In C++, you can pass a pointer to a function to allow the function to modify the value of the variable being pointed to, as shown in the following example:
#include <iostream>
void increment(int* ptr) {
(*ptr)++;
}
int main() {
int value = 5;
increment(&value);
std::cout << "Value after increment: " << value << std::endl; // Output: 6
return 0;
}
Understanding Pointers
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. Pointers are fundamental in C++, allowing for more efficient data manipulation and memory control. They enable developers to directly access and modify the content of memory locations.
Declaring and Initializing Pointers
To work with pointers, you first need to declare them. The syntax is straightforward: you specify the data type followed by an asterisk (*) before the pointer name. For example:
int* ptr; // Declares a pointer to an integer
You can initialize a pointer by assigning it the address of another variable using the address-of operator (&):
int var = 10;
int* ptr = &var; // Pointer initialized to the address of var
In this code, `ptr` now holds the memory address of `var`, allowing you to manipulate `var` through `ptr`.
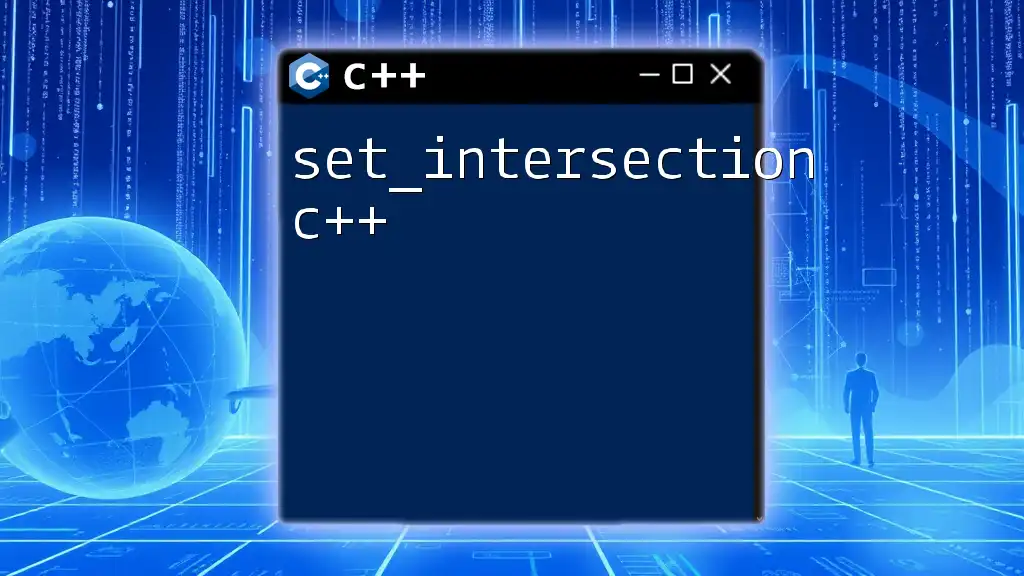
What are Functions in C++?
Definition and Signature of Functions
In C++, a function is a block of code that performs a specific task. Functions are defined with a signature that includes a return type, name, and parameters.
Types of Function Parameters
Functions can accept parameters in various ways, primarily:
- Value parameters: When a function takes a copy of an argument, changes do not affect the original variable.
- Reference parameters: When a function accepts a reference to an argument, any changes will directly affect the original variable.
Passing pointers as parameters is advantageous when you need the function to modify the original data or avoid making copies of large data structures.
