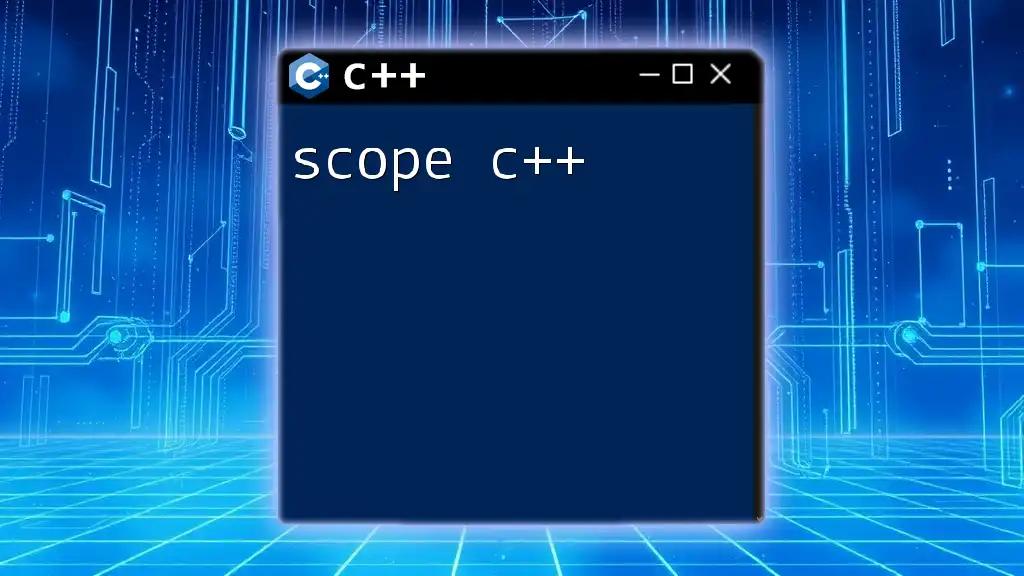
Call to Action
Stay connected for more tips and tricks on C++. Subscribe to our newsletter to keep up with upcoming posts, and share your experiences using `cout` with the community!