In C++, arrays are collections of elements of the same type that are stored in contiguous memory locations, enabling efficient access and manipulation of multiple data items through a single identifier.
#include <iostream>
int main() {
int numbers[5] = {1, 2, 3, 4, 5}; // Example of an integer array
for(int i = 0; i < 5; i++) {
std::cout << numbers[i] << " "; // Output: 1 2 3 4 5
}
return 0;
}
What is an Array?
An array in C++ is a collection of elements, all of the same type, stored in contiguous memory locations. This allows you to manage a series of data items under a single name, making it easier to manipulate them. Arrays are essential for performing operations that require grouping multiple values of the same type together.
The basic syntax for declaring an array is as follows:
data_type array_name[array_size];
For example, if you want to declare an array of integers with five elements, you would write:
int arr[5];
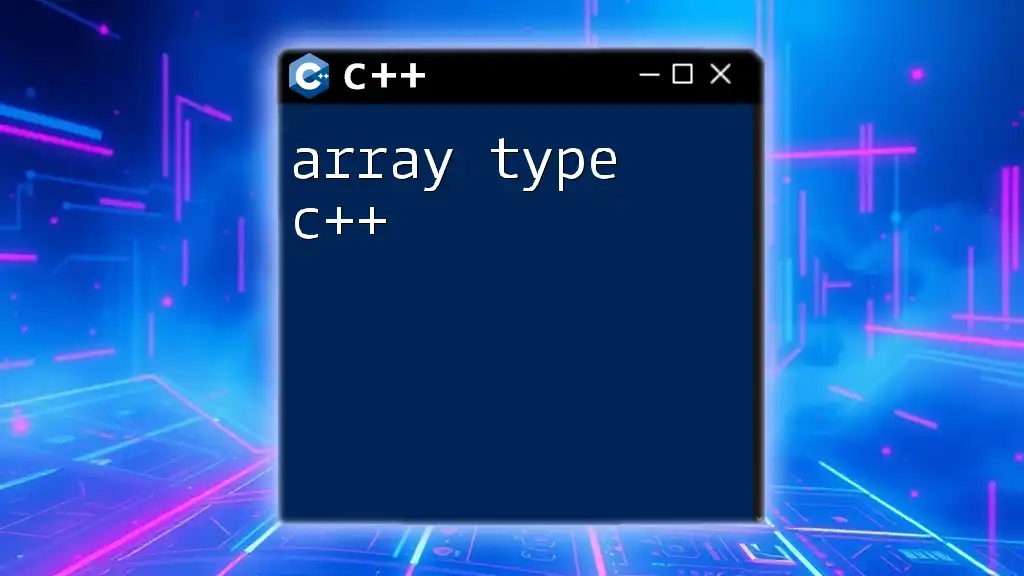
Why Use Arrays?
Using arrays offers several advantages in programming:
- Efficiency: Arrays allow easy access and modification of data using indices.
- Simplified Management: They provide a way of organizing multiple variables under a single identifier.
- Increased Performance: Operations involving a series of data can be handled more efficiently in arrays, especially when using loops.
However, arrays do have limitations. The size is fixed once allocated, and inserting or deleting elements requires shifting, which can add overhead.