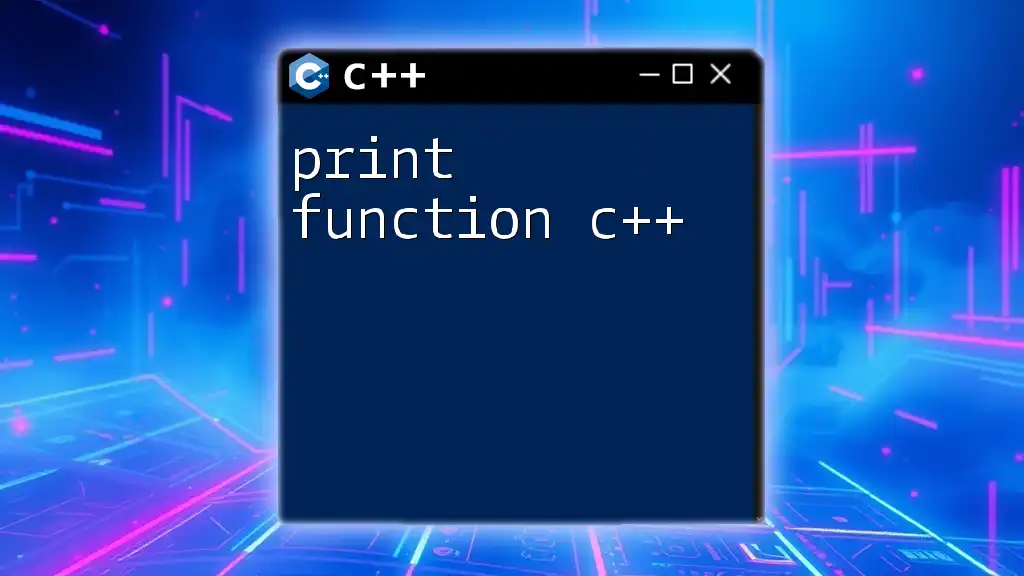
Passing Pointers to Functions
Syntax for Passing Pointers
When you pass a pointer to a function, you allow that function to access and modify the variable it points to. Here’s the syntax:
void exampleFunction(int* ptr) {
*ptr = 20; // Change the value at the memory address to 20
}
Passing Pointers vs Passing by Value
The fundamental difference between passing pointers and passing by value is how the argument is treated inside the function. When you pass by value, a copy of the variable is created. Thus, any modifications inside the function do not reflect back to the original variable. In contrast, passing a pointer allows direct access to the variable’s memory address.
Example of passing by value:
void passByValue(int num) {
num = 30; // Changes only for this function call
}
Example of passing by pointer:
void passByPointer(int* numPtr) {
*numPtr = 30; // Changes will affect the original variable
}
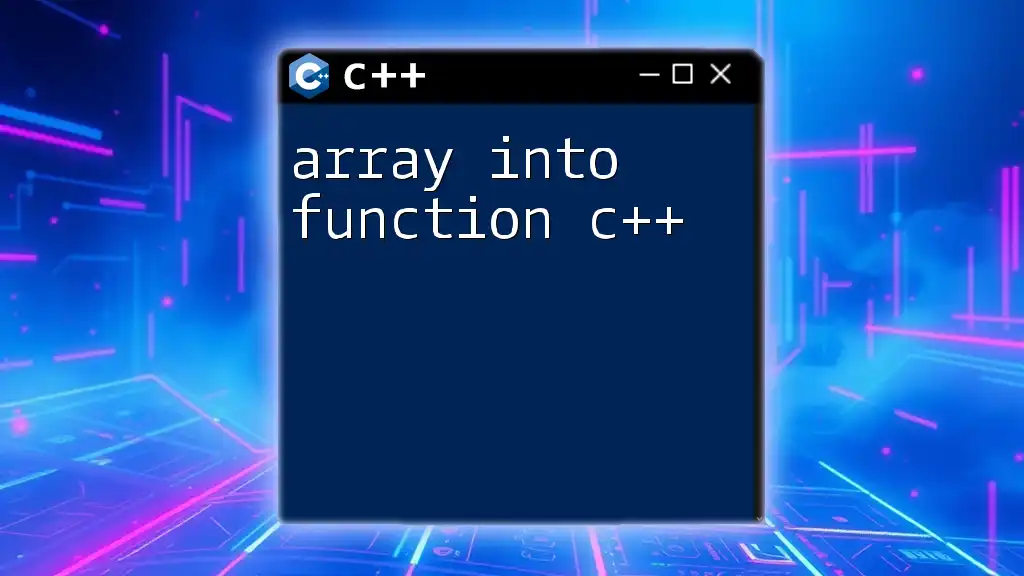
Practical Examples
Example 1: Swapping Two Numbers Using Pointers
A classic example illustrating the power of pointers is swapping two variables. Here's how it's done:
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
In this function, `a` and `b` are pointers to the integers you want to swap. The function will update the values at the respective memory addresses, effectively swapping the values of `a` and `b`.
