In C++, an array is a collection of elements of the same type stored in contiguous memory locations, allowing for efficient data manipulation and access.
#include <iostream>
int main() {
int numbers[5] = {1, 2, 3, 4, 5}; // Declaring and initializing an array of integers
for(int i = 0; i < 5; i++) {
std::cout << numbers[i] << " "; // Accessing and printing each element
}
return 0;
}
Understanding C++ Array Types
What is an Array in C++?
At its core, an array in C++ is a collection of variables that are accessed with a common name. Each element in an array is stored in contiguous memory locations. This structure allows for efficient data management and processing. With arrays, you can handle a collection of data points as a single entity rather than as separate variables.
Types of Arrays in C++
Single-Dimensional Arrays
Single-dimensional arrays are the simplest form of arrays. They can be visualized as a list of items stored in a single row.
To declare a single-dimensional array, you can utilize the following syntax:
int myArray[5];
This declaration creates an array of 5 integers. The elements in the array can be accessed by their indices, starting from 0. For example, to modify an element in an array:
myArray[0] = 10;
std::cout << myArray[0]; // Output: 10
Here, the value 10 is assigned to the first element of `myArray`, which is then printed to the console.
Multi-Dimensional Arrays
In contrast to single-dimensional arrays, multi-dimensional arrays allow for the storage of data in a grid-like structure, containing rows and columns. Typically, a two-dimensional array is used for this purpose.
A two-dimensional array can be declared like this:
int matrix[3][3];
The above code defines a 3x3 matrix of integers. Accessing elements in this structure is similar to single-dimensional arrays but requires two indices. For example:
matrix[0][1] = 5;
std::cout << matrix[0][1]; // Output: 5
This code snippet assigns the value 5 to the element located at the first row and second column of the matrix.
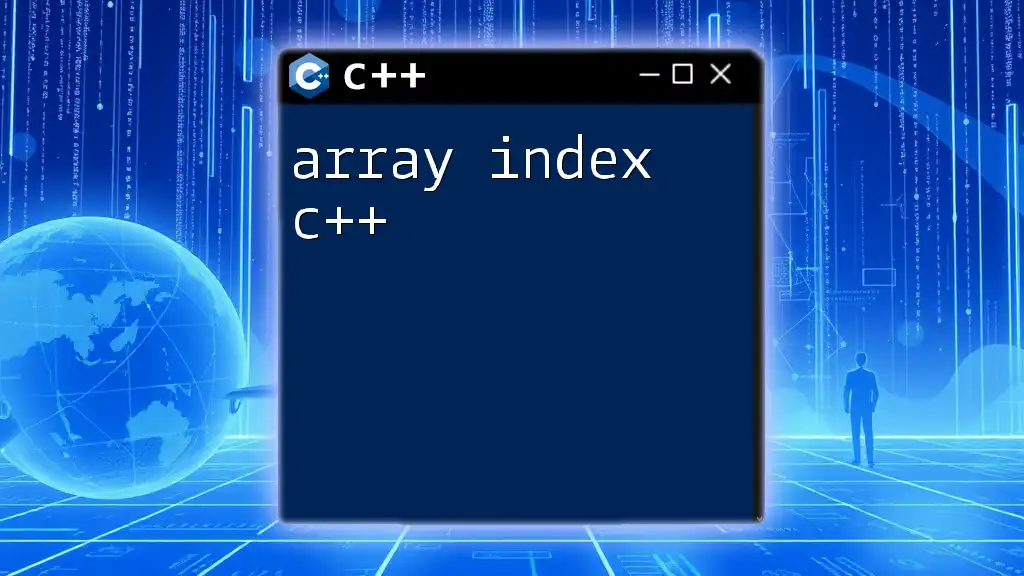
Working with Arrays in C++
Initializing Arrays
Arrays can be initialized in different ways. The method of initialization can affect both the size of the array and whether the values are assigned at the time of declaration.
Static Initialization
Static initialization occurs at the time of declaration. To create and initialize an array with specific values, you can use:
int arr[5] = {1, 2, 3, 4, 5};
This creates an array of 5 integers with values 1 through 5.
Dynamic Initialization
Dynamic initialization allows for flexibility in memory usage. An array can be dynamically allocated using pointers:
int* dynamicArr = new int[5]{1, 2, 3, 4, 5};
Here, `dynamicArr` points to an array of integers allocated on the heap. Remember to release this memory later using `delete[]` to avoid memory leaks.
Common Array Operations
Iterating Through an Array
Accessing each element in an array can be performed using loops. Below is an example of how to traverse a single-dimensional array:
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
This code snippet prints all elements of `arr`, demonstrating how to loop through an array effectively.
Searching Elements in an Array
Searching for a specific element within an array can be handled with algorithms like linear search. Here’s a function that demonstrates this approach:
bool linearSearch(int arr[], int size, int key) {
for (int i = 0; i < size; i++) {
if (arr[i] == key) return true;
}
return false;
}
This function iterates through `arr`, whether the element specified by `key` exists, returning `true` or `false` accordingly.
Sorting an Array
Sorting is another common operation. The bubble sort algorithm is a straightforward method to sort an array. Here’s how it works:
void bubbleSort(int arr[], int size) {
for (int i = 0; i < size-1; i++) {
for (int j = 0; j < size-i-1; j++) {
if (arr[j] > arr[j+1]) {
std::swap(arr[j], arr[j+1]);
}
}
}
}
In this code, adjacent elements are compared and swapped if they are in the wrong order, ensuring that the largest element "bubbles" to the end with each iteration.