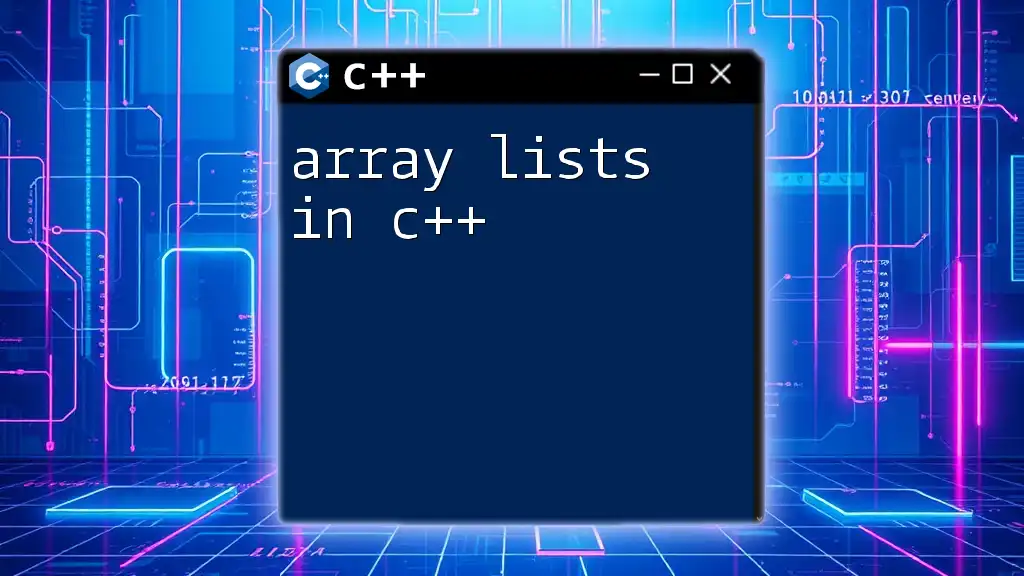
Types of Arrays in C++
One-Dimensional Arrays
Definition and Syntax
A one-dimensional array is the simplest form of an array, consisting of a series of elements aligned in a single line. This type of array is suitable for storing lists of items such as integers, characters, or floats.
To declare and initialize a one-dimensional array, you write:
int arr[5] = {1, 2, 3, 4, 5};
Accessing and Modifying Elements
Accessing elements in a one-dimensional array uses a zero-based index. For example, `arr[0]` represents the first element.
You can also modify elements easily. Consider the following code snippet that changes the third element of the array:
arr[2] = 10; // Changing the third element to 10
Two-Dimensional Arrays
Definition and Syntax
A two-dimensional array can be visualized as a grid or a table, where data is organized in rows and columns. It is useful for managing datasets that require two indices to access their values.
The syntax for declaring a two-dimensional array is:
int matrix[3][3];
To initialize it with values, you can write:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Accessing and Modifying Elements
To access an element in a two-dimensional array, you use two indices—one for the row and one for the column. For instance:
int value = matrix[1][2]; // Accessing element at row 1, column 2
You can modify an element in the same way:
matrix[1][2] = 10; // Changing the element at row 1, column 2
Multi-Dimensional Arrays
Definition and Syntax
Multi-dimensional arrays extend this concept to more than two dimensions, where data can be organized in a cube or even higher dimensions. They are helpful in cases such as 3D graphics or complex simulations.
The syntax for declaring a three-dimensional array is:
int cube[4][3][2]; // Declaring a 3D array (cube)
You can initialize a three-dimensional array using similar syntax, similar to what you would do with two-dimensional arrays, but extending the initialization pattern.
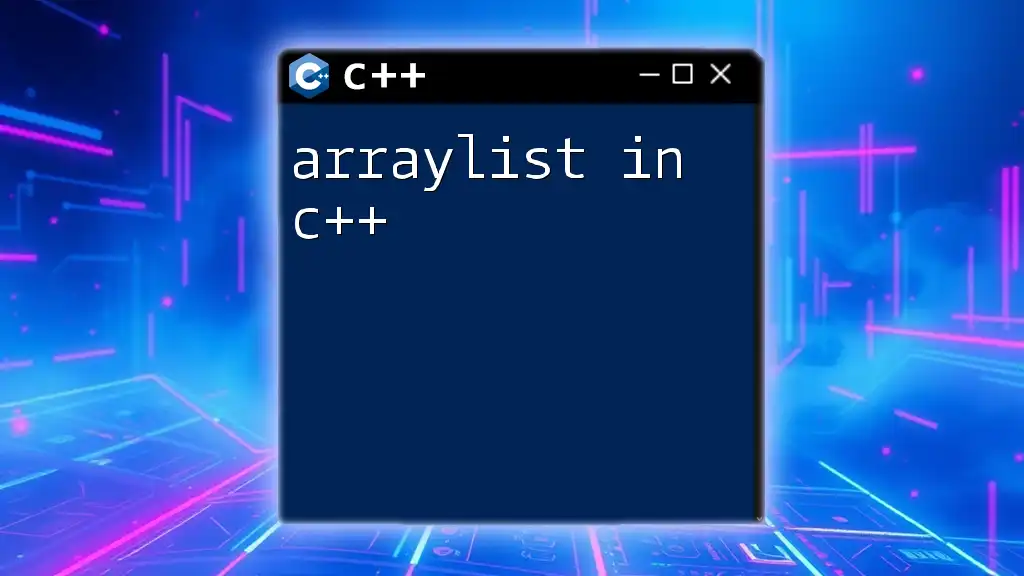
Special Types of Arrays in C++
Dynamic Arrays
What are Dynamic Arrays?