Example 2: Dynamically Allocating Memory and Passing Pointers
Dynamically allocating memory allows the creation of flexible data structures. Here’s how you might pass a pointer to a dynamically allocated array:
void createArray(int** arr, int size) {
*arr = new int[size]; // Allocate memory for an array
}
In this example, the function `createArray` takes a pointer to a pointer. This allows the function to allocate memory for an array and update the caller's pointer to reference this new memory.
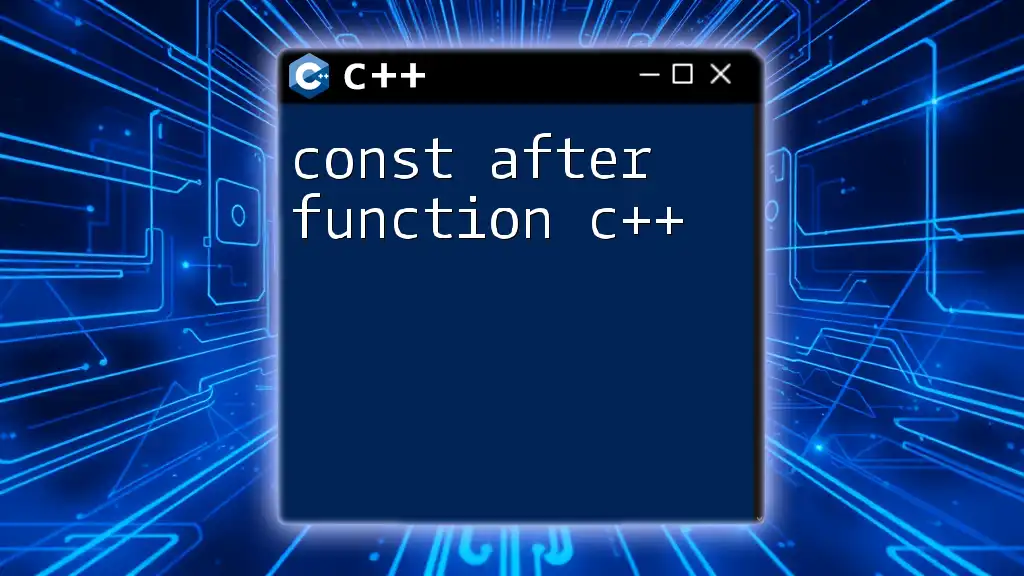
Use Cases for Pointers in Functions
Modifying Original Data
When you need a function to modify a variable directly, passing a pointer is an effective strategy. This direct access avoids the overhead of making copies, particularly for large data types.
Efficient Memory Usage
Pointers enable more efficient memory usage by allowing functions to work with external data without creating multiple copies. This is especially crucial when dealing with large structures or classes, making your code not only efficient but also faster.
