Folly is a library developed by Facebook that provides a variety of reusable C++ components and utilities designed to enhance performance and development efficiency in C++ applications.
#include <folly/Range.h>
#include <iostream>
int main() {
folly::StringPiece str = "Hello, Folly!";
std::cout << str << std::endl;
return 0;
}
What is Folly?
Folly is a powerful and versatile C++ library developed by Facebook, designed to complement and extend the standard C++ library. It encompasses a wide array of components and features that facilitate efficient programming practices, offering optimizations that are crucial for performance-intensive applications. Folly leverages modern C++ capabilities, making it particularly attractive for developers working in high-performance environments.
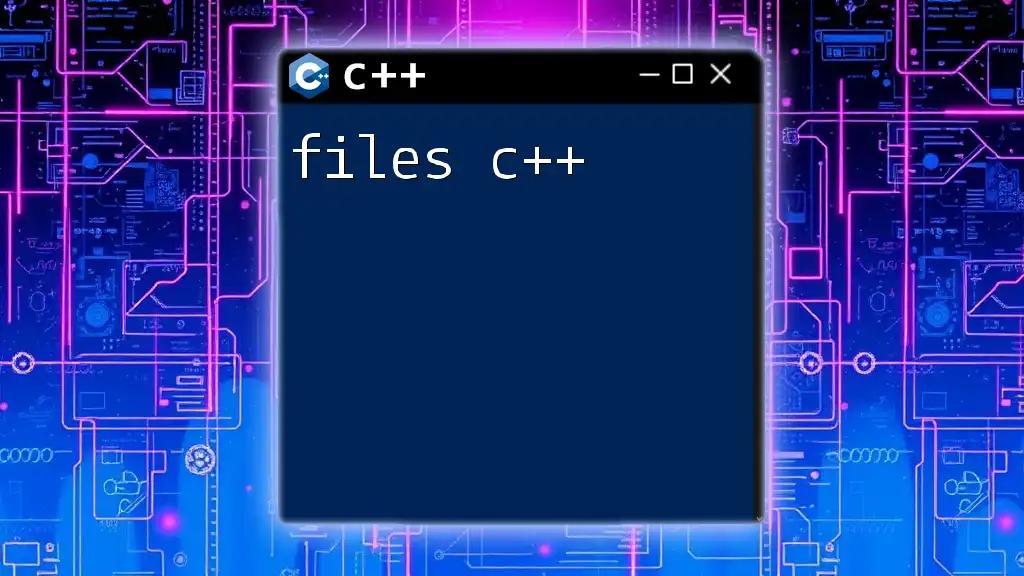
Why Use Folly?
There are several compelling reasons to incorporate Folly C++ into your projects:
- Optimized Performance: Folly is tailored for speed and efficiency, implementing data structures and algorithms with performance in mind.
- Advanced Features: The library provides a plethora of abstractions that encourage the use of advanced programming techniques, such as asynchronous programming and complex data structures.
- Community Support: As an open-source project driven by Facebook, Folly benefits from a vibrant community that continuously contributes to its improvement.

Getting Started with Folly
Prerequisites
Before diving into Folly, ensure you have a solid grasp of modern C++ (C++11 and above), as well as a compatible build system like CMake. Folly supports various platforms, but it's crucial to check the specific version requirements.
Installation Guide
Folly can be installed using various methods, depending on your platform and environment preferences. Below are two common approaches:
Using CMake
To install Folly via CMake, follow these commands:
git clone https://github.com/facebook/folly.git
cd folly
mkdir _build
cd _build
cmake ..
make
sudo make install
This installs Folly C++ to your local environment, and the library will be ready for inclusion in your projects.
Using Package Managers
For those who prefer package managers, Folly can often be found in repositories associated with systems like Homebrew or APT:
- For Homebrew on macOS:
brew install folly
- For APT on Ubuntu:
sudo apt install folly

Core Features of Folly
Data Structures
Folly's Immutable Data Structures
Folly's support for immutable data structures offers significant benefits in programming, most notably data integrity and easier reasoning about code. Immutability ensures that once an object is created, it cannot be altered, leading to fewer bugs and improved concurrency handling.
Example: Using `folly::ImmutableVector`
#include <folly/ImmutableVector.h>
folly::ImmutableVector<int> vec = folly::ImmutableVector<int>::withItems({1, 2, 3, 4});
auto newVec = vec.push_back(5);
In this example, `vec` remains unchanged while `newVec` contains the modified data, illustrating the immutable nature of these data structures.
Concurrent Data Structures
Folly provides a rich set of concurrent data structures designed for performance in multi-threaded environments, such as `folly::ConcurrentHashMap`.
Example: `folly::ConcurrentHashMap`
#include <folly/ConcurrentHashMap.h>
folly::ConcurrentHashMap<int, std::string> map;
map.insert(1, "One");
map.insert(2, "Two");
std::string value = map[1]; // value == "One"
This code snippet demonstrates how to easily create and access concurrent data without worrying about thread contention.
Concurrency and Threading
Thread Pool Implementation
Folly offers an efficient thread pool implementation, allowing developers to manage and run tasks concurrently.
Example: Creating and using a Thread Pool
#include <folly/ThreadPoolExecutor.h>
folly::ThreadPoolExecutor executor(4);
executor.add([&] {
std::cout << "Hello from thread pool!" << std::endl;
});
executor.join();
In this example, a thread pool with four threads is created, and a task is added for execution. The `join` method ensures all tasks complete before the program exits.
Promises and Futures
Asynchronous programming is made simpler with promises and futures provided by Folly. These abstractions help in managing asynchronous computations more effectively.
Code Example: Creating and handling promises
#include <folly/futures/Future.h>
folly::Promise<int> promise;
auto future = promise.getFuture();
std::thread([&] {
promise.setValue(42); // Setting the promise's value
}).detach();
future.wait(); // Wait for the value to be set
std::cout << future.value() << std::endl; // Outputs 42
This demonstrates the creation of a promise, which is resolved in a separate thread, allowing the main thread to wait for the result.