Dynamic arrays allow you to allocate memory for an array at runtime, making the array size flexible. This adaptability is beneficial when the required size of the array is not known in advance.
Implementing Dynamic Arrays
To declare a dynamic array in C++, you can use the `new` keyword:
int* dynArr = new int[5]; // Creating a dynamic array of size 5
To free the memory allocated for a dynamic array, use the `delete` keyword:
delete[] dynArr; // Deleting dynamic array
This is crucial for preventing memory leaks in applications.
Array of Structures
Explanation of Arrays of Structures
An array of structures combines the advantages of arrays and structures. You can define a structure to hold various attributes and then create an array to hold multiple records of that structure type.
For example, you can define a `Student` structure as follows:
struct Student {
int id;
char name[50];
};
You can then declare an array of `Student`:
Student students[100]; // Array of 100 students
This allows you to efficiently manage multiple `Student` records.
Pointers and Arrays
Relationship Between Pointers and Arrays
In C++, pointers and arrays are closely related. An array name acts as a pointer to its first element. This property allows for effective memory access and manipulation.
You can demonstrate this with the following code snippet:
int* ptr = arr; // Pointer to the first element of arr
cout << *(ptr + 1); // Accessing the second element using pointer arithmetic
This illustrates how pointers can provide an alternative approach for accessing array elements.
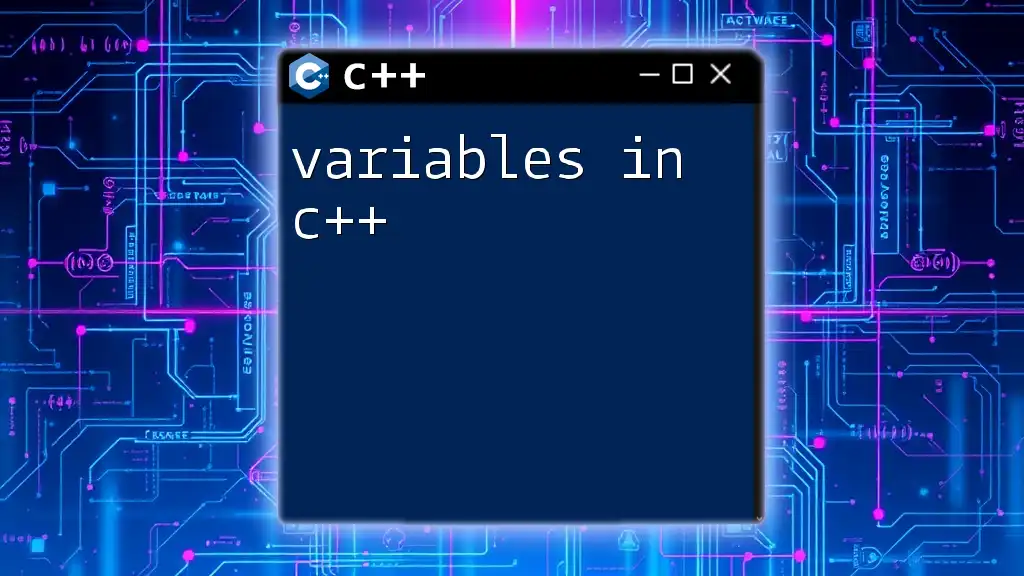
Conclusion
Recap of Array Types in C++
In summary, understanding array types in C++ is essential for effective programming. We covered one-dimensional, two-dimensional, and multi-dimensional arrays, as well as dynamic arrays and arrays of structures. Each type has its own unique use cases and benefits, making it crucial to select the right type based on your program's requirements.
Further Learning
To deepen your knowledge, consider exploring resources like official C++ documentation, online coding platforms, and community forums. Practice implementing different array types to solidify your understanding and improve your skills in C++ programming.