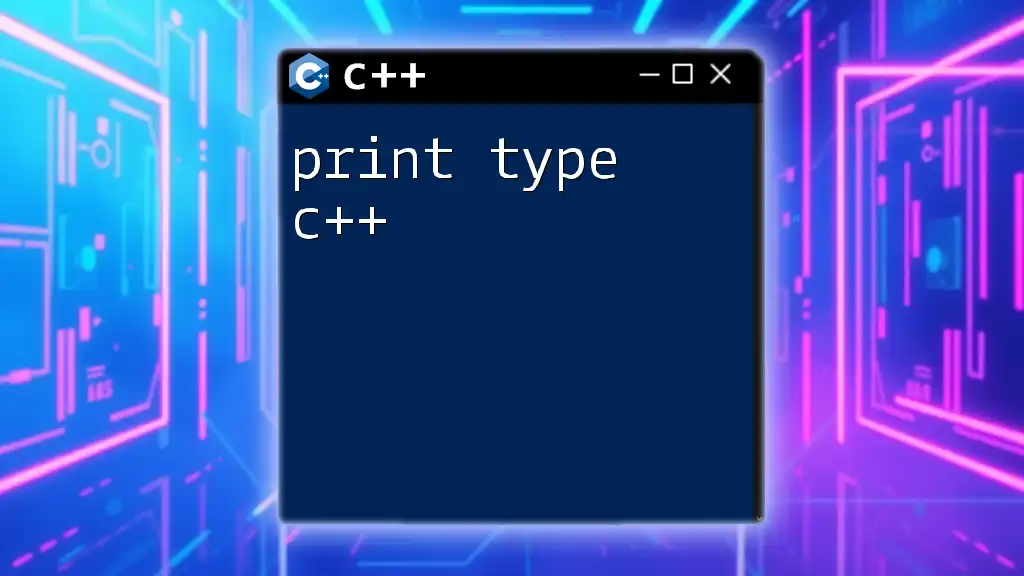
Best Practices for Using Arrays in C++
Avoiding Common Pitfalls
One of the most significant challenges when working with arrays is out-of-bounds access. Accessing an index larger than the array size can cause undefined behavior or memory corruption. Always ensure that indices stay within the bounds of the declared size.
When using dynamic arrays, memory management is critical. Always free memory allocated with `new` using `delete[]`:
delete[] dynamicArr;
This step is crucial to prevent memory leaks, where memory is allocated but never freed, ultimately reducing the efficiency of your application.
When to Use Arrays vs. Other Data Structures
While arrays are powerful, they may not always be the best choice for all scenarios. In contexts where flexibility is required, such as needing to change the size of the structure dynamically, consider alternative data structures like vectors or lists.
Arrays offer fast access and require less overhead when the size is known and fixed, making them suitable for performance-critical applications. On the other hand, STL containers like `std::vector` are better suited for scenarios requiring frequent resizing or insertion and deletion of elements.
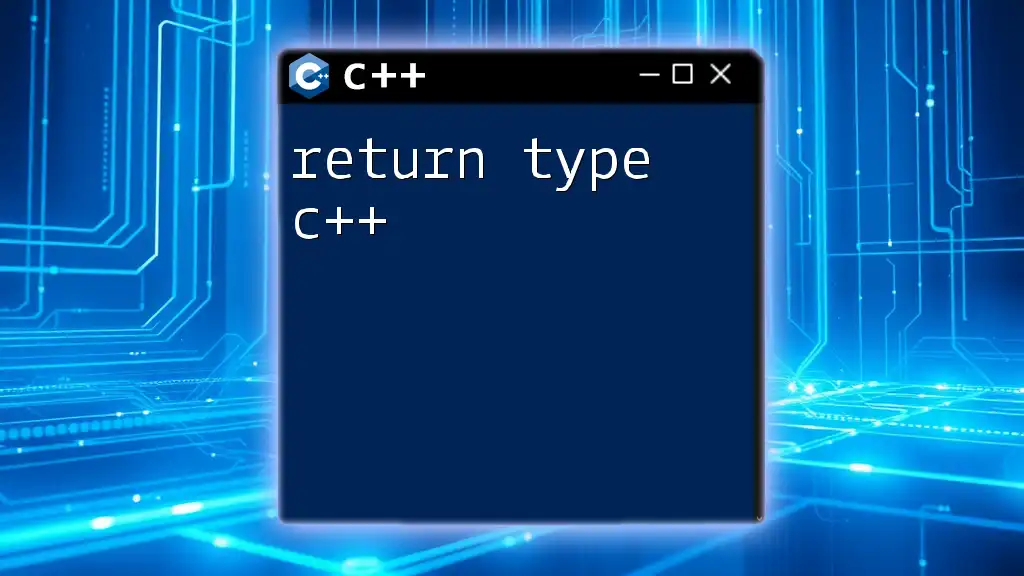
Conclusion
Mastering the array type in C++ is crucial for efficient programming. With insights into single-dimensional and multi-dimensional arrays, initialization methods, and various operations, you are now equipped to leverage arrays effectively.
Practice is essential—experiment with different array types and functions to strengthen your understanding of their potential. For further exploration, seek resources that challenge your skills, and consider engaging with programming communities to share tips and insights on C++ programming!