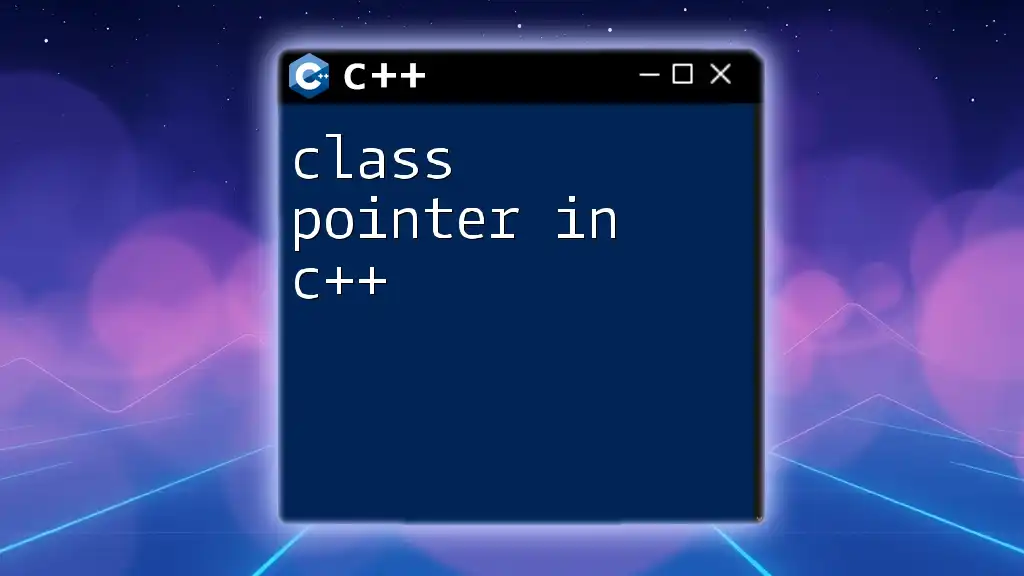
Common Pitfalls and Best Practices
Avoiding Dangling Pointers
A dangling pointer occurs when a pointer still points to a memory location after the memory has been deallocated. To avoid this, set pointers to `nullptr` after freeing memory:
delete[] arr; // Release memory
arr = nullptr; // Avoid dangling pointer
Proper Memory Management
Memory management is essential when working with pointers. Always ensure that every allocation has a corresponding deallocation using `delete` or `delete[]`. This practice helps prevent memory leaks that can degrade performance and lead to crashes.
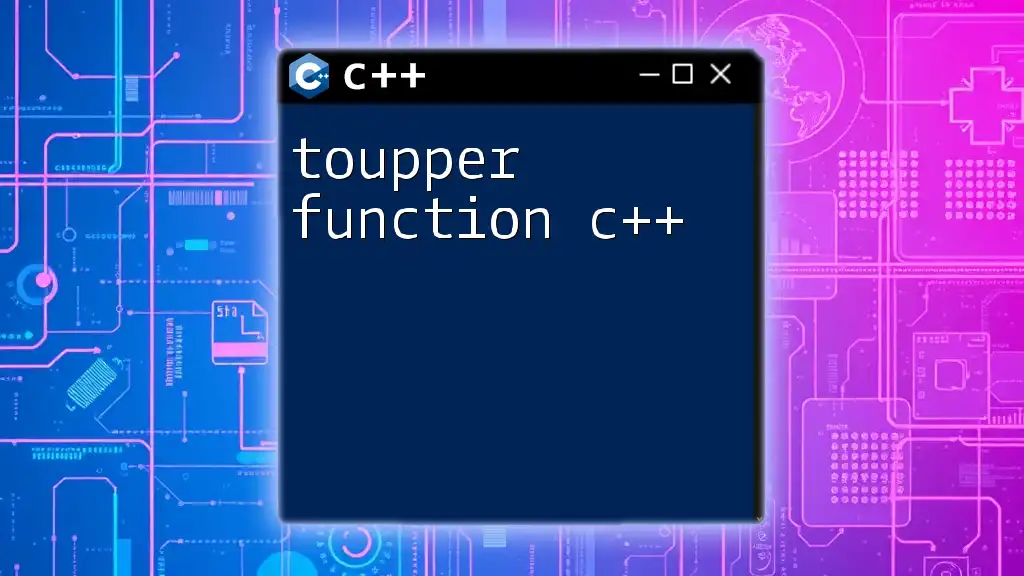
Conclusion
Passing pointers to functions in C++ enhances efficiency and flexibility in your programming. By understanding how pointers work and applying them correctly, you can manipulate data effectively and avoid unnecessary overhead. Experimenting with pointers in your code will deepen your understanding and familiarity with this essential aspect of C++. Now is the time to dive into practice and refine your skills!
