In C++, the maximum value for a `long long` data type can be obtained using the constant `LLONG_MAX` defined in the `<climits>` header.
Here’s a code snippet demonstrating how to use it:
#include <iostream>
#include <climits>
int main() {
std::cout << "The maximum value of long long is: " << LLONG_MAX << std::endl;
return 0;
}
Understanding Data Types in C++
What is a Data Type?
A data type is a classification that specifies which type of value a variable can hold. Understanding data types is crucial in programming because it determines the types of operations you can perform on that data and the amount of memory that data occupies.
Overview of Integer Data Types
In C++, integers can be represented using various data types, including `int`, `long`, and `long long`. Each type has its own range, which is primarily determined by the number of bits allocated to store values. Here's a quick comparison:
- `int`: Typically 32 bits, ranging from -2,147,483,648 to 2,147,483,647.
- `long`: Depends on the architecture (32-bit or 64-bit), but usually at least 32 bits.
- `long long`: A standardized type that is guaranteed to be at least 64 bits, offering a much larger range for integer values.
Size and Range of C++ Data Types
Understanding the size and range of data types is essential when designing applications that handle large numerical computations. Here is a reference table for standard C++ integer types and their ranges:
- int: -2,147,483,648 to 2,147,483,647
- long: -2,147,483,648 to 2,147,483,647 (on most systems)
- long long: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
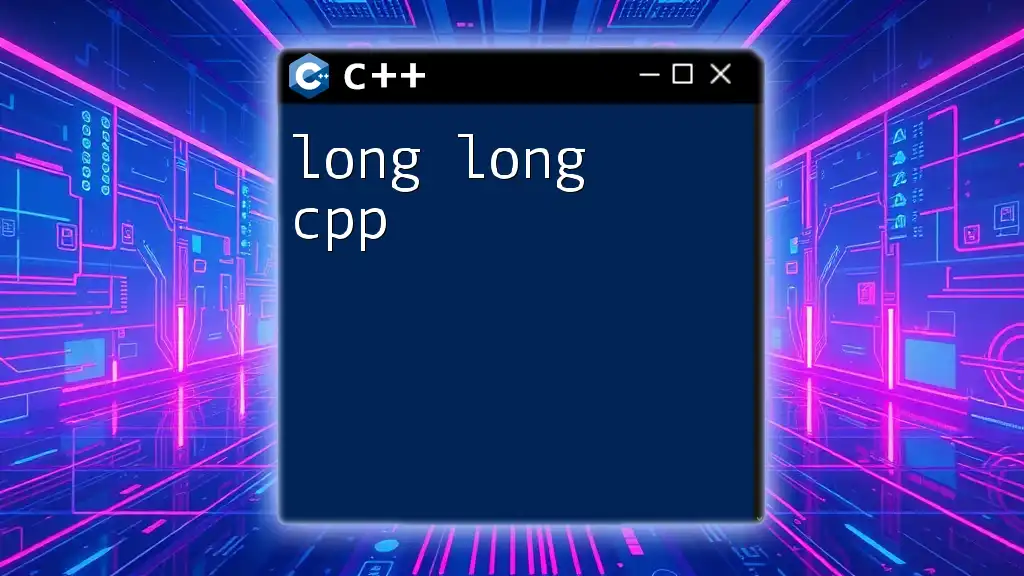
Long Long Data Type
Definition and Use of Long Long
In C++, the `long long` data type is designed for scenarios where an integer exceeds the range of standard data types. It is particularly useful in applications such as financial modeling, scientific computing, and any situation where large integer computations are required.
Maximum Value of Long Long
The maximum value that a `long long` variable can hold is defined by the constant `LLONG_MAX`, which is included in the `<climits>` header. When working with `long long`, it is essential to know this limit to avoid potential overflow errors in your applications.
Example
Here's a simple example demonstrating how to access the maximum value of the `long long` type:
#include <iostream>
#include <climits> // for LLONG_MAX
int main() {
std::cout << "The maximum value of long long is: " << LLONG_MAX << std::endl;
return 0;
}
Binary Representation
The binary representation of `long long` values consists of 64 bits, allowing for a wide range of positive and negative integers. The most significant bit is used to indicate the sign (0 for positive, 1 for negative). Understanding this representation helps programmers predict the outcomes of mathematical operations accurately.