Advanced Folly Features
Asynchronous IO with Folly
Folly introduces powerful asynchronous IO operations through its coroutine support. This functionality is essential for building scalable applications.
Example: Using `folly::coro` for coroutines
#include <folly/Coro.h>
folly::coro::Task<void> myCoroutine() {
int result = co_await folly::coro::blockingWait(folly::coro::co_invoke([]() -> folly::coro::Task<int> {
co_return 1;
}));
std::cout << "Result: " << result << std::endl;
}
This coroutine example demonstrates how to use the library to manage asynchronous operations naturally.
Performance Optimization Tools
Folly is equipped with various profiling and benchmarking tools to help developers optimize their applications.
Example: Using `folly::Benchmark`
#include <folly/Benchmark.h>
void funcToBenchmark() {
// Some code to measure
}
BENCHMARK(funcToBenchmark);
In this segment, a benchmark for `funcToBenchmark` is created, allowing performance metrics to be collected and evaluated.
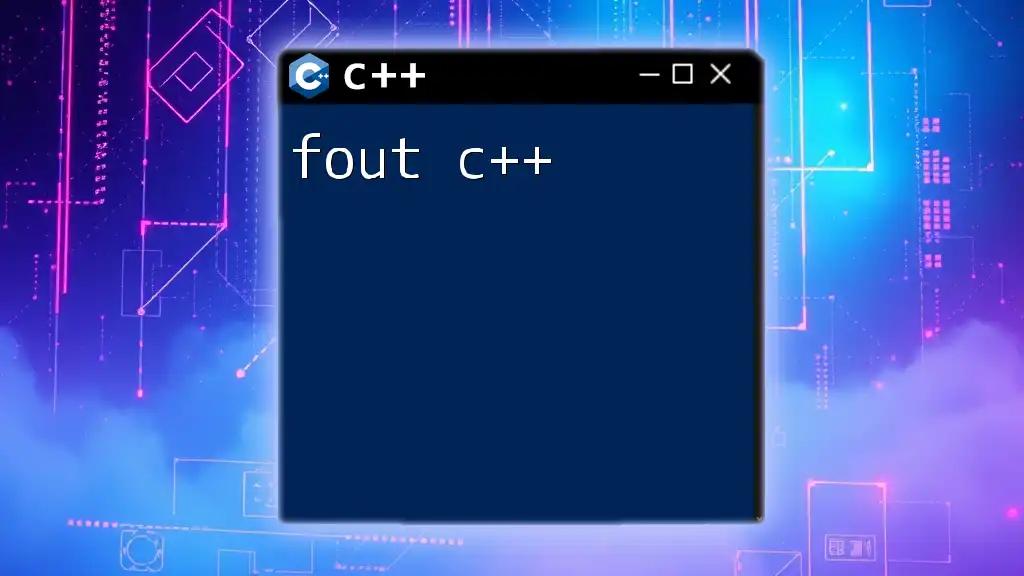
Common Use Cases of Folly
Real-time Data Processing
Folly is extensively used in applications that require real-time data processing. Its data structures and threading capabilities facilitate the development of high-performance streaming applications.
Networking Applications
When building networking applications, Folly’s features cater to the demands of high throughput and low latency. Libraries like Folly are invaluable for developers creating scalable server applications or microservices.