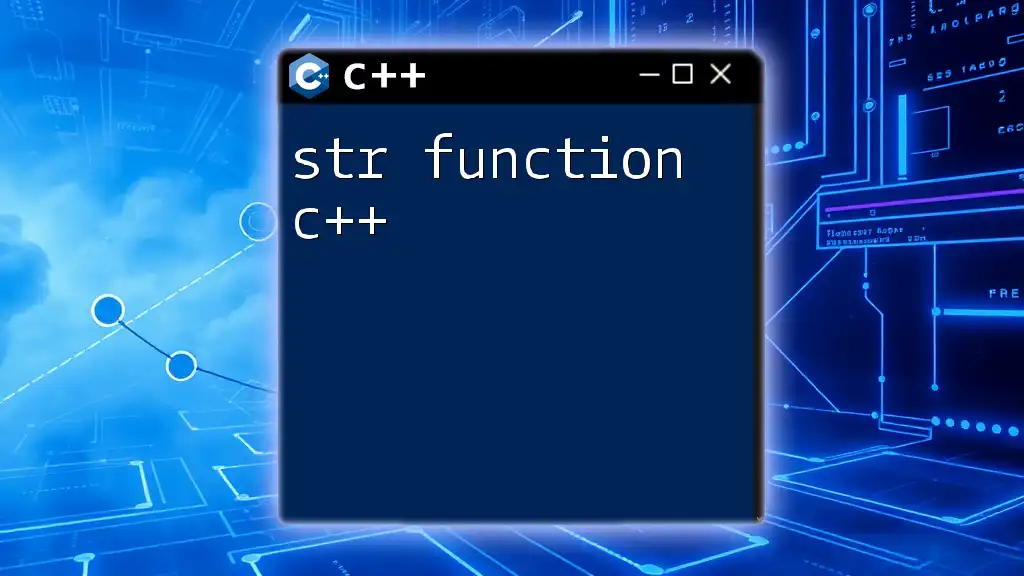
Additional Resources
For further learning, consider exploring online tutorials, coding platforms, and textbooks devoted to C++. Documentation such as The C++ Programming Language by Bjarne Stroustrup and C++ Primer by Lippman et al. can provide comprehensive insights into using pointers and functions effectively.
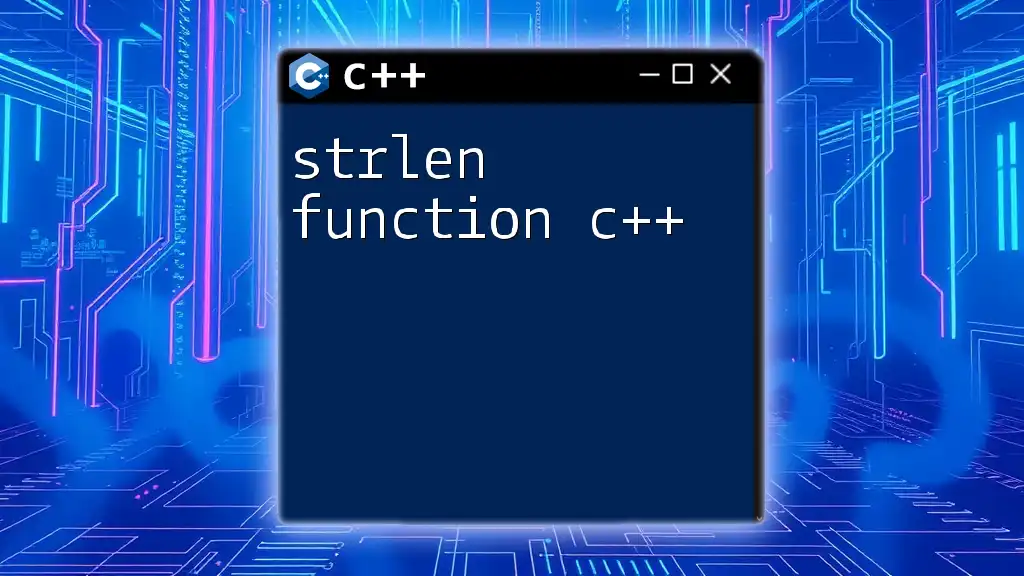
Call to Action
Ready to elevate your C++ skills? Join our program for quick, concise lessons on mastering C++ commands, including pointer manipulation! With our guidance, transform your understanding and application of this powerful programming language.