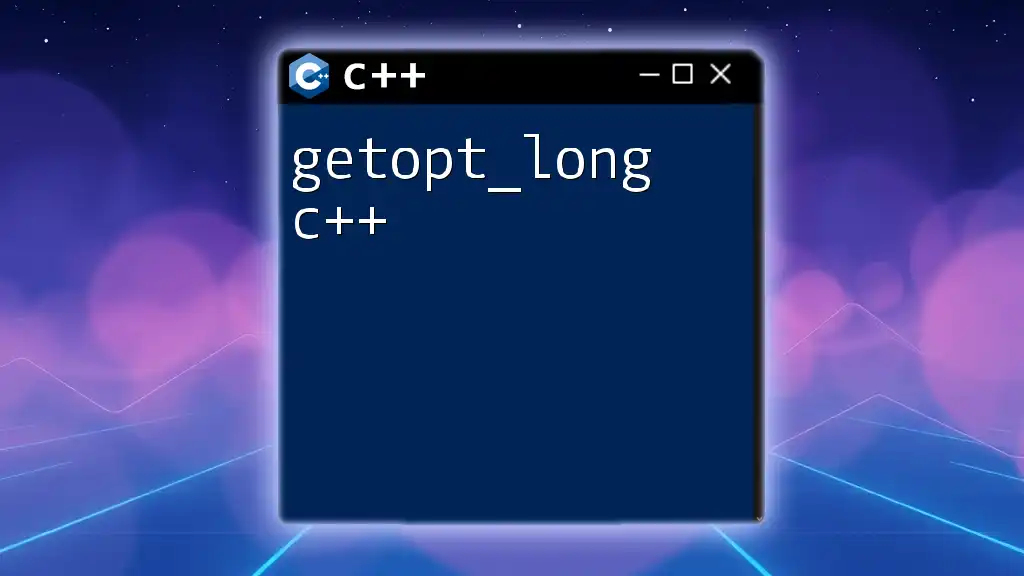
Working with Long Long in C++
Declaring Long Long Variables
Declaring variables of type `long long` is straightforward. You can define it similarly to other types in C++. For instance:
long long a; // simple declaration
long long b = 123456789012345; // initialized declaration
Practical Uses of Long Long
Long long is an invaluable tool when dealing with large datasets or performing computations that might exceed the limits of regular integer types. This includes:
- Financial applications where high precision is required.
- Algorithms that involve large combinatorial calculations.
- Numerical simulations that generate large datasets.
Example Use Case
Here's an example to illustrate a situation where `long long` is necessary:
#include <iostream>
int main() {
long long large_number1 = 9223372036854775807; // LLONG_MAX
long long large_number2 = 1;
long long result = large_number1 + large_number2; // Overflow case
std::cout << "Result of addition (may overflow): " << result << std::endl;
return 0;
}
In this case, adding `1` to `LLONG_MAX` will lead to an overflow. This highlights the importance of managing data types properly in C++.
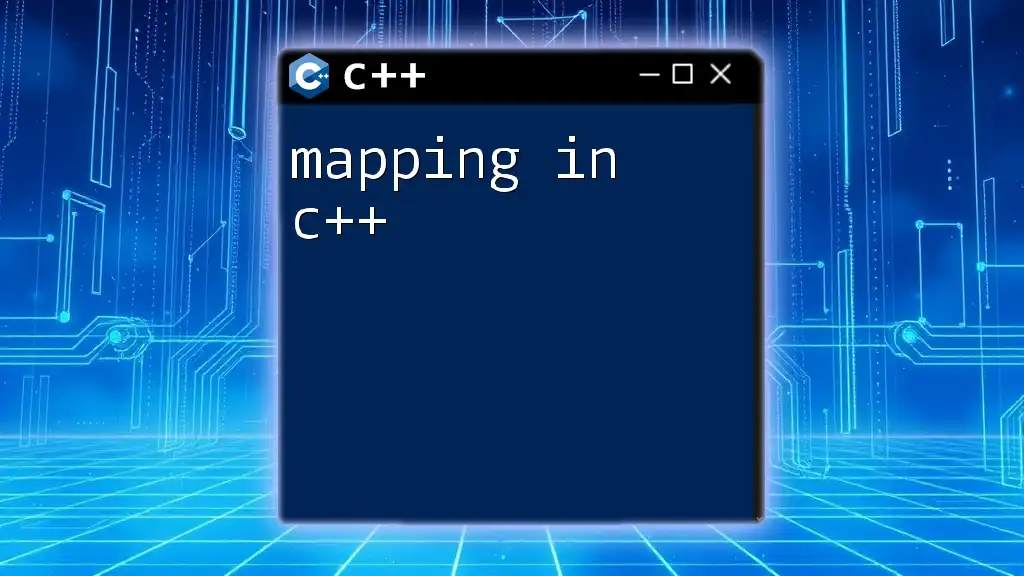
Handling Maximum Values and Overflow
Understanding Integer Overflow
Integer overflow occurs when an arithmetic operation produces a value outside the range that can be represented with the allocated bits. For `long long`, this can result in wrapping around to the negative or truncating the number, leading to severe bugs in applications.