Debugging and Testing with Folly
Debugging Tools
Folly incorporates a range of helpful tools to assist in debugging code. Among these, FOLLY_CHECK is utilized for runtime assertions, enabling developers to catch issues early.
Example: Using `FOLLY_CHECK` for assertions
#include <folly/portability/GMock.h>
#include <folly/portability/GTest.h>
void someFunction(int x) {
FOLLY_CHECK(x != 0, "x must not be zero");
}
By employing assertions like this, developers can enforce assumptions about their code, making it clearer and more resilient.
Testing Framework
Folly provides a robust framework for testing, empowering developers to write comprehensive unit tests for their code.
Code Example: Writing tests in Folly
#include <folly/portability/GMock.h>
#include <folly/portability/GTest.h>
TEST(MyTestSuite, MyTestCase) {
EXPECT_EQ(1 + 1, 2);
}
This basic test checks if the expression is correct, showcasing the straightforwardness of writing tests within the Folly ecosystem.
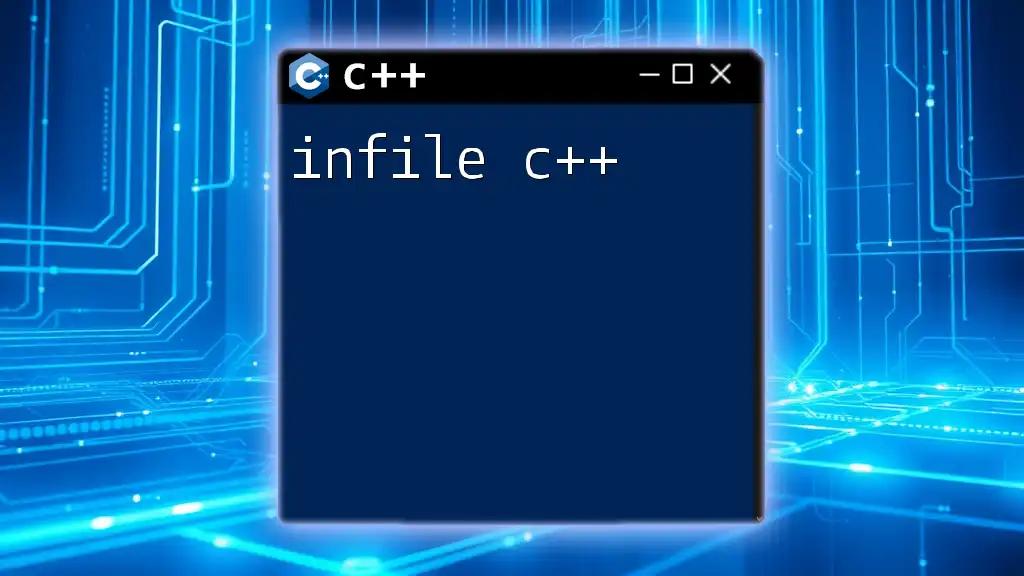
Best Practices and Recommendations
Design Patterns with Folly
When using Folly, consider employing design patterns that align well with its features. Common patterns include the factory pattern for object creation, observer pattern for event management, and the strategy pattern for handling algorithms.
Performance Tuning
To optimize performance in your Folly applications, regularly profile your application, identify bottlenecks, and utilize appropriate Folly features such as its concurrent data structures and asynchronous IO operations.

Conclusion
Folly C++ is undoubtedly a formidable library for C++ developers who seek to build high-performance applications. By incorporating its rich features and adopting best practices, you can unlock new levels of efficiency and reliability within your software. Whether you are dealing with complex data processing or developing responsive networking applications, Folly provides the tools you need to succeed in today’s demanding development landscape.
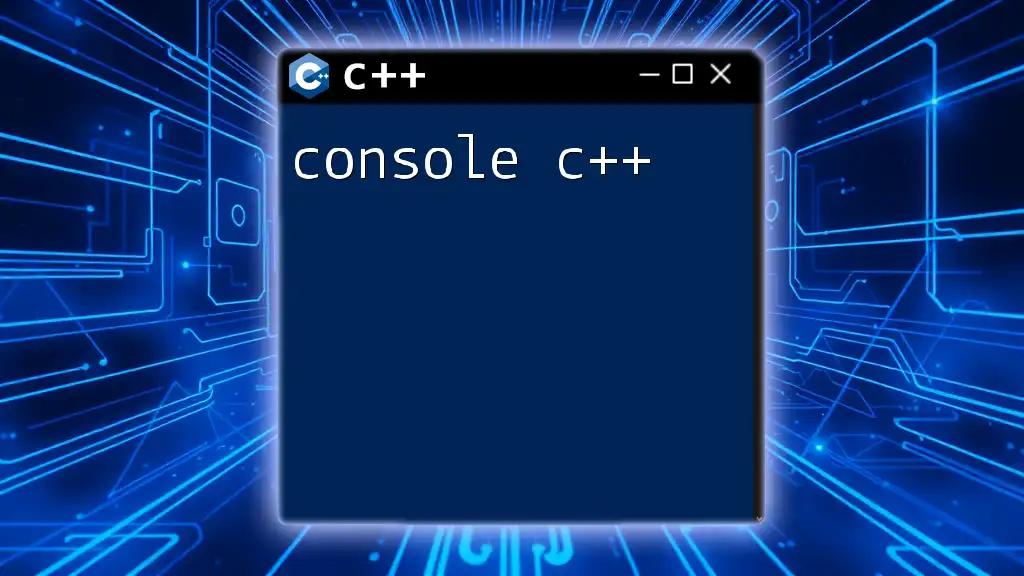
Additional Resources
For further exploration, consider reviewing the official Folly documentation, engaging with forums, or checking GitHub repositories dedicated to Folly. These resources will augment your understanding and ability to leverage the power of Folly in your C++ projects.