Preventing Overflow in C++
To prevent overflow, developers can incorporate checks before performing calculations. For example, before performing addition, always check if the result would exceed `LLONG_MAX`.
Example of Overflow Prevention
Here's a code snippet to illustrate how you can prevent overflow in your calculations:
#include <iostream>
#include <climits>
int main() {
long long number1 = LLONG_MAX;
long long number2 = 1;
if (number1 > LLONG_MAX - number2) {
std::cout << "Addition will overflow!" << std::endl;
} else {
long long result = number1 + number2;
std::cout << "Result: " << result << std::endl;
}
return 0;
}
In this example, the program checks if adding `number2` to `number1` would result in overflow before proceeding with the addition. This simple check can save you from critical bugs in larger applications.
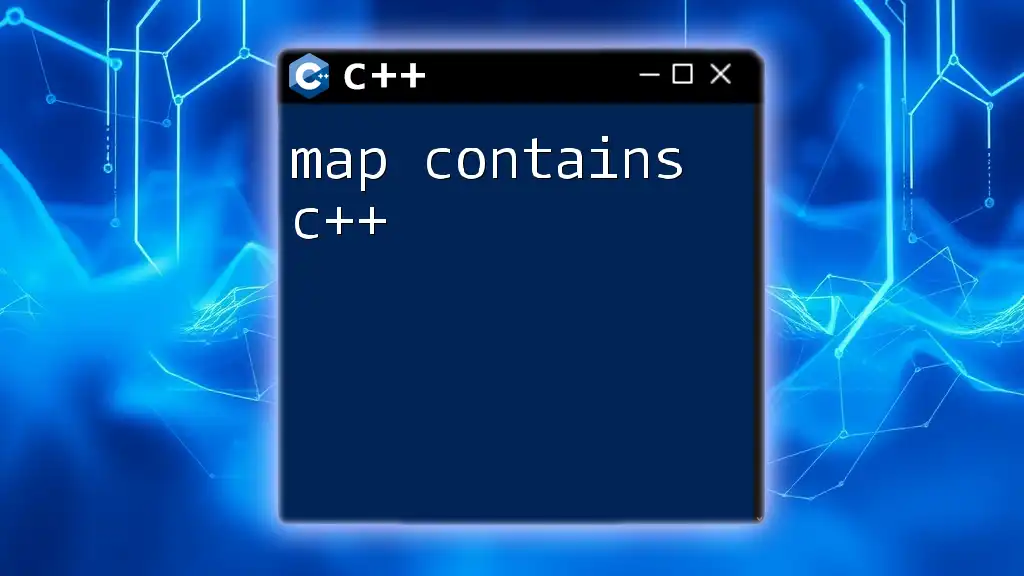
Conclusion
Understanding the concept of max long long c++ is crucial for any programmer dealing with large integer values. Recognizing the maximum value of `long long`, knowing how to declare and use this data type effectively, and implementing methods to avoid overflow will contribute significantly to the reliability and efficiency of your C++ applications. Adopting best practices and becoming familiar with the implications of using `long long` will enhance your skill set as a C++